// JOSEPH ZHANG
// SECTION E
// HAOZHEZ@ANDREW.CMU.EDU
// PROJECT-02
// GLOBAL VARS
var eyeSize = 20;
var faceWidth = 100;
var faceHeight = 150;
var mouthX = 18;
var mouthY = 32;
var mouthPosY = 192;
var bodyW = 150;
var browWeight = 5;
var browHeight = 5;
// CANVAS
function setup() {
createCanvas(640, 480);
}
function draw() {
background('#B4A4D0');
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
// BODY
noStroke();
fill('#EFB616');
ellipse(width/2,height/2 + 170,bodyW,265)
// FACE
fill('#FFD100');
noStroke();
ellipse(width / 2, height / 2, faceWidth, faceHeight);
ellipse(width / 2, height / 2 + 40, faceWidth * 1.2, faceHeight / 1.4);
// EYES
fill('black')
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
// INNER EYE
noStroke();
fill('white')
ellipse(eyeLX, height / 2, eyeSize / 2, eyeSize / 2);
ellipse(eyeRX, height / 2, eyeSize / 2, eyeSize / 2);
// MOUTH
stroke('#871200');
strokeWeight(2);
fill('#FF7D62');
ellipse(width / 2,mouthPosY + 90, mouthX, mouthY);
// BROWS
strokeWeight(browWeight);
// Left Brow
line(eyeLX - 10, height / 2 - 20 + browHeight, eyeLX + 10 , height / 2 - 7);
// Right Brow
line(eyeRX - 7, height / 2 - 10, eyeRX + 10 , height / 2 - 20 + browHeight);
}
function mousePressed() {
// FACE WIDTH + HEIGHT
faceWidth = random(75,150);
faceHeight = random(100,150);
//EYE SIZE
eyeSize = random(10,30);
// MOUTH RATIOS
mouthX = random(22,42);
mouthY = random(22,42);
// BODY WIDTH
bodyW = random(50,180)
//BROW WIDTH + HEIGHT
browWeight = random(3,6);
browHeight = random(-5,5);
}
Varying Faces
To built this project, I experimented a lot with the manipulation of shapes and how to combine them with others to create more interesting forms; the variables I chose to manipulate included, the eye size, eyebrow form, head shape, body width, and mouth. To begin the process, I mocked up what I wanted to make in Adobe Illustrator and from there, adapted to what actually could and couldn’t be done. Unfortunately, I don’t have the Illustrator file anymore so I can’t attach a screenshot. But I did use the surprised Pikachu meme as initial inspiration!
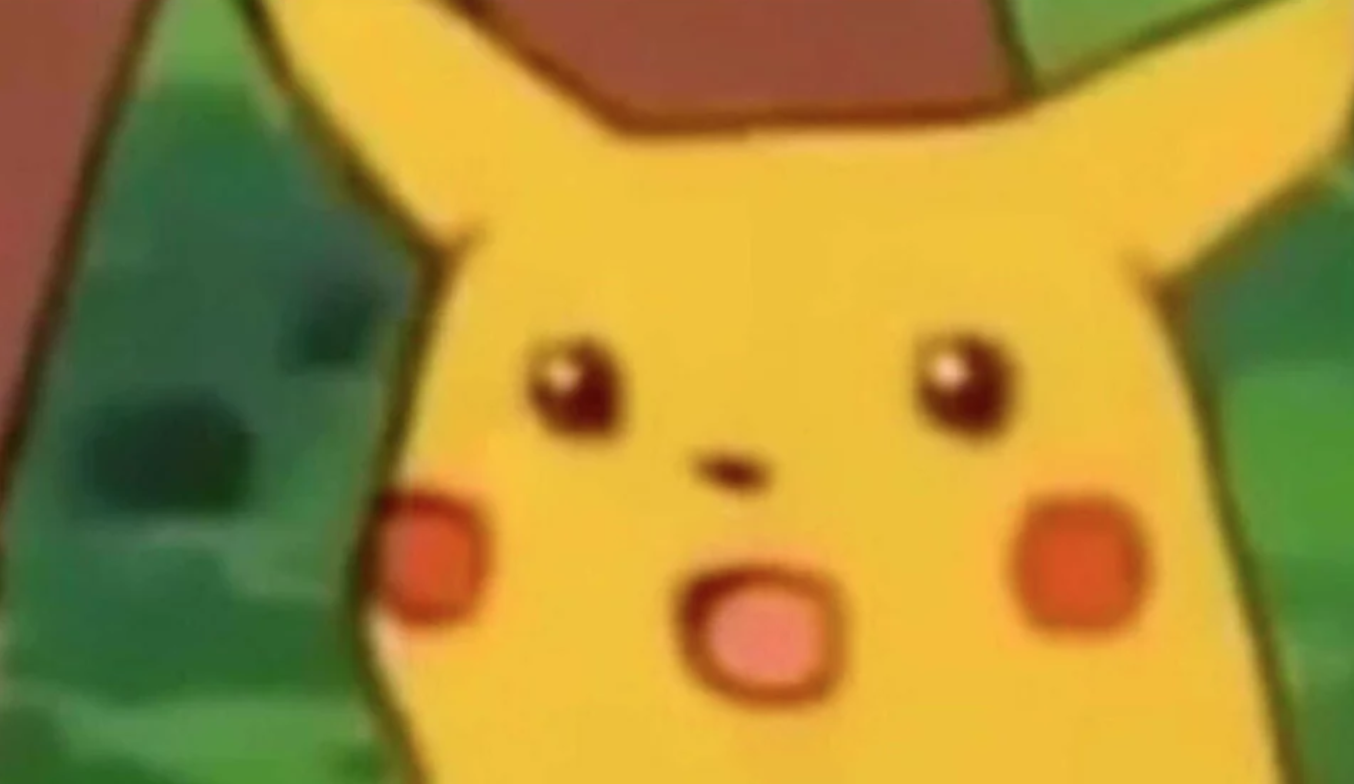