For this project, I wanted to play with hypotrochoid forms and astroids in hopes of making something pretty dynamic. I had really brief understanding of roulettes from high school mathematics so a lot of my learning came from going through the Wolfram Alpha website and learning about each form. At the end, these were the two that I wanted to continue playing with.
Quiet honestly, I had no idea how it would come out at the end and much of the process just came from experimenting with mouse positions, mapping, and tweaking with equations. Below are some screenshots of its different forms.
// Joseph Zhang
// Section E
// haozhez@andrew.cmu.edu
// Project-07: Composition with Curves
var points = 2000;
var rotateCount = 0;
function setup() {
createCanvas(480, 480);
}
// calls each shape and continuously rotates the form
function draw() {
background(20);
push();
translate(width / 2, height / 2);
rotate(rotateCount);
rotateCount++;
drawAstroid();
drawHypo();
pop();
}
// draws the Astroid form (inside shape)
function drawAstroid() {
// colors is dependent on mouse position
colorR = map(mouseY, 0, width, 0, 255);
colorG = map(mouseX , 0, width, 0, 255);
colorB = map(mouseX , 0, width, 0, 255);
stroke(colorR , colorG, colorB);
noFill();
strokeWeight(1);
// for loop to draw astorid form
beginShape();
petal = int(map(mouseX, 0, width, 4, 10));
size = int(map(mouseX, 0, width, 0, 100));
for (var i = 0; i < points; i++){
t = map(i, 0, points, 0, TWO_PI);
x = (size / petal) * ((petal - 1) * cos(t) + cos((petal - 1) * t));
y = (size / petal) * ((petal - 1) * sin(t) - sin((petal - 1) * t));
vertex(x,y);
}
endShape();
}
// draws the hypotrochoid form (outside shape)
function drawHypo() {
// colors is dependent on mouse position
colorR = map(mouseX, 0, width, 0, 255);
colorG = map(mouseY , 0, width, 0, 255);
colorB = map(mouseY , 0, width, 0, 255);
stroke(colorR , colorG, colorB);
strokeWeight(1);
//for loop to draw astroid form
a = map(mouseX, 0 , width, 200, 350);
b = map(mouseY, 0 , width, 100, 200);
h = map(mouseY, 0 , width, 2, 105);
beginShape();
for (var i = 0; i < 10000; i++){
t = map(i, 0, 500, 0, TWO_PI);
x = (a - b) * cos(t) + h * cos((a - b) * (t / b));
y = (a - b) * sin(t) - h * sin((a - b) * (t / b));
vertex(x, y);
}
endShape();
}
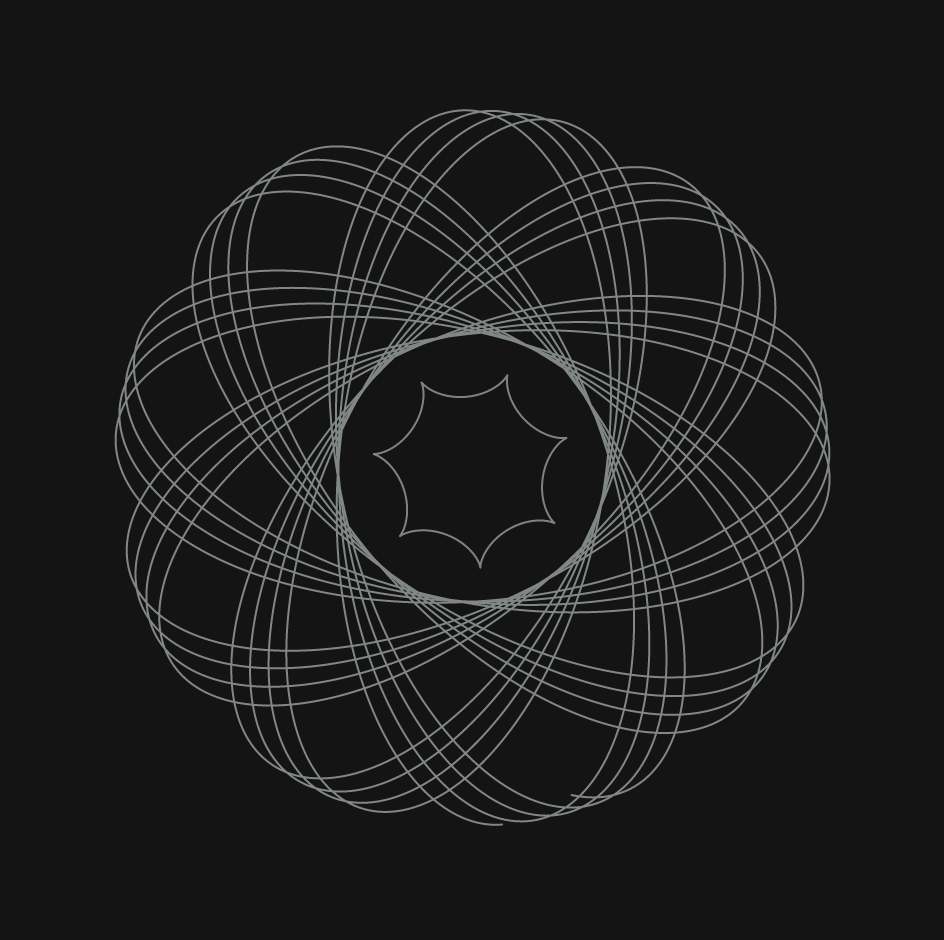
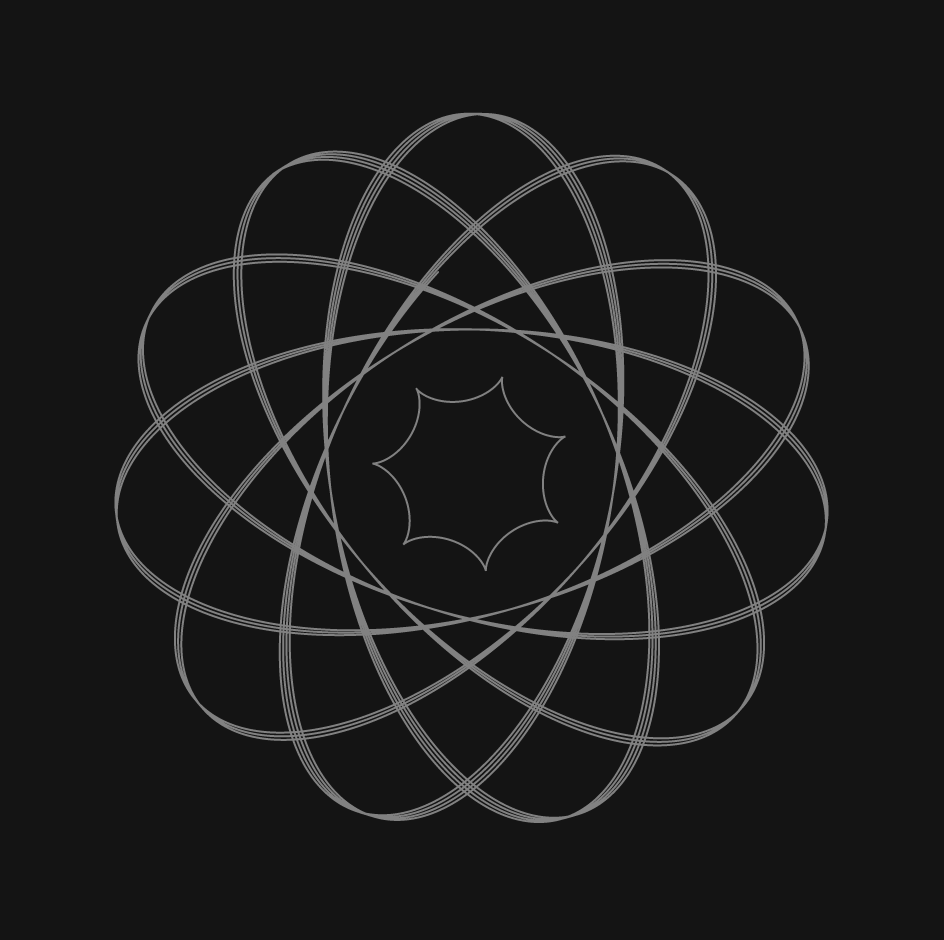
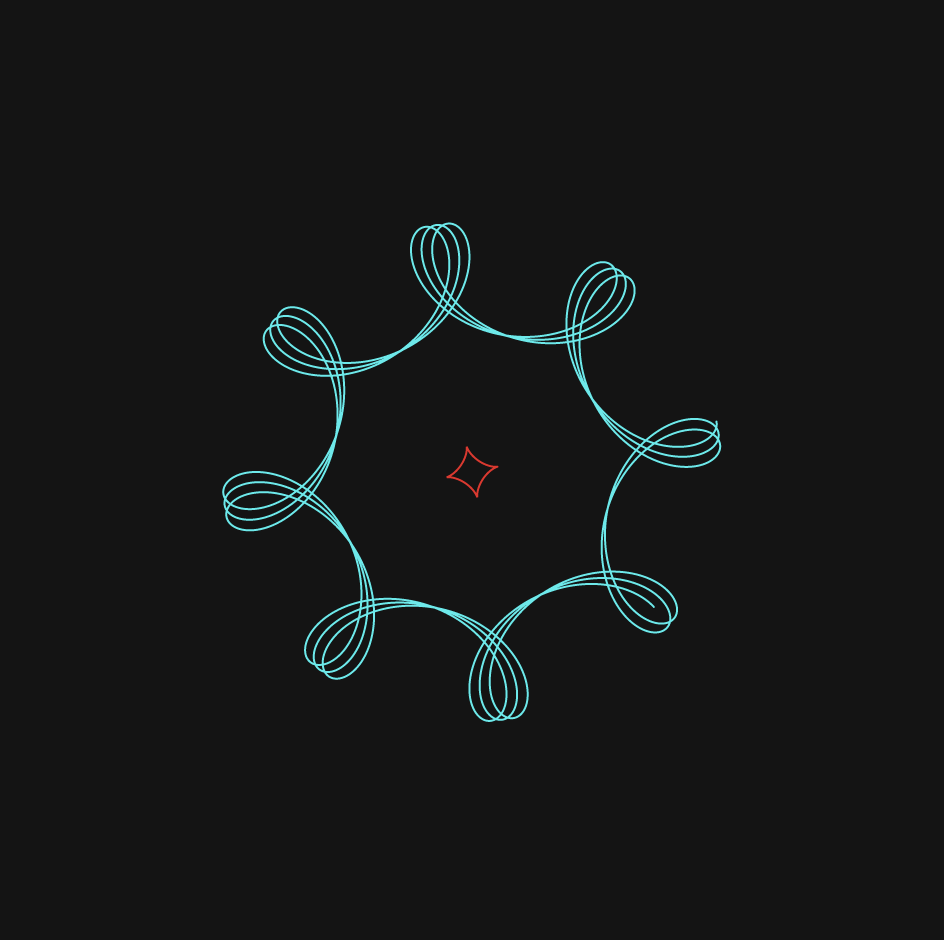
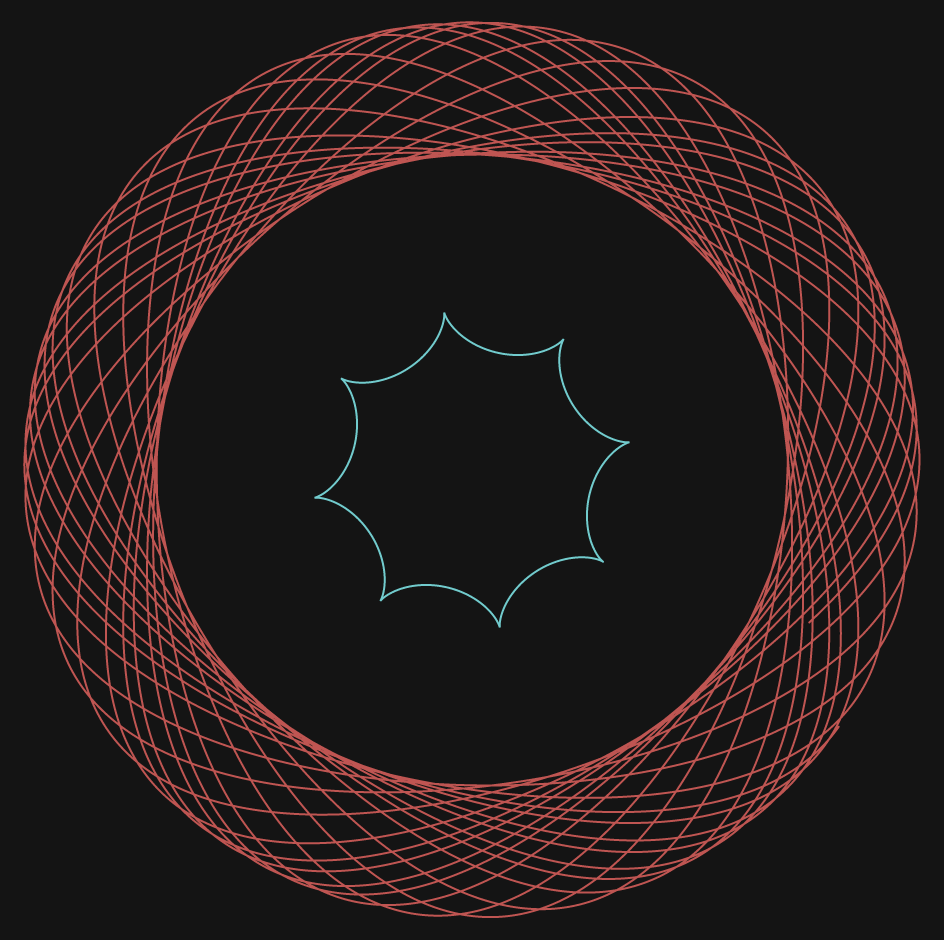