// Joseph Zhang
// Section E
// haozhez@andrew.cmu.edu
// Assignment-06C: Simple Clock
function setup() {
createCanvas(480, 480);
}
//translates everything
function draw() {
// to make sure hour() never goes past 13
var hr = hour();
if(hour() > 12) {
hr = hour() - 12;
}
// push and eventual pop so that I can position the clock
push();
translate(228 + mouseX / 20, 228 + mouseY / 20);
drawClock();
pop();
// shows the time
noStroke();
fill(map(mouseX, 0, width, 150, 255));
text(nf(hr, 2, 0) + ":" + nf(minute(), 2, 0) + ":" + nf(second(), 2, 0), width - 70, height - 30, 100, 30);
}
//draws everything
function drawClock(){
threeSixty = 2 * PI;
background(30);
strokeWeight(0);
// radial grid
for ( i = 1; i < 13; i++) {
stroke(68);
strokeWeight(1);
line(0, 0, 600 * cos(threeSixty * i / 12), 600 * sin(threeSixty * i / 12));
stroke(0);
}
//background circles
stroke(0);
fill(0, 0, 0, 80);
ellipse(0, 0, 300, 300);
ellipse(0, 0, 140, 140);
//displays millisecond (smaller circle)
// stroke(255,255,255, 50);
strokeWeight(2);
ellipse(200 * cos(threeSixty * millis() / 60000 - (PI / 2)), 200 * sin(threeSixty * millis() / 60000 - (PI / 2)), 30, 30);
ellipse(-200 * cos(threeSixty * millis() / 60000 - (PI / 2)), -200 * sin(threeSixty * millis() / 60000 - (PI / 2)), 30, 30);
// displays minutes (bigger circle)
strokeWeight(2);
stroke(255,255,255, 190);
line(70 * cos(threeSixty * minute() / 60 - (PI / 2)),
70 * sin(threeSixty * minute() / 60 - (PI / 2)),
700 * cos(threeSixty * minute() / 60 - (PI / 2)),
700 * sin(threeSixty * minute() / 60 - (PI / 2)));
line(-70 * cos(threeSixty * minute() / 60 - (PI / 2)),
-70 * sin(threeSixty * minute() / 60 - (PI / 2)),
-700 * cos(threeSixty * minute() / 60 - (PI / 2)),
-700 * sin(threeSixty * minute() / 60 - (PI / 2)));
fill(0,0,0,255);
ellipse(70 * cos(threeSixty * minute() / 60 - (PI / 2)), 70 * sin(threeSixty * minute() / 60 - (PI / 2)), 50, 50);
ellipse(-70 * cos(threeSixty * minute() / 60 - (PI / 2)), -70 * sin(threeSixty * minute() / 60 - (PI / 2)), 50 , 50);
strokeWeight(1);
//displays seconds (smaller circle)
stroke(255, 255, 255, 100);
strokeWeight(2);
line(150 * cos(threeSixty * second() / 60 - (PI / 2)),
150 * sin(threeSixty * second() / 60 - (PI / 2)),
500 * cos(threeSixty * second() / 60 - (PI / 2)),
500 * sin(threeSixty * second() / 60 - (PI / 2)));
line(-150 * cos(threeSixty * second() / 60 - (PI / 2)),
-150 * sin(threeSixty * second() / 60 - (PI / 2)),
-500 * cos(threeSixty * second() / 60 - (PI / 2)),
-500 * sin(threeSixty * second() / 60 - (PI / 2)));
ellipse(150 * cos(threeSixty * second() / 60 - (PI / 2)), 150 * sin(threeSixty * second() / 60 - (PI / 2)), 30, 30);
ellipse(-150 * cos(threeSixty * second() / 60 - (PI / 2)), -150 * sin(threeSixty * second() / 60 - (PI / 2)), 30, 30);
//displays hour
strokeWeight(2);
stroke(255, 255, 255, 230);
line(0, 0,
30 * cos(threeSixty * (hour() / 12 + minute() / 720) - (PI / 2)),
30 * sin(threeSixty * (hour() / 12 + minute() / 720) - (PI / 2)));
ellipse(0,0,10,10);
}
For this abstract clock, I really wanted to do something that grow out radially and moves in proportion to its time sequencing. I wanted to build something that gives the resemblance of a clock but doesn’t necessarily function entirely as one. This prompted me to utilize ellipses and to move them around the screen depending on their associated time measurement.
The wider you move out the ring, the more micro the time gets. The innermost ring represents hour while the black circle on the outside represents milliseconds. As you move from innermost ring to outermost ring, the opacity getter lighter and lighter until it becomes black (millisecond).
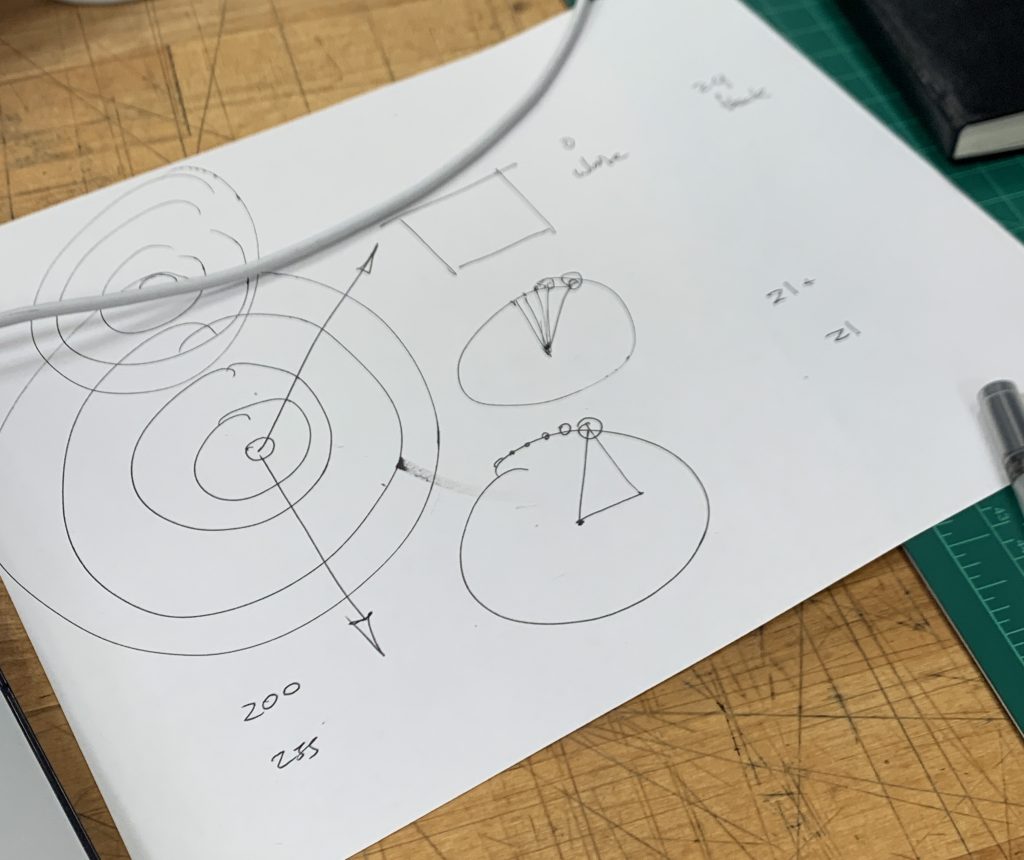