//Minjae Jeong
//Section B
//minjaej@andrew.cmu.edu
//Project-07
function setup() {
createCanvas(480, 480);
background('black');
}
function draw() {
push();// draw in the middle
translate(width / 2, height / 2);
drawHypotrochoid();
pop();
}
//Equations from http://mathworld.wolfram.com/Hypotrochoid.html
function drawHypotrochoid() {
var x;
var y;
var a1 = mouseX; //move mouse to control hypotrochoids drawing
var a2 = mouseY;
var h = 5;
beginShape(); //draw hypotrochoid
for (i = 0; i < 480; i += 5){
var t = map(i, 0, 200, 0, width);
x = (a1- a2) * cos(t) + h * cos(t * (a1 - a2) / a2);
y = (a1- a2) * sin(t) - h * sin(t * (a1 - a2) / a2);
vertex(x, y);
}
endShape();
}
This simple looking black and white drawing with hypotrochoids attracted me more than other colorful tries in many reasons. First of all, the first impression is very wild, and simple. With the mouse cursor starting at the middle of the canvas (240, 240), the canvas starts black, and creates white spaces as the mouse moves. The fun part is, the background will never be pitch black once you move your mouse.
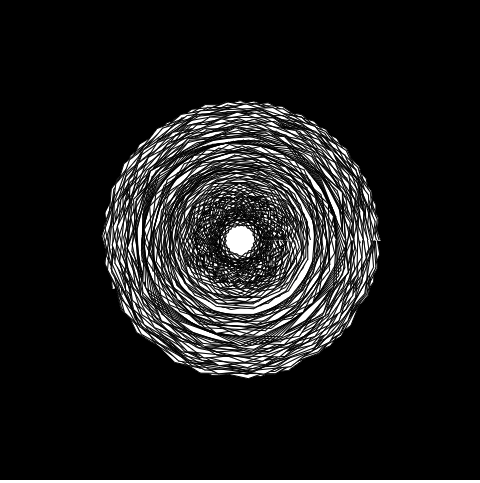
The mouse movement is sensitive, the drawing can change in many ways according to the user control. Although it can’t go full black unless refreshing the page, the drawing can go all-white which can reset the drawing.
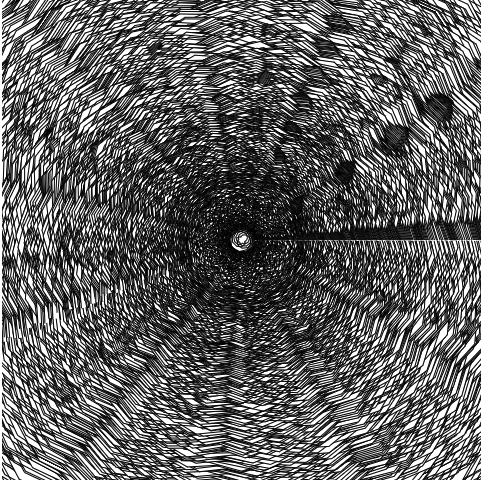
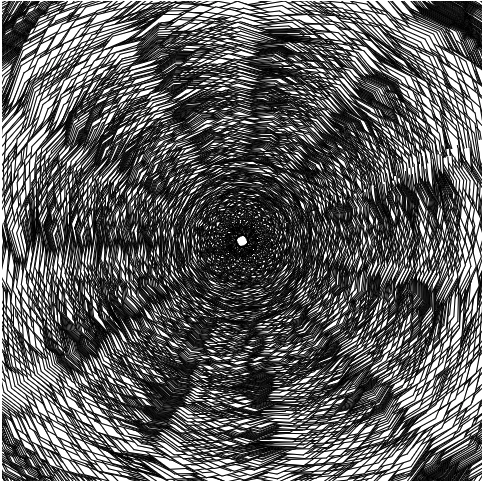
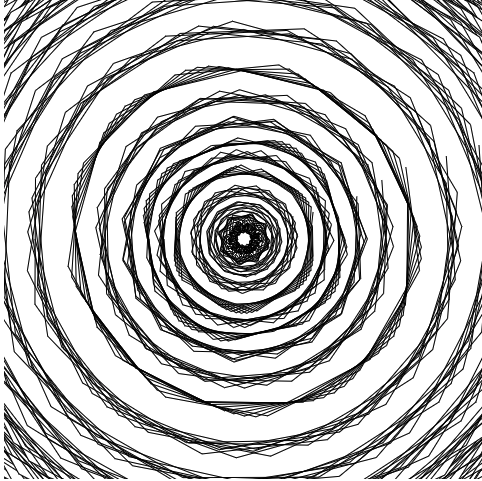