//Siwei Xie
//Section B
//sxie1@andrew.cmu.edu
//project-07
var nPoints = 500;
var t;
var angle = 0;
var adj = 2;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(220);
noStroke();
//draw Quadrifolium with variant colors
push();
fill(mouseX, mouseY, 80);
translate(mouseX, mouseY);
rotate(radians(angle));
drawQuadrifolium();
angle = (angle + adj) % 360;
pop();
//draw Epitrochoid with variant colors
push();
fill(80, mouseX, mouseY);
translate(200, 200);
drawEpitrochoid();
pop();
}
//draw rotating & moving windmill
function drawQuadrifolium() {
var a = 70;
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
//http://mathworld.wolfram.com/Quadrifolium.html
r = a * sin(2 * t);
x = r * cos(t);
y = r * sin(t);
vertex(x, y);
}
endShape(CLOSE);
}
//draw variant curve in the middle
function drawEpitrochoid(){
var a1 = 40;
var b1 = 200;
var h = constrain(mouseY/ 10.0, 0, b1);
var t;
var r = map(mouseX, 0, 600, 0, 1);
beginShape();
fill(200, mouseX, mouseY);
for (var i = 0; i < nPoints; i++) {
stroke("black");
vertex(x, y);
var t = map(i, 0, nPoints, 0, TWO_PI * 2); // mess with curves within shapes
//Parametric equations for Epitrochoid Curve
x = r* ((a1 + b1) * cos(t) - h * cos((t * (a1 + b1) / b1) + mouseX));
y = r* ((a1 + b1) * sin(t) - h * sin((t * (a1 + b1) / b1) + mouseX));
}
endShape(CLOSE);
}
I had fun creating this image. I used Quadrifolium and Epitrochoid in my drawing, and adjusting them according to the position of mouse.
Quadrifolium is a type of rose curve with n=2. It has the polar equation. Epitrochoid is a roulette traced by a point attached to a circle of radius r rolling around the outside of a fixed circle of radius R, where the point is at a distance d from the center of the exterior circle.
By modifying the limits of parameters and changing value of parameters in the formula, I was able to play with the curves.
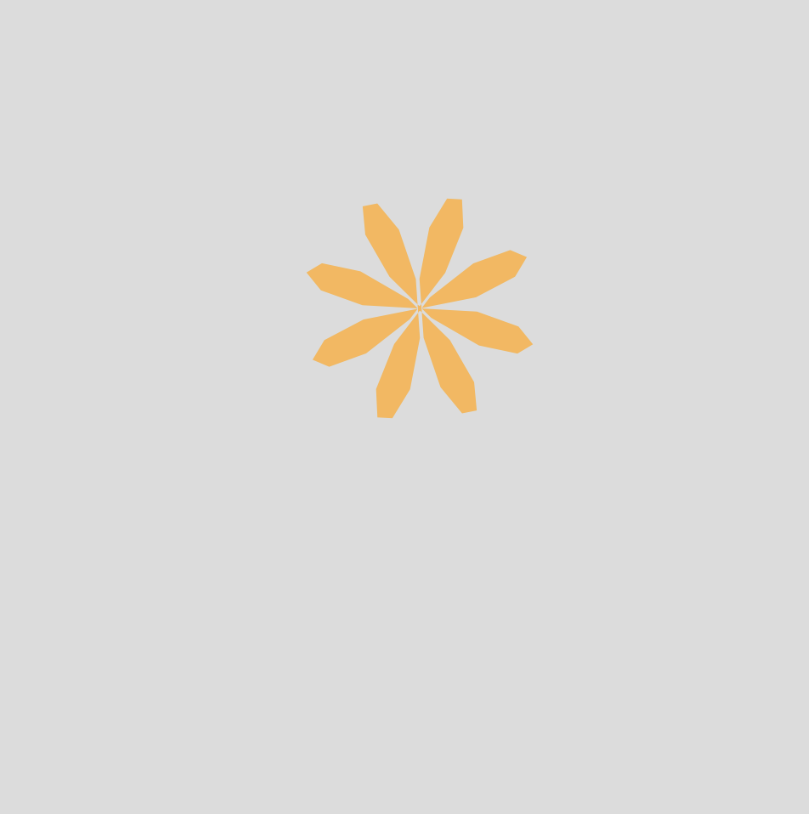