//Sarah Choi
//Section D
//sychoi@andrew.cmu.edu
//Project-07
var col;
function setup() {
createCanvas(400, 400);
}
function draw() {
background(0);
//randomize color
col = color(random(255), random(255), random(255));
x = constrain(mouseX, 0, width);
y = constrain(mouseY, 0, height);
// draw the frame
fill(255);
noStroke();
text("The Butterfly", 20, 40);
stroke(255);
noFill();
rect(0, 0, width-1, height-1);
// draw the curve, rotate on mouseX
push();
translate(width / 2, height / 2);
rotate(map(mouseX, 0, width, 0, 1));
drawButterfly();
pop();
}
//--------------------------------------------------
function drawButterfly() {
// Butterfly Curve:
// http://mathworld.wolfram.com/ButterflyCurve.html
//adding variability and movement
var m = (map(x, 0, mouseY, 0, width));
var m = (map(y, 0, mouseX, 0, height));
stroke(255);
fill(col);
beginShape();
for (var i = 0; i < 300; i++) {
var t = map(i, 0, 300, 0, 2 * TWO_PI);
//butterfly curve equations
r = (exp(cos(t))) - (2 * cos(4 * t)) + (sin((1 / 12) * t));
x = r * sin(t) * m
y = r * cos(t) * m
vertex(x, y)
}
endShape(CLOSE);
}
I chose a curve that could turn on an angle. Thinking of fully randomizing and creating something abstract on the basis of mathematics was my main goal for this project. Because we have been focusing and reflecting on computational art, I wanted to be able to portray this with my own work. It was interesting to play around with the variables as they made a big difference in how the curve was portrayed.
Below, I have shown three different aspects of the curve showing the beginning and how it manifests as it follows the x and y coordinates of the mouse. I wanted to show portray nature by showing the beginning of a butterfly as pure blackness. As one moves the mouse, the shapes get bigger with colors constantly changing showing the beauty butterflies bring into our world. As the mouse moves more across the screen, the butterfly gets bigger to depict what the human eye takes in as a butterfly flies closer to him or her.

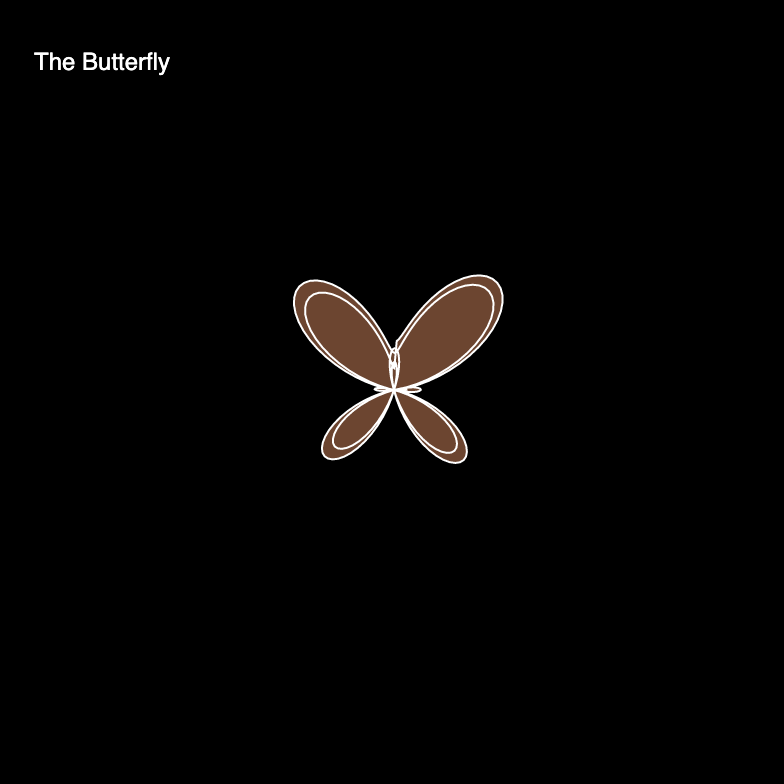
