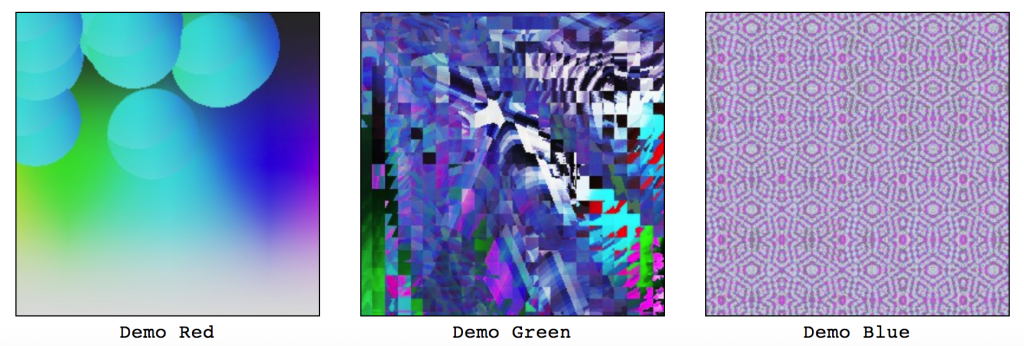
http://www.random-art.org/about/
The Random Art Generator is a free to download python program which is why I was initially interested in this project as i’ve frequently used python for a lot of my work in the past. According to the site, the picture name is the seed and is used for a “random number generator” (nothing is truly random) which constructs a mathematical formula. The formula determines the color of each pixel in the picture. The same name always determines the same sequence of random choices, and consequently the same formula and the same picture.
I appreciate the thought of using a random number generator to create completely new and cool pieces of art. Although random number generators aren’t actually “random”, it just shows the endlessness of possibilities you can make with just a bunch of pixels and changing their colors.