// Your name: Ashley Su
// Your andrew ID: ashleysu
// Your section (C):
var Man;
var grass = 0.4;
var grassSpeed = 0.07;
var mountainFront = 0.008;
var mountainFrontSpeed = 0.0005;
var mountainBack = 0.01;
var mountainBackSpeed = 0.0002;
var bubbles = [];
function setup() {
createCanvas(480, 480);
frameRate(20);
// setting up bubble array
for (var i = 0; i < 9; i++){
var bubblesX = random(width);
var bubblesY = random(0, 200);
bubbles[i] = makebubbles(bubblesY, bubblesX);
}
}
function draw(){
background(221,191,218);
Sun();
MountainBack();
MountainFront();
Grass();
image(Man,mouseX,300,80,80);
updatebubbles();
addbubbles();
}
// draw sun
function Sun(){
noStroke();
fill(250,70,22,120);
ellipse(350, 200, mouseX+500);
fill(250,70,22,40);
ellipse(350, 200, mouseX+350);
fill(250,70,22,100);
ellipse(350, 200, mouseX+200);
}
//pizza man loading
function preload(){
Man = loadImage("https://i.imgur.com/9AUtJm7.png");
}
//draws first mountain
function MountainBack(){
noStroke();
fill(0,109,87);
beginShape();
for (var i = 0; i < width; i ++){
var x = (i * mountainBack) + (millis() * mountainBackSpeed);
var y = map(noise(x), 0, 1.5, 50, 300);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
// drawing second mountain in front of first mountain
function MountainFront(){
noStroke();
fill(208,240,192);
beginShape();
for (var i = 0; i < width; i ++){
var x = (i * mountainFront) + (millis() * mountainFrontSpeed);
var y = map(noise(x), 0, 1.2, 150, 250);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
// draws the grass
function Grass(){
noStroke();
fill(156,176,113);
beginShape();
for (var i = 0; i < width; i ++){
var x = (i * grass) + (millis() * grassSpeed);
var y = map(noise(x), 0, 1.1, 350, 370);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
//constructing the bubbles as objects
function makebubbles(bubblesX, bubblesY){
var bubbles = {
x: bubblesX,
y: bubblesY,
velocity: random(3, 8),
size: random(5, 15),
move: movebubbles,
show: showbubbles,
}
return bubbles;
}
// defining the speed of bubbles
function movebubbles(){
this.x -= this.velocity;
this.y -= this.velocity / random(5, 20);
}
// defning bubbles characteristics
function showbubbles(){
strokeWeight(1);
stroke('LightBlue');
fill(101,208,255,80);
circle(this.x, this.y, this.size);
curve(this.x + this.size, this.y, this.size, this.size/2);
}
//telling bubbbles to move on the screen
function updatebubbles(){
for(i = 0; i < bubbles.length; i++){
bubbles[i].move();
bubbles[i].show();
}
}
// making bubbles contiuesly move accross the screen
function addbubbles(){
if (random(0,2) < 0.5){
var bubblesX = width;
var bubblesY = random(0, height/2);
bubbles.push(makebubbles(bubblesX, bubblesY));
}
}
Category: Project-11-Landscape
Project 11 – Airplane and parachute
/* Jiayi Chen
jiayiche Section A */
//arrays for display
var birds=[];
var clouds=[];
var flyObject =[];
//array for image storage
var birdsImg=[];
var cloudsImg=[];
var flyObjectImg =[];
//array for links
var cloudLinks = [
"https://i.imgur.com/suIXMup.png",
"https://i.imgur.com/7ZHCI63.png",
"https://i.imgur.com/EFb0w3u.png",
"https://i.imgur.com/PxLKy41.png"];
var birdLinks = [
"https://i.imgur.com/Gr1VTU5.png",
"https://i.imgur.com/EmRp42l.png",
"https://i.imgur.com/vLWSU4h.png",
"https://i.imgur.com/Y9BecjA.png"];
var flyObjectLink = [
"https://i.imgur.com/IXz53Lj.png",
'https://i.imgur.com/UsKzDwg.png'];
//load the images
function preload(){
airplane = loadImage("https://i.imgur.com/bEPeF8a.png");
for (var i = 0; i < cloudLinks.length; i++){
cloudsImg[i] = loadImage(cloudLinks[i]);
}
for (var i = 0; i < birdLinks.length; i++){
birdsImg[i] = loadImage(birdLinks[i]);
}
for (var i = 0; i < flyObjectLink.length; i++){
flyObjectImg[i] = loadImage(flyObjectLink[i]);
}
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
//initial clouds
for (var i = 0; i < 3; i++){
var rc = floor(random(cloudsImg.length));
clouds[i] = makeCloud(random(width),random(100,300),random(height),cloudsImg[rc]);
}
frameRate(10);
}
function draw() {
background(135,206,235);
sun()
updateAndDisplayClouds();
addNewobjectsWithSomeRandomProbability();
removeObjectsThatHaveSlippedOutOfView();
image(airplane,200,constrain(250+random(-5,5),240,260),200,200);
}
function sun(){
push();
fill('orange');
circle(60,60,50);
fill('red');
circle(60,60,40);
}
function updateAndDisplayClouds(){
// Update the clouds's positions, and display them.
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].display();
}
// Update the birds's positions, and display them.
for (var i = 0; i < birds.length; i++){
birds[i].move();
birds[i].display();
}
// Update the flyThings's positions, and display them.
for (var i = 0; i < flyObject.length; i++){
flyObject[i].move();
flyObject[i].display();
}
}
//remove out of sight things
function removeObjectsThatHaveSlippedOutOfView(){
//remove out of sight clouds
var cloudsToKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].size/2 > 0) {
cloudsToKeep.push(clouds[i]);
}
}
clouds = cloudsToKeep; // remember the surviving clouds
//remove out of sight birds
var birdsToKeep = [];
for (var i = 0; i < birds.length; i++){
if (birds[i].x + birds[i].size/2 > 0){
birdsToKeep.push(birds[i]);
}
}
birds = birdsToKeep; // remember the surviving birds
//remove out of sight fly things
var FlyesToKeep = [];
for (var i = 0; i < flyObject.length; i++){
if (flyObject[i].x + flyObject[i].size/2 > 0) {
FlyesToKeep.push(flyObject[i]);
}
}
flyObject = FlyesToKeep; // remember the surviving fly things
}
function addNewobjectsWithSomeRandomProbability() {
// With a low probability, add a new clouds to the end.
var newCloudLikelihood = 0.02;
if (random(0,1) < newCloudLikelihood) {
var rc = floor(random(cloudsImg.length));
clouds.push(makeCloud(width+75,random(100,150),random(height),cloudsImg[rc]));
}
// With a low probability, add a new birds to the end.
var newbirdLikelihood = 0.03;
if (random(0,1) < newbirdLikelihood) {
var rc = floor(random(birdsImg.length));
clouds.push(makeBirds(width+30,random(30,60),random(height),floor(random(birdsImg.length))));
}
// With a low probability, add a new flying things to the end.
var newObejctLikelihood = 0.01;
if (random(0,1) < newObejctLikelihood) {
var rc = floor(random(flyObjectImg.length));
clouds.push(makeFly(width+50,random(50,100),random(0,240),flyObjectImg[rc]));
}
}
//make clouds as objects
function makeCloud(birthLocationX,cs,ch,cloudCartoon) {
var cldg = {x: birthLocationX,
size: cs,
y:ch,
speed: -1.0,
cartoon:cloudCartoon,
move: cloudMove,
display: cloudDisplay}
return cldg;
}
function cloudMove() {//move clouds
this.x += this.speed;
}
function cloudDisplay(){
image(this.cartoon, this.x, this.y,this.size, this.size);
}
//make birds as objects
function makeBirds(birthLocationX,cs,ch,birdCartoon) {
var mb = {x: birthLocationX,
size: cs,
y:ch,
speed: -3.0,
cartoonNumber:birdCartoon,
move: birdsMove,
display: birdsDisplay}
return mb;
}
function birdsMove() {
if(this.cartoonNumber == 0 || this.cartoonNumber == 1){
this.x += this.speed/2; //birds facing to the left are flying slower
this.y += random(-3,3); //randomly fly
}else{
this.x += this.speed;
this.y += random(-5,5); //randomly fly
}
}
function birdsDisplay(){
image(birdsImg[this.cartoonNumber], this.x, this.y,this.size, this.size);
}
//make other flying things as objects
function makeFly(birthLocationX,cs,ch,flyCartoon) {
var mf = {x: birthLocationX,
size: cs,
y:ch,
speed: -2.0,
cartoon:flyCartoon,
move: flyMove,
display: flyDisplay}
return mf;
}
function flyMove() {//move flying objects
this.x += this.speed;
//gravity for things without wings
this.y -=this.speed/4;
}
function flyDisplay(){
image(this.cartoon, this.x, this.y,this.size, this.size);
}
Project 11
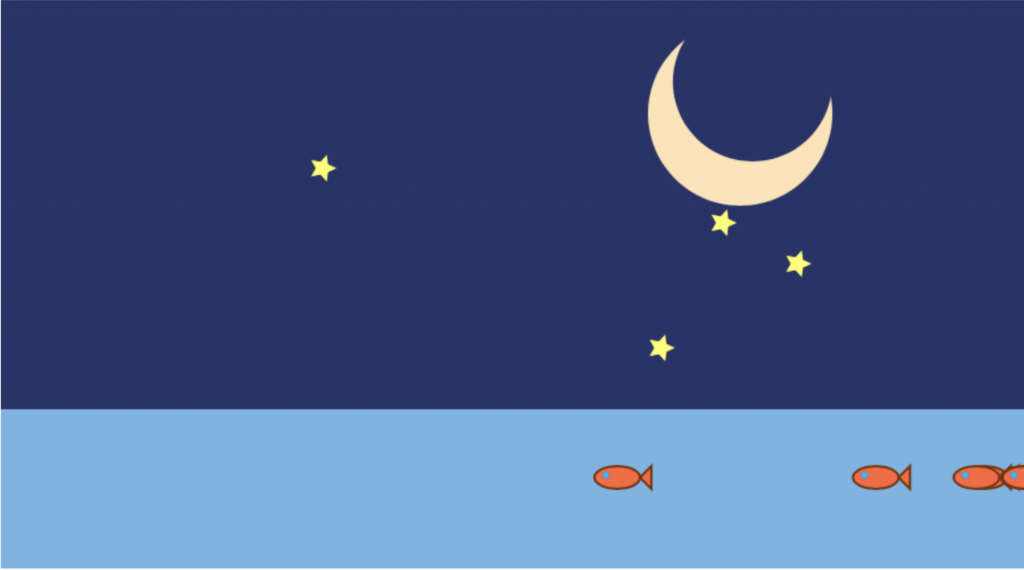
//stars and sea
var stars =[]
var fish =[]
//object,flying fish
var flyfish
var x=350
var y=200
var dx;
var c;
function setup() {
createCanvas(450, 250);
//stars in the sky created
for (var i = 0; i < 7; i++){
var rx = random(width);
stars[i] = makeStars(rx);
}
//fish in the ocean created
for (var i = 0; i < 10; i++){
var rx = random(width);
fish[i] = makeFish(rx);
}
//framerate
frameRate(10);
}
function draw() {
background(40,50,105)
//moon in the sky spinning
push()
translate(325,50)
stroke(255,228,181)
fill(255,228,181)
ellipse(0,0,80,80)
pop()
push()
strokeWeight(0)
translate(325,50)
rotate(frameCount / -100.0);
fill(40,50,105)
ellipse(0,15,70,70)
pop()
//the ocean
push();
strokeWeight(0)
fill(116,180,230);
rect(0,180,width, height);
pop();
//moving star and cloud
loadStar()
addStars()
clearStars()
//moving fish
loadFish()
clearFish()
addFish();
}
Project 11 – Generative Landscape
A ferris wheel standing by the beach:
//Angela Yang
//Section C
var myFerris;
var mySeat = [];
var angle = 0;
var cloudx=200
var cloudy=50
var clouddx = 1;
var cloudx2 = 100;
var cloudy2 = 200;
var clouddx2 = 0.3;
function setup(){
createCanvas(480, 480);
angleMode(DEGREES);
var bc = color(random(0, 255), random(0, 255), random(0, 255));
myFerris = makeFerris(240+180, 230, 0.3, 330);
for(var i = 0; i<6; i++){
var bc = color(random(0, 255), random(0, 255), random(0, 255));
mySeat[i] = makeSeat(200, 200, 20, bc, i);
}
}
function updateFerris(){
this.angle += this.angleSpeed;
}
function ferrisDraw(){
push();
noFill();
strokeWeight(4);
ellipse(this.x, this.y, this.r, this.r);
pop();
push();
stroke(255);
strokeWeight(8);
line(this.x, this.y, this.x-80, height);
line(this.x, this.y, this.x+80, height);
pop();
push();
translate(this.x, this.y);
for(var i = 0; i<6; i++){
push();
rotate(i*60 + this.angle);
stroke(255);
strokeWeight(3);
line(0, 0, this.r/2, 0);
pop();
}
pop();
}
function makeFerris(wx, wy, as, wr){
var wheel = {x:wx, y:wy, angleSpeed:as, r:wr, angle:0,
update: updateFerris,
draw: ferrisDraw};
return wheel;
}
function updateSeat(){
this.x = myFerris.x + myFerris.r/2*cos(this.index*60 + myFerris.angle);
this.y = myFerris.y + myFerris.r/2*sin(this.index*60 + myFerris.angle);
}
function seatDraw(){
push();
stroke(255);
strokeWeight(2);
line(this.x, this.y, this.x, this.y+10);
pop();
push();
stroke(255);
fill(this.c);
rect(this.x-10, this.y+10, 25, 25);
pop();
}
function makeSeat(bx, by, br, bc, seatIndex){
var basket = {x:bx, y:by, r:br, c:bc, index:seatIndex,
update: updateSeat,
draw: seatDraw};
return basket;
}
function draw(){
background(240,145,110);
//Ground
push();
fill(87,71,70);
rect(0, 350, width, 130);
noStroke();
fill(60,40,20,255);
rect(0, 350, width, 30);
pop();
//Trees
push();
noStroke();
fill(30,63,79);
triangle(80, 240, 0, 350, 160, 350);
fill(43,96,119);
triangle(330, 235, 245, 350, 450, 350);
fill(61,118,127);
triangle(240, 235, 220, 360, 350, 360);
fill(84,149,132);
triangle(400, 250, 280, 360, 530, 360);
fill(100,200,200);
triangle(150, 150, 50, 380, 280, 380);
pop();
//Clouds
push();
noStroke();
fill(255);
ellipse(cloudx, cloudy, 80, 40);
ellipse(cloudx+20, cloudy+20, 90, 30);
ellipse(cloudx-35, cloudy+20, 80, 45);
cloudx+=clouddx;
if(cloudx-80>=width){
cloudx = -120;
}
ellipse(cloudx2, cloudy2, 90, 30);
ellipse(cloudx2+30, cloudy2+20, 70, 25);
ellipse(cloudx2-20, cloudy2+20, 80, 30);
cloudx2+=clouddx2;
if(cloudx2-80>=width){
cloudx2 = -120;
}
pop();
//Lake
push();
noStroke();
fill(150);
ellipse(0, 530, width+500, 280);
fill(235, 239, 229);
ellipse(0, 530, width+400, 250);
pop();
myFerris.draw();
myFerris.update();
for(var i = 0; i<6; i++){
mySeat[i].draw();
mySeat[i].update();
}
}
Project 11: Generative Landscape
//Alicia Kim
//Section B
var terrainHeight = [];
var noiseParam = 0;
var noiseStep = 0.1;
var offset=0;
var maxi=1;
var fish = [];
var loc1 = 600;
var loc2 = 400;
var loc3 = 100;
var loc4 = 230;
var starX=[];
var starY=[];
var r = 144;
var g = 209;
var b = 206;
var by = 240;
var sx =240;
var sy = 60;
var frameCount_=0;
function setup() {
createCanvas(480, 240);
background(144, 209, 206);
frameRate(8);
for (var i=0; i<=width/5; i++) {
noiseParam += noiseStep;
var n = noise (noiseParam);
var value = map (n, 0, 1, 0, height);
terrainHeight.push(value);
noiseParam += noiseStep
}
for (var i=0;i<20;i++){
starX.push(random(0,width));
starY.push(random(0,height-100));
}
}
function draw() {
background(r, g, b);
loc1-=5;
loc2-=5;
loc3-=5;
loc4-=5;
if (loc1<-100){
loc1 = 600;
}
if (loc2<-100){
loc2 = 600;
}
if (loc3<-100){
loc3 = 600;
}
if (loc4<-100){
loc4 = 600;
}
noStroke();
drawSun();
if (frameCount_>50 & frameCount_<103) {
r-=20;
g-=8;
b-=2;
}
if (r<180 & g<180 && b<180) {
stars();
}
if (r<=0 & g<=0 && b<=0) { //reset
r=144;
g=209;
b=206;
sy = 60;
sx = 240;
frameCount_=0;
} else {
r+=6;
g-=0.1;
b-=1;
}
drawCloud(loc1,loc2,loc3,loc4);
drawMountain();
drawLake();
drawBoat();
frameCount_+=1;
}
function drawSun(){
fill (255, 150, 51); //yellow
circle (sx,sy,40,40);
sy+=1.5;
sx+=3;
}
function drawLake(){
fill (60, 111, 166,220); //blue
beginShape();
vertex(0,height);
for (var x = 0; x < width; x++) {
// wave
var theta = offset+0.07*x;
var y = map(sin(theta),-maxi,maxi,height-60,height-70);
vertex(x,y);
}
vertex(width, height);
endShape();
offset += 0.4;
}
function drawBoat(){
fill(235, 100, 52); //orange
strokeWeight(1);
ellipse(width/2,by,300,60);
fill(100);
ellipse(width/2,by,80,30);
// make boat float
if (by <= 210) { // when it gets higher than lake
by += 4;
} else {
by -= 1;
}
}
function drawCloud(loc){
drawCloudHelper(1.3,loc1,-4);
drawCloudHelper(1,loc2,25);
drawCloudHelper(0.8,loc3,15);
drawCloudHelper(1.6,loc4,0);
}
function drawCloudHelper(scale,loc,dy){
fill(255);
circle(loc,30+dy,30*scale);
circle(loc-10,36+dy,25*scale);
circle(loc-20,43+dy,20*scale);
circle(loc-3,40+dy,25*scale);
circle(loc+10,38+dy,25*scale);
circle(loc+21,41+dy,14*scale);
}
function drawMountain(){
terrainHeight.shift();
var n = noise(noiseParam);
var value = map (n, 0, 1, 0, height);
terrainHeight.push(value);
noiseParam += noiseStep
for (var i = 0; i < width/5; i++) {
noStroke();
fill (101, 138, 41); //green
beginShape();
vertex(i*5,terrainHeight[i]);
vertex((i+1)*5, terrainHeight[i+1]);
vertex ((i+1)*5, height-40);
vertex(i*5, height-40);
endShape();
}
}
function stars (){
for (var i=0;i<20;i++){
drawStar(starX[i],starY[i],1,4,5);
}
}
// adapted from p5js website (https://p5js.org/examples/form-star.html)
function drawStar(x, y, radius1, radius2, npoints) {
let angle = TWO_PI / npoints;
let halfAngle = angle / 2.0;
beginShape();
for (let a = 0; a < TWO_PI; a += angle) {
let sx = x + cos(a) * radius2;
let sy = y + sin(a) * radius2;
vertex(sx, sy);
sx = x + cos(a + halfAngle) * radius1;
sy = y + sin(a + halfAngle) * radius1;
vertex(sx, sy);
}
endShape(CLOSE);
}
Project – 11 – Landscape
In this animation, I wanted to create a simple and playful but mysterious atmosphere. I choose to make it only with shades of purple and set the scene in the woods during a full moon.
// Emily Franco
// efranco
// Section C
// Project 11
//CHANGING FEATURES
//verticle coordinates
var hill = [];
var noiseParam = 0;
//hill frequency
var noiseStep = 0.02;
var tree;
var forest = [];
var sky = [];
//state of person walking
var state = 0;
function moon(){
var oMoon = 30;
var dim = 100;
noStroke();
fill(252,250,252,230);
circle(300,90,dim);
//halo
for(var i=0; i<3; i++){
oMoon -= 5*i;
dim +=30;
fill(252,250,252,oMoon);
circle(300,90,dim);
}
}
//constructor for stars
function makeStar(){
var star = {x:random(width),y:random(height),size:random(0.25,2),
opacity:random(100,255),
drawFunction: drawStar};
return star;
}
function drawStar(){
stroke(252,250,252,this.opacity);
strokeWeight(this.size);
point(this.x,this.y);
}
function stepTree(){
this.x++;
}
function drawTree(){
noStroke();
var side;
var triangleY;
var branchLen;
fill(this.color);
rect(this.x,0,this.w,height);
//branches
switch(this.side){
case 0:
triangle(this.x,this.triangleY,this.x-this.branchLen,this.triangleY-6,this.x,this.triangleY+10);
break;
case 1:
triangle(this.x+this.w,this.triangleY,this.x+this.w+this.branchLen,this.triangleY-6,this.x+this.w,this.triangleY+10);
break;
}
}
//tree constructor
function makeTrees(tx,tw,treeColor){
var tree = {x: tx, w:tw, color:treeColor, side:floor(random(2)),
triangleY:random(height-100), branchLen:random(10,55),
drawFunction:drawTree, stepFunction: stepTree};
return tree;
}
//center if person is center of face circle
function person(x, y,state){
//LEGS
//state 1
if(state==0){
strokeWeight(2);
stroke(248,232,212);
line(x-6,y+58,x-8,y+68);
line(x+4,y+58,x+4,y+68);
stroke(0);
line(x-8,y+68,x-9,y+81);
line(x+4,y+68,x+7,y+81);
//shadow
noStroke();
fill(0,0,0,90);
rect(x-10,y+81,30,5);
}
//state 2
if(state==1){
strokeWeight(2);
stroke(248,232,212);
line(x-3,y+58,x-8,y+67);
line(x+5,y+58,x+6,y+68);
stroke(0);
line(x-8,y+67,x-6,y+81);
line(x+6,y+68,x+9,y+81);
//shadow
noStroke();
fill(0,0,0,90);
rect(x-12,y+81,35,5);
}
//state 3
if(state==2){
strokeWeight(2);
stroke(248,232,212);
line(x-3,y+58,x-6,y+69);
line(x,y+58,x,y+68);
stroke(0);
line(x-6,y+69,x+3,y+81);
line(x,y+68,x,y+81);
//shadow
noStroke();
fill(0,0,0,90);
rect(x-5,y+81,15,5);
}
noStroke();
//BODY
//hair in back
fill(43,28,28);
rect(x-10,y,17.5,29.8);
//head
fill(248,232,212);
circle(x,y,20);
//neck
rect(x-1,y+10,2,5);
//hair
fill(56,38,33);
arc(x,y,20,20,radians(180),radians(0),CHORD);
rect(x+4.6,y,5.4,29.9);
//eye
ellipse(x-6,y+2,1,2);
//dress
fill(33,30,45);
beginShape();
vertex(x-6.5,y+15);
vertex(x-15,y+59);
vertex(x+15,y+59);
vertex(x+6.5,y+15);
endShape(CLOSE);
}
function moveHill(){
//hill.shift();
//add new coordinate to hill arry
n = noise(noiseParam);
val = map(n,0,1,height-100,height);
hill.pop();
hill.unshift(val);
noiseParam = noiseParam + noiseStep;
}
function defineHill(){
var n;
var val;
for(var i=0; i<(width/5)+1; i++){
n = noise(noiseParam);
//map noise output to height of canvas
val = map(n,0,1,height-100,height);
hill.unshift(val);
//increment to change curve
noiseParam = noiseParam + noiseStep;
}
}
function setup(){
createCanvas(400, 400);
frameRate(5);
//STARS
for(var i=0; i<200; i++){
sky[i] = makeStar();
}
//TREES
for(var i=0; i<=18;i++){
//furthest back
if(i<=4){
c = color(50,36,81);
}
//middle
if(i>4 & i<=10){
c = color(35,29,71);
}
if(i>10){
c = color(25,18,66);
}
//front
tree = makeTrees(random(width),random(5,15),c);
forest.push(tree);
}
//HILL
defineHill();
}
function draw(){
background(69,55,89);
var drawSetBack = floor(random(30));
//------BACKGROUND--------
moon();
for(var i=0; i<sky.length-1; i++){
//make stars twinkle
if(i%5==0){
sky[i].size = random(0.25,3.5);
}
var str = sky[i];
str.drawFunction();
}
//back hills
fill(49, 34, 66);
noStroke();
beginShape();
curveVertex(400,235);
curveVertex(400,235);
curveVertex(316,283);
curveVertex(232,285);
curveVertex(194,400);
curveVertex(400,400);
endShape(CLOSE);
fill(62, 47, 79);
noStroke();
beginShape();
curveVertex(0,245);
curveVertex(0,245);
curveVertex(35,204);
curveVertex(87,273);
curveVertex(192,258);
curveVertex(272,320);
curveVertex(400,400);
curveVertex(0,400);
endShape(CLOSE);
//------MIDGROUND--------
//find index of most right tree
var currentX=0;
var xHigh=0;
for(var i=0; i<=forest.length-1;i++){
currentX = forest[i].x;
if(currentX>xHigh){
xHigh=currentX;
furthestTree = i;
}
}
//move last tree to start of canvas
if(forest[furthestTree].x > width){
forest[furthestTree].x = 0-forest[furthestTree].w;
}
for(var i=0; i<=forest.length-1;i++){
var t = forest[i];
t.drawFunction();
t.stepFunction();
}
//------FOREGROUND--------
//draw hill
for(var i=0; i<(width/5);i++){
fill(125, 104, 135);
beginShape();
vertex(i*5,hill[i]);
vertex(i*5,height);
vertex((i+1)*5,height);
vertex((i+1)*5,hill[i+1]);
endShape(CLOSE);
}
moveHill();
//move person according to hill value
person(260,hill[53]-79,state%3);
state++;
}
Project 11 – Moving Landscape
The project is a relaxing view of sunset in an air balloon – if you take the time to watch the full animation, you can see the colors of the sky, sun, and clouds change as well through gradual RGB changes and color theory. I wanted a lowpoly style animation, and worked to get effect through the “shifting clouds”
//Aarnav Patel
//aarnavp@andrew.cmu.edu
//Section D
var r = 140;
var g = 200;
var b = 230;
var cloudR = 255;
var cloudG = 255;
var cloudB = 255;
var sunX;
var sunY = 0;
var sunR = 255;
var sunG = 247;
var sunB = 197;
var sunSize = 50;
var airBalloons = [];
var clouds = [];
var sceneCount = 0;
var bPics = ["https://i.imgur.com/El159Qi.png", "https://i.imgur.com/Inz2K7i.png", "https://i.imgur.com/IjQkLRr.png"]
var balloons = []
function preload() {
//load the images
for (var i = 0; i < bPics.length; i++) {
balloons.push(loadImage(bPics[i]));
}
}
function setup() {
createCanvas(480, 200);
sunX = width;
frameRate(10);
}
function draw() {
var sky = color(r, g, b);
fill(sky);
rect(0, 0, width, height);
//sky color change
if (r < 255) {
r += 0.1
}
if (g > 150) {
g -= 0.1;
}
if (b > 87) {
b -= 0.1;
}
//sky grandient
for (var i = 0; i < 50; i+= 0.5) {
stroke(r + i, g + i, b + i);
var amount = map(i * 2, 0, 100, height * 0.75, height);
line(0, amount, width, amount);
}
//SUN
if (sunR > 242) {
sunR -= 0.1;
}
if (sunG > 60) {
sunG -= 0.1;
}
if (sunB > 10) {
sunB -= 0.1;
}
noStroke();
fill(sunR, sunG, sunB);
ellipse(sunX, sunY, sunSize, sunSize)
sunX -= 0.25;
sunY += 0.1;
sunSize += 0.01;
//cloud color change
if (cloudR >= 244) {
cloudR -= 0.05;
}
if (cloudG >= 223) {
cloudG -= 0.05;
}
if (cloudB > 208) {
cloudB -= 0.05;
}
generateClouds();
generateAirBalloons();
updateClouds();
updateBalloons();
sceneCount++;
}
function makeCloud(stormCloud) {
var cloud = {
cX: random(0, 1.5 * width), cY: random(0, height), cDx:0,
cW: random(100, 300),
isStorm: stormCloud,
show: drawCloud,
move: moveCloud,
}
return cloud;
}
function drawCloud() {
noStroke();
var xInterval = this.cW / 8; //makes sure the x points never exceed the assigned cloudWidth
var xPoint = this.cX
var yPoint = this.cY
//foreground clouds are lightest
if (this.cW > 200) {
this.cDx = 5;
fill(cloudR, cloudG, cloudB, 255);
} else if (this.cW > 100){ //midGround are slightly opaque
fill(cloudR, cloudG, cloudB, 200)
this.cDx = 3;
} else {
fill(cloudR, cloudG, cloudB, 100); //background are most opaque
this.cDx = 1;
}
//checks if its storm cloud
if (this.isStorm) {
fill(200, 255);
}
//generating points for the lowPoly Clouds
var y = [yPoint, yPoint - 15, yPoint - 20, yPoint - 15, yPoint, yPoint + 15, yPoint + 20, yPoint + 15];
//Y points are fixed
var x = [xPoint];
for (var i = 0; i < 8; i++) {
if (i < 4) {
xPoint += xInterval
} else {
xPoint -= xInterval
}
x.push(xPoint);
}
//gives animating effect
for (var i = 0; i < x.length; i++) {
x[i] += random(-5, 5);
y[i] += random(-5, 5);
}
//draw cloud based on array made
beginShape();
for (var i = 0; i < x.length; i++) {
vertex(x[i], y[i]);
}
endShape(CLOSE);
}
function moveCloud() {
//updates cloudX by its speed
this.cX -= this.cDx;
//when cloudX is off left of screen, assigns it new position
if (this.cX + this.cW < 0) { //checks right side of cloud
this.cX = random(width, 1.5 * width);
this.cY = random(0, height / 1.5);
}
}
function updateClouds() {
//moves all the clouds from global cloud array
for (var i = 0; i < clouds.length; i++) {
clouds[i].move();
}
}
function generateClouds() {
//creates clouds with a 1/8 chance of a cloud being a storm cloud
var isStorm = false;
for (var i = 0; i < 15; i++) {
if (amount = Math.floor(random(1, 8)) == 2) {
isStorm = true;
}
var c = makeCloud(isStorm);
clouds.push(c);
clouds[i].show();
isStorm = false;
}
}
function generateAirBalloons() {
//generates air balloons from image I have
for (var i = 0; i < 10; i++) {
var b = makeBalloon()
airBalloons.push(b);
airBalloons[i].show();
}
}
function makeBalloon() {
var b = {
bX: random(0, width), bY: random(height * -5, height),
bDx: Math.floor(random(-4, 4)), bDy: Math.floor(random(2, 4)),
bImage: Math.floor(random(0, 3)), //ranodm number to select the type of balloon image
ratio: Math.floor(random(0, 2)),
show: drawBalloon,
float: floatBalloon,
}
return b;
}
function updateBalloons() {
for (var i = 0; i < airBalloons.length; i++) {
airBalloons[i].float();
}
}
function floatBalloon() {
this.bX += this.bDx;
this.bY += this.bDy;
if (this.bY > height) {
this.bY = random(height * -5, height * -0.25);
this.bX = random(0, width);
}
}
function drawBalloon() {
image(balloons[this.bImage], this.bX, this.bY, balloons[this.bImage].width * this.ratio, balloons[this.bImage].height * this.ratio);
}
Project 11
just my lil German boy
// Sowang Kundeling Section C Project 11
var backgroundX = 355;
var foregroundX = 250;
function preload() {
germanboy = loadImage("https://i.imgur.com/8g7kXHX.png");
neighborhood = loadImage("https://i.imgur.com/ueqfD0Q.png");
house = loadImage("https://i.imgur.com/VeVnWaO.png");
montain = loadImage("https://i.imgur.com/xImWYKs.png");
long = loadImage("https://i.imgur.com/6kAoEti.png");
short = loadImage("https://i.imgur.com/hGhArWq.png");
}
function setup() {
createCanvas(480, 240);
}
function draw() {
// sky
noStroke();
fill(180, 227, 242);
rect(0, 0, 480, 25); // x7
fill(163, 194, 207);
rect(0, 25, 480, 25);
fill(137, 165, 176);
rect(0, 50, 480, 25);
fill(121, 146, 156);
rect(0, 75, 480, 25);
fill(98, 119, 128);
rect(0, 100, 480, 25);
fill(79, 95, 102);
rect(0, 125, 480, 25);
fill(60, 74, 79);
rect(0, 150, 480, 25);
imageMode(CENTER);
// background elements
for (var i = 0; i < 2; i ++) {
image(short, backgroundX - (i * 150), 159, short.width/2, short.height/2);
image(long, (backgroundX + 90) - (i * 200), 145, long.width/2, long.height/2);
}
// land
fill(107, 96, 81);
rect(0, 175, 480, 65); // land
// foreground elements
image(montain, foregroundX-120, 115, 265, montain.width/4, montain.height/4);
image(neighborhood, foregroundX - 140, 137, neighborhood.width/2, neighborhood.height/2);
image(house, foregroundX + 250, 140, house.width/4, house.height/4);
image(germanboy, 100, 200, germanboy.width/2, germanboy.height/2);
backgroundX -= 1;
foregroundX -= 1;
// reset
if (backgroundX <= -300) {
backgroundX = 900;
}
if (foregroundX <= -300) {
foregroundX = 825
}
}
Project-11-Landscape
I take references from this animation/rendering competition and made this robot pushing a sphere in an alien planet:
I have trees generation in the background
boulders in front
and hills in the back
/*
* Andrew J Wang
* ajw2@andrew.cmu.edu
* Section A
*
* This Program is walking
*/
//sets of links
//location of feets of the walking person
var pointXG = 0;
var pointYG = 0;
//steps (frame) locations of the feets
var stepsX = [0,1,2,3,4,5,6,7,8,9,10,10,10,10,10,10,9,8,7,6,5,4,3,2,1,0];
var stepsY = [0,0.5,1,1.5,2,2.5,3,3.5,4,4.5,5,4,3,2,1,0,0,0,0,0,0,0,0,0,0,0];
//counters for multiple frames (feet + mountains)
var counter = 0;
//location of the person on the drawing
var locationX = 120;
var locationY = 200;
//arrays for trees for clusters of boulders
var trees = [];
var clusters = [];
//set a array for land heights (FROM PREVIOUS ASSIGNMENT)
var landHeight = [];
var landHeight2 = [];
//create noice parameter and steps
var noiseParam = 0;
var noiseParam2 = 0;
var noiseStep = 0.005;
var noiseStep2 = 0.01;
function setup() {
createCanvas(480,300);
// create an initial collection of trees
for (var i = 0; i < 5; i++){
var rx = random(width);
trees[i] = makeTrees(rx);
}
// create an initial collection of boulders
for (var i = 0; i < 10; i++)
{
var rx2 = random(width);
clusters[i] = makeClusters(rx2);
}
//hill #1
for (var k=0; k<=480; k++)
{
//get noise through noise param
var n = noise(noiseParam);
//remapping based on height
var value = map(n,0,1,0,height/4)+130;
//add to array
landHeight.push(value);
//plus steps
noiseParam += noiseStep;
}
//hill #2
for (var k=0; k<=480; k++)
{
//get noise through noise param
var n2 = noise(noiseParam2);
//remapping based on height
var value2 = map(n2,0,1,0,height/3)+80;
//add to array
landHeight2.push(value2);
//plus steps
noiseParam2 += noiseStep2;
}
}
function draw() {
background(100);
//MOON
push();
noStroke();
fill(255,255,220);
ellipse(380,0,250,250);
pop();
//draw first sets of hill
push();
noStroke();
fill(240);
beginShape();
//fist vertex
vertex(0,(locationY+70+60)*0.8-80);
//for loop to grab all the vertex
for (var k=0; k<=480; k++)
{
vertex(k,landHeight2[k]);
}
//last vertex
vertex(width,(locationY+70+60)*0.8-80);
endShape(CLOSE);
//adding another point by shifting
var n2=noise(noiseParam2);
var value2 = map(n2,0,1,0,height/3)+80;
noiseParam2 += 0.01/20;
//slowing the speed of refreshing by using a counter
if (counter%40==0)
{
landHeight2.shift();
landHeight2.push(value2);
}
pop();
//draw second sets of hill
push();
noStroke();
fill(220);
beginShape();
//fist vertex
vertex(0,(locationY+70+60)*0.8-80);
//for loop to grab all the vertex
for (var k=0; k<=480; k++)
{
vertex(k,landHeight[k]);
}
//last vertex
vertex(width,(locationY+70+60)*0.8-80);
endShape(CLOSE);
//adding another point by shifting
var n=noise(noiseParam);
var value = map(n,0,1,0,height/4)+130;
noiseParam += 0.005/5;
//slowing the speed of refreshing by using a counter
if (counter%10==0)
{
landHeight.shift();
landHeight.push(value);
}
pop();
//ground plane
push();
noStroke();
fill(200);
rect(0,(locationY+70+60)*0.8-80,width, height-((locationY+70+60)*0.8-80));
pop();
//set trees and clusters by refreshing the removed objects and clusters
updateDisplay();
removeTrees();
addTrees();
removeClusters();
addClusters();
//walking person
strokeWeight(3);
push();
//scaling it
scale(0.6);
translate(0,150);
walking(locationX,locationY);
walking2(locationX,locationY);
body(locationX,locationY);
//butt
push();
strokeWeight(2);
ellipse(locationX,locationY,15,15);
pop();
pop();
//display clusters in the end
updateDisplay2();
}
//refreashing trees and display them
function updateDisplay(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
//refreshing boulders and display them
function updateDisplay2(){
for (var i = 0; i < clusters.length; i++){
clusters[i].move();
clusters[i].display();
}
}
//removing trees if it is too far away from the screen
function removeTrees(){
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x + trees[i].breadth > 0) {
treesToKeep.push(trees[i]);
}
}
}
//removing boulders if it is too far away from the screen
function removeClusters(){
var clustersToKeep = [];
for (var i = 0; i < clusters.length; i++){
if (clusters[i].x + clusters[i].breadth > 0) {
clustersToKeep.push(clusters[i]);
}
}
}
//add trees 100 units away from border
function addTrees() {
var Likelihood = 0.01;
if (random(0,1) < Likelihood) {
trees.push(makeTrees(width+100));
}
}
//add boulders 500 units away from border
function addClusters() {
var Likelihood = 0.01;
if (random(0,1) < Likelihood) {
clusters.push(makeClusters(width+500));
}
}
//set trees values and function
function makeTrees(birthLocationX) {
var tree = {x: birthLocationX,
breadth: 100,
speed: -0.4,
y: 160+round(random(20)),
treeHeight: round(random(40,50)),
size: round(random(30,50)),
move: objectMove,
display: treeDisplay}
return tree;
}
//set boulders values and functions
function makeClusters(birthLocationX) {
var tree = {x: birthLocationX,
breadth: 100,
speed: -2.0,
treeHeight: round(random(40,50)),
size: round(random(200,350)),
move: object2Move,
display: clusterDisplay}
return tree;
}
//move objects
function objectMove() {
this.x += this.speed;
}
function object2Move() {
this.x += this.speed;
}
//draw trees
function treeDisplay() {
line(this.x, this.y , this.x, this.y+this.treeHeight);
push();
translate(this.x,this.y);
rectMode(CENTER);
noFill();
strokeWeight(1);
push();
rotate(-counter/180*Math.PI);
rect(0,0,this.size,this.size);
pop();
push();
rotate(counter/180*Math.PI);
rect(0,0,this.size-10,this.size-10);
pop();
pop();
}
//draw bolders
function clusterDisplay() {
push();
fill(0);
ellipse(this.x,height+30,this.size,this.size/2);
strokeWeight(1.5);
noFill();
ellipse(this.x,height+30,this.size+20,this.size/2+10);
pop();
}
//leg #1
function walking(xL,yL)
{
//counter/10 get frame number
var counterK = Math.floor(counter/10)%(stepsX.length);
//Feet locations
pointXG = xL-70+stepsX[counterK]*6;
pointYG = yL+70-stepsY[counterK]*4;
//Pathegorean theorm to get the knee and legs
var dis = Math.sqrt((xL-pointXG)*(xL-pointXG)+(yL-pointYG)*(yL-pointYG));
var num = (10000)-(dis*dis);
var sid = sqrt(num);
var midX = xL-(xL-pointXG)/2;
var midY = yL-(yL-pointYG)/2;
var tan = atan2(pointXG-xL,pointYG-yL);
ellipse ((pointXG-xL)/2+xL+cos(tan)*sid/2, (pointYG-yL)/2+yL-sin(tan)*sid/2, 5,5);
line(xL,yL,(pointXG-xL)/2+xL+cos(tan)*sid/2, (pointYG-yL)/2+yL-sin(tan)*sid/2);
line(pointXG,pointYG,(pointXG-xL)/2+xL+cos(tan)*sid/2, (pointYG-yL)/2+yL-sin(tan)*sid/2);
//feet bending
if (stepsY[counterK]==0)
{
line(pointXG,pointYG,pointXG+20,pointYG);
}
else
{
var tanF = atan2(Math.sqrt(400-stepsY[counterK]*2),stepsY[counterK]*4);
line(pointXG,pointYG,pointXG+20*sin(tanF),pointYG+20*cos(tanF));
}
counter++;
}
//repeat for the second leg
function walking2(xL,yL)
{
var counterK = (Math.floor(counter/10)+stepsX.length/2)%(stepsX.length);
pointXG = xL-70+stepsX[counterK]*6;
pointYG = yL+70-stepsY[counterK]*4;
var dis = Math.sqrt((xL-pointXG)*(xL-pointXG)+(yL-pointYG)*(yL-pointYG));
var num = (10000)-(dis*dis);
var sid = sqrt(num);
var midX = xL-(xL-pointXG)/2;
var midY = yL-(yL-pointYG)/2;
var tan = atan2(pointXG-xL,pointYG-yL);
ellipse ((pointXG-xL)/2+xL+cos(tan)*sid/2, (pointYG-yL)/2+yL-sin(tan)*sid/2, 5,5);
line(xL,yL,(pointXG-xL)/2+xL+cos(tan)*sid/2, (pointYG-yL)/2+yL-sin(tan)*sid/2);
line(pointXG,pointYG,(pointXG-xL)/2+xL+cos(tan)*sid/2, (pointYG-yL)/2+yL-sin(tan)*sid/2);
if (stepsY[counterK]==0)
{
line(pointXG,pointYG,pointXG+20,pointYG);
}
else
{
var tanF = atan2(Math.sqrt(400-stepsY[counterK]*2),stepsY[counterK]*4);
line(pointXG,pointYG,pointXG+20*sin(tanF),pointYG+20*cos(tanF));
}
counter++;
}
//body parts and other stuff
function body(xL,yL)
{
var counterK = (Math.floor(counter/10)+stepsX.length/2)%(stepsX.length);
var counterK2 = (Math.floor(counter/10)+10)%(stepsX.length);
var counterK3 = (Math.floor(counter/10)+25)%(stepsX.length);
push();
strokeWeight(2);
fill("grey")
//shoulder 1
ellipse(xL+35+stepsY[counterK2],yL-45-stepsX[counterK2],30,30);
//hand
ellipse(xL+35+60,yL-45-stepsX[counterK]+10,10,10);
pop();
//arms
line(xL+35+stepsY[counterK],yL-45-stepsX[counterK],xL+35+30,yL-45-stepsX[counterK]+20);
line(xL+35+30,yL-45-stepsX[counterK]+20,xL+35+60,yL-45-stepsX[counterK]+10);
//body
line(xL,yL,xL+35+stepsY[counterK],yL-45-stepsX[counterK]);
push();
fill("black");
ellipse(xL+35+30,yL-45-stepsX[counterK]+20,5,5);
pop();
//Round thingy
push();
noFill();
strokeWeight(2);
ellipse(xL+35+175,yL-45,230,230);
fill(255);
ellipse(xL+35+175,yL-45,220,220);
stroke(255);
pop();
//shoulder 2
push();
noStroke();
ellipse(xL+35+stepsY[counterK2],yL-45-stepsX[counterK2],15,15);
pop();
//head
push();
strokeWeight(1);
noFill();
translate(xL+55+stepsY[counterK3],yL-85-stepsX[counterK3]);
rectMode(CENTER);
rotate(-counter/180*Math.PI);
rect(0,0,40,40);
rect(0,0,30,30);
pop();
push();
strokeWeight(1);
noFill();
translate(xL+65+stepsY[counterK2]*2,yL-95-stepsX[counterK2]);
rectMode(CENTER);
rotate(counter/180*Math.PI);
rect(0,0,25,25);
rect(0,0,30,30);
pop();
push();
strokeWeight(1);
noFill();
translate(xL+45+stepsY[counterK]*2,yL-75-stepsX[counterK]);
rectMode(CENTER);
rotate(counter/180*Math.PI);
rect(0,0,25,25);
rect(0,0,15,15);
pop();
}
A day in the future city
I took inspiration from a scene in cyberpunk edgerunner and made a city landscape based on that. I am surprised by the flexibility of object, the properties can be manipulated in different ways to create completely different stuff. For example, the pillars, foreground, and background buildings are created with one single object.
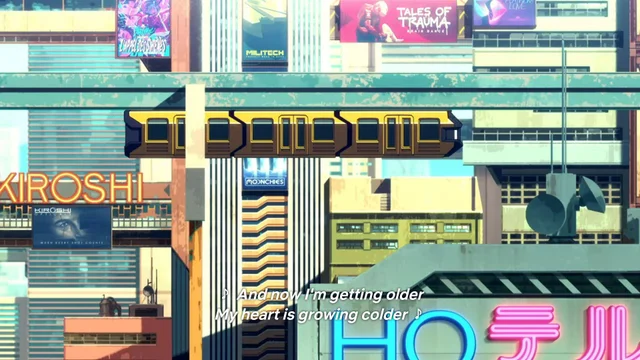
//Jason Jiang
//Section E
//setting up arrays of objects
var bldg = [];
var bldgBack = []
var pillar = []
var vhc = []
//number of objects in each array
var bldgN = 6;
var bldgBackN = 11;
var vhcN = 2;
//color palatte for buildings
var palatte = ['#7670b2', '#5ab6b0', '#5ab6b0', '#3f5c60', '#1c4167', '#3f5c60', '#7e9868'];
//image links of assets
var facadeLink = [
"https://i.imgur.com/oPA4x4y.png",
"https://i.imgur.com/xeOW3sz.png",
"https://i.imgur.com/gbr6ySL.png",
"https://i.imgur.com/WqUehK3.png"];
var facadeImg = [];
var vehicleLink = [
"https://i.imgur.com/gFtwhqV.png",
"https://i.imgur.com/KX1dLCi.png",
"https://i.imgur.com/Fo43Kep.png"];
var vehicleImg = [];
//load assets
function preload(){
train = loadImage("https://i.imgur.com/BFxe31d.png");
for (var i = 0; i < facadeLink.length; i++){
facadeImg[i] = loadImage(facadeLink[i]);
}
for (var i = 0; i < vehicleLink.length; i++){
vehicleImg[i] = loadImage(vehicleLink[i]);
}
}
function setup() {
createCanvas(400, 200);
imageMode(CENTER);
colorMode(HSB);
//create front building arrays
for(var i = 0; i < bldgN; i++){
//randomly pick color from array
var palatteIndex = floor(random(palatte.length));
//randomly pick an image from array
var facadeIndex = floor(random(facadeImg.length));
var b = building(80*i, random(-height/3, height/3), random(80, 100), color(palatte[palatteIndex]), facadeImg[facadeIndex]);
bldg.push(b);
}
//create back building arrays
for(var i = 0; i < bldgBackN; i++){
var b = building(40*i, random(150), 40, color(20, 10, 30, 0.5), -1);
bldgBack.push(b);
}
//create pillars
for (var i = 0; i < 2; i++) {
var p = building(200*i, 70, 20, color(80), -1);
pillar.push(p);
}
//creating vehicles
for (var i = 0; i < 2; i++) {
//randomly pick an image from array
var vehicleIndex = floor(random(vehicleImg.length));
//randomize vehicle moving direction
if (random(1) <= 0.5){
//vehicles from left to right
var v = vehicle(random(-width/2, 0), random(50, 150), random(5, 10), vehicleImg[vehicleIndex]);
}
else{
//vehicles from right to left
var v = vehicle(random(width, 1.5*width), random(50, 150), random(-5, -10), vehicleImg[vehicleIndex]);
}
vhc.push(v);
}
}
function draw() {
background(200, 20, 100);
//update information in each frame
updateObjs();
updateArray();
//add train image
image(train, 200, 90);
}
function updateObjs(){
//updating building background
for(var i = 0; i < bldgBack.length; i++){
bldgBack[i].move(2);
bldgBack[i].display();
}
//updating building foreground
for(var i = 0; i < bldg.length; i++){
bldg[i].move(5);
bldg[i].display();
}
//updating pillars
for(var i = 0; i < pillar.length; i++){
pillar[i].move(5);
pillar[i].display();
}
//updating vehicles
for (var i = 0; i < vhc.length; i++) {
vhc[i].move();
vhc[i].display();
}
}
//displaying buildings
function buildingDisplay(){
//draw rectangles
noStroke();
fill(this.color);
rect(this.x, this.y, this.w, height-this.y);
var centerX = this.x + 0.5*this.w ;
var centerY = this.y + 0.5*(height - this.y);
//see if the detail property of object is an image, since not pillars and background buildings dont need facade details on them
if (this.detail != -1){
//add details on building facade
push();
translate(centerX, centerY);
image(this.detail, 0, 0, 0.8*this.w, 0.95*(height-this.y));
pop();
}
}
//update building position
function buildingMove(s){
this.x -= s;
}
//displaying vehicles
function vehicleDisplay(){
push();
//flip the image if going from right to left
if(this.s < 0){
scale(-1, 1);
image(this.detail, -this.x, this.y);
}
else{
image(this.detail, this.x, this.y);
}
pop();
}
//update vehicles position and age
function vehicleMove(){
this.x += this.s;
this.age += 1;
}
function updateArray(){
//replacing foreground buildings outside canvas
for(var i = 0; i < bldg.length; i++){
if (bldg[i].x <= -bldg[i].w){
var palatteIndex = floor(random(palatte.length));
var facadeIndex = floor(random(facadeImg.length));
var b = building(400, random(-height/3, height/3), random(80, 100), color(palatte[palatteIndex]), facadeImg[facadeIndex]);
bldg[i] = b;
}
}
//replacing background buildings outside canvas
for(var i = 0; i < bldgBack.length; i++){
if (bldgBack[i].x <= -bldgBack[i].w){
var b = building(400, random(150), 40, color(20, 10, 30, 0.5), -1);
bldgBack[i] = b;
}
}
//replacing pillars outside canvas
for(var i = 0; i < pillar.length; i++){
if (pillar[i].x <= -pillar[i].w){
var p = building(400, 70, 20, color(80), -1);
pillar[i] = p;
}
}
//replacing vehicles after a certain time
for(var i = 0; i < vhc.length; i++){
if (vhc[i].age > 200){
var vehicleIndex = floor(random(vehicleImg.length));
if (random(1) <= 0.5){
var v = vehicle(random(-width/2, 0), random(50, 150), random(5, 10), vehicleImg[vehicleIndex]);
}
else{
var v = vehicle(random(width, 1.5*width), random(50, 150), random(-5, -10), vehicleImg[vehicleIndex]);
}
vhc[i] = v;
}
}
}
//create building objects
function building(buildingX, buildingY, buildingWidth, buildingColor, buildingDetail) {
var b = { x: buildingX,
y: buildingY,
w: buildingWidth,
color: buildingColor,
detail: buildingDetail,
display: buildingDisplay,
move: buildingMove
}
return b;
}
//create vehicle objects
function vehicle(vehicleX, vehicleY, vehicleSpeed, vehicleDetail) {
var v = { x: vehicleX,
y: vehicleY,
s: vehicleSpeed,
age: 0,
detail: vehicleDetail,
display: vehicleDisplay,
move: vehicleMove
}
return v;
}