In my process, I wanted to figure out how to play with color and how that could change through pressing the mouse. I also was interested in and had a lot of fun figuring out how simple shape changes could affect expression.
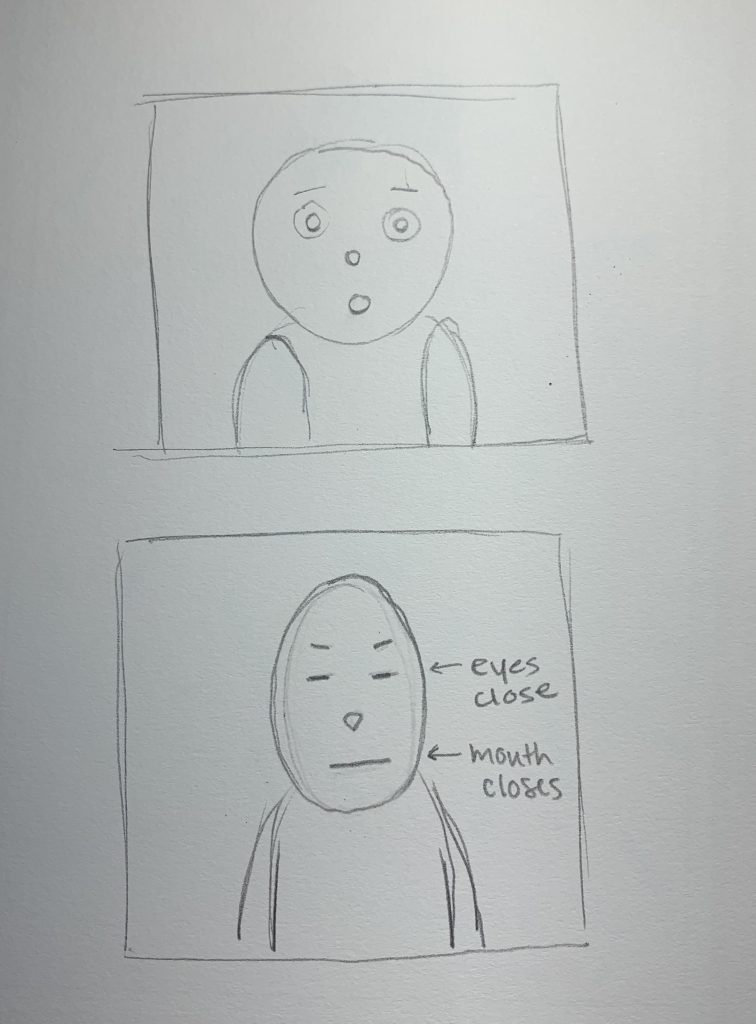
project2
//Rachel Legg Class Section C
var eyeSize = 50;
var eyeHeight = 25;
var faceWidth = 300;
var bodyWidth = 400;
var faceHeight = 300;
var eyebrowHeight = 200;
var r; // making rgb variable
var g;
var b;
var on = false;
var x = 0;
var y = 0;
function setup() {
createCanvas(640, 480);
background(220);
}
function draw() {
noStroke();
if(on){ //alternate background color on click
background(252, 169, 133); //orange
}else{
background(204, 236, 239); //blue
}
//shirt
fill(r, g, b);
ellipse(width / 2, height, bodyWidth, height);
fill(r + 10, g + 10, b +10);
ellipse(width / 4, height, bodyWidth / 4, height / 2);
ellipse(width * 3/4, height, bodyWidth / 4, height / 2);
//head
if(on){ //alternate head color on click
fill(253, 222, 238); //pink
}else{
fill(224, 243, 176); //green
}
ellipse(width / 2, height / 2, faceWidth, faceHeight);
//eyes
fill(r, g, b, 200);
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
if(mouseX < width / 2){
ellipse(eyeLX, height / 2, eyeSize, eyeHeight);
ellipse(eyeRX, height / 2, eyeSize, eyeHeight);
fill(0); //pupils
ellipse(eyeLX, height / 2, 1/2 * eyeSize, 3/4 * eyeHeight);
ellipse(eyeRX, height / 2, 1/2 * eyeSize, 3/4 * eyeHeight);
fill(255, 255, 255, 200);
ellipse(eyeLX + 4, (height / 2) - 4, 1/8 * eyeSize, 1/8 * eyeHeight); //glare
ellipse(eyeRX + 4, (height / 2) - 4, 1/8 * eyeSize, 1/8 * eyeHeight);
}else{
if(on){ //alternate nose color on click
stroke(251, 182, 209); //darker pink
}else{
stroke(145, 210, 144); //darker green
}
strokeWeight(2);
line(eyeLX - 10, height / 2, eyeLX + 10, height / 2); //closing eyes when mouse is on right side
line(eyeRX + 10, height / 2, eyeRX - 10, height / 2);
}
//mouth
if(mouseY > height / 2){
fill(0);
noStroke();
circle(width / 2, 2/3 * height, 20, 20); //open mouth when below half
}else{
if(on){ //alternate nose color on click
stroke(251, 182, 209); //darker pink
}else{
stroke(145, 210, 144); //darker green
}
strokeWeight(2);
line(2/5 * width, 2/3 * height, 3/5 * width, 2/3 * height);
}
//nose
noStroke();
if(on){ //alternate nose color on click
fill(251, 182, 209); //darker pink
}else{
fill(145, 210, 144); //darker green
}
beginShape();
curveVertex(1/5 * width, 4/5 * height);
curveVertex(1/2 * width, 5/9 * height);
curveVertex(1/2 * width, 5/9 * height);
curveVertex(4/5 * width, 4/5 * height);
endShape();
//eyebrow
if(on){ //alternate eyebrow color on click
stroke(251, 182, 209); //darker pink
}else{
stroke(145, 210, 144); //darker green
}
strokeWeight(3);
if(mouseX < width/ 2){
line(eyeLX - 10, eyebrowHeight - 10, eyeLX + 15, eyebrowHeight - 15);
line(eyeRX + 10, eyebrowHeight - 5, eyeRX - 15, eyebrowHeight - 15);
}else{
line(eyeLX - 10, eyebrowHeight - 30, eyeLX + 15, eyebrowHeight - 20);
line(eyeRX + 10, eyebrowHeight - 30, eyeRX - 15, eyebrowHeight - 20);
}
}
function mousePressed() {
if(on){ //change background on click
on = false;
}else{
on =true;
}
faceWidth = random(300, 500); //with click, face width changes
bodyWidth = random(375, 415); //with click, shirt width changes along with arms
faceHeight = random(200, 400); //face height changes
eyeSize = random(20, 80); //eye size changes
eyeHeight = random (20, 60);
r = random(0, 255); //eye and shirt color change
g = random (0, 255);
b = random (0, 255);
eyebrowHeight = random(180, 200); //eyebrow position changes
}