My process for this project was to alter basic shapes and colors to create a kaleidoscope. I used transformations so I could draw the same images many times in different locations. Moving the mouse left and right shrinks, enlarges, and repositions different elements. Crossing the horizontal midpoint reverses the rotation. Vertical motion with the mouse speeds and slows the rotation. Clicking changes colors.
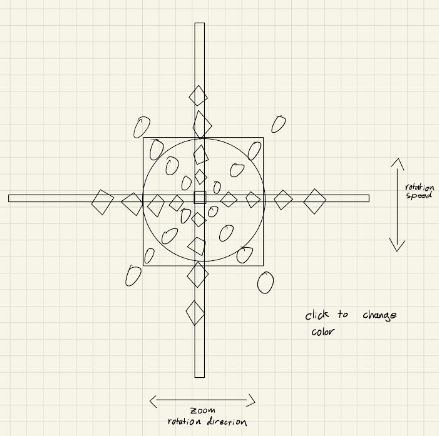
sketch
// Evan Stuhlfire
// estuhlfi, section B
// Project-03: Dynamic Drawing
var angle = 0; // Angle to increment for rotation
var angleInc = 2; // Controls speed of rotation
var rotateDir = 1; // Direction of rotation
var centerX; // Center of original canvas
var centerY;
var shapeSpace = 30; // Space between shapes
var rectSize = 20;
var startSize = 10;
var circleStart = 10;
var littleCircle = 10;
var colorScheme = 1; // standard = 1, switched = -1
function setup() {
createCanvas(600, 450);
centerX = width/2;
centerY = height/2;
}
function draw() {
background(50);
// If the mouse is left of center set rotation counterclockwise
if(mouseX < centerX){
rotateDir = -1;
} else {
rotateDir = 1; // Set rotation clockwise
}
// Translate the canvas origin to the center
translate(centerX, centerY);
// Save the current settings
push();
// Rotate the canvas for the shape redraw
rotate(radians(angle * rotateDir));
// Draw background circle
fill(120, 88, 111);
stroke(120, 88, 111);
ellipse(0, 0, height, height);
// Draw center rectangle
fill(230, 199, 156);
stroke(230, 199, 156);
rectMode(CENTER); // Center rect around (0,0)
rect(0,0, rectSize, rectSize);
rect(0, 0, rectSize * mouseX/15, rectSize * mouseX/15);
// Draw center crossing rectangles
if(colorScheme == 1){
fill(123, 158, 168); // grey blue
stroke(123, 158, 168);
} else {
fill(111, 208, 140); // green
stroke(111, 208, 140);
}
rect(0, 0, 25, width * 1.5);
rect(0,0, width * 1.5, 25);
// Draw little circle
fill(120, 88, 111);
stroke(120, 88, 111);
ellipse(0, 0, littleCircle * mouseX/15, littleCircle * mouseX/15);
// Draw four spokes of ellipses and diamonds
// at 90 degree intervals
drawEllipse();
drawRects();
rotate(radians(90));
drawEllipse();
drawRects();
rotate(radians(90));
drawEllipse();
drawRects();
rotate(radians(90));
drawEllipse();
drawRects();
// Return to saved settings
pop();
angle = angle + mouseY/100;
}
function drawEllipse(){
// Set color of ellipses
if(colorScheme == 1){
fill(111, 208, 140); // green
stroke(111, 208, 140);
} else {
fill(123, 158, 168); // grey blue
stroke(123, 158, 168);
}
var circleSize = circleStart;
var circleInc = 5;
circleSize = circleSize * (width - mouseX)/150;
// Draw a line of ellipses
ellipse(shapeSpace, shapeSpace, circleSize, circleSize);
// Draw first row dots
var dotSize = 4;
push(); // Save settings
fill(205, 223, 160); // yellow
stroke(0);
rect(shapeSpace, shapeSpace, circleSize/dotSize, circleSize/dotSize);
pop(); // restore settings
circleSize += circleInc;
ellipse(2 * shapeSpace, 2 * shapeSpace, circleSize, circleSize);
// Draw second row dots
push(); // Save settings
fill(120, 88, 111); // purple
rect(2 * shapeSpace, 2 * shapeSpace, circleSize/dotSize, circleSize/dotSize);
pop(); // restore settings
circleSize += circleInc;
ellipse(3 * shapeSpace, 3 * shapeSpace, circleSize, circleSize);
// Draw third row of dots
push(); // Save settings
fill(205, 223, 160); // yellow
stroke(0);
rect(3 * shapeSpace, 3 * shapeSpace, circleSize/dotSize, circleSize/dotSize);
pop(); // Restore settings
circleSize += circleInc;
ellipse(4 * shapeSpace, 4 * shapeSpace, circleSize, circleSize);
// Draw fourth row of dots
push(); // Save settings
fill(120, 88, 111); // purple
rect(4 * shapeSpace, 4 * shapeSpace, circleSize/dotSize, circleSize/dotSize);
}
function drawRects(){
// Draws a line of rectangles
// Set color of rectangles
fill(205, 223, 160); // yellow
stroke(120, 88, 111); // purple
// Save current settings
push();
// Rotate canvase so rectangles craw as diamonds
rotate(radians(45));
rectMode(CENTER); // draw rects from center
var sqSize = startSize * mouseX/100;
var sqInc = 5;
// Draw first rectangle
rect(shapeSpace, shapeSpace, sqSize, sqSize);
// Draw second rectangle
sqSize += sqInc;
rect(shapeSpace + 2 * sqSize, shapeSpace + 2 * sqSize, sqSize, sqSize);
// Draw third rectangle
sqSize += sqInc;
rect(shapeSpace + 3 * sqSize, shapeSpace + 3 * sqSize, sqSize, sqSize);
// Draw fourth rectangle
sqSize += sqInc;
rect(shapeSpace + 4 * sqSize, shapeSpace + 4 * sqSize, sqSize, sqSize);
// Draw dots
stroke(0);
fill(111, 208, 140); // green
var dotSize = 3;
ellipse(shapeSpace + 4 * sqSize, shapeSpace + 4 * sqSize, sqSize/dotSize,
sqSize/dotSize);
// third row dots
fill(120, 88, 111); // purple
sqSize -= sqInc; // Set sqSize to third square
ellipse(shapeSpace + 3 * sqSize, shapeSpace + 3 * sqSize, sqSize/dotSize,
sqSize/dotSize);
// second row dots
fill(111, 208, 140); // green
sqSize -= sqInc;
ellipse(shapeSpace + 2 * sqSize, shapeSpace + 2 * sqSize, sqSize/dotSize,
sqSize/dotSize);
// first row dot
fill(120, 88, 111); // purple
ellipse(shapeSpace, shapeSpace, sqSize/dotSize,
sqSize/dotSize);
// Return to saved settings
pop();
}
function mousePressed(){
colorScheme = -colorScheme;
}