I wanted to make my abstract clock a simple clean representation of time. I liked the idea of revolving the time around a center of normal analog clocks, but instead make it something that built up rather than ticking by.
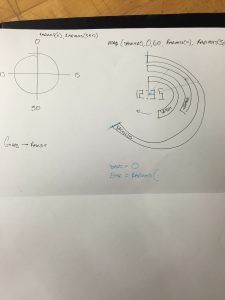
function setup() {
createCanvas(300, 300);
}
function draw() {
background(255);
//digital time vars
var s = second();
var m = minute();
var h = hour();
var hx = 125;//digital hour x location
var mx = 144;//digital minute x location
var sx = 164;//digital second x location
var ty = 154;//digital time y location
//types digital time
textStyle(BOLD);
text(nf(h%12, 2, 0) + ":", hx, ty);//digital hour
text(nf(m, 2, 0) + ":", mx, ty);//digital minute
text(nf(s, 2, 0), sx, ty);//digital second
//radius vars
var sRadius = 120;//second hand length
var mRadius = 90;//minute hand length
var hRadius = 60;//hour hand length
//circle arc angle vars
var sAngle = map(second(), 0, 60, radians(0), radians(360));//converting 60 sec to 360 degrees
var mAngle = map(minute(), 0, 60, radians(0), radians(360));//converting 60 min to 360 degrees
var hAngle = map(hour()%12, 0, 12, radians(0), radians(360));//converting 12 hr to 360 degrees
//x & y revolving locations
var sx = cos(sAngle) * sRadius;//rotating s hand X
var sy = sin(sAngle) * sRadius;//rotating s hand Y
var mx = cos(mAngle) * mRadius;//rotating m hand X
var my = sin(mAngle) * mRadius;//rotating m hand Y
var hx = cos(hAngle) * hRadius;//rotation h hand X
var hy = sin(hAngle) * hRadius;//rotation h hand Y
push();//begin drawing board
translate(width/2, height/2)
rotate(radians(270));//rotates from 0 or 2pi so starting point is upper vertical
stroke("#F18F32");//orange seconds
noFill();
strokeWeight(20);
arc(0, 0, sRadius * 2, sRadius * 2, 0, sAngle);
dot(sx, sy);
stroke("#E186CF")//purple minutes
noFill();
strokeWeight(20);
arc(0, 0, mRadius * 2, mRadius * 2, 0, mAngle);
dot(mx, my);
stroke("#2CD9DE")//blue hours
noFill();
strokeWeight(20);
arc(0, 0, hRadius * 2, hRadius * 2, 0, hAngle);
dot(hx, hy);
pop();
}
function dot(x, y){
fill(255);
stroke(0);
strokeWeight(2);
ellipse(x, y, 20, 20);
}