Click ‘u’ to move grid amount up and ‘d’ to move grid amount down!!!
(If it doesn’t work make sure your caps lock is off and click on the image first before typing)
//Taisei Manheim
//Section C
//tmanheim@andrew.cmu.edu
//Assignment-09
var underlyingImage;
//starting grid size
var grid = 20;
function preload() {
var myImageURL = "https://i.imgur.com/9Jjikbd.jpg";
underlyingImage = loadImage(myImageURL);
}
function setup() {
createCanvas(360, 480);
background(255);
underlyingImage.loadPixels();
}
function draw() {
//creates grid of circles
noStroke();
for (var x = 0; x < underlyingImage.width; x += grid) {
for (var y = 0; y < underlyingImage.height; y += grid) {
var theColorAtLocationXY = underlyingImage.get(x, y)
fill(theColorAtLocationXY);
ellipse(x, y, grid, grid);
}
}
}
function keyTyped() {
//if grid reaches 5 it wont get smaller (or else it would crash)
if (grid < 5) {
grid = 5;
}
//if grid reaches 100 it wont get bigger
if (grid > 100) {
grid = 100;
}
//if you hit u key size of grid would increase
if (key === 'u') {
clear(); //need this or else old grids would show underneath
grid += 1
}
//if you hit d key size of grid would decrease
if (key === 'd') {
clear(); //need this or else old grids would show underneath
grid -= 1
}
}
For my portrait I did a picture of myself. When I first started this project I was experimenting with the amount of circles in a grid to create a more abstract or realistic portrait. I then thought it would be interesting to have it controllable so that people looking at the portrait could control the resolution. I tried to tie the grid size to mouse location at first but it was too much for the program to process that quickly so I made it so that you hit the ‘u’ and ‘d’ key to move the grid amount up and down.
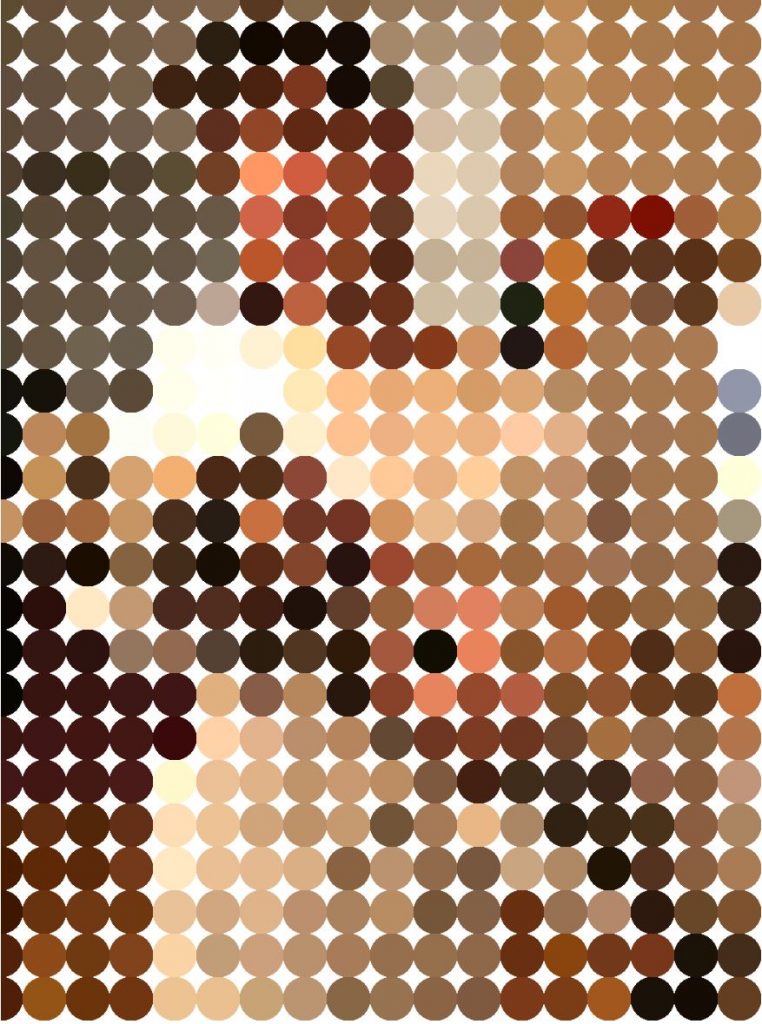
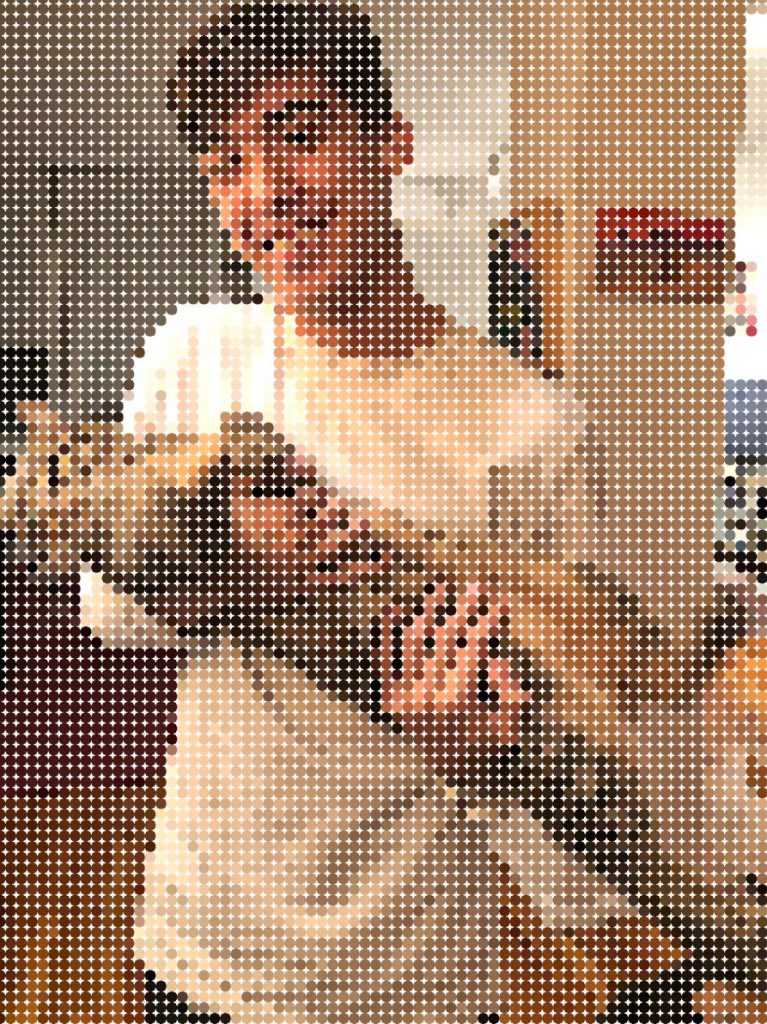
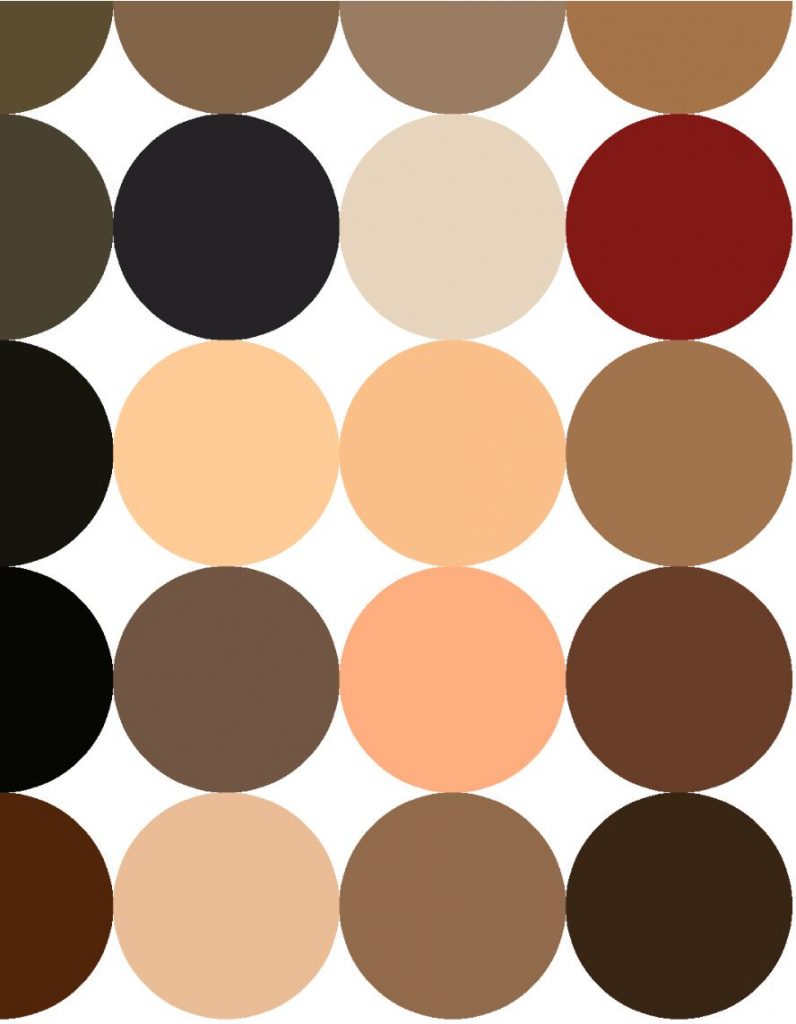
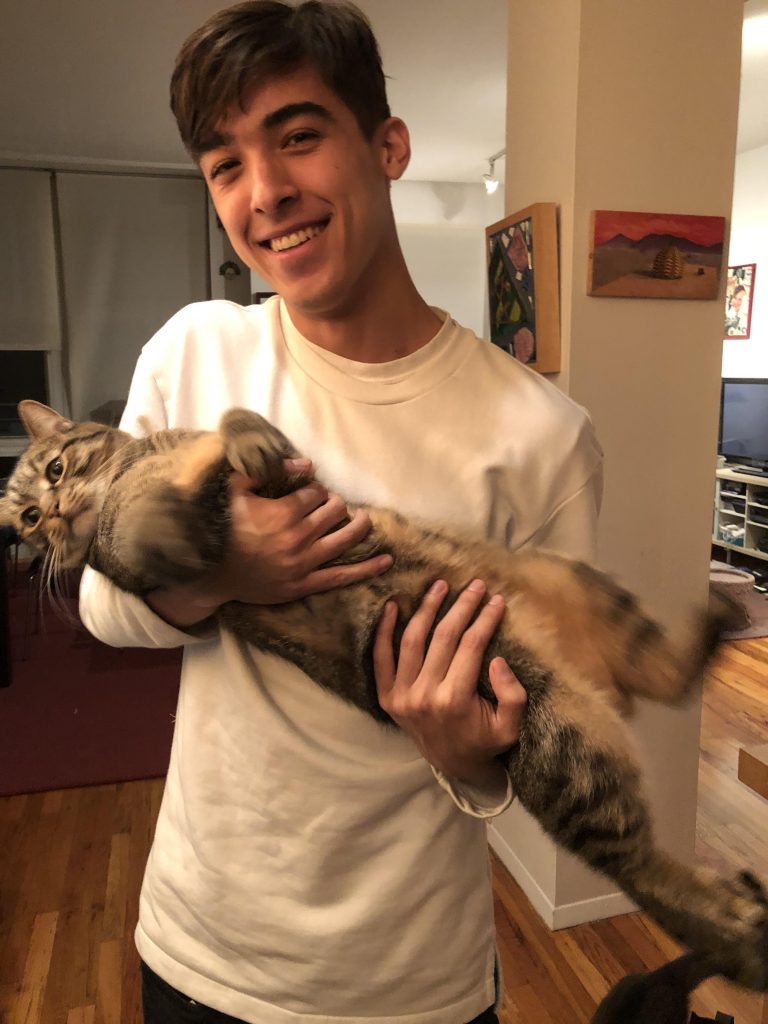