//Taisei Manheim
//Section C
//tmanheim@andrew.cmu.edu
//Assignment-10
var trees = [];
var frames;
function preload() {
//background gradient
backgroundImage = loadImage("https://i.imgur.com/L0VpcqE.jpg")
//frames for person animation
frames = [];
frames[0] = loadImage("http://i.imgur.com/svA3cqA.png");
frames[1] = loadImage("http://i.imgur.com/jV3FsVQ.png");
frames[2] = loadImage("http://i.imgur.com/IgQDmRK.png");
frames[3] = loadImage("http://i.imgur.com/kmVGuo9.png");
frames[4] = loadImage("http://i.imgur.com/jcMNeGq.png");
frames[5] = loadImage("http://i.imgur.com/ttJGwkt.png");
frames[6] = loadImage("http://i.imgur.com/9tL5TRr.png");
frames[7] = loadImage("http://i.imgur.com/IYn7mIB.png");
}
function setup() {
createCanvas(480, 480);
// create an initial collection of trees
for (var i = 0; i < 10; i++){
var rx = random(width);
trees[i] = makeTree(rx);
}
frameRate(10);
}
function draw() {
image(backgroundImage, 0, 0, width * 2, height);
mountain();
mountain2();
//ground
fill(210,218,255);
rect(-1, height-50, width + 1 , 50)
updateAndDisplayTrees();
removeTrees();
addNewTrees();
//person on ground
push();
scale(.35, .35);
image(frames[frameCount % 8], width * 2.75, height * 2.33);
pop();
}
//upper mountain
function mountain() {
var speed = 0.0005;
var terrain = 0.01;
stroke(70,119,187);
for (var x = 0; x < width; x += 1) {
var t = (x * terrain) + (millis() * speed);
var y = map(noise(t), 0, 1, 0 + 100, height / 2 + 100);
line(x, y, x, height);
}
//person on mountain
push();
scale(.10, .10);
image(frames[frameCount % 8], width * 9.85, y * 10 - 100);
pop();
}
//lower mountain
function mountain2() {
var speed = 0.0003;
var terrain = 0.005;
stroke(50,99,167);
for (var x = 0; x < width; x += 1) {
var t = (x * terrain) + (millis() * speed);
var y = map(noise(t), 0, 1, height / 2 + 150, height / 4 + 150);
line(x, y, x, height);
}
//person on mountain
push();
scale(.25, .25);
image(frames[frameCount % 8], width * 3.9, y * 4 - 110);
pop();
}
function updateAndDisplayTrees(){
// Update the tree's positions, and display them.
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
function removeTrees(){
// Copy all the trees we want to keep into a new array.
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x + trees[i].treeWidth > 0) {
treesToKeep.push(trees[i]);
}
}
trees = treesToKeep; // remember the surviving trees
}
function addNewTrees() {
// With a very tiny probability, add a new tree to the end.
var newTreeLikelihood = 0.05;
if (random(0,1) < newTreeLikelihood) {
trees.push(makeTree(width));
}
}
// method to update position of tree every frame
function treeMove() {
this.x += this.speed;
}
// draw the tree
function treeDisplay() {
//tree leaves
fill(22,138,130);
noStroke();
push();
translate(this.x, height - 60);
triangle(0, -this.treeHeight, 0 - this.treeWidth / 2, 0, 0 + this.treeWidth / 2, 0)
pop();
//tree trunk
fill(40,59,107);
push();
translate(this.x, height - 60);
rect(- 2.5, 0, 5, 10);
pop();
}
function makeTree(birthLocationX) {
var tr = {x: birthLocationX,
treeWidth: random(20,30),
speed: -5.0,
treeHeight: random(30,60),
move: treeMove,
display: treeDisplay}
return tr;
}
For this project I spent some time messing around with different colors and mountain heights in order to get a look that I liked. I couldn’t get the sky to gradient in a way that I thought looked good so I used an image to create the gradient. The trees are at random heights and come at random intervals. The hardest part was to get the racing people on the right to run along the mountains rather than at a consistent y-value. I had the people decrease in size in order to give a sense of depth, but it was difficult to control the movements of the people once they were scaled down. Overall, I am pretty happy with this project.
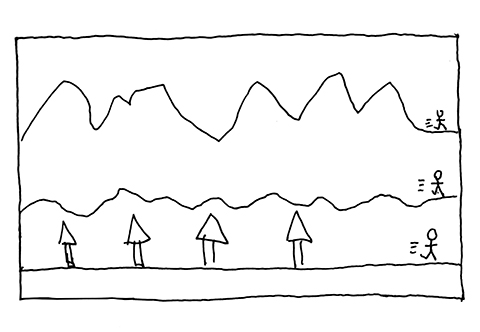