// Ankitha Vasudev
// Section B
// ankithav@andrew.cmu.edu
// Project-11
// global variables
var birds = []; // bird objects
var terrainSpeed1 = 0.0005; // back mountains speed
var terrainDetail1 = 0.015; // back mountains detail
var terrainSpeed2 = 0.001; // front mountains speed
var terrainDetail2 = 0.008; // front mountains detail
function setup() {
createCanvas(450, 400);
// create an initial collection of birds
for (var i = 0; i<10; i++) {
var rx = random(width);
birds[i] = makeBirds(rx);
}
frameRate(10);
}
function draw() {
background(80);
// window arc frame
fill(230);
arc(width/2, 400, width*2, 750, PI, 0);
// landscape elements
sun();
backMountains();
carWindowandRoad();
frontMountains();
mountainreflection();
// displaying birds
updateAndDisplayBirds();
removeOldBirds();
addNewBirds();
// vertical window frame line
stroke(0);
strokeWeight(7);
line(75, 50, 75, height);
}
function carWindowandRoad() {
// window frame
stroke(0);
strokeWeight(7);
noFill();
arc(width/2, 400, width*2, 750, PI, 0);
// water
noStroke();
fill(230);
rect(0, 340, width, 100);
// road
stroke(255);
strokeWeight(2);
fill(100);
rect(-10, 380, width+20, 70);
// bottom window frame
noStroke();
fill(0);
rect(0, 393, width, height);
}
function backMountains() {
// large mountains in the back
stroke(215);
beginShape();
for(var x=0; x<=width; x++) {
var t = (x * terrainDetail1) + (millis() * terrainSpeed1);
var y = map(noise(t), 0, 1, 100, 350);
line(x, y, x, 380);
}
endShape();
}
function frontMountains() {
// smaller mountains in the front
stroke(150);
beginShape();
for(var x=0; x<=width; x++) {
var t = (x * terrainDetail2) + (millis() * terrainSpeed2);
var y = map(noise(t), 0, 2, 300, 200);
line(x, y, x, 340);
}
endShape();
}
function mountainreflection() {
// reflection of small mountains in the water
stroke(200);
beginShape();
for(var x=0; x<=width; x++) {
var t = (x * terrainDetail2) + (millis() * terrainSpeed2);
var y = map(noise(t), 0, 5, 120, 250);
line(x, y+220, x, 340);
}
endShape();
}
function sun() {
//sun
noStroke();
fill(238);
ellipse(370,100, 60);
fill(247);
ellipse(370, 100, 55);
fill(255);
ellipse(370, 100, 50);
}
function updateAndDisplayBirds() {
// display and move birds
for (var i=0; i<birds.length; i++) {
birds[i].move();
birds[i].display();
}
}
function removeOldBirds() {
// remove old birds off canvas
var birdsToKeep = [];
for (var i=0; i<birds.length; i++) {
if (birds[i].x + birds[i].breadth > 0) {
birdsToKeep.push(birds[i]);
}
}
birds = birdsToKeep;
}
function addNewBirds() {
// add new birds with a probability of 0.05
var newBirds = 0.05;
if (random(0,1) < newBirds) {
birds.push(makeBirds(width));
}
}
function moveBirds() {
this.x += this.speed;
}
function displayBirds() {
// code to draw each bird
noFill();
stroke(this.col);
strokeWeight(0.8);
arc(this.x, this.y, this.width, this.height, PI, TWO_PI);
arc(this.x+this.width, this.y, this.width, this.height, PI, TWO_PI);
}
function makeBirds(birthLocationX) {
// randomization of birds
var birdy = {x: birthLocationX,
y: random(140, 230),
col: random(30,100),
breadth: 50,
speed: random(-3,-6),
width: random(3,8),
height: random(2,4),
move: moveBirds,
display: displayBirds
}
return birdy;
}
For this project I created a generative landscape of a view out a car window. I wanted to create a strong sense of foreground vs background and did this by including many elements: mountains in the back and front, water with the mountain reflections and a thin strip of the road. I used birds as my objects and randomized the width, height and color. I also used this project as an opportunity to play with a monotone color scheme (since all my previous project use a lot of color). Overall, it was fun and I’m much more confident about using objects.
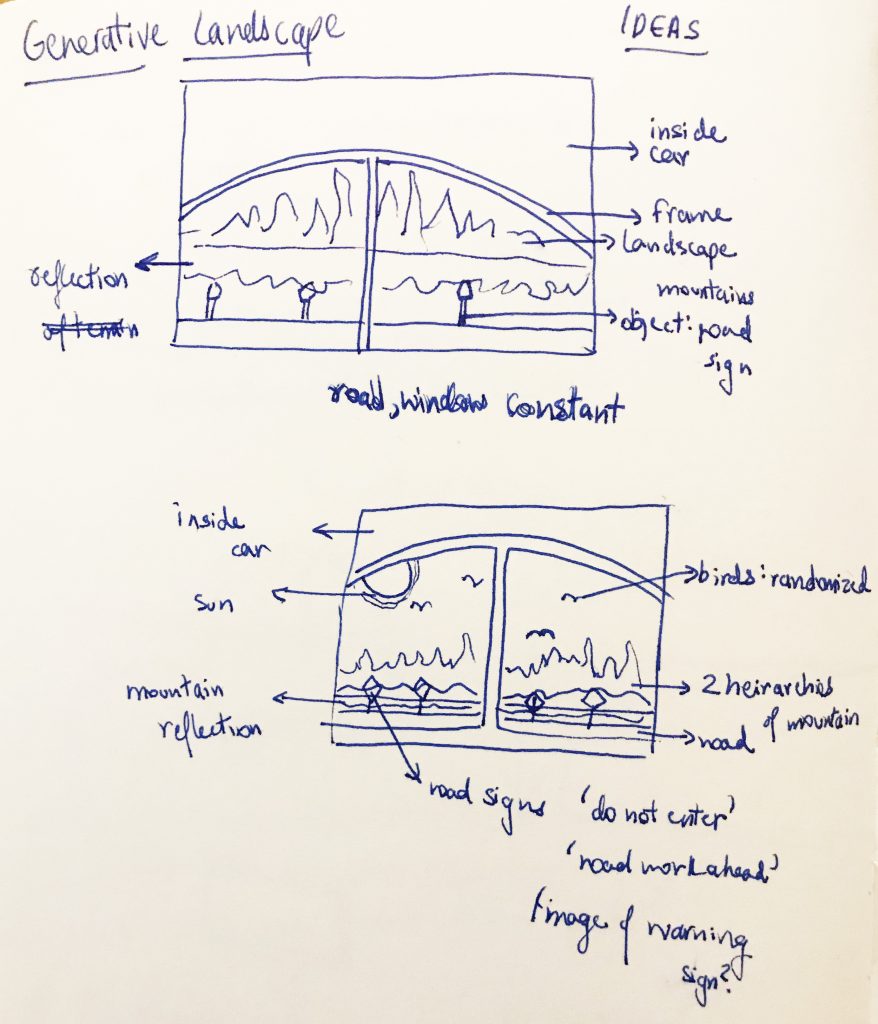