//Mihika Bansal
//mbansal@andrew.cmu.edu
//Section E
//Project 9
var bubbles;
var move = 5;
var cx = 100; //starting location of clouds
var cy = 100;
var clouds = [];
var ripples = [];
var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
function setup() {
createCanvas(480, 480);
frameRate(4); //slowed down for ripples
//initial display of clouds
for(var i = 0; i < 3; i++){
var cX = random(width);
var cY = random(75, 150);
clouds[i] = makeClouds(cX, cY);
}
}
function draw() {
background("#F05353");
//draw sun
noStroke();
fill("#F7915B");
ellipse(width / 2, height / 3, 125, 125);
var mountain1 = mountains("#E2BE9A", height * 0.20, height * 0.65, 4); //backmost mountain
var mountain2 = mountains("#EFAD6C", height * 0.37, height * 0.65, 2); // middle ground mountains
var mountain3 = mountains("#EF8F30", height * 0.50, height * 0.65, 3); //foreground mountains
//draw ocean
noStroke();
fill("#006160");
rect(0, height * 0.65, width, height * 0.35);
//draw sun reflection
noStroke();
fill(250, 103, 71, 100);
arc(width / 2, height * 0.65, 125, 125, 0, PI, OPEN);
//draw ripples that are changing like waves
noStroke();
fill(171,209,197, 100);
for(var i = 0; i < 6; i ++){
var xR = random(-205, 205);
var yR = random(10, 125);
var w = random(50, 100);
var h = random(5, 10);
ellipse(width / 2 + xR, height * 0.65 + yR, w, h);
}
update();
removeClouds();
addCloud();
}
function update(){ //update all the clouds
for(var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].display();
}
}
function removeClouds(){ //checks to see where clouds are and changes positions accordingly
var cloudKeep = [];
for(var i = 0; i < clouds.length; i++){
if(clouds[i].x + 25 > 0){ // cloud is 50 wide, so 25 is point from center
cloudKeep.push(clouds[i]);
}
}
clouds = cloudKeep; //keep only clouds still on screen
}
function addCloud(){ //randomly adds clouds at intervals
var newCloud = 0.03;
if(random(0,1) < newCloud){
var cloudY = random(75, 150);
clouds.push(makeClouds(480, cloudY));
}
}
function cloudMove(){ // move the clouds
this.x += this.speed;
}
function cloudDisplay(){ //draw the clouds
fill("#FFD79E");
noStroke();
ellipse(this.x, this.y, 90, 15);
ellipse(this.x - 25, this.y - 10, 35, 30);
ellipse(this.x, this.y - 20, 40, 40);
ellipse(this.x + 20, this.y - 15, 35, 30);
}
function makeClouds(cloudLocX, cloudLocY){ //cloud object definition
var cloud = { x: cloudLocX,
y: cloudLocY,
speed: -5,
move: cloudMove,
display: cloudDisplay}
return cloud;
}
function mountains(color, min, max, noiseS) {
noStroke();
fill(color);
noiseSeed(noiseS);
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetail + (millis() * terrainSpeed));
var y = map(noise(t), 0, 1, min, max);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
This project was very fun to do. I was inspired by the mountain terrain that was shown in the deliverable section. I immediately thought to the serene sunset nights that a person could see when they are on a cruise ship. So I worked with this color palette and recreated an ocean scene with ripples in the ocean, a setting sun, and mountain ranges.
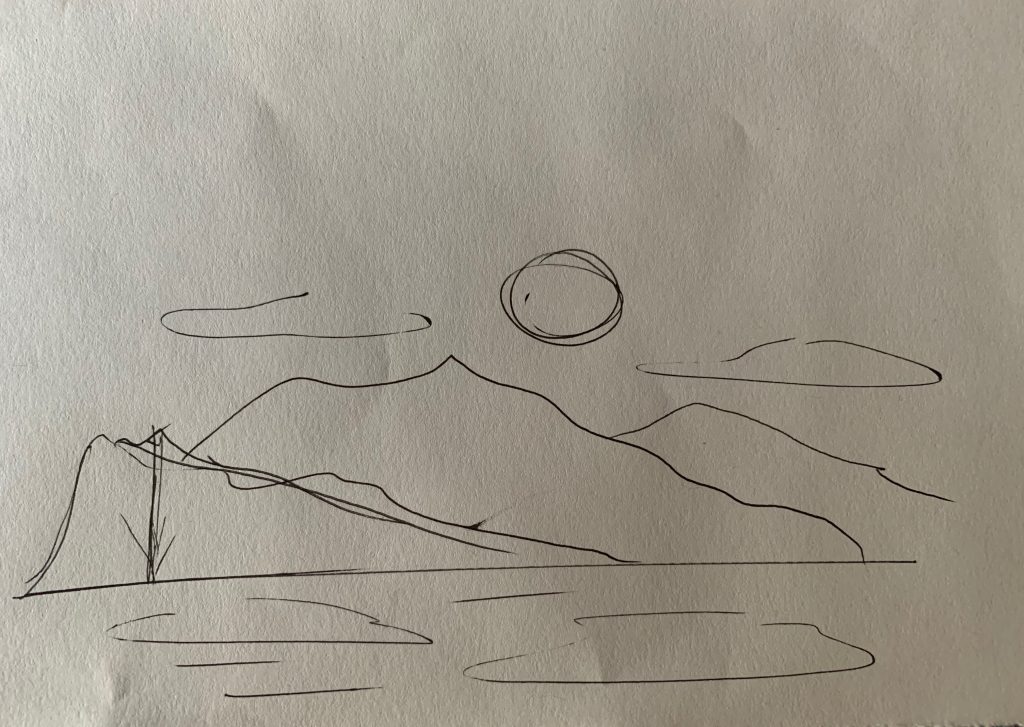