//Monica Chang
//mjchang@andrew.cmu.edu
//Section D
//Project 11 - Generative Landscapes
//LANDSCAPE DESCRIPTION:
// SURPRISE! THERE IS HIDDEN LAVA BEHIND THE FIRST TERRAIN!
var tethered = [];
var terrainSpeed = 0.0008;// speed of orange terrain and middle terrain
var terrainSpeedThree = 0.0007; // speed of very back mountain
var terrainDetail = 0.008;
var terrainDetailTwo = 0.001;
var terrainDetailThree = 0.02; //smoothness of the terrains
function setup() {
createCanvas(480, 480);
frameRate(20);
//initial lava
for (i = 0; i < 30; i++) {
var tx = random(width);
var ty = random(300, height);
tethered[i] = makeTethered(tx, ty);
}
}
function draw() {
//lavendar background
background(236, 225, 250);
//arranging the landscape elements(three terrains, lava spots)
renderTerrainTwo(); // middle, low-opacity mountain
renderTerrainThree(); // third mountain in the very back
updateAndDisplayTethered(); //hidden lava behind the front terrain
renderTerrainOne(); // first terrain int he very front
}
function displayTethered() {
//drawing the "tethered" lava
noStroke(); //no outline
fill(255, 11, 5); //red tethered coat color
push();
translate(this.x0, this.y0); //locate lava body at x0, y0
ellipse(5, 5, 10, 5); //tethered lava body
pop();
}
function makeTethered(birthLocationX, birthLocationY) {
var theTethered = {x0: birthLocationX,
y0: birthLocationY,
tx: random(0, width),
ty: random(300, height),
speed: -3.0,
move: moveTethered,
display: displayTethered}
return theTethered;
}
function moveTethered() {
this.x0 += this.speed; //speed of lava moving
if (this.x0 <= -10) { //new lava appears at the right as they disappear to the left
this.x0 += width + 10;
}
}
function updateAndDisplayTethered() {
for(i = 0; i < tethered.length; i++) {
tethered[i].move();
tethered[i].display();
}
}
function renderTerrainThree(){
// drawing the terrain in the back
noStroke();
fill(51, 16, 84);
beginShape();
for (i = 0; i < width; i++) {
var t = (i * terrainDetailThree) + (millis() * terrainSpeedThree);
//terrains y coordinate
var y = map(noise(t), 0, 1.5, height / 8, height);
//keep drawing terrain
vertex(i, y);
}
//terrain constraints
vertex(width, height);
vertex(0, height);
endShape();
}
function renderTerrainTwo() {
// drawing terrain number two(in the middle)
noStroke();
fill(71, 11, 6, 200); //low-opacity color of maroon
beginShape();
for(var a = 0; a < width; a++){
var b = (a * terrainDetail) + (millis() * terrainSpeed);
var c = map(noise(b), 0, 1, 0, height / 4);
vertex(a, c);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
function renderTerrainOne() {
//drawing the terrain in the very front
noStroke();
fill(235, 64, 52);
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetailTwo) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, 0.55, height + 100);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
I was originally inspired by the horror film, ‘Us’, which was released this year and wanted to illustrate the “Tethered”. However, they ended up looking more like lava due to the color so I ended up creating a landscape with holes of lava. This project was really fun and helpful in making me understand objects better.
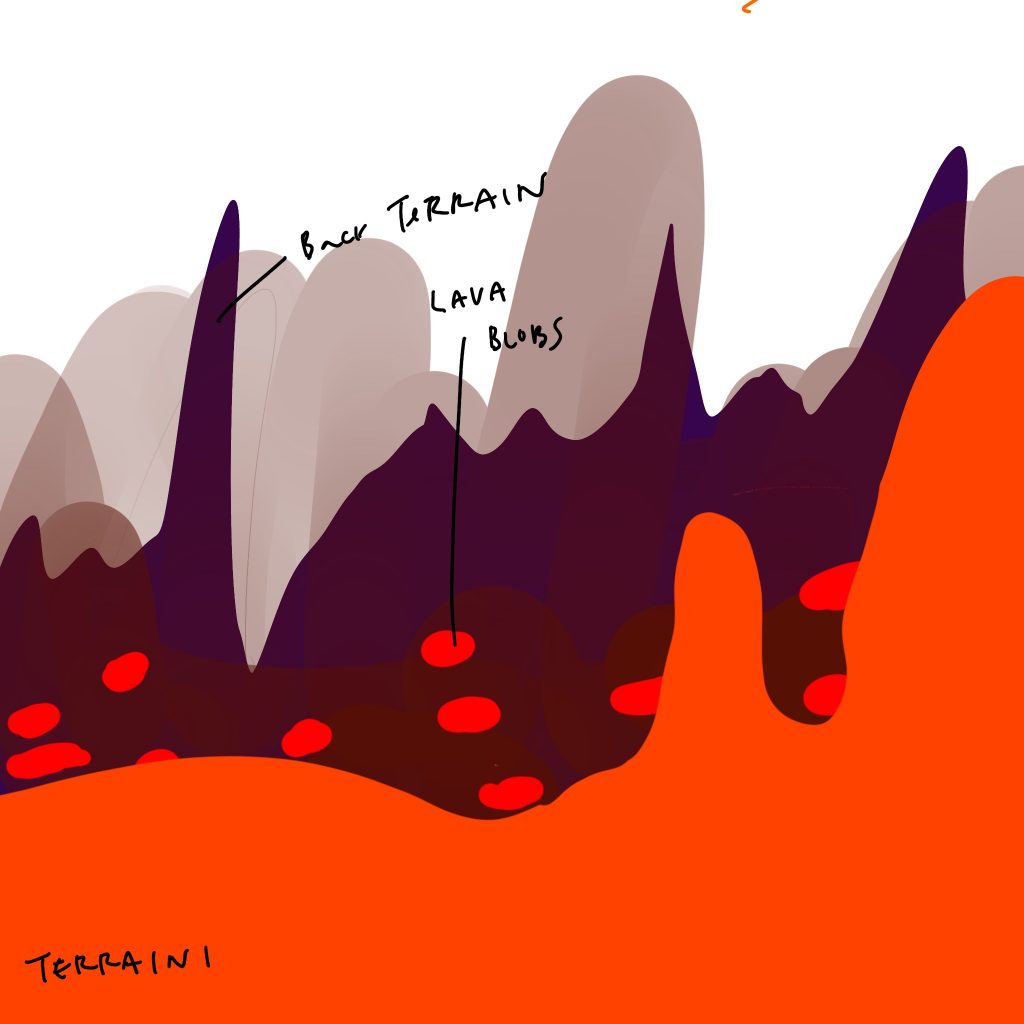