I had to upload through a zip file because my canvas size was 650 in width(a little over the max size for WordPress). Thus, continue reading to learn how to access the game.
Instructions on accessing the game: To begin this game, you must download the zip file attached above. Once the file is downloaded, a zip file called “Monica-Chang-Final-Project” should appear. Open the file and a folder called “104final” will appear; then, open “sketch.js” to access the code behind it.
Because this is outside of WordPress and my code has implemented sound, it is crucial to trigger the sound file playback and open the game by following the instructions below(taken from Lab Week 10).
- Open a Terminal in OS X or a command window (
cmd
) in Windows. - Change your current directory to the directory you want to serve:Type
cd path-to-your-directory
(ex. cd Desktop/104final ) - Type in Terminal:
python -m SimpleHTTPServer
Or if you are using Python 3, type:python -m http.server
- Visit the URL
http://localhost:8000
in your browser to test your sketch.
Description: For this final project, I developed a game in which the player or virtual “photographer” will be responsible for capturing pictures of the flying birds with the virtual camera by pressing the SPACEBAR. The problem is the birds fly by way too fast but the players are required to capture enough pictures to reach “captured” score of 100. There are no losing points in this game.
Another element that I implemented is changing the weather. Since the initial weather is gloomy and raining, the player may press his/her mouse to change the screen to a happy, blue sky. The field audio/sounds will change accordingly.
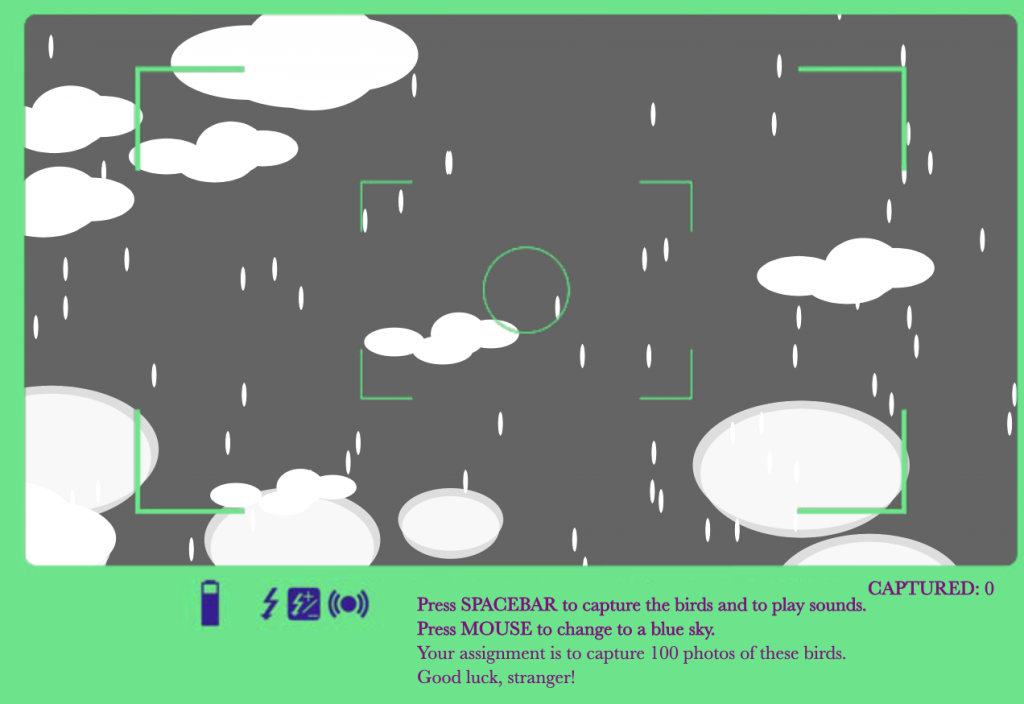
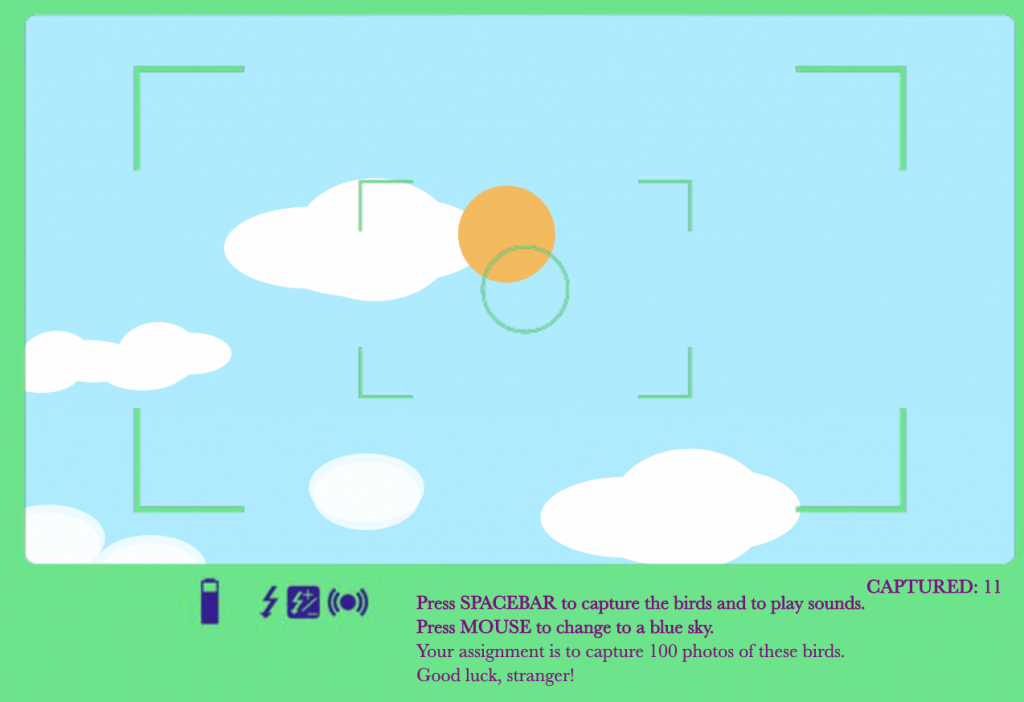
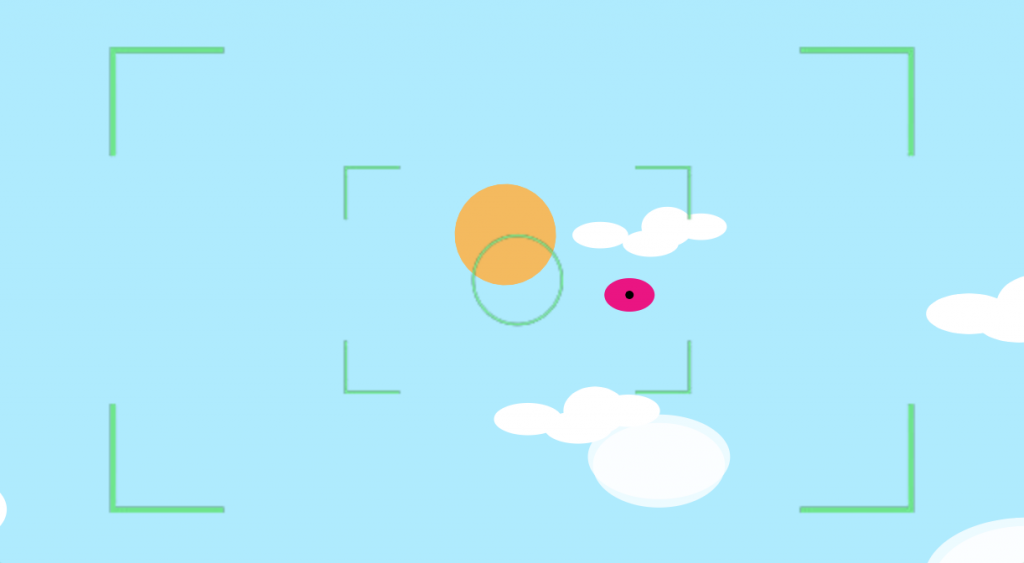