/* Cathy Dong
Section D
yinhuid@andrew.cmu.edu
Project 11 - Landscape
*/
// mountain slope and move speed variables
var tDetail1 = 0.002;
var tSpeed1 = 0.0001;
var tDetail2 = 0.005;
var tSpeed2 = 0.0003;
var tDetail3 = 0.01;
var tSpeed3 = 0.0008;
// mountain colors
var terrC1 = "#597081";
var terrC2 = "#7EA0B7";
var terrC3 = "#D6EEFF";
//mountain heights range
var h1Min = 0;
var h1Max = 300;
var h2Min = 150;
var h2Max = 400;
var h3Min = 250;
var h3Max = 480;
// star variables
var sList = [];
var sNum = 20; // number of stars
var sLeft = -20; // reset start to canvas right
var sSpeed = -0.5;
// car variables
var cList = [];
var cHeight = 20; // car height
var cWidth = 50; // car width
var cNum = 5; //number of cars
var cLeft = -20 - cWidth; // reset car to start
var cSpeed = -1.5; // car speed
var wSize = 10; // car wheel size
function setup() {
createCanvas(480, 480);
// star start at random px and py
for (var j = 0; j < sNum; j++) {
var px = random(width);
var py = random(height / 3);
sList[j] = starMake(px, py);
}
// car cx and cy
var ground = height - height / 15;
for (var k = 0; k < cNum; k++) {
var cx = random(width);
var cy = ground - wSize / 2 - cHeight;
cList[k] = carMake(cx, cy);
}
}
function draw() {
// sky color
background("#2B2D42");
// render stars
starRender();
// render mountains
terrainDraw(tDetail1, tSpeed1, terrC1, h1Min, h1Max);
terrainDraw(tDetail2, tSpeed2, terrC2, h2Min, h2Max);
terrainDraw(tDetail3, tSpeed3, terrC3, h3Min, h3Max);
// draw ground
fill("#8D99AE");
rect(0, height - height / 15, width, height / 15);
// render car
carRender();
}
// mountain draw function
function terrainDraw(terrainDetail, terrainSpeed, terrC, terrMin, terrMax) {
noStroke();
// fill with layer color
fill(terrC);
// start drawing the shape
beginShape();
vertex(0, height);
// generate terrian
for (var i = 0; i < width; i++) {
var t = (i * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, terrMin, terrMax);
vertex(i, y);
}
vertex(width, height);
endShape();
}
// star object
function starMake(px, py) {
var star = {x: px, y:py, speed: sSpeed,
update: starUpdate,
draw: starDraw}
return star;
}
// update star px and py
function starUpdate() {
this.x += this.speed;
if (this.x <= sLeft) {
this.x += width - sLeft;
}
}
// draw star at this.x and this.y location
function starDraw() {
noStroke();
fill("yellow");
var size = random(1, 5); // make it shine
push();
translate(this.x, this.y);
circle(0, 0, size);
pop();
}
// render the star based on previous info
function starRender() {
for (var i = 0; i < sList.length; i++) {
sList[i].update();
sList[i].draw();
}
}
// car info
// a is car x position; b is car y position
function carMake(cx, cy) {
var car = {a: cx, b: cy, speed: cSpeed, c: color(random(100), random(0), random(255)),
update: carUpdate,
draw: carDraw}
return car;
}
// update car position
function carUpdate() {
this. a += this.speed;
if(this.a <= cLeft) {
this.a += width - cLeft;
}
}
// draw initial car
function carDraw() {
// wheel location
var wX1 = cWidth / 4;
var wX2 = cWidth - wX1;
noStroke();
push();
translate(this.a, this.b);
// draw car
fill(this.c);
rect(0, 0, cWidth, cHeight);
// draw car wheels
fill(0);
circle(wX1, cHeight, wSize);
circle(wX2, cHeight, wSize);
pop();
}
// render car to this.a and this.b
function carRender() {
for (var m = 0; m < cList.length; m++) {
cList[m].update();
cList[m].draw();
}
}
The idea is to create a night scene on a highway. In the scene, stars and mountains move relative to the cars in a various speed based on depth relationship.
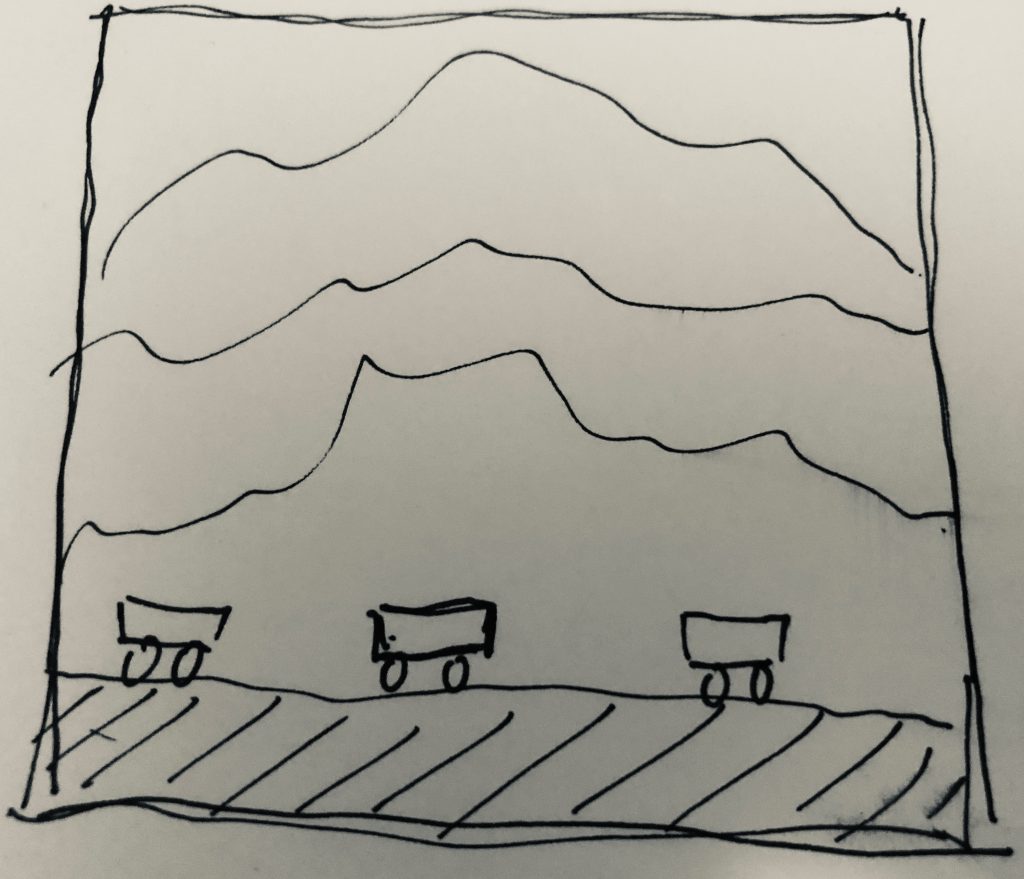