// Jina Lee
// jinal2@andrew.cmu.edu
// Project 11
// Section E
var sushi = [];
var count = 0;
var riceSize = 70;
function setup() {
createCanvas(480, 280);
// for loop to create the sushi
for (var i = 0; i < 5; i += 20){
sushi[i] = makeSushi(width);
}
frameRate(120);
}
function draw() {
count += 1;
// beige
background(207, 185, 151);
sushiText();
sushiConveyorBelt();
updateAndDisplaySushi();
removeSushiThatHaveSlippedOutOfView();
addNewSushiConstantly();
}
function sushiText(){
// frame for sign
fill(150, 0, 0);
rect(340, 22, 120, 48, 5);
// white sign
fill(255);
rect(350, 28, 100, 35, 5);
// turned on open sign
fill(150, 0, 0);
textSize(18);
text("OPEN", 370, 52);
// menu
fill(255);
rect(50, 22, 250, 150);
fill(0);
textSize(30);
text("menu", 70, 60);
stroke(0);
strokeWeight(3);
line(75, 100, 240, 100);
line(75, 80, 280, 80);
line(75, 90, 280, 90);
line(75, 110, 280, 110);
line(75, 120, 100, 120);
line(75, 130, 240, 130);
line(75, 140, 240, 140);
line(75, 150, 280, 150);
}
function updateAndDisplaySushi(){
for (var i = 0; i < sushi.length; i++){
sushi[i].move();
sushi[i].display();
}
}
function removeSushiThatHaveSlippedOutOfView(){
var sushiToKeep = [];
for (var i = 0; i < sushi.length; i++){
if (sushi[i].x + sushi[i].breadth > -200) {
sushiToKeep.push(sushi[i]);
}
}
sushi = sushiToKeep;
}
// keeps adding sushi to the end
function addNewSushiConstantly() {
if (count > 270) {
sushi.push(makeSushi(width));
count = 0;
}
}
// update sushi position every frame
function sushiMove() {
this.x += this.speed;
}
// draw the sushi
function sushiDisplay() {
var Height = 30;
fill(255);
noStroke();
push();
translate(this.x, height - 40);
// plate
fill(161, 206, 94);
ellipse(35, -Height/7, 110, 30)
rect(5, 5, 60, 10);
// rice
fill(255);
rect(0, -Height, riceSize, 30);
// eel
fill(139,69,19);
rect(0, -Height - 20, riceSize, 20);
// seaweed
fill(0);
rect(25, -Height - 20, riceSize/4, 20);
// plate
fill(16, 52, 166);
ellipse(175, -Height/7, 110, 30);
rect(145, 5, 60, 10);
// rice
fill(255);
rect(140, -Height, riceSize, 30);
// tuna
fill(234, 60, 83);
rect(140, -Height - 20, riceSize, 20);
pop();
}
function makeSushi(birthLocationX) {
var sushi = {x: birthLocationX,
breadth: 60,
speed: -1.0,
move: sushiMove,
display: sushiDisplay}
return sushi;
}
function sushiConveyorBelt() {
// grey
stroke(200);
strokeWeight(30);
line (0, height - 10, width, height - 10);
}
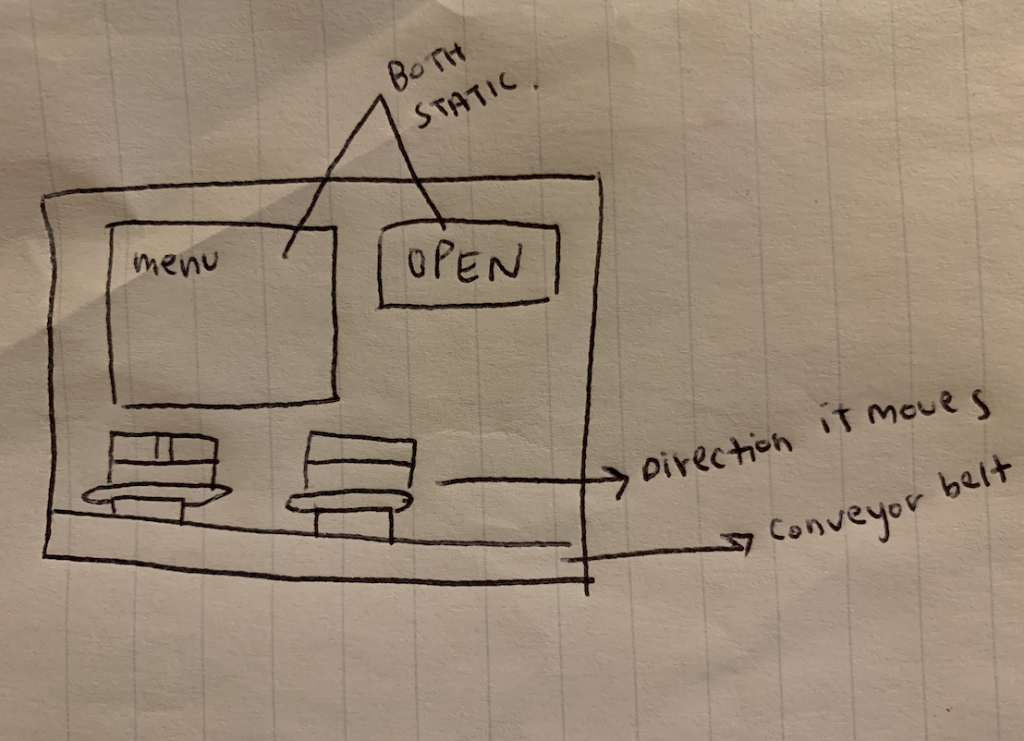
For this project, I am creating a sushi conveyor belt. I was inspired because back home, there is an all you can eat sushi restaurant that has sushi on a conveyor belt. The sushi is what moves. I made the signs static so it seems as if you are sitting at the table watching the sushi pass through. I created eel and tuna sushi.