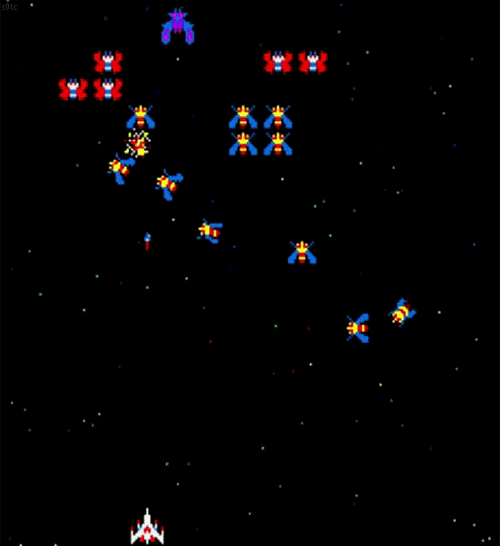
Soource: Galaga
For this project, I wanted to maximize randomization of the space objects, but still have some sort of coherency. Mostly, I was trying to become more comfortable with creating different classes of objects.
//Ghalya Alsanea
//galsanea@andrew.cmu.edu
//Section B
//Project 11
var lines;
var totalLines = 0;
var j; //jiggle
var f; //flower
var p1, p2, p3; //planets
var sp; //spinning planet
//stars
var star;
var star2;
function preload() {
//
}
function setup() {
createCanvas(600, 600);
background(0);
lines = new Array(500);
//initiate the dif class ojects
j = new Jiggle();
f = new Flower();
p1 = new Planet();
p2 = new Planet2();
p3 = new Planet3();
sp = new SpinPlanet();
star = new Star();
star2 = new Star();
}
function draw() {
background(0);
//create the shooting starts lines
lines[totalLines] = new Line();
totalLines++;
for (var i = 0; i < totalLines; i++) {
lines[i].move();
lines[i].display();
}
//show and move the objects
//jiggle circle
j.move();
j.show();
//flower
f.move();
f.show();
// planets
p1.move();
p1.show();
p2.move();
p2.show();
p3.move();
p3.show();
//spinplanet
sp.move();
sp.show();
//star 1
star.move();
star.show();
//star 2
star2.move();
star2.show();
}
//////////////////////////////////////////////////////////////////////
// define the classes //
//////////////////////////////////////////////////////////////////////
class Jiggle {
constructor() {
this.x = random(0, width); //start at a random xpos
this.y = height + 100; //make sure to start off the canvas
this.size = random(20, 50); //create a new size
this.col = color("orange"); //define the color
}
move() {
this.y-=2; //move up
this.x = this.x + random(-5, 5); //make the "jiggle" movement
//if it moves too far up, reset the object
if (this.y < -this.size) {
this.y = height;
this.x = random(0, width);
this.size = random(20, 50);
}
}
show() {
//draw the object
noStroke();
strokeWeight(1);
fill(this.col);
circle(this.x, this.y, this.size);
}
}
//////////////////////////////////////////////////////////////////////
class Flower {
constructor() {
this.x = random(0, width); //start at a random xpos
this.y = height + 100; //start y loc off the canvas
this.size = random(40, 80); //randomize size
}
move() {
this.y--; //move up
this.x = this.x + random(-1, 1);//cause the jiggle
//reset if it goes too far up
if (this.y < -this.size) {
this.y = height;
this.x = random(0, width);
this.size = random(40, 80);
}
}
show() {
// draw the object
noStroke();
fill(204, 101, 192, 180);
//rotate
push();
translate(this.x, this.y);
for (var i = 0; i < 10; i++) {
ellipse(0, 30, this.size / 6, this.size);
rotate(PI / 5);
}
pop();
}
}
//////////////////////////////////////////////////////////////////////
class Star {
constructor() {
this.x = random(0, width); //start at random xpos
this.y = height - 50; //start y loc off canvas
this.col = color("yellow");
this.npoints = random(5, 12); //points in star
this.angle = TWO_PI / this.npoints;
this.halfAngle = this.angle / 2.0;
this.r1 = random(5, 20); //inner radius
this.r2 = random(20, 40); //outer radius
}
move() {
this.x;
this.y = this.y - random(0.8, 1.2); //move in a random speed
// reset if it goes off cnavas
if (this.y < -100) {
this.y = height;
this.y -= random(0.8, 1.2);
this.x = random(0, width);
this.npoints = random(5, 12);
this.angle = TWO_PI / this.npoints;
this.halfAngle = this.angle / 2.0;
this.r1 = random(10, 30);
this.r2 = random(60, 100);
}
}
show() {
//draw the object
noStroke();
fill(this.col);
//rotate it
push();
translate(this.x, this.y);
rotate(radians(frameCount)/2);
beginShape();
//draw a star based on angles and npoints
for (var a = 0; a < TWO_PI; a += this.angle) {
var sx = cos(a) * this.r2;
var sy = sin(a) * this.r2;
vertex(sx, sy);
sx = cos(a + this.halfAngle) * this.r1;
sy = sin(a + this.halfAngle) * this.r1;
vertex(sx, sy);
}
endShape();
pop();
}
}
//////////////////////////////////////////////////////////////////////
class Planet {
constructor() {
this.x = random(0, width);
this.size = random(10, 50);
this.y = height - this.size;
this.col = color("red");
}
move() {
this.x;
//Moving up at a constant speed
this.y -= 0.75;
// Reset to the bottom
if (this.y < -100) {
this.y = height + 250;
this.size = random(10, 50);
}
if (this.x < 0 || this.x > width) {
this.x = (random(10, 500)) - 25;
}
}
show() {
//draw planet with ring
fill(this.col);
noStroke();
circle(this.x, this.y, this.size);
noFill();
stroke(this.col);
strokeWeight(1);
ellipse(this.x, this.y, this.size * 2, this.size / 2);
}
}
//////////////////////////////////////////////////////////////////////
class Planet2 {
constructor() {
this.x = random(0, width);
this.y = height + 100;
this.size = random(50, 80);
this.col = color("pink");
}
move() {
this.x;
//Moving up at a constant speed
this.y-=1.1;
// Reset to the bottom
if (this.y < -50) {
this.y = height;
this.size = random(50, 80);
}
if (this.x < 0 || this.x > width) {
this.x = (random(10, 500)) - 25;
}
}
show() {
//draw planet with rotated ring
fill(this.col);
noStroke();
circle(this.x, this.y, this.size);
stroke(this.col);
strokeWeight(2);
noFill();
push();
translate(this.x, this.y);
rotate(PI/4);
ellipse(0, 0, this.size * 1.5, this.size / 3);
pop();
}
}
//////////////////////////////////////////////////////////////////////
class Planet3 {
constructor() {
this.x = random(0, width);
this.y = height + 100; //start below window
this.size = random(50, 80);
this.col = color("coral");
}
move() {
this.x = this.x + random(-1, 1);
//Moving up at a constant speed
this.y -= 0.85;
// Reset to the bottom
if (this.y < -100) {
this.y = height;
this.size = random(50, 80);
}
if (this.x < 0 || this.x > width) {
this.x = (random(10, 500)) - 25;
}
}
show() {
//draw planet with rotating ring
fill(this.col);
noStroke();
circle(this.x, this.y, this.size);
noFill();
stroke(this.col);
push();
translate(this.x, this.y);
rotate(radians(frameCount)/2);
strokeWeight(1);
ellipse(0, 0, this.size / 2, this.size * 2);
pop();
}
}
//////////////////////////////////////////////////////////////////////
class SpinPlanet {
constructor() {
this.x = random(0, width);
this.y = height + 100; //start below window
this.size = random(50, 90);
this.col = color("green");
}
move() {
this.x = this.x + random(-1, 1);
//Moving up at a constant speed
this.y -= 0.65;
// Reset to the bottom
if (this.y < -100) {
this.y = height;
this.size = random(50, 90);
}
if (this.x < 0 || this.x > width) {
this.x = (random(10, 500))-40;
}
}
show() {
//draw a spinning planet
fill(this.col);
noStroke();
circle(this.x, this.y, this.size);
noFill();
stroke(this.col);
strokeWeight(2);
push();
translate(this.x, this.y);
rotate(frameCount);
ellipse(random(-1,1), 0, this.size / 2, this.size * 2);
pop();
}
}
//////////////////////////////////////////////////////////////////////
class Line {
constructor() {
this.r = height + 200; // starting pt + 200 so to make sure it starts off canvas
this.x = random(width); // Start with a random x location
this.y = this.r; // Start pt below the window
this.speed = random(2, 5); // Pick a random speed
this.l = random(100, 200); //randomize length of line
}
move() {
// move y upwards by speed
this.y -= this.speed;
}
display() {
//only draw if it's in the canvas
//draw the shooting stars lines
if (this.y > 0) {
strokeWeight(1);
stroke(random(100, 255), random(100, 255), random(100, 255), 200);
line(this.x, this.y, this.x, this.y - this.l);
}
}
}