//Sean Meng
//hmeng@andrew.cmu.edu
//Section C
//Project-11-Generative Landscape
var sushi = [];
function setup() {
createCanvas(480, 480);
frameRate(50);
//set an initial group of sushi
for (var i = 0; i < 3; i++){
var rx = random(width);
sushi[i] = makeSushi(rx);
}
}
function draw() {
background(255, 200, 200);
//the lattern
fill(30);
rect(60, 20, 40, 160);
rect(78, 0, 5, 20)
fill(235, 70, 70);
stroke(235, 70, 70);
strokeWeight(50);
rect(60, 60, 40, 80)
noStroke();
//ribs on lattern
for(i = 0; i < 8; i++){
fill(120, 0, 0);
rect(35, 57 + i * 12, 90, 1.5);
}
//the belt
fill(50);
rect(0, 320, width, 80);
fill(180);
rect(0, 400, width, 10);
//the table
fill(255, 237, 199);
rect(0, 410, width, 190);
//the plate
fill(255);
ellipse(170, 440, 300, 40);
fill(220);
ellipse(180, 435, 200, 20);
fill(250);
ellipse(186, 438, 180, 20);
//thie chopsticks
fill(250);
rect(385, 435, 35, 10);
fill(128, 55, 0);
quad(400, 430, 405, 430, 455, 480, 443, 480);
fill(128, 55, 0);
quad(390, 430, 395, 430, 435, 480, 425, 480);
updateSushi();
removeSushiOutOfView();
addSushi();
}
function updateSushi() {
for (var i = 0; i < sushi.length; i++) {
sushi[i].move();
sushi[i].display();
}
}
function removeSushiOutOfView() {
//remove sushi from array if it's out of sight
var sushiToKeep = [];
for (var i = 0; i < sushi.length; i++) {
if (sushi[i].x + sushi[i].breadth > 0) {
sushiToKeep.push(sushi[i]);
}
}
sushi = sushiToKeep; //remember the surviving sushi
}
function addSushi() {
//with a very small probability, add a new sushi to the end
var newSushiProb = 0.005;
if (random(0,1) < newSushiProb) {
sushi.push(makeSushi(width));
}
}
function sushiDisplay(){
//rice
stroke(this.c3);
strokeWeight(10);
strokeJoin(ROUND);
fill(this.c3);
rect(this.x, 350, this.breadth, this.height);
//salmon on top of rice
var top = 350 + this.height;
var top2 = 350 + this.height - 5;
stroke(255, this.c1, this.c2);
fill(255, this.c1, this.c2);
rect(this.x - 10, top, this.breadth + 20, 20);
noStroke();
fill(255, this.c1 + 50, this.c2 + 80);
triangle(this.x, top2, this.x + 10, top2, this.x + 5, top + 15);
triangle(this.x + 30, top2, this.x + 40, top2, this.x + 35, top + 15);
triangle(this.x + 60, top2, this.x + 70, top2, this.x + 65, top + 15);
//the kelp
fill(0);
rect(this.x + this.breadth / 2, top2, 30, -this.height + 5);
}
function sushiMove(){
this.x += this.speed;
}
function makeSushi(startX){
var mySushi = {x: startX,
speed: -1.0,
breadth: random(80, 120),
height: -random(50, 70),
c1: random(100, 150),
c2: random(50, 90),
c3: random(240, 255),
move: sushiMove,
display: sushiDisplay}
return mySushi;
}
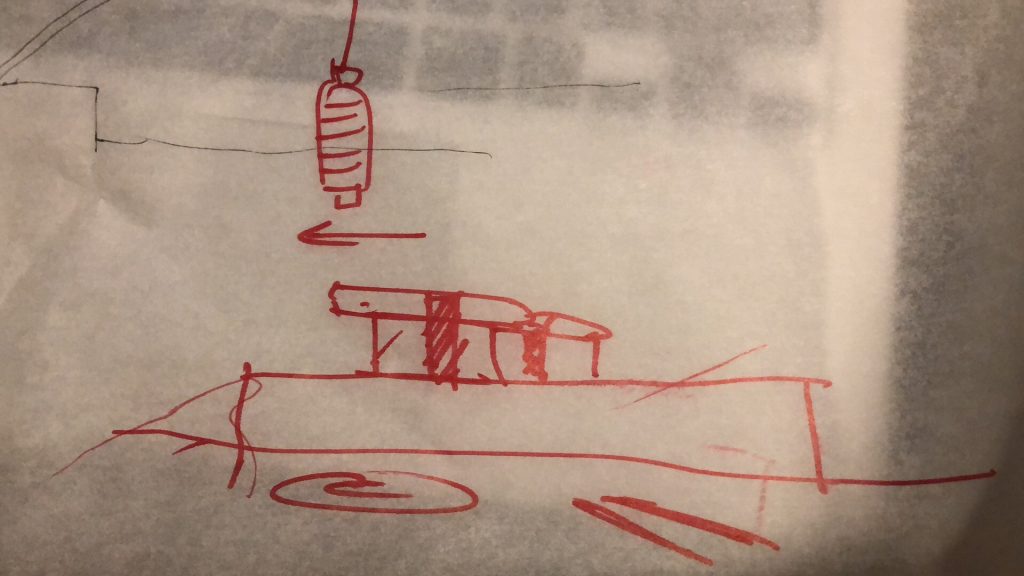
In this project, I intend to create this “rotation sushi” scene. Using generative drawing methods, sushi with different shapes, sizes and toppings come out and move along the belt.