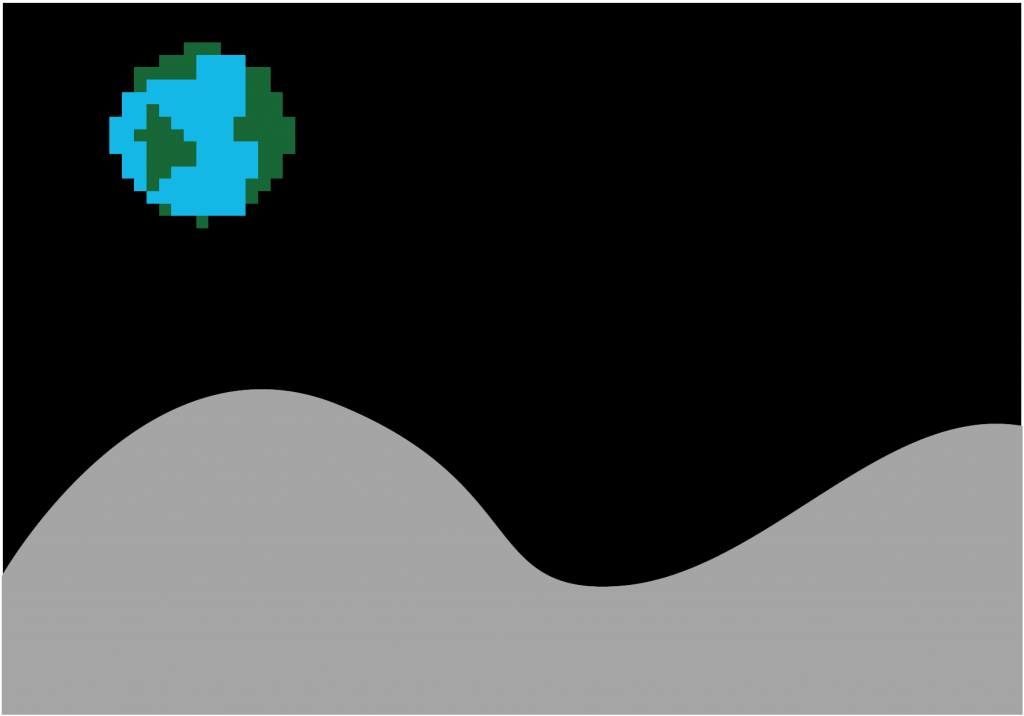
I wanted to be a moon rover, so I sketched an illustration of being on the moon. The ground is grey and you slowly move across the moon’s surface.
sketch
///RAYMOND PAI
///SECTION D
///RPAI@ANDREW.CMU.EDU
///PROJECT 11
var stars = [];
var sX = 0;
var sY = 0;
var moonSpeed = 0.00009;
var moonDetail = 0.005;
function setup(){
createCanvas(480, 300);
//initialize stars
for(var i = 0; i < 30; i++){
sX = random(width);
sY = random(height);
stars[i] = makeS(sX, sY);
}
}
function draw() {
background(0);
//draw earth
noStroke();
fill(90, 90, 255);
ellipse(130, 80, 70, 70);
fill(0, 255, 0);
rect(130, 80, 30, 20);
fill(0, 255, 0);
rect(100, 60, 20, 10);
//moon ground
drawMoon();
//stars
drawS();
}
function drawS(min, max, moise) {
noStroke();
fill(255, 255, 0);
push();
translate(this.x, this.y);
ellipse(10, 10, 5, 5);
pop();
}
function makeS(stX, stY){
var makeStar = {x: stX,
y: stY,
speed: -3,
move: moveS,
draw: drawS}
return makeStar;
}
function moveS(){
this.x += this.speed;
if(this.x <= -5){
this.x += width;
}
}
function displayS(){
for(var i = 0; i < 50; i++){
stars[i].move();
stars[i].draw();
}
}
function drawMoon(min, max, moise) {
fill(150);
beginShape();
//makes ground of the moon surface
for (var x = 0; x < width; x++) {
var t = (x * moonDetail) + (millis() * moonSpeed);
var y = map(noise(t), 0, 1, 0, 400, 400);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}