//Shannon Ha
//Section D
//sha2@andrew.cmu.edu
//Project 11 Generative Landscape
var terrainSpeed = 0.0001;
var terrainDetail = 0.006;
var martians = [];
function setup() {
createCanvas(480, 300);
for (var i = 0; i < 4; i++){ //create initial collection of martians displayed on screen.
var rx = random(width);
martians[i] = makeMartian(rx);
}
for (var i = 0; i < stars; i++) { //randomly generates star size and position.
sX.push(random(0, width)); // x position of star
sY.push(random(0, height / 3)); // y position of star
starSize.push(random(0.1, 1)); // star size
}
frameRate(15);
}
function draw() { // calls all the objects
background(43, 14, 7);
drawStars();
drawMountain();
drawMountainB();
//calls martian objects
updateAndDisplayMartians();
removeMartians();
addMartians();
// draw distant planet A
fill(130, 67, 52);
noStroke();
ellipse(400, 20, 30, 30);
// draw distant planet B
fill(176, 91, 70);
ellipse(350, 15, 10, 10);
}
var stars = 300; //number of star points
var sX = []; //x position array
var sY = []; //y position array
var starSize = [];// star size array
function drawStars() {
noStroke();
for (var i = 0; i < stars; i++) { // draws the stars
stroke(random(100, 255)); // randomize grayscale for stroke to give the twinkle effect
strokeWeight(starSize[i]);
point(sX[i], sY[i], 10, 10);
}
}
function drawMountain(){ //background terrain
push();
fill(79, 16, 0);
noStroke();
beginShape();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);// adjusts flatness of terrain
var y = map(noise(t), 0,1, height/2.5, height * 0.2);
vertex(x, y);
}
vertex(width, height);
endShape();
pop();
}
function drawMountainB(){ //terrain in the front
push();
fill(138, 31, 4);
noStroke();
beginShape();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed * 2);
var y = map(noise(t), 0,1, height , height * 0.1);
vertex(x, y);
}
vertex(width, height);
endShape();
pop();
}
function updateAndDisplayMartians(){
// Update the martians' positions, and display them
for (var i = 0; i < martians.length; i++){
martians[i].move();
martians[i].display();
}
}
function removeMartians(){ // removes all martians that go off the canvas.
var martiansToKeep = [];
for (var i = 0; i < martians.length; i++){
if (martians[i].x + martians[i].breadth > 0) {
martiansToKeep.push(martians[i]);
}
}
martians = martiansToKeep; // remembers the remaining martians on canvas.
}
function addMartians(){ // adds new martians onto canvas
var newMartiansLikelihood = 0.017;
if (random(0,1) < newMartiansLikelihood) {
martians.push(makeMartian(0));
}
}
function martianMove() { // allows the martians to glide across screen
this.x += this.speed;
}
function displayMartian() { //draws the martian.
fill(12, 63, 117);
noStroke();
push();
translate(this.x, height - 60);
// body
ellipse(20, 30, this.breadth, this.height);
// white part of eye
fill(255);
ellipse(20, 20, this.breadth / 2, this.breadth / 2);
//blue part of eye
fill(105, 160, 219);
ellipse(20, 20, 10, 10);
//antennas
stroke(105, 160, 219);
strokeWeight(4);
line(10, 10, 5, 5);
line(30, 10, 35, 5);
//ends of antenna
fill(random(255), random(100), random(200));
noStroke();
ellipse(5, 5, 10, 10);
ellipse(35, 5, 10, 10);
pop();
}
function makeMartian(birthLocationX){ // martian characteristics
var alien = {x: birthLocationX,
breadth: 30,
height: 50,
speed: random(3, 7),
move: martianMove,
display: displayMartian};
return alien;
}
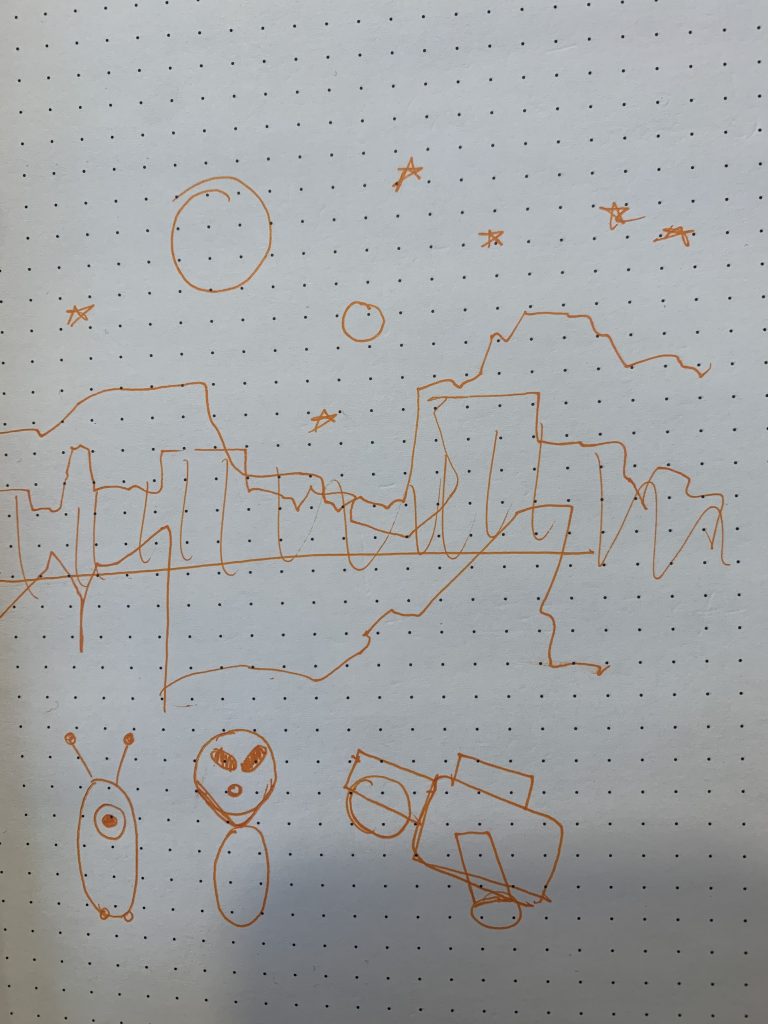
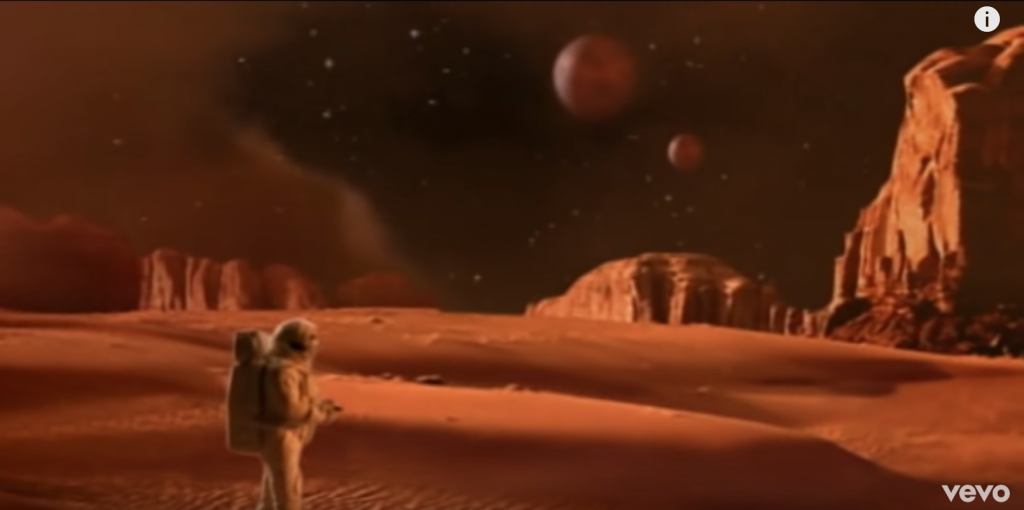
For this project, I wanted to create a fictional landscape of Mars, so I took a bit of inspiration from the iconic Britney Spears’ Oops I did it again Music Video (I wanted to add an astronaut but I didn’t know how to remove the background of a picture I found online) and added my own twist by putting in blue aliens that greet you with their antennas as you pass glance over the Martian landscape. I had a lot of fun making this project as it helped me understand the use of objects and characteristics better!