// Nadia Susanto
// Section B
// nsusanto@andrew.cmu.edu
// Project-07-Curves
var angle = 0;
function setup() {
createCanvas(480, 480);
background(220);
angleMode(radians);
}
function draw() {
//making the fill to be a combo of random colors
var r = random(0, 100);
var g = random(100, 150);
var b = random(200, 275);
frameRate(5);
push();
fill(r, g, b);
translate(width/2, height/2); //drawn to be in the middle
//rotating the asteroid
angle += 1;
rotate(angle);
drawCurve(); //calling function
pop();
}
function drawCurve() {
var b = mouseY/2;
var t = map(mouseY, 0, 200, 40, 120); //constraint
//creating the astroid
beginShape();
for (var i = 0; i < 100; i++) {
t = map(i, 0, 100, 0, TWO_PI);
//reference from http://mathworld.wolfram.com/Astroid.html
var x = 3 * b * cos(t) + b * cos(3 * t);
var y = 3 * b * sin(t) - b * sin(3 * t);
vertex(x, y);
}
endShape();
}
It was hard figuring out which curve to use as there were so many that were pretty, but I ended up choosing to go with the asteroid curve. I figured that a star-shaped curve could be beautiful especially when rotating. With the rotation it looks like a christmas tree star or it even looks flowerly-like. I also played around with the random colors and constrained it to be only blue/purple shades. I then used mouseX and mouse Y in order to determine the size of the asteroid. Overall, I am pretty happy with the result and it looks beautiful.
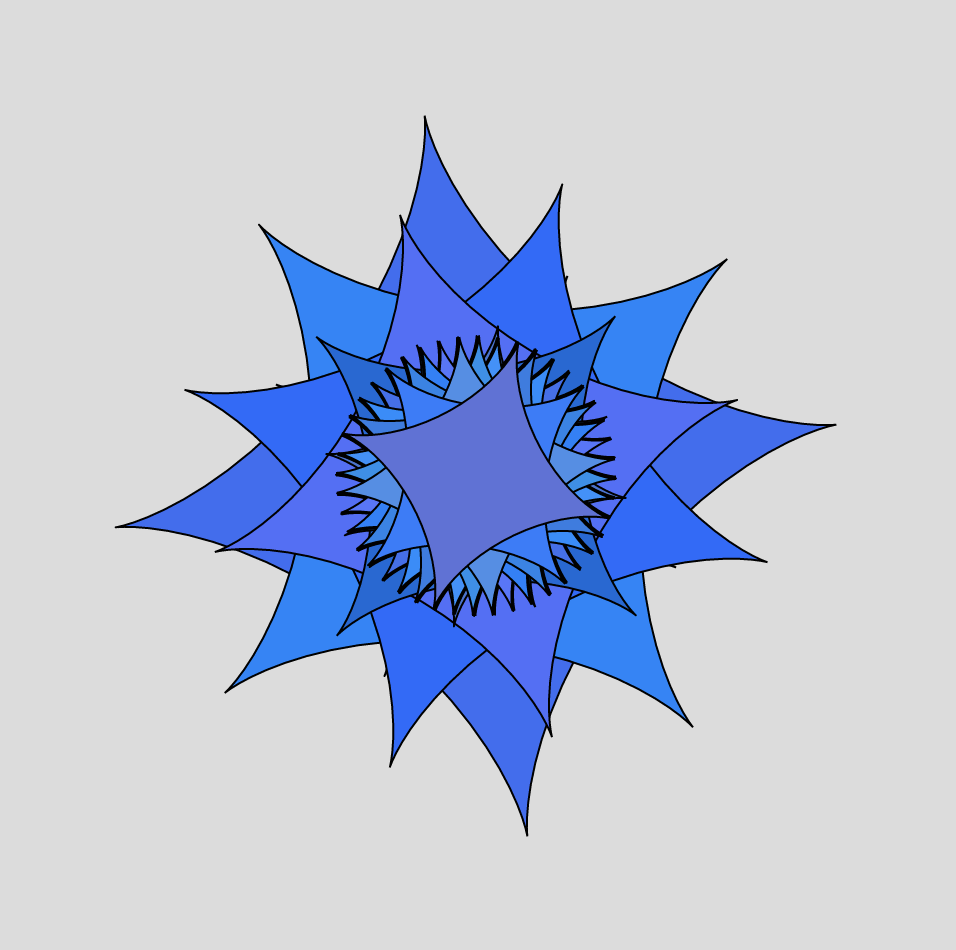
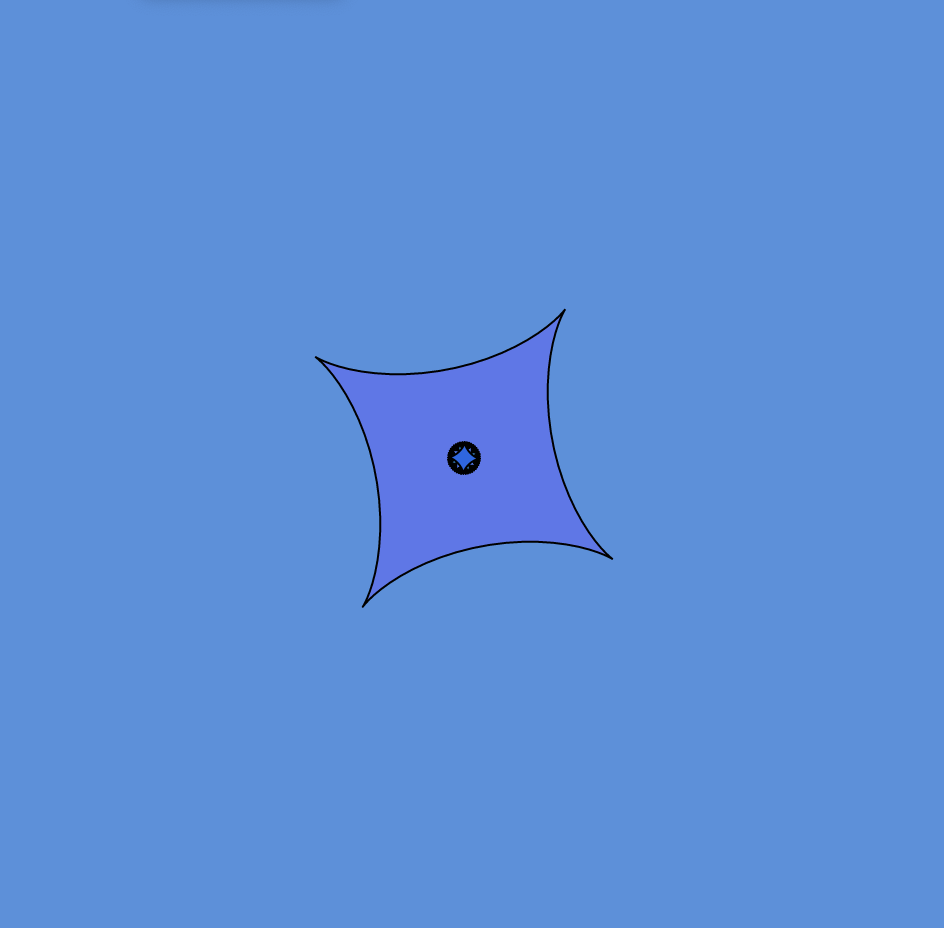