function setup() {
createCanvas(480, 480);
angleMode(DEGREES);
}
function draw() {
background(241, 225, 174);
//background elements art
stroke(0);
noFill();
ellipse(300, 360, 90); //minute ellipse outline
triangle(350, 30, 430, 90, 410, 105); //top r corner triangle
fill(41, 72, 108, 220); //blue quad
quad(170, 100, 190, 270, 290, 320, 60, 450);
fill(41, 72, 108, 180); //blue circle
ellipse(300, 160, 40);
fill(120, 79, 58, 220); //brown quad
quad(50, 160, 130, 370, 340, 465, 180, 350);
fill(226, 170, 56, 150); //yellow circles
ellipse(110, 300, 130);
ellipse(180, 30, 30);
push();
noStroke();
fill(192, 50, 47, 220); //red triangle and ellipse
triangle(80, 35, 135, 90, 20, 130);
ellipse(230, 450, 25);
fill(120, 79, 58, 200); //brown triangle
triangle(270, 240, 430, 380, 240, 350);
fill(0); //black triangle
triangle(380, 350, 440, 400, 370, 390);
pop();
//hours red arc
push();
translate(300, 200);
rotate(-135);
hr();
pop();
//wavy lines
push();
noFill();
strokeWeight(2);
strokeCap(SQUARE);
translate(370, 200);
rotate(30);
bezier(40, -40, -40, -40, 40, 40, -40, 45);
translate(10, 10);
bezier(35, -35, -40, -40, 35, 35, -20, 35);
pop();
//minutes yellow arc
push();
translate(300, 360);
rotate(-90);
scale(.5);
mins();
pop();
//seconds black line
push();
translate(240, 240);
rotate(-135);
sec();
pop();
}
//hours, minutes, seconds functions
function hr() {
var h = hour();
noFill();
strokeCap(SQUARE);
strokeWeight(10);
stroke(192, 50, 47);
var hourHand = map(h % 24, 0, 24, 0, 360);
arc(0, 0, 200, 200, 0, hourHand);
}
function mins() {
push();
var m = minute();
noFill();
strokeCap(SQUARE);
strokeWeight(80);
stroke(226, 170, 56, 200);
var minHand = map(m, 0, 60, 0, 360);
arc(0, 0, 100, 100, 0, minHand);
pop();
push();
fill(0);
ellipse(0, 0, 50);
pop();
}
function sec() {
var s = second();
strokeCap(SQUARE);
strokeWeight(10);
stroke(0);
var secHand = map(s, 0, 60, 0, 360);
rotate(secHand);
line(0, 0, 150, 150);
}
For my project, I was inspired by Bauhaus art and design and decided to make an abstract clock based on abstract artwork. When I started the project, I knew I wanted to use a combination of basic graphic elements to represent time instead of making a representational scene. After looking through some Bauhaus work, I found Kandinsky’s “Arch and Point” (1923). I think that the composition and interaction of shapes is really pleasing in this painting.
I did some quick sketches to think about what elements could represent elements of time. I simplified the composition to capture the most prominent elements of the piece and used the red arc to represent hours, the yellow arc to represent minutes, and the black line to represent seconds for a 24-hour clock.
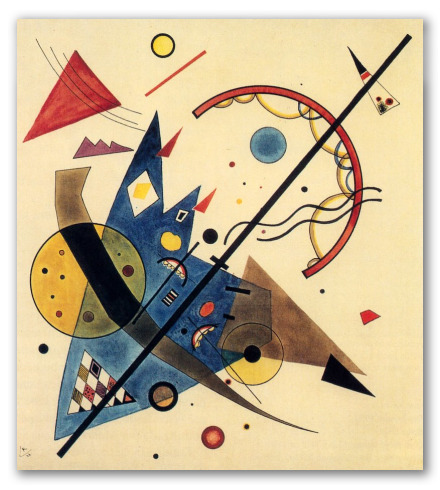
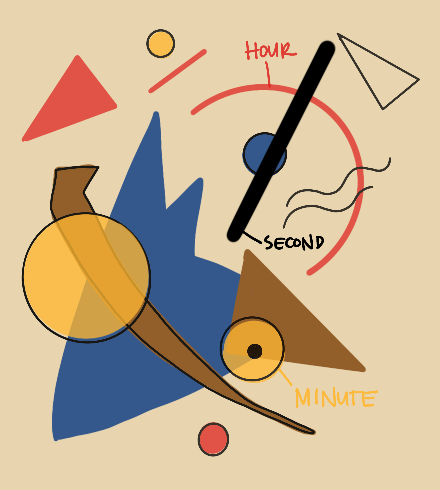