//Dreami Chambers; Section C; dreamic@andrew.cmu.edu; Assignment-05-Project
var x = 0
var y = 0
function setup() {
createCanvas(400, 600);
}
function draw() {
background(255, 205, 225)
//calls the functions with random spacing
for (var x = -10; x <= width-100; x += 150) {
for (var y = 5;y <= height; y+= 170) {
push()
translate(x, y)
rotate(radians(random(0, 20)))
cherries()
pop()
}
}
for (var x = 70; x <= width-100; x += random(150, 210)) {
for (var y = 0;y <= height; y+= random(170, 200)) {
push()
translate(x, y)
rotate(radians(random(0, 20)))
flowers()
pop()
}
}
for (var x = 20; x <= width; x += random(100, 150)) {
for (var y = 20;y <= height; y+= random(100, 170)) {
push()
rotate(radians(random(0, 10)))
translate(x, y)
dots()
pop()
}
}
noLoop()
}
//cherries drawing
function cherries() {
noFill()
beginShape()
strokeWeight(2)
stroke(95, 175, 90)
curveVertex(80, 20)
curveVertex(60, 20)
curveVertex(50, 40)
curveVertex(50, 40)
endShape()
beginShape()
strokeWeight(2)
curveVertex(60, 20)
curveVertex(60, 20)
curveVertex(75, 31)
curveVertex(80, 31)
endShape()
strokeWeight(1)
fill(255, 85, 150)
strokeWeight(0)
circle(50, 50, 20)
circle(80, 40, 20)
fill(95, 165, 90)
push()
translate(70, -30)
rotate(radians(90))
ellipse(50, 20, 10, 20)
pop()
push()
rotate(radians(-13))
ellipse(64, 32, 20, 10)
pop()
}
//flowers drawing
function flowers(){
fill(255)
push()
noStroke()
translate(50, 100)
ellipse(0, 0, 20, 30)
translate(15, 10)
rotate(radians(80))
ellipse(0, 2, 20, 30)
translate(15, 10)
rotate(radians(65))
ellipse(0, 0, 20, 30)
translate(15, 10)
rotate(radians(70))
ellipse(0, 0, 20, 30)
translate(15, 10)
rotate(radians(75))
ellipse(0, 2, 20, 30)
translate(15, 10)
pop()
fill(255, 140, 180)
strokeWeight(0)
ellipse(49, 115, 10, 10)
}
//random circles
function dots(){
strokeWeight(0)
var r = random(0, 15)
fill(255)
circle(0, 0, r)
}
// For this assignment, I wanted to make a design that looked similar to a phone wallpaper.
// I wanted it to look like something I would actually used, so I added colors and shapes that I like a lot.
// I also didn't want the shapes to have the same spacing between each other, as it makes the design look too symmetrical. So I used random to create some variation.
Category: Project-05-Wallpaper
Project-05-Wallpaper
//Isabel Xu
//15-104 Section A
const Y_AXIS = 1;
function setup() {
createCanvas(480,480);
// Two color in the end of the neon hue
c1 = color(223,255,0);
c2 = color(116, 0, 225);
noLoop();
}
function draw() {
// background
setGradient(0, 0, 480, 480, c1, c2, Y_AXIS);
//ellipseShape
drawPattern();
}
function setGradient(x, y, w, h, c1, c2, axis) {
noFill();
for (let i = y; i <= y + h; i++) {
let inter = map(i, y, y + h, 0, 1);
let c = lerpColor(c1, c2, inter);
stroke(c);
line(x, i, x + w, i);
}
}
function drawPattern(){
cirW=150;
for (var x = 0; x <= width;x +=150){
cirH=150;
for (var y = 0;y <= height;y+=150){
noStroke();
fill(250);
ellipseMode(CORNER)
ellipse(x,y,cirW,cirH);
cirH-=40;
}
cirW-=40;
}
}
A study on geometric symbols evolved from circles.
Project-05-WallPaper
Project 05 – Wallpaper
I thought it would be an interesting exercise to simplify the intricate orchid flower with code. It was a fun challenge determining the most essential shapes out of the organic form. After getting the basic shapes down on paper, I made a sketch in Illustrator, then translated it into p5.js coordinates to create a “tile.” I then used loops to create a wallpaper out of the original tile design.
orchid et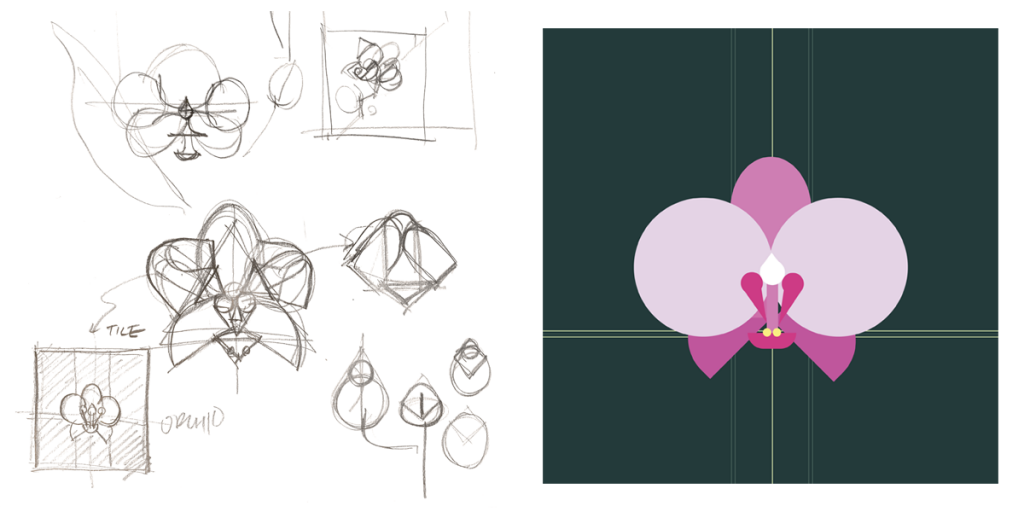
function setup() {
createCanvas(600, 600);
}
function draw() {
background(200);
scale(.2);//scale down coordinates based on 600x600 canvas
for (var x = 0; x <= 5*width; x += 600) {
for (var y = 0; y <= 5*height; y += 600) {
push();
translate(x, y);
tile();
pop();
}
noLoop(0);
//}
}
}
function tile() { //use repeating tile to simplify loop function
noStroke();
fill(35, 58, 58);//bg dark green color
rect(0, 0, 600, 600);
//decorative lines
stroke(227, 239, 177); //yellow line
line(0, 399, 600, 399);
line(0, 407, 600, 407);
line(300, 0, 300, 600);
stroke(115, 140, 127); //green line
strokeWeight(0.5);
line(0, 403, 600, 403);
line(250, 0, 250, 600);
line(255, 0, 255, 600);
line(350, 0, 350, 600);
line(355, 0, 355, 600);
push();
orchid();
pop();
}
function orchid() {
noStroke();
push();
fill(206, 126, 179); //midtone magenta
ellipse(300, 240, 107, 142); //back petal
fill(191, 86, 156); //darker magenta
translate(-165, 310);
rotate(radians(-45));
rect(180, 312, 115, 76, 60, 0, 0, 0);
pop();
push();
fill(191, 86, 156); //midtone magenta
translate(340,-115);
rotate(radians(45));
rect(305, 312, 115, 76, 0, 60, 0, 0);
pop();
push();
fill(228, 211, 229); //light magenta
ellipse(212, 315, 183);//large petal1
ellipse(388, 315, 183);//large petal2
pop();
push();
fill(206, 126, 179); //midtone magenta
rect(292, 329, 16, 80);//center rectangle
pop();
push(); //top "sandle"
fill(255); //white
ellipse(300, 320, 33);
triangle (300, 295, 317, 315, 283, 315);
fill(205, 59, 113); //true magenta
ellipse(273, 335, 24);
triangle(264, 343, 278, 343, 295, 387);
ellipse(327, 335, 24);
triangle(336, 343, 322, 343, 305, 387);
rect(268, 401, 64, 21, 0, 0, 21, 21);
fill(249, 239, 130);//yellow
ellipse(293, 400, 11);
ellipse(307, 400, 11);
pop();
}
PROJECT 05 – WALLPAPER
function setup() {
createCanvas(600, 500);
}
function draw() {
background(214, 204, 135);
//stars
for(var x = 25; x <= 600; x += 150) {
for(var y = 0; y <= 500; y += 160) {
push();
translate(x, y);
poopiB(x, y);
pop();
}
}
//pizza
for(var x = -50; x <=600; x += 150) {
for(var y = -50; y <= 500; y += 160) {
push();
translate(x, y);
pizza(x, y);
pop();
}
}
}
function poopiB(x, y) {
//head
stroke(0);
fill(255, 254, 143)
rect(35, 70, 25, 70, 17)
//hat
fill(0);
rect(32, 65, 31, 8)
rect(38, 35, 20, 30)
//hat detail
fill(35, 105, 94)
rect(38, 60, 20, 5)
//eyes
fill(255);
circle(35, 95, 18, 18);
circle(60, 95, 18, 18);
//pupils
strokeWeight(3);
fill(0);
point(35, 95);
point(60, 95);
//nose
strokeWeight(1);
noFill();
beginShape();
curveVertex(44.5, 102);
curveVertex(44.5, 102);
curveVertex(45.5, 112);
curveVertex(47.5, 115);
curveVertex(49.5, 112);
curveVertex(50.5, 102);
curveVertex(50.5, 102);
endShape();
//mouth
fill(105, 35, 37);
beginShape();
curveVertex(39, 118);
curveVertex(39, 118);
curveVertex(56, 118);
curveVertex(56, 121);
curveVertex(54, 122.5);
curveVertex(47.5, 125);
curveVertex(40.5, 122.5);
curveVertex(39.5, 121);
curveVertex(39, 119);
curveVertex(39, 119);
endShape();
fill(202, 66, 68);
beginShape();
curveVertex(44.5, 124);
curveVertex(44.5, 124);
curveVertex(45, 122);
curveVertex(46, 121);
curveVertex(49, 120.5);
curveVertex(52, 123.5);
curveVertex(51, 124.5);
endShape();
}
function pizza(x, y) {
//crust
stroke(0);
fill(208, 166, 116);
triangle(28, 75, 60, 55, 85, 85);
quad(24, 69.5, 85, 80, 85, 85, 24, 75);
quad(24, 69.5, 33, 70.5, 60, 50, 58, 47);
//cheese
fill(255, 248, 194);
triangle(28, 70, 60, 50, 85, 80);
//pepperoni
fill(223, 78, 73);
circle(45, 67, 8, 8);
circle(68, 70, 8, 8);
circle(59, 60, 8, 8);
}
I love Rick and Morty, and I wanted to create a wallpaper of the additional characters that show up in certain episodes. One of my favorite characters is Mr. Poopybutthole. My wallpaper is inspired by a scene in the show where he is also surrounded by pizza.
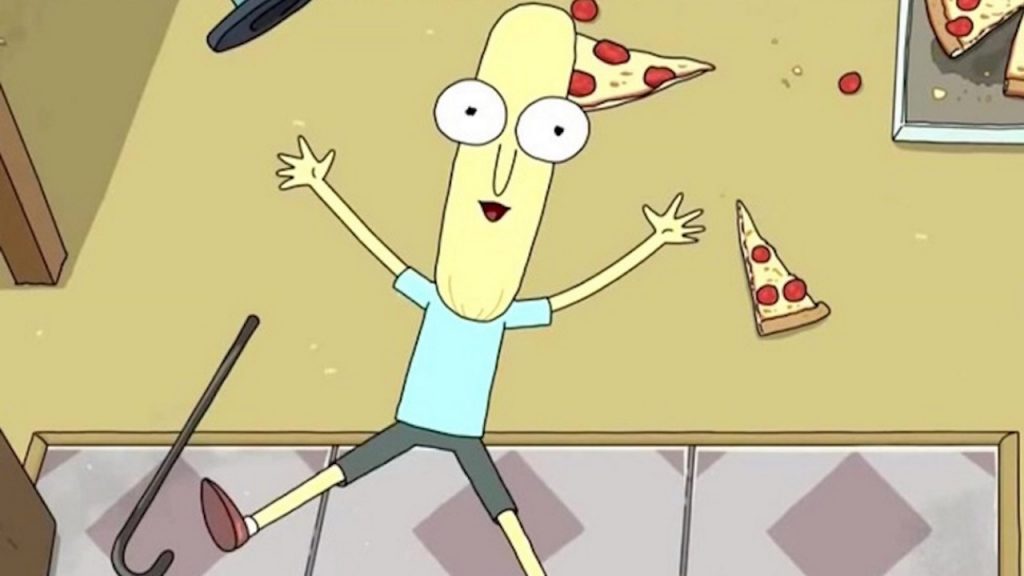
Project – 05: Wallpaper
For this project, I wanted to create a simple wallpaper of clouds that had a cute smile with rosy red cheeks. In order to do so, I first sketched out one friendly cloud on the illustrator and began coding from there.
//Rachel Kim
//15-104(Section C)
//rachelki@andrew.cmu.edu
//Project 05
function setup() {
createCanvas(450, 500);
noLoop();
}
function draw() {
background(213, 232, 241);
for (var x = 90; x <= 700; x += 170) {
for (var y = 90; y <= 700; y += 80) {
push();
translate(x, y);
cloud();
pop();
}
}
}
//cloud background
function cloud() {
//white clouds
noStroke();
fill(255);
ellipse(-10, -122, 51, 35);
ellipse(-37, -107, 51, 35);
ellipse(-49, -134, 51, 35);
ellipse(-68, -110, 51, 35);
ellipse(-89, -128, 51, 35);
//cloud eyes
noStroke();
fill(0);
ellipse(-20, -200, 4, 4);
ellipse(-50, -200, 4, 4);
//cloud smile mouth
stroke(0);
strokeWeight(1);
noFill();
arc(-203, -193, 15, 10, TWO_PI, PI, OPEN);
//cute cheeks
noStroke();
fill(255, 0, 0, 100);
ellipse(-216, -115, 9, 3);
ellipse(-190, -115, 9, 3);
}
Project 05: Wallpaper
My first idea when trying to create a wallpaper was using Penrose tiling, but I couldn’t find a good algorithm without directly copying code, which wouldn’t make for a very good learning exercise. My second idea, using a theme of symmetry, came in the form of recursion. I decided to loosely base a recursive wallpaper off of a Koch snowflake. I think that the resolution is a little too low for it to be properly viewed, but it is still interesting. I’d like to imagine this pattern looped across my wall, each recursive segment being repeated.
function setup() {
createCanvas(600, 600);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(0);
// just the rainbow
let colors = ['#FF0000', '#FF7F00', '#FFFF00', '#00FF00',
'#0000FF', '#4B0082', '#9400D3'];
// move center for ease of programming
translate(width / 2, height / 2);
recursiveSnowflake(360, colors);
noLoop();
}
// use default parameters for recursive depth
function recursiveSnowflake(initSize, colors, depth=0) {
if (depth === 7) { // base case
// do nothing
} else { // recursive case
fill(colors[depth]);
drawTriCenter([0, 0], initSize, 0);
drawTriCenter([0, 0], initSize, 180);
var dAngle = 60;
for (var i = 0; i < 6; i++) {
push();
rotate(radians((dAngle * i)));
translate(0, Math.sqrt(3) * initSize / 2);
recursiveSnowflake(initSize / 3, colors, depth+1);
pop();
}
}
}
// draws an equilateral triangle, point facing up
function drawTriCenter(c, sideLength, rot) {
// math to get tri points from center
var distance = sideLength / Math.sqrt(3);
let p1 = [c[0] - distance * cos(radians(90 + rot)),
c[1] - distance * sin(radians(90 + rot))];
let p2 = [c[0] - distance * cos(radians(210 + rot)),
c[1] - distance * sin(radians(210 + rot))];
let p3 = [c[0] - distance * cos(radians(330 + rot)),
c[1] - distance * sin(radians(330 + rot))];
strokeWeight(0);
triangle(p1[0], p1[1], p2[0], p2[1], p3[0], p3[1]);
}
Project Wallpaper
var x = 0
var y = 0
var side = 50
function setup() {
createCanvas(400, 400);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function coolShape(x, y) {
strokeWeight(3);
stroke(95, 57, 1);
fill(250, 195, 14);
//left quad
quad(x, y, (x + 2*(side/3)), (y + (-side/2)), (x + 2*(side/3)), (y + (side/2)), x, (y + side))
//right quad
quad((x + 2*(side/3)), (y + (-side/2)), (x + 4*(side/3)), y, (x + 4*(side/3)), (y + side), (x + 2*(side/3)), (y + (side/2)));
//bottom quad
quad(x, (y + side), (x + 2*(side/3)), (y + (side/2)), (x + 4*(side/3)), (y + side), (x + 2*(side/3)), (y + 2*side));
}
function draw() {
background(250, 195, 14);
for (var r = 0; r <= 400; r += (dist(x, (y + side), (x + 4*(side/3)), (y + side)))) {
for (var i = 0; i <= 500; i += 4*(side/3)) {
coolShape(r, i);
}
}
}
Project 5: Wallpaper
I was feeling a little dead this week so why not skulls!
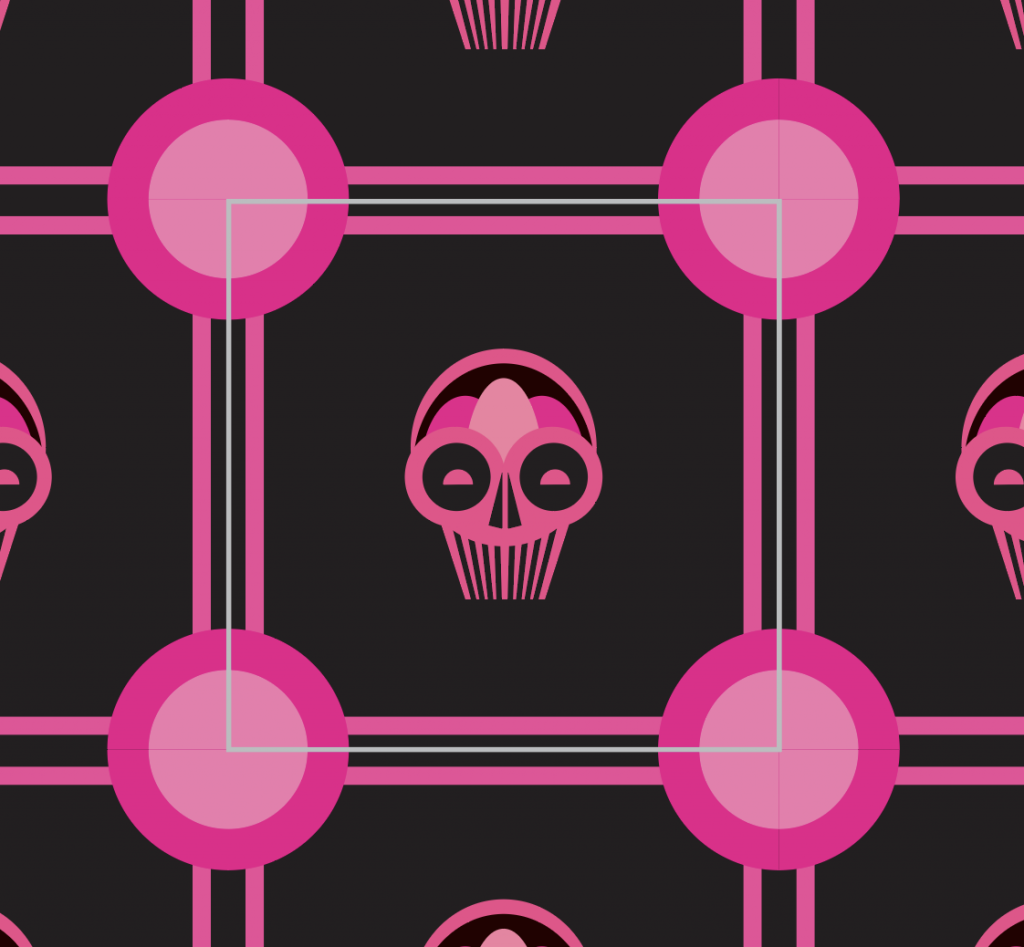
//FrancisPark
//Section C
function setup() {
createCanvas(600, 600);
noStroke();
}
function draw() {
background(0);
noLoop();
for (var y = 1; y < height + 120; y += 119) {
for (var x =1; x < width + 120; x += 119) {
skull(x,y);
}
}
}
function skull(x,y) {
push();
translate(x,y);
noStroke();
//border shapes
fill("#ED4999");
rect(0,24,2,73);
rect(23,0,73,2);
rect(118,21,2,73);
rect(23,117,73,2);
fill("#EB008B");
arc(0,0,52.5,52.5,0,HALF_PI);
arc(119.8,0,52.5,52.5,HALF_PI,PI);
arc(119.8,120,52.5,52.5,PI,PI* 3/2);
arc(0,120,52.5,52.5,PI*3/2,0);
fill("#FF96CF");
arc(0,0,34.5,34.5,0,HALF_PI);
arc(119.8,0,34.5,34.5,HALF_PI,PI);
arc(119.8,120,34.5,34.5,PI,PI* 3/2);
arc(0,120,34.5,34.5,PI*3/2,0);
//skull shape
fill("#EE498A");
quad(43,60,77.5,60,69,87,51.5,87); //jaw
fill("#231F20"); //teeth
quad(48.5,70.5,49.7,70.5,54,87,52.8,87);
quad(51.5,70.6,52.8,70.5,55.7,87,54.5,87);
quad(54.5,70.5,56,70.5,57.5,87,56.5,87);
quad(57.7,70.5,59,70.5,59.5,87,58.5,87);
quad(61.3,70.5,62.5,70.5,62,87,60.8,87);
quad(64.3,70.5,65.5,70.5,63.7,87,62.6,87);
quad(67.6,70.5,68.7,70.5,65.5,87,64.5,87);
quad(70.5,70.5,71.7,70.5,67.5,87,66.4,87);
fill("#EE498A"); //various parts of eyes and head
ellipse(60,54,40.5,43);
fill("#231F20");
arc(60,58,39,44,PI,0);
fill("#EB068C");
arc(51.5,58,19,31,PI,0);
arc(68,58,19,31,PI,0);
fill("#F1A9C1");
arc(60,60,17,42,PI,0);
fill("#EE498A");
circle(49.3,60.5,21.8);
circle(70.5,60.5,21.8);
fill("#231F20");
circle(49.3,60.5,14.7);
circle(70.8,60.5,14.7);
fill("#EE498A");
arc(50,62,6.5,6.5,PI,0);
arc(71,62, 6.5,6.5,PI,0);
fill("#231F20");
triangle(60.8,59.6,63.7,71,60.8,71.8);
triangle(59.5,59.6,59.5,71.8,56.6,71);
pop();
}
Project 5: Wallpaper
My process for creating this wallpaper assignment was to first create the repeating sun pattern and then the repeating snake pattern. To accomplish this, I created a separate sun function and snake function. After I created the two functions, I used a series of for loops to create the patterns. The symbols of the snake and the sun were inspired by the Temple of the Feathered Serpent in Teotihuacán.
function setup() {
createCanvas(500, 500);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(97,104,181);
//sun pattern
push();
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,50);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,100);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,150);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,200);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,250);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,300);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,350);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,400);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
push();
translate(0,450);
for(sunRow = 0; sunRow <= 9; sunRow += 1){
sun();
translate(50,0);
}
pop();
translate(-20,0);
for(drawSnake = 0; drawSnake <= 5; drawSnake += 1){
snake();
translate(0,105);
}
noLoop();
}
function sun() {
noStroke();
fill(97,104,181);
square(0,0,50);
fill(249,235,219);
//sun 1
circle(25,25,25);
//sun rays 1
stroke(249,235,219);
line(25,25,25,5);
line(25,25,25,45);
line(5,25,25,25);
line(45,25,25,25);
line(25,25,10,10);
line(25,25,40,40);
line(25,25,10,40);
line(25,25,40,10);
}
function snake(){
noStroke();
fill(10,random(100,255),0);
//body
beginShape();
vertex(125,25);
curveVertex(150,35);
curveVertex(200,25);
curveVertex(250,35);
curveVertex(300,25);
curveVertex(320,30);
curveVertex(350,40);
curveVertex(375,30);
curveVertex(400,25);
vertex(475,32.5);
curveVertex(400,34);
curveVertex(350,50);
curveVertex(300,35);
curveVertex(250,50);
curveVertex(200,35);
curveVertex(150,50);
curveVertex(125,30);
endShape(CLOSE);
push();
//tongue
stroke(255,10,0);
line(90,29,80,29);
//head
noStroke();
ellipse(110,29,40,15);
//eye
fill(255);
ellipse(110,27,5,3);
strokeWeight(3);
stroke(0);
//pupil
point(110,27);
}