var angle = 0 ;
var x=50 ;
var y=50 ;
function setup() {
createCanvas(600, 450);
}
function draw() {
background(mouseX/5,mouseY/5,(mouseY+mouseX)/10);
stroke(0,mouseX/5,width-mouseY/5);
strokeWeight(mouseX/100);
for (let w=0; w<=width;w+=30){ //setting up circle grid
for(let h=0;h<=height; h+=30) {
r = 75 * exp(((-1)*dist(mouseX, mouseY,w,h))/100); //varying size with mouse position
fill(width-mouseX,height-mouseY,mouseY/5) //varying color of balls with mouse positoin
ellipse(w,h,r) ; //circle
}
}
push();
translate(mouseY,mouseX);
rotate(radians(mouseX));
fill(mouseY);
strokeWeight(mouseY/100);
ellipse(x,y+100,dist(mouseX,mouseY,x,y+100)/10,dist(mouseX,mouseY,x,y+100)/10); //circle floating on top
translate(mouseX,mouseY);
rotate(radians(mouseY));
ellipse (x,y, dist(mouseX,mouseY,x,y)/10, dist(mouseX,mouseY,x,y)/10) ;
translate(mouseX*0.4,mouseY*0.8);
rotate(radians(mouseY*0.5));
ellipse(x+80,y,dist(mouseX,mouseY,x+80,y)/10,dist(mouseX,mouseY,x+80,y)/10);
pop();
}
Category: Project-03-Dynamic-Drawing
Project – Dynamic Drawing
My process was starting with a sketch and then systematically testing and working out the individual controls for each circle.
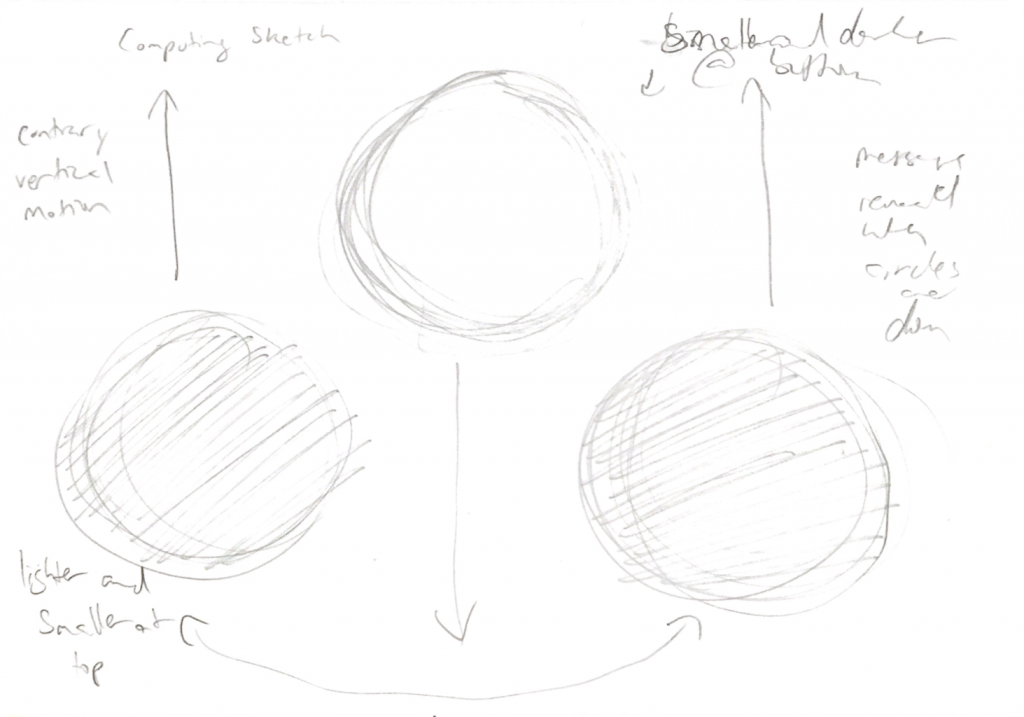
//4 parameters: shade of circles/text, size of circles, position of circles, which message appears
function setup() {
createCanvas(600, 450);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(255,0,0);
var grayscale = max(min(mouseX,255),0); //as mouse moves right circles become lighter
var size1 = max(min(mouseY,227),70); //increases size as mouse moves down. Min diameter is 70, max diameter is 227
var size2 = 297 - size1; //opposite of size 1, makes the middle circle have the opposite diameter of the other two
var yShift = constrain(mouseY,0,height/3); //as mouse moves down circles move down
var yShift1 = -yShift; //two circles move opposite direction of center circle
//mouse makes different messages appear
if(yShift == height/3){ //when the middle circle is at its lowest position the message "Et hoco modo nihil" appears
textSize(45);
fill(abs(255-grayscale));
textAlign(LEFT);
text('Et hoc',50,400);
textAlign(CENTER);
text('modo',width/2,50);
textAlign(RIGHT);
text('nihil',550,400);
}
if(yShift == 0){ //when the middle circle is at its highest position the message "In Quarta Parametri" appears
textSize(45);
fill(abs(255-grayscale));
textAlign(LEFT);
text('In',50,50);
textAlign(CENTER);
text('Quarta', width/2, 400);
textAlign(RIGHT);
text('Parametri',550,50);
}
if(height/3 + yShift + 30 == height/2){ //When all three circles are aligned the message "Quid est amor? Infantem et non cocuerunt mihi" appears
textSize(45);
textAlign(LEFT);
fill(grayscale);
text('Quid est amor?',50,50);
textSize(30);
textAlign(RIGHT);
fill(abs(255-grayscale));
text('Infantem et non nocuerunt mihi.',550,400);
}
noStroke();
fill(grayscale);
circle(width/5,(height*2)/3 + yShift1 + 10,size1);
circle((width*4)/5,(height*2)/3 + yShift1 + 10,size1);
fill(abs(255-grayscale)); //this circle experiences the opposite color of the other two
circle(width/2,height/3 + yShift + 30,size2);
}
PROJECT 03 – DYNAMIC DRAWING
function setup() {
createCanvas(600, 450);
}
function draw() {
if (mouseX < width / 2) {
background(133, 199, 222);
} else if (mouseX >= width / 2) {
background(69, 123, 157);
}
noStroke();
fill(230, 250, 252);
circle (300, 225, -max(min(mouseX, 800), 0));
//balloon string
strokeWeight(3);
fill(101, 101, 94);
beginShape();
curveVertex(20 + (mouseX - 50), 200);
curveVertex(20 + (mouseX - 50), 200);
curveVertex(15 + (mouseX - 50), 250);
curveVertex(5 + (mouseX - 50), 280);
curveVertex(5 + (mouseX - 50), 280);
endShape();
//balloon
fill(249, 112, 104);
ellipse(20 + (mouseX - 50), 160, 65, 80);
triangle(5 + (mouseX - 50), 210, 20 + (mouseX - 50), 200, 35 + (mouseX - 50), 210);
//bike
/*wheel*/
strokeWeight(1);
fill(255);
ellipse(15 + (mouseX - 200), 400, 50, 50);
ellipse(-55 + (mouseX - 200), 400, 50, 50);
/*skeleton*/
stroke(0);
line(-55 + (mouseX - 200), 400, -43 + (mouseX - 200), 370);
line(-43 + (mouseX - 200), 370, 15 + (mouseX - 200), 350);
line(15 + (mouseX - 200), 330, 20 + (mouseX - 200), 400);
line(15 + (mouseX - 200), 350, -20 + (mouseX - 200), 400);
line(-20 + (mouseX - 200), 400, -55 + (mouseX - 200), 400);
line(-43 + (mouseX - 200), 370, -47 + (mouseX - 200), 350);
line(-43 + (mouseX - 200), 370, -15 + (mouseX - 200), 407);
/*seat&handle*/
fill(0);
rect(-55 + (mouseX - 200), 350, 20, 10, 20);
rect(-20 + (mouseX - 200), 407, 7, 5, 5);
rect(10 + (mouseX - 200), 330, 10, 7, 2);
//path
noStroke();
fill(187, 181, 189);
rect(0, 425, 600, 200);
//bob on bike
/*body*/
fill(153, 178, 221);
noStroke();
beginShape();
curveVertex(-20 + (mouseX - 200), 275);
curveVertex(-20 + (mouseX - 200), 275);
curveVertex(-24 + (mouseX - 200), 280);
curveVertex(-26 + (mouseX - 200), 280);
curveVertex(-28 + (mouseX - 200), 300);
curveVertex(-20 + (mouseX - 200), 320);
curveVertex(-20 + (mouseX - 200), 340);
curveVertex(-25 + (mouseX - 200), 350);
curveVertex(-28 + (mouseX - 200), 350);
curveVertex(-30 + (mouseX - 200), 350);
curveVertex(-31 + (mouseX - 200), 350);
curveVertex(-32 + (mouseX - 200), 350);
curveVertex(-32 + (mouseX - 200), 350);
curveVertex(-33 + (mouseX - 200), 350);
curveVertex(-34 + (mouseX - 200), 350);
curveVertex(-35 + (mouseX - 200), 350);
curveVertex(-40 + (mouseX - 200), 350);
curveVertex(-43 + (mouseX - 200), 350);
curveVertex(-45 + (mouseX - 200), 350);
curveVertex(-50 + (mouseX - 200), 350);
curveVertex(-53 + (mouseX - 200), 350);
curveVertex(-65 + (mouseX - 200), 340);
curveVertex(-60 + (mouseX - 200), 300);
curveVertex(-50 + (mouseX - 200), 280);
curveVertex(-45 + (mouseX - 200), 277);
curveVertex(-40 + (mouseX - 200), 277);
curveVertex(-36 + (mouseX - 200), 278);
curveVertex(-33 + (mouseX - 200), 276);
curveVertex(-30 + (mouseX - 200), 274);
curveVertex(-28 + (mouseX - 200), 272);
curveVertex(-28 + (mouseX - 200), 272);
endShape();
/*legs*/
strokeWeight(1);
stroke(153, 178, 221);
line(-26 + (mouseX - 200), 350, -20 + (mouseX - 200), 380);
line(-52 + (mouseX - 200), 350, -47 + (mouseX - 200), 380);
line(-80 + (mouseX - 200), 320, -50 + (mouseX - 200), 300);
line(-50 + (mouseX - 200), 310, -12 + (mouseX - 200), 290);
/*eyes*/
noStroke();
fill(0);
ellipse(-47 + (mouseX - 200), 300, 5, 10);
ellipse(-37 + (mouseX - 200), 298, 5, 10);
/*background shapes*/
if (mouseX < 200) {
fill(251, 216, 127);
} else if (mouseX < 300) {
fill(230, 170, 206);
} else if (mouseX < 400) {
fill(216, 241, 160);
} else if (mouseX < 500) {
fill(164, 145, 211);
} else if (mouseX >= 500) {
fill(224, 96, 126);
}
translate(width / 2, height / 2);
rotate(PI / 3.0);
rect(-26, -26, 12, 12);
rect(-80, 80, 22, 22);
rect(-36, 180, 32, 32);
rect(10, 35, 18, 18);
rect(100, -160, 20, 20);
}
I was inspired by a game I used to play all the time called Dumb Ways to Die. I found it challenging making sure different components weren’t overlapping each other in places I didn’t want them to be.
03 Generative Art
//Julia Steinweh-Adler
//Section D
var value = 0;
var bg = 0;
function setup(){
createCanvas(600, 450);
rectMode(CENTER);
}
function draw() {
background(value/2, value/4, value*2);
noStroke();
fill(value * 1.3, value * 0.7, value * 0.5); // fill color uses mouse position
let size = dist(mouseX, mouseY, width/2, height);
let sizeI = dist(mouseY, mouseX, width/2, height);
strokeWeight(10);
stroke(value-80, 0, 0); //stroke color uses mouse position in red value
//CAMERA BODY
rect(width/2, height/2, sizeI, sizeI, 20); //rounded cornered square
rect(width/2, height/2, sizeI, value); //widest rectangle
fill(200, 100, 100); //color red
rect(width/2, height/2, size, value); //inner rectangle
fill(value * 1.3, value * 0.7, value * 0.5); //mouse position influenced orange
ellipse(width/2, height/2, size, size); //outer circle
fill(200, 100, 100); //red
ellipse(width/2, height/2, size*0.7, size*0.7); //inner circle
fill(value/2, value/4, value*2)
ellipse(width/2, height/2, size/2.5, size/2.5); //cut center hole
//FLASH - mouse pressed "flash" green square that rotates with mouse coordinates
if (mouseIsPressed) {
fill(100, 200, 150); //green
push();
translate(width/2, height/2); //change origin to center
rotate(radians(mouseY)); //mouse Y coordinates value for degrees of rotation
rect(0, 0, size/6, size/6); //rectangle that is size responsive
pop();
} else { //when mouse not clicked, use constant ellipse
ellipse(width/2, height/2, 50, 50);
}
}
// Fade in and Out Background
function mouseMoved() {
if (bg == 0) {
value += 0.5; //value increase
} if (value == 255) { //value can only increase to 255 before decreasing
bg = 1
} if (bg == 1) {
value -= 0.5; // value decrease
} if (value == 140) { //value can only decrease to 140 before increasing
bg =0
}
}
Project 3 – Dynamic Drawing
This project was designed to produce the numbers “104” if the user puts their mouse in the right location. The red moving square grows bigger and more red the farther you get from the correct point. I was inspired by the spinning motion of many other peer projects as well as some of the examples given. The change I wanted to make was to try and make it a game in which the user has to rotate back and forth until they can find the hidden message. I was also inspired by Prof. Cortina in using “104” as the hidden Easter egg like he has in past examples/ labs.
var xvel = +7 //sets velocity for red square //bgoeke
var x = 0
var y = 0
var r = 225
function setup() {
createCanvas(600, 450);
background(220);
}
function draw() {
background(150, 150, 0);
push();
translate(300, 225);
rotate(radians(mouseX)); //rotates according to position of mouse
fill(255, 255, 0);
rect(0, 0, mouseX, mouseY); //rectangle that makes #1
pop();
push();
translate(425, 300);
rotate(radians(mouseX));
fill(0, 255, 255);
ellipse(0, 0, 50 + mouseX, 50 + mouseY); //ellipse that makes #0
pop();
push();
translate(550, 225);
rotate(radians(mouseX + 40));
fill(0, 255, 255);
rect(0, 0, mouseX, 100); //rectangle that makes top left line of #4
rotate(radians(mouseX - 40));
fill(0, 255, 255);
rect(0, 0, mouseX, 300); //rectangle that makes vertical line of #4
pop();
push();
translate(490, 300);
rotate(radians(0));
fill(255, 255, 0);
rect(mouseX, 0, 100 - mouseX, 225 - mouseY); //rectangle that makes horizontal line of #4
pop();
fill(mouseX - 255, 0, 0);
rect(x, y, mouseX, mouseY - 225); //red rectangle that covers screen when mouse is in wrong direction
x += xvel;
if(x>600-(r/2)) { //makes rect not go past 600
xvel =-xvel
};
if(x<0){ //makes rect not go past 0
xvel =-xvel
};
}
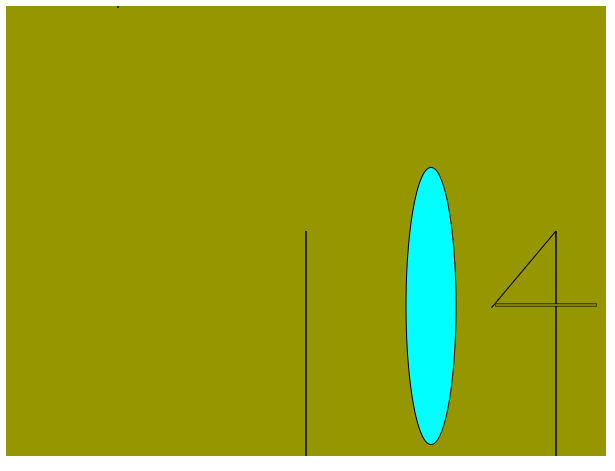
Dynamic Drawing
function setup() {
createCanvas(450, 600);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
noCursor();
fromBlue = color( 130, 255, 240);
toBlue = color(85, 215, 255);
backColor = lerpColor(fromBlue, toBlue, mouseX/mouseY);
background(backColor);
noStroke();
fill(255, 255, 255);
ellipse(mouseX-100, 100, 100, 30);
ellipse(mouseX-100, 90, 50, 30);
ellipse(mouseX+300, 75, 160, 60);
ellipse(mouseX+300, 55, 80, 50)
ellipse(mouseY-200, 230, 160, 60);
ellipse(mouseY-200, 210, 80, 50);
ellipse(mouseY, 150, 100, 30);
ellipse(mouseY, 140, 50, 30);
fill(0, 255, 200);
fromBlue = color( 90, 255, 90);
toBlue = color(40, 255, 190);
blueColor = lerpColor(fromBlue, toBlue, mouseX/mouseY);
fill(blueColor);
ellipse(350, 400, 650, 350);
fill(0, 255, 100);
fromBlue = color( 40, 255, 60);
toBlue = color(30, 255, 150);
blueColor = lerpColor(fromBlue, toBlue, mouseX/mouseY);
fill(blueColor);
ellipse(100, 475, 625, 350);
fill(0, 255, 0);
fromBlue = color( 35, 250, 30);
toBlue = color(20, 245, 140);
blueColor = lerpColor(fromBlue, toBlue, mouseX/mouseY);
fill(blueColor);
ellipse(300, 550, 700, 350);
stroke(220,200,220);
strokeWeight(2);
line(mouseX, mouseY+40, 125, 315);
noStroke();
fill(255, 0, 255);
triangle(mouseX, mouseY, mouseX, mouseY-40, mouseX-30, mouseY);
push();
fill(255, 0, 255);
triangle(mouseX, mouseY, mouseX, mouseY+40, mouseX+30, mouseY);
fill(255, 0, 200);
triangle(mouseX, mouseY, mouseX, mouseY+40, mouseX-30, mouseY);
fill(255, 0, 200);
triangle(mouseX, mouseY, mouseX, mouseY-40, mouseX+30, mouseY);
stroke(40,100,200);
strokeWeight(20)
line(30, 450, 30, 550);
stroke(40,100,200);
strokeWeight(20);
line(70, 450, 70, 550);
noStroke();
fill(40, 100, 200);
ellipse(50, 450, 60, 30);
rect(20, 380, 60, 70);
rect(36,365,24,30);
ellipse(48,350,50);
stroke(40,100,200);
strokeWeight(15);
line(70, 390, 125, 315);
stroke(40,100,200);
strokeWeight(15);
line(22.5, 387.5, 1, 470);
}
Project-03: Dynamic Drawing
// Lauren Kenny (lkenny)
// Project 3 - Dynamic Drawing
// Section A
var xpos = 10;
var ypos = 10;
var xstep = 30;
var ystep = 30;
var r = 200;
var g = 10;
var b = 10;
var d = 5;
function setup() {
createCanvas(450, 600);
frameRate(35);
background(220);
}
function draw() {
//background gets lighter as you move your mouse down
background(0+mouseY*0.1);
//color changes with mouse position
r = .5*mouseX;
g = .5*mouseY;
b = 75;
//creates grid of circles
//color and size change with mouse position
noStroke();
fill(r, g, b);
for (let j = 0; j < width; j++) {
for (let i = 0; i < height; i++) {
ellipse(xpos+(xstep*j), ypos+(ystep*i), (d+mouseY*0.02), (d+mouseY*0.02));
}
}
//ellipse moves with mouse
stroke(r, g, b);
noFill();
ellipse(mouseX, mouseY, 150, 150);
//changes the horizontal spacing between the circles
if (xstep > 40) {
xstep = 30
}
if (30<xstep<40) {
xstep = xstep + 1
}
}
Project 03 – Dynamic Drawing
This was inspired by an app I used to use on my mom’s iPod as a kid. I’m not very good at drawing, so I figured I would give the user a chance to draw. I’ve created a program based on degrees of symmetry that lets you alter the size of the brush, number of brushes, color of the brush, color of the background, and movement of the drawn elements. You can also save your creation by pressing p.
// variable definitions
var degOfSymmetry = 36;
var curAngle = 0;
var diameter = 10;
let drawnCircs = [];
var radiate = false;
var radiateVelocity = 1;
var showRef = true;
let currentColor = ["red", "green", "blue"];
let colorVals = [255, 255, 255];
let backgroundCols = [0, 0, 0];
var colorIndex = 0;
var currentSelect = "Currently selected: " + currentColor[colorIndex];
var whatKey = "";
var rainbowMode = false;
var rainbowCtr = 0;
var freq = 0.01;
function setup() {
createCanvas(600, 600);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
// define background based on color arrays
background(backgroundCols[0], backgroundCols[1], backgroundCols[2]);
noStroke();
// set circle color based on user choice
let c = color(colorVals[0], colorVals[1], colorVals[2]);
if (rainbowMode) {
var rr = sin(rainbowCtr) * 127 + 128;
var rg = sin(rainbowCtr + (2*PI/3)) * 127 + 128;
var rb = sin(rainbowCtr + (4*PI/3)) * 127 + 128;
c = color(rr, rg, rb);
}
fill(c);
// self explanatory
getAngle();
// define a center, get relative mouse radius
var centerX = width / 2;
var centerY = height / 2;
var radX = mouseX - centerX;
var radY = mouseY - centerY;
var mouseRad = sqrt(pow(radX, 2) + pow(radY, 2));
// draw a circle for each degree of symmetry
for (i = 0; i < degOfSymmetry; i++) {
var loopAngle = curAngle - (i * 2*PI / degOfSymmetry)
var tX = centerX + mouseRad * cos(loopAngle);
var tY = centerY + mouseRad * sin(loopAngle);
circle(tX, tY, diameter);
// add to circle history if clicked
if (mouseIsPressed) {
drawnCircs.push([tX, tY, diameter, c, loopAngle]);
}
}
// draw each circle from history
for (i = 0; i < drawnCircs.length; i++) {
fill(drawnCircs[i][3]);
circle(drawnCircs[i][0], drawnCircs[i][1], drawnCircs[i][2]);
// if radiating, begin changing circle positions
if (radiate) {
drawnCircs[i][0] += radiateVelocity * cos(drawnCircs[i][4]);
drawnCircs[i][1] += radiateVelocity * sin(drawnCircs[i][4]);
if (drawnCircs[i][0] > width || drawnCircs[i][0] < 0) {
drawnCircs.splice(i, 1); // remove circles for speed
} else if (drawnCircs[i][1] > height || drawnCircs[i][1] < 0) {
drawnCircs.splice(i, 1); // remove circles for speed
}
}
}
// increment counter for rainbow mode
rainbowCtr = (rainbowCtr + freq) % (2*PI);
// display onscreen reference
if (showRef) {
fill(255);
text("ref: c=change color, d=change degrees of symmetry " +
"s=change circle size, b=background color \n" +
"o=clear screen, r=radiate outwards, p=save screen as png " +
"v=set radiate velocity\n q=rainbow color cycle, " +
"up/down arrow=color/velocity val+-1, right/left arrow=+-10\n" +
"i=change selected color, enter=hide ref, y=rainbow speed",
10, height - 50);
text(currentSelect, 10, 15);
text("RGB: " + (colorVals.toString(10)), 10, 30);
text("Radiate Velocity " + radiateVelocity.toString(10), 10, 45);
text("Background RGB: " + (backgroundCols.toString(10)), 10, 60);
text("Circle Size: " + (diameter.toString(10)), 10, 75);
text("Degrees of Symmetry: " + (degOfSymmetry.toString(10)), 10, 90);
text("Rainbow Frequency: " + (freq.toString(10)), 10, 105);
}
}
// gets angle from mouse pos
function getAngle() {
curAngle = atan2(mouseY - height / 2, mouseX - width / 2);
if (mouseX - width / 2 == 0) {
if (mouseY - height / 2 > 0) {
curAngle = HALF_PI;
} else {
curAngle = 3 * HALF_PI;
}
}
}
function keyPressed() {
// checks what key is pressed, performs an action, or sets a flag
if (key == "c") {
whatKey = "c";
currentSelect = "Currently selected: " + currentColor[colorIndex];
}
if (key == "o") {
drawnCircs = [];
}
if (key == "s") {
whatKey = "s";
currentSelect = "Currently selected: circle size";
}
if (key == "b") {
whatKey = "b";
currentSelect = "Currently selected: background color";
}
if (key == "d") {
whatKey = "d";
currentSelect = "Currently selected: degrees of symmetry";
}
if (key == "r") {
radiate = !radiate;
}
if (key == "q") {
rainbowMode = !rainbowMode;
}
if (key == "v") {
whatKey = "v";
currentSelect = "Currently selected: velocity"
}
if (key == "p") {
save("canvas_drawing.png");
}
if (key == "i") {
colorIndex = (colorIndex + 1) % 3;
currentSelect = "Currently selected: " + currentColor[colorIndex];
}
if (key == "Enter") {
showRef = !showRef;
}
if (key == "y") {
whatKey = "y";
currentSelect = "Currently selected: Rainbow Cycle Speed";
}
// changing parameters that affect drawing and motion
if (keyCode == UP_ARROW) {
if (whatKey == "d") {
degOfSymmetry += 1;
}
if (whatKey == "c") {
colorVals[colorIndex] += 1;
}
if (whatKey == "v") {
radiateVelocity += 1;
}
if (whatKey == "b") {
backgroundCols[colorIndex] += 1;
}
if (whatKey == "s") {
diameter += 1;
}
if (whatKey == "y") {
freq += 0.01;
}
}
if (keyCode == DOWN_ARROW) {
if (whatKey == "d") {
degOfSymmetry -= 1;
}
if (whatKey == "c") {
colorVals[colorIndex] -= 1;
}
if (whatKey == "v") {
radiateVelocity -= 1;
}
if (whatKey == "b") {
backgroundCols[colorIndex] -= 1;
}
if (whatKey == "s") {
diameter -= 1;
}
if (whatKey == "y") {
freq -= 0.01;
}
}
if (keyCode == RIGHT_ARROW) {
if (whatKey == "d") {
degOfSymmetry += 10;
}
if (whatKey == "c") {
colorVals[colorIndex] += 10;
}
if (whatKey == "v") {
radiateVelocity += 10;
}
if (whatKey == "b") {
backgroundCols[colorIndex] += 10;
}
if (whatKey == "s") {
diameter += 10;
}
if (whatKey == "y") {
freq += 0.10;
}
}
if (keyCode == LEFT_ARROW) {
if (whatKey == "d") {
degOfSymmetry -= 10;
}
if (whatKey == "c") {
colorVals[colorIndex] -= 10;
}
if (whatKey == "v") {
radiateVelocity -= 10;
}
if (whatKey == "b") {
backgroundCols[colorIndex] -= 10;
}
if (whatKey == "s") {
diameter -= 10;
}
if (whatKey == "y") {
freq -= 0.10;
}
}
return false;
}
Getting the symmetry correct was a little difficult, as well as figuring out how to deal with user input.
Project 03 – Dynamic Drawing
Is the code more complicated than it needs to be? Yes. Did it still take me way too long to figure out? Also Yes.
//Iris Yip
//15104 Section D
var wwidth = 600,
wheight = 600,
ballsize, //radius
ballx,
bally,
hvelocity,
vvelocity,
clickdistance,
rvalue,greenvalue,bluevalue;
function setup() {
createCanvas(wwidth, wheight);
// starting point
ballx = wwidth/2;
bally = wheight/2;
// starting velocity
hvelocity = random(-5,5);
vvelocity = random(-5,5);
// starting ball size
ballsize = random(10,150);
// ball color
redvalue = random(100,255);
greenvalue = random(100,255);
bluevalue = random(100,255);
};
function draw() {
//background
background(0)
let rectBlue = map(mouseY, 0, height, 0, 255);
fill(255, rectBlue, 200);
rect(0,0, 600, 600);
fill(redvalue, greenvalue, bluevalue);
ellipse(mouseX, mouseY, 100, 100);
// ball bounce-back conditions
if(ballx >= width - ballsize || ballx <= ballsize){
hvelocity = -hvelocity;
};
if(bally >= height - ballsize || bally <= ballsize){
vvelocity = -vvelocity;
};
// ball location change
ballx += hvelocity;
bally += vvelocity;
// render ball
noStroke();
fill(redvalue, greenvalue, bluevalue);
ellipse(ballx, bally, ballsize * 2, ballsize * 2);
};
function mouseClicked() {
{
clickdistance = dist(mouseX, mouseY, ballx, bally);
if(clickdistance <= ballsize) {
// change velocity if clicked on the ball
hvelocity = random(-5,5);
vvelocity = random(-5,5);
// change ball color
redvalue = random(100,255);
greenvalue = random(100,255);
bluevalue = random(100,255);
//changing ball size
ballsize = random(50,100);
};
};
};
PROJECT 03- DYNAMIC DRAWING
//JUNE LEE
//SECTION C
var offset=20;
var diam=0;
function setup() {
createCanvas(600,450);
}
function draw() {
//background change
if (mouseX>(width/2)) {
background(245,245,142);
} else {
background(240,220,106);
}
// purple LIGHT increasing size USING
noStroke();
fill(215,209,240);
diam=mouseY;
ellipse(600/2,height/2,diam,diam);
//circle slant line mouse Y
fill(148,132,215);
noStroke();
ellipse(0,mouseY-40,30,30);
ellipse(0+(offset*2),mouseY-35,30,30);
ellipse(0+(offset*4),mouseY-30,30,30);
ellipse(0+(offset*6),mouseY-25,30,30);
ellipse(0+(offset*8),mouseY-20,30,30);
ellipse(0+(offset*10),mouseY-15,30,30);
ellipse(0+(offset*12),mouseY-10,30);
ellipse(0+(offset*14),mouseY-5,30,30);
ellipse(0+(offset*16),mouseY,30,30);
ellipse(0+(offset*18),mouseY+5,30,30);
ellipse(0+(offset*20),mouseY+10,30,30);
ellipse(0+(offset*22),mouseY+15,30,30);
ellipse(0+(offset*24),mouseY+20,30,30);
ellipse(0+(offset*26),mouseY+25,30,30);
ellipse(0+(offset*28),mouseY+30,30,30);
ellipse(0+(offset*30),mouseY+35,30,30);
//rect line mouse X
noStroke();
fill(111,88,209);
rect(0,mouseX+40,20,30);
rect(0+(offset*2),mouseX+35,20,30);
rect(0+(offset*4),mouseX+30,20,30);
rect(0+(offset*6),mouseX+25,20,30);
rect(0+(offset*8),mouseX+20,20,30);
rect(0+(offset*10),mouseX+15,20,30);
rect(0+(offset*12),mouseX+10,20,30);
rect(0+(offset*14),mouseX+5,20,30);
rect(0+(offset*16),mouseX,20,30);
rect(0+(offset*18),mouseX-5,20,30);
rect(0+(offset*20),mouseX-10,20,30);
rect(0+(offset*22),mouseX-15,20,30);
rect(0+(offset*24),mouseX-20,20,30);
rect(0+(offset*26),mouseX-25,20,30);
rect(0+(offset*28),mouseX-30,20,30);
rect(0+(offset*30),mouseX-35,20,30);
rect(0+(offset*32),mouseX-40,20,30);
//text fixed
translate(0,0);
textAlign(CENTER);
fill(0);
noStroke();
textSize(10);
text("JUNE LEE / SECTION C",width/2,10);
//text curve
translate(100,150);
rotate(radians(mouseX/7));
fill(0);
noStroke();
textSize(20);
text("JUNE LEE / SECTION C",200,20);
}
I wanted to make all aspects relate to the mouse position. I explored size, position (x and y), color, and position (rotation) through this drawing.