var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 50;
function setup() {
createCanvas(400, 300);
background(200);
line(0, 0, 0, 300);
line(300, 300, 350, 100);
dx1 = (0-0)/numLines;
dy1 = (300-0)/numLines;
dx2 = (550-100)/numLines;
dy2 = (1)/numLines;
}
function draw() {
background(0)
var x1 = 40;
var y1 = 0;
var x2 = 0;
var y2 = 300;
var x4 = 0;
var y4 = 400
for (var i = 0; i <= numLines; i += 1) {
stroke(0,0,255);
line(mouseX/1.7, 0, 400, y1); //blue curves
stroke(255,0,0)
line(mouseX-30,y1,x2,y2); //red curves
stroke(0,255,0)
line(mouseX/1.2,y1,y4,y2) //green curves
x1 +=dx1
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
}
Category: Project-04-String-Art
Project 04-String Art
var dx2;
var dy2;
var dy3;
var numLines = 30;
function setup() {
createCanvas(400, 300);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
noStroke();
line(200, 50, 200, 250); //vertical guide line
line(100, 50, 300, 250); //diagonal guide line
dx2 = (200)/numLines;
dy2 = (200)/numLines;
dy3 = (300)/numLines;
}
function draw() {
background(0);
// outer ring
push();
translate(200, 150); //origin in the middle
for (let i = 1; i <= 200; i += 1) {
rotate(2); //rotating around the origin
stroke(184, 156, 46, 70)
line(-50, -130, 200, -150);
}
pop();
// the middle square
var x1 = 100;
var y1 = 250;
var x2 = 100;
var y2 = 50;
for (let i = 0; i <= numLines; i += 1) {
stroke(111, 162, 179, 150);
line(x1, y1, x2, y2);
line(x1+200, y1-200, x2, y2);
x2 += dx2;
y2 += dy2;
}
var x1 = 100;
var y1 = 150;
var x2 = 200;
var y2 = 50;
for (let i = 0; i <= numLines; i += 1) {
//biggest diamond
stroke(40, 77, 89);
line(x1-100, y1, x2, y2-100);
line(x1-100, y1, x2, y2+100);
line(x1+300, y1, x2, y2-100);
line(x1+300, y1, x2, y2+100);
//smallest diamond
stroke(111, 162, 179);
line(x1, y1, x2, y2);
line(x1+200, y1, x2, y2)
y2 += dy2;
}
noLoop();
}
This is my string art inspired by a diamond ring. I wanted the diamonds to look three-dimensional.
Project-04-String-Art
//Isabel Xu
//Project 04
var numLines = 50;
var LineEndX = 200
var LineEndY = 390
function setup() {
createCanvas(400, 300);
}
function draw() {
var LineEndX = constrain(mouseX,50,350);
var LineEndY = constrain(mouseY,50,250);
//the two lines of the movable cape
background(136,136,195);
stroke(255);
line(110, 80, LineEndX, LineEndY);
line(200, 50, 50, 240);
dx1 = (LineEndX-110)/numLines;
dy1 = (LineEndY-80)/numLines;
dx2 = (50-200)/numLines;
dy2 = (240-50)/numLines;
//the two lines of headpiece
line(290, 80, LineEndX, LineEndY);
line(200, 50, 350, 240);
hx3 = (LineEndX-290)/numLines;
hy3 = (LineEndY-80)/numLines;
hx4 = (350-200)/numLines;
hy4 = (240-50)/numLines;
var x1 = 110;
var y1 = 80;
var x2 = 200;
var y2 = 50;
var x3 = 290
var y3 = 80
var x4= 200
var y4 = 50
//human figure body
noStroke();
fill(159,160,195);
rect(140,65,120,400,50,50,45,45);
//generate the strings between lines
for (var i = 0; i <= numLines; i += 1) {
stroke(random(255),200,random(255));
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
line(x3, y3, x4, y4);
x3 += hx3;
y3 += hy3;
x4 += hx4;
y4 += hy4;
}
for (var i = 0; i <= numLines; i++){
strokeWeight(0.75);
stroke(220,220,random(255));
line(mouseX, i * 2+30, 220 - i, 80);
}
noStroke();
fill(159,160,215);
ellipse(200,40,60,60);//head
noStroke();
fill(191,154,143);
ellipse(175,50,18,14);
ellipse(225,50,18,14);//blush
}
The idea of this project is having an adjustable wearable cape that is made with interactive e-textile. Mouse location updates the shape of the cape.
Project 4 – String Art
//Bennett Goeke
var angle = 0
function setup() {
createCanvas(400, 400);
background(50);
}
function draw() {
strokeWeight(10) // background lines
stroke(255, 0, 255) // pink
for (x = 0; x <= width; x += 30)
line(x, 0 , x, height) //repeating lines in loop
noFill();
stroke(0); // black
push();
translate(200, 200) // origin moved to center
rotate(radians(angle)); // rotates record
strokeWeight(1);
for (var r = 100; r <= 250; r += 4){ // repeating circles
circle( 0, 0, r)
}
strokeWeight(10)
fill(27); // shade of black
circle(0, 0, 100); // inner fill circle of record
translate(-200, -200); // origin back to 0,0
stroke(255, 255, 0); //yellow
for (var y = 180; y <= 220; y += 5){
line(180, y, 200, 200) // yellow V-shape
}
push();
translate( 200, 200); // origin back to center
fill( 255, 0, 0); // red
rect( 10, -20, 15, 40); // yellow label with red inside
pop();
angle += 3 // rotataing angle increases 3 degrees per frame
pop();
}
I decided to make a spinning record with a gold label and then kind of transformed into into a logo of sorts.
Project-04-String-Art
// Xander Fann xmf Section B
var bx = 0
var len = 10
var up = 0
function setup() {
createCanvas(400, 300);
background(0);
}
function draw() {
push()
translate(0,up)
background(255)
stroke(200,200,255)
for (var y = -5000; y <= 5000; y += 5){
line(bx,y,bx+width,y);
}
//balloon
noFill()
stroke(255,0,0) // red
for(var arcH = 0; arcH <= 130; arcH += 3){
arc(200,180,arcH,130,-PI/2,PI/2)
}
noFill()
stroke(0,255,0) // green
for(var arcH = 0; arcH <= 130; arcH += 3){
arc(200,180,arcH,130,PI/2,-PI/2)
}
noFill()
stroke(0,0,255,70) // blue
for(var arcH =- 70; arcH <= 0; arcH += 1){
arc(200,180,arcH,130,PI/2,-PI/2);arc(200,180,arcH,130,-PI/2,PI/2)
}
//basket
//basket horizontal
stroke(214, 174, 56)
for(var y = 260; y<= 290; y += 2){
line(180,y,220,y)
}
//basket vertical
stroke(214, 174, 56)
for(var x = 180; x <= 220; x += 2){
line(x,260,x,290)
}
//connection strings
stroke(0)
for(var x = 160; x <= 240; x += 20){
line(x,230,200,260)
}
//movement
up -= 3
if (mouseIsPressed) {up += 10}
pop()
//moutains
noFill()
stroke(100,255,0)
for(r = 200; r<= 300; r += 3)
ellipse(200,400,800,r)
}
I started by thinking about how the density of strings can affect each other and how overlapping strings is similar to the idea of hatching make an object look 3 dimensional like a sphere. I thought of a hot air balloon where multiple colors can also be used to show the roundness of the balloon. When the screen is pressed the balloon also moves downwards.
Project 04: String Art
//Carson Michaelis
//Section C
var yy = -25
var xx = -25
function setup() {
createCanvas(400, 300);
background(220);
}
function draw() {
var y = 300
var y3 = 0
//make sure there's no trails
background(220);
//black ellipse in middle
push();
noStroke();
fill(0);
ellipse(200,150,280,225);
pop();
noFill();
strokeWeight(.5);
//moving points in middle
if (yy == -25 & xx < 25) {
xx += 1
} else if (xx == 25 && yy < 25) {
yy += 1
} else if (yy == 25 && xx > -25) {
xx -= 1
} else if (xx == -25 && yy > -25) {
yy -= 1
}
//generating lines from side to side
for (let x = 0; x <= 400; x += 400/24) {
line(x,0,0,y);
line(x,0,400,300-y);
line(x,300,0,300-y);
line(x,300,400,y)
y -= 300/24;
}
push();
stroke(255);
//lines connecting middle portion to outer lines
line(200,25,365,150);
line(200,25,35,150);
line(200,275,35,150);
line(200,275,365,150)
print(xx.toString());
print(yy.toString());
//generating moving lines inside ellipse
for (let x = 0; x <= 20; x += 1) {
line((x*(33/4))+200,y3+25,200+xx,150+yy);
line((x*(-33/4))+200,y3+25,200-xx,150+yy);
line((x*(33/4))+200,300-(y3+25),200+xx,150-yy);
line((x*(-33/4))+200,300-(y3+25),200-xx,150-yy);
y3 += (25/4);
}
pop();
}
For this project I wanted a portion of the lines to move, as well as to create black and white lines that contrast with each other. Here they explore the idea of an open versus occupied middle, as well as the way overlapping lines interact.
Project-04 stringArt
// Huijun Shen session D
// huijuns@andrew.cmu.edu
var gray = 0;
function setup() {
createCanvas(600,450);
background(100);
strokeWeight(0.5);
}
function draw() {
background(100);
gray = mouseX/ 2;
fill(255-gray);
noStroke();
circle(width / 2, height / 2, 450);
dist(mouseX , mouseY , width/2, height/2 )
for(var i = 50; i <= 600; i += 50){
if(dist (mouseX, mouseY, width/2, height/2 ) < 225 ){
stroke(255);
line(i, 0, constrain(mouseX, 75, 525), constrain(mouseY,0,450));
line(constrain(mouseX, 75, 525), constrain(mouseY,0,450), i , height);
} else {
stroke(0);
line(i, 0, constrain(mouseX, 75, 525), constrain(mouseY,0,450));
line(constrain(mouseX, 75, 525), constrain(mouseY,0,450), i , height);
}
}
for( var e = 30; e <= 450 ; e += 10){
line(0,e,mouseX,i);
line(600,e,mouseX,i);
}
}
Project 04 – String Art
//Rachel Kim
//15-104(Section C)
//rachelki@andrew.cmu.edu
//Project-04
// x & y coordinates for lines
var x = 0;
var y = 0;
function setup() {
//assigned canvas size
createCanvas(600,600);
}
function draw() {
//dark blue background color
background(3, 43, 67);
//criss-crossed lines
for (var i = 0; i <= 50; i += 1) {
//blueA lines
stroke(155, 189, 249);
strokeWeight(mouseX/300); //changes line thickness
line(x, height - i*10, width - i*8, height + i);
//blueB lines
stroke(196, 224, 249);
strokeWeight(mouseY/300); //changes line thickness
line(height + i*0.5, width + i*40, x + i*10, y - i*30);
//green lines
stroke(178, 255, 168);
strokeWeight(mouseX/300); //changes line thickness
line(height - i*15, y + i/0.08, x, height - i/0.03);
//yellow lines
stroke(255, 249, 165);
strokeWeight(mouseY/300); //changes line thickness
line(width + i/0.05, y + i*50, x, height - i/0.07);
//pink lines
stroke(252, 109, 171);
strokeWeight(mouseX/300); //changes line thickness
line(x + i*20, height - i/20, width - i/2, height - i*20);
}
}
/*
//blueC
stroke(79, 100, 158);
strokeWeight(1);
line(height + i*10, y + i/0.05, x, width - i/0.03);
*/
I wanted to explore the different patterns that the lines are able to create by using a crisscross technique. I also wanted to play around with the weight of the stroke of those lines as the user moves around the canvas.
Project 4: String Art
let yPerl = 0.0;
function setup() {
createCanvas(400, 300);
pinkSky = color(252, 224, 217);
blueSky = color(190, 228, 235);
mountainColor = color(46, 130, 129);
treeColor = color(162, 179, 137);
sunColor = color(255, 181, 181);
}
function draw() {
background(0);
//the sky
let changeSky = map(mouseY, 0, height, 0, 1);
var skyColor = lerpColor(pinkSky, blueSky, changeSky);
fill(skyColor);
rect(0, 0, width, height/1.5);
//the sun
noStroke();
ellipseMode(CENTER);
fill(sunColor);
var stopMouseX = constrain(mouseX, 100, 300);
var stopMouseY = constrain(mouseY, 0, 300);
let sunX = map(stopMouseX, 0, width, 0, 400);
let sunY = map(stopMouseY, 0, height, 0, 300);
ellipse(sunX, sunY, 90, 90);
//generative mountains
fill(mountainColor);
beginShape();
let xPerl = 0;
for (let x = 0; x<= width; x += 10) {
let y = map(noise(xPerl), 0, 1, 200, 50);
vertex(x, y);
xPerl += 0.2;
}
yPerl += 0.2;
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
//trees
push();
for (var i = 0; i <= 400; i += 1) {
stroke(treeColor);
strokeWeight(0.5);
line(50, i + 50, i, height); //1st tree from the left
line(100, i + 20, i + 50, height + 25); //2nd tree
line(150, i + 70, i + 70, height + 50); //3rd tree
line(225, i + 80, i + 200, height + 50); //4th tree
line(width/2, i + 125, i + 150, height); //5th tree
line(300, i + 60, i + 250, 300); //6th tree
line(350, i + 100, i + 300, 350); //7th tree
line(400, i + 100, i + 350, 400); //8th tree on far right
}
pop();
}

I was loosely inspired by the sunrises and sunsets at Yosemite National Park. The mountains regenerate randomly every time the page is reloaded.
Project 4: String Art
// Susie Kim
// Section A
function setup() {
createCanvas(300, 400);
background(235, 215, 192);
}
function draw() {
background(235, 215, 192);
for (var i = 0; i <= 100; i+= 5) {
// back mountain range lines
strokeWeight(1);
stroke(173, 178, 185);
line(0, 100 + i*2, i*5, 120 + i*4); // left set
line(300, 120 + i, i*3, 200 + i*3); // right set
// water lines
stroke(81, 99, 174);
line(300, 220 + i*4, i*3, 220 + i*4);
// water lines darker
strokeWeight(0.5);
stroke(27, 50, 148);
line(300, 265 + i*5, i*4, 265 + i*5);
// black forefront lines
stroke(0);
strokeWeight(1);
line(0, 260 + i*2, i*9, 280 + i*4); // left set
strokeWeight(1.5);
line(300, 330 + i*2, i*0.1, 360 + i*3); // darker right set
// sun reflection
stroke(255, 133, 71);
strokeWeight(1);
noFill();
ellipse(100, 100 + i*2, 60-i, 60-i);
// birbs
stroke(50);
strokeWeight(0.5);
ellipse(200+i*2, 100 - i*3, 30-i, 30-i);
ellipse(200+i*2, 40 - i*4, 20-i, 20-i);
}
}
For this week’s project, I tried to take the painting pictured below and convert it into an abstract string art design. I had a lot of fun playing with the shapes both lines and ellipses could create!
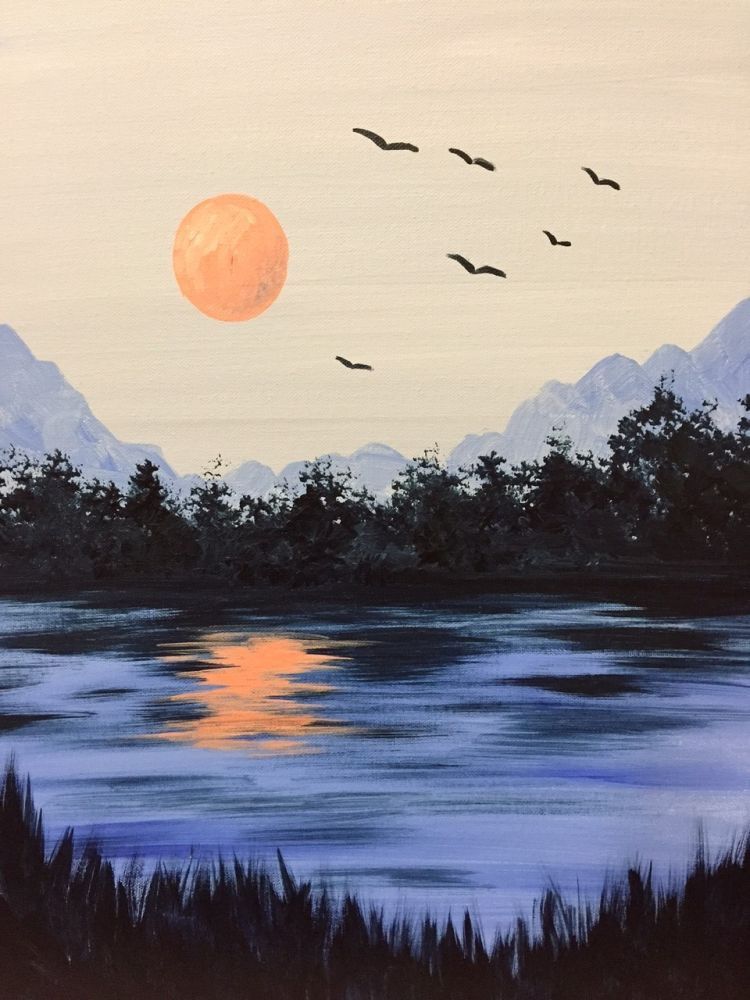