var y = 0;
function setup() {
createCanvas(480, 480);
frameRate(20);
}
function draw() {
var v = 25
background(230, 207, 106);
//glass beaker
fill(225, 230, 255);
noStroke();
rect(130,480,220,-270);
stroke(80);
strokeWeight(3);
line(130,480,130,480-270);
line(480-130,480,480-130,480-270);
ellipse(240,480-270,220,10);
//waterdrop
fill(103,197,255);
noStroke()
ellipse(240,y,45);
y = y + v;
if (y > 480) {
y = 0
}
//Pipe dropping water from the top
noStroke();
fill(71, 76, 84);
rect(210,0,60,90);
fill(0);
ellipse(240,90,60,4);
noStroke();
//water level indicating seconds
noStroke()
fill(103,197,255);
rect(130,480,220,-second()*4.5);
stroke(83,167,245)
strokeWeight(0.5)
ellipse(240,480-second()*4.5,220,10);
loop()
//beaker outline
noFill();
stroke(80);
strokeWeight(3)
line(130,480,130,480-270);
line(480-130,480,480-130,480-270);
ellipse(240,480-270,220,10);
}
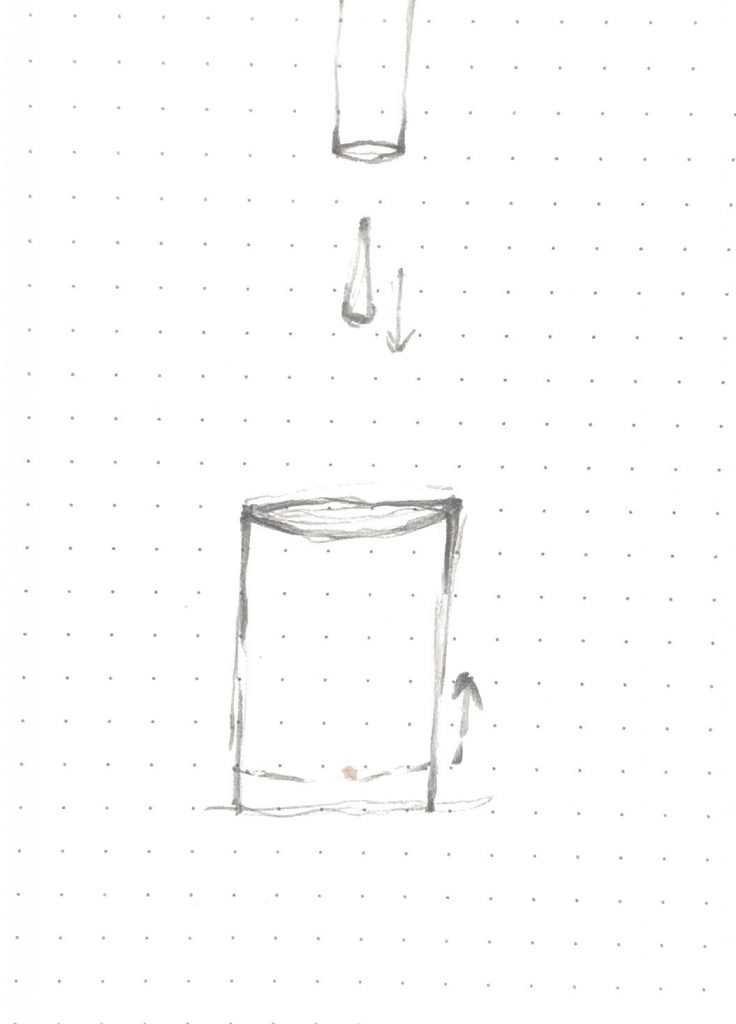
When thinking of a clock, or the idea of a clock, I was fascinated by the idea of how only something constant can be used as a device to define time. Systems that are perfectly constant are hard to find in this world, therefore we try to fine tune what we have to create a machine we can rely on. Water droplet’s relationship with gravity is constant most of the time. Therefore I thought it would be interesting to measure time with how fast droplets of water fill up a glass. Realistically, there are many other components in play that will affect how accurate this “clock” is but I found this alternate method of measuring time very fascinating.