var strokeX = 7 //horizontal size of dots
var strokeY = 7 //vertical size of dots
var size = 7 //space between dots
var portrait; //my image
var painted = []; //the painted
var erased = []; //the erased area
var erasing; //boolen for whether or not erasing has been activated
var brushSize = 20 //defines what is "near mouse"
function preload(){
portrait = loadImage("https://i.imgur.com/iJq4Jtv.jpg") //loading imgur image into variable
}
function paint(){ //draw function for paint object
fill(portrait.get(this.x,this.y)) //calling the color at the certain image coordinate
ellipse(this.x,this.y,strokeX,strokeY) //drawing an ellipse at that coordinate
}
function erase(){
fill(255,241,175)
ellipse(this.x,this.y,strokeX,strokeY)
}
function makePaint(px,py){ //constructor for paint object
p = {x:px,y:py,
drawfunction:paint
}
return p;
}
function makeErase(ex,ey){ //constructor for erase objecg
e = {x:ex,y:ey,
drawfunction:erase
}
return e;
}
function setup() {
createCanvas(300, 400);
background(255,241,175); //beige
noStroke();
ellipseMode(CORNER); //ellipses defined by "corner"
}
function draw() {
background(255,241,175) //beige
for(i=0;i<painted.length;i++){ //display function for paint object
painted[i].drawfunction() //calling the draw function for the array of objects
}
if(mouseIsPressed){
for(x=0;x<=width;x+=size){ //for loop to set up dots
for(y=0;y<=height;y+=size){
if (nearMouse(x,y) == true){
if(erasing == false){
var p = makePaint(x,y)
painted.push(p)
brush(mouseX,mouseY)
}
if(erasing == true){
var e = makeErase(x,y)
painted.push(e)
eraser(mouseX,mouseY)
}
}
}
}
}
fill(128,88,43); //brown
rect(0,0,width,20); //drawing the border
rect(0,0,20,height);
rect(0,380,width,20);
rect(280,0,20,height)
fill(255);
textSize(7);
text('Press P and Drag to Paint',20,13); //instructions
text('Press E and Drag to Erase', 195,13);
text('Press A to paint abstractly',20,391);
text('Press R to paint realistically',190,391);
text('b for smaller brush',120,8);
text("B for bigger brush", 120,15);
}
function nearMouse(x,y){
if (dist(x,y,mouseX,mouseY)<brushSize){ //if the mouse is within "brush size" of given x and y coordinates
return true;
}
return false;
}
function brush(x,y){ //drawing the paintbrush
push();
rectMode(CENTER);
fill(222,184,142); //light brown
rect(x,y+20,15,70); //the handle
ellipseMode(CENTER);
if(mouseIsPressed){
fill(portrait.get(x,y)) //fill with color of image coordinate that it is above
}else{
fill(0) //fill black is not pressing
}
ellipse(x,y-5,20,12) //the brush
triangle(x,y-25,x-10,y-5,x+10,y-5)
pop();
}
function eraser(x,y){
push();
rectMode(CENTER);
fill(245,116,240) //pink
rect(x,y,30,40) //eraser shape
pop();
}
function keyPressed (){
if(key=="a"){
strokeX = random(5,100) //pick random shape of the dots, and random seperation
strokeY = random(5,100)
size = random(0,5)
}
if(key=="r"){ //shrink the dots and space between
strokeX = 2
strokeY = 2
size = 2
}
if(key == "B"){
brushSize +=5 //increase the brush size (more dots included in nearmouse function)
}
if(key == "b"){ //decrease brush size (less dots included)
brushSize -=5
}
if(key == "p"){
erasing = false //not erasing (painting)
}
if(key =="e"){
erasing = true //erasing (not painting)
}
}
Category: Project-09-Portrait
Project- 09- Portrait
//Shruti Prasanth
//Project 9- Portrait
//Section C
function preload() {
var portrait="https://i.imgur.com/CDcRoOA.jpeg"; // image by Aliena85
photo=loadImage(portrait); //image variable
}
function setup() {
createCanvas(300,400);
background(0);
photo.resize(300,400); //resizing the image to fit the canvas
photo.loadPixels();
frameRate(10000000000000000000000*100000000000000000);
}
function draw() {
var x=random(width);
var y=random(height);
var pixelx=constrain(floor(x),0,width);
var pixely=constrain(floor(y),0,height);
var pixelcolor=photo.get(pixelx,pixely); //calling each pixel color
noStroke();
fill(pixelcolor); //colors of the squares that appear
square(x,y,random(0,5)); // squares that form the image
}
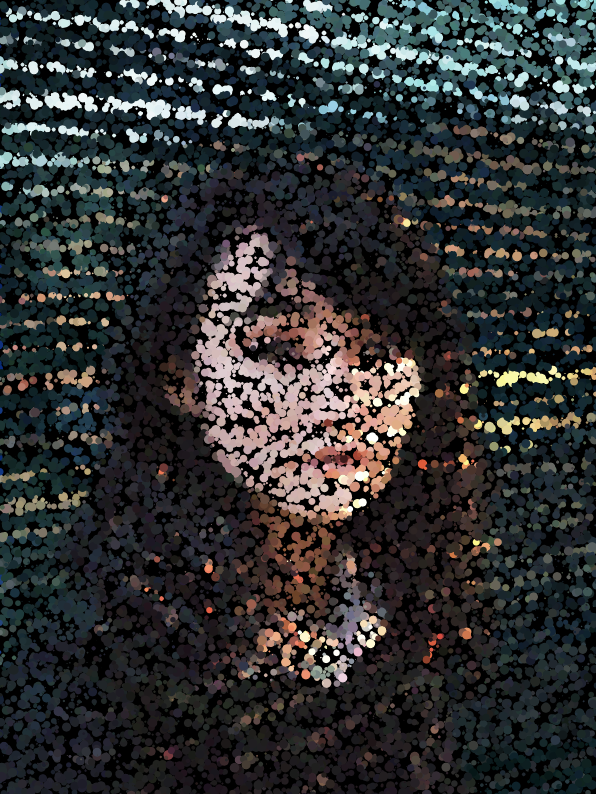
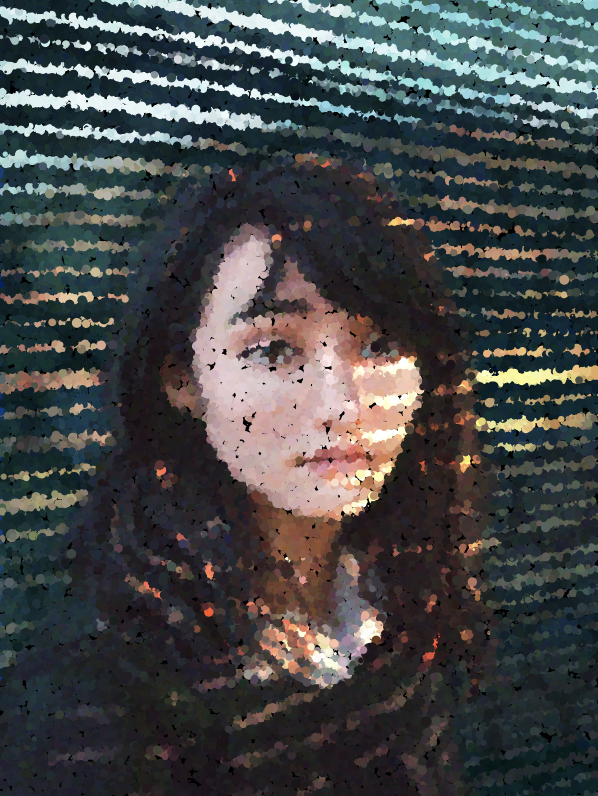
My inspiration for this portait was this painting of a girl submitted by Aliena85 on imagur.com. I thought the strokes that created the background and the sunlight filtering through the blinds would be interesting if they appeared in a stippling colored pixel form. It feels magical as the image starts to form because the specs look like colored dust, and gives the portrait of the girl a glowy quality.
Project-09 Portrait
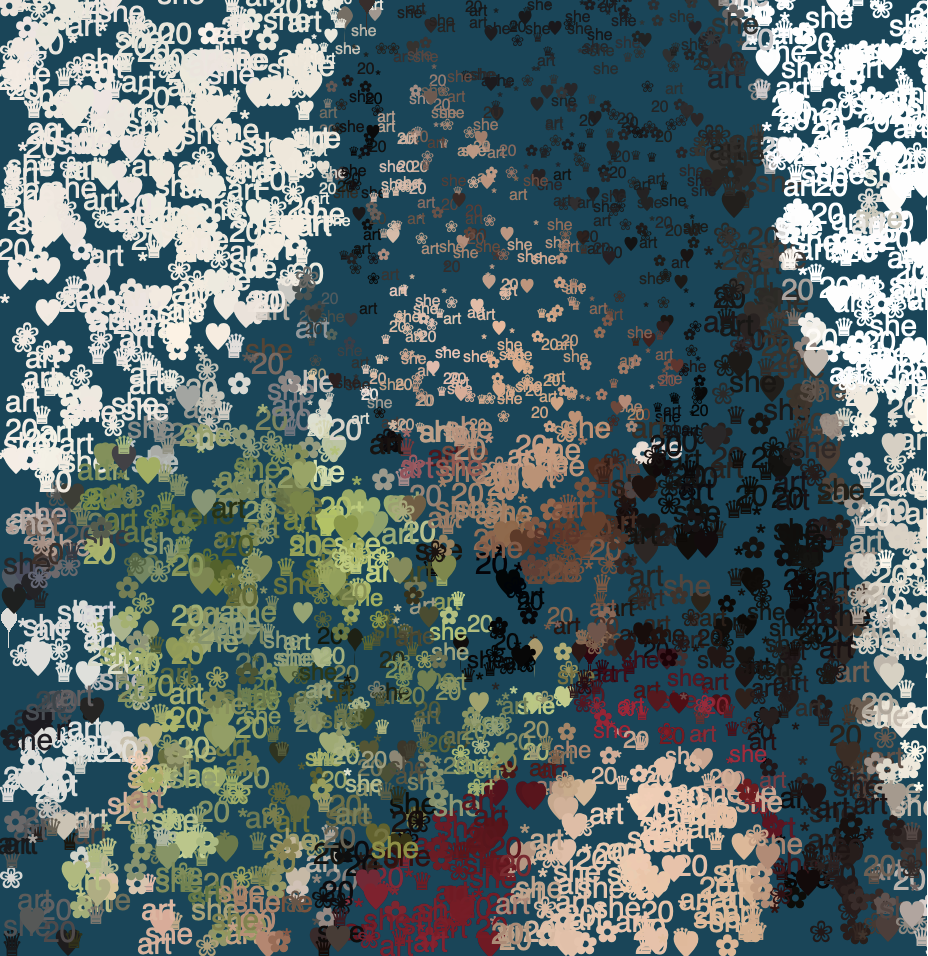
I made this interactive work which allows the user to move their mouse around to fill out the image with the brush feature. The brush has the shape of leaves. The user can change the brush shape with key press. I want to create a painting like atmosphere. The elements at each pixel consists of text “she, 20, art” and patterns such as flowers, stars, hearts, etc.
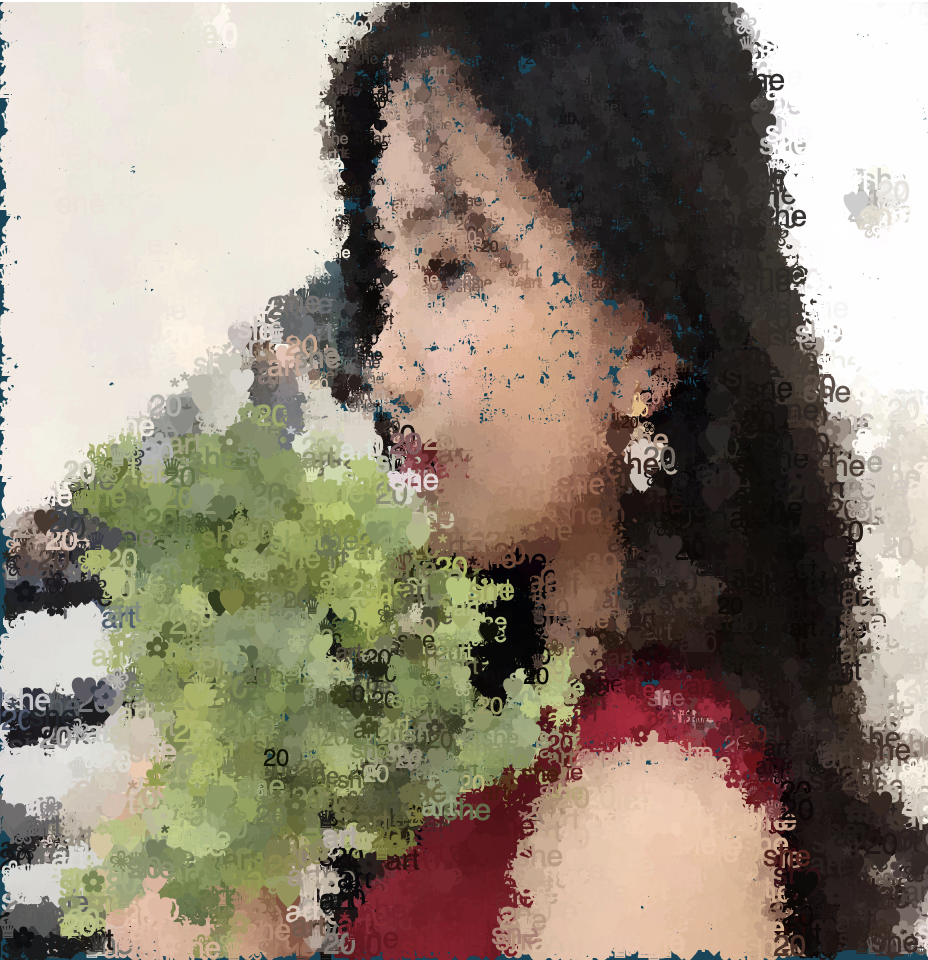
//Heying Wang
//section B
let img;
//key words abut me
let words=['20','she','art','✿','*','♥︎','❀','♛']
var leafShape='serrate'
function preload() {
img = loadImage('https://i.imgur.com/fgOhCaN.jpeg');
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
noStroke();
background(255);
img.loadPixels();
img.resize(480,480);
background(map(mouseX,0,width,0,255),70,90);
frameRate(10000);
}
//find the pixel color
function draw() {
let x = floor(random(img.width));
let y = floor(random(img.height));
let pc = img.get(x, y);
fill(pc);
//smaller founts for important parts of the image
//so that there will be more details
if(x>150 & x<350 && y>0 && y<220){
textSize(8);
}
else if(x>120 & x<400 && y>300 && y<400){
textSize(12);
}else{
textSize(15)
}
/* brush Feature: the player can move
the mouse around to fill out the image faster
the brush stroke has the shape of leaves */
text(random(words),x,y);
if(leafShape=='serrate'){
fill(img.get(mouseX,mouseY));
beginShape()
curveVertex(mouseX,mouseY);
curveVertex(mouseX,mouseY-8);
curveVertex(mouseX-4,mouseY-15);
curveVertex(mouseX,mouseY-20);
curveVertex(mouseX+4,mouseY-15);
curveVertex(mouseX,mouseY-8);
endShape(CLOSE);
}
//change brushes
else if (leafShape=='digitate'){
fill(img.get(mouseX,mouseY));
line(mouseX,mouseY,mouseX,mouseY-6);
beginShape();
vertex(mouseX,mouseY);
vertex(mouseX,mouseY-5);
vertex(mouseX+8,mouseY-8);
vertex(mouseX,mouseY-11);
vertex(mouseX-8,mouseY-8);
endShape(CLOSE);
}
console.log(leafShape);
}
function keyTyped(){
if(key==='d'){
leafShape='digitate'
}
else if(key==='s'){
leafShape='serrate'
}
}
Project 9
This week, I coded a self-portrait based on an old picture of myself. I wanted to express my love for drawing and painting, so I included a draw and erase mode. As the portrait renders, it adopts a painterly style with brushstrokes in the shape of a pencil. When mouse is pressed, it goes into erase mode. Together, they demonstrate the imperfect creative process which I’ve become familiar with.
sketch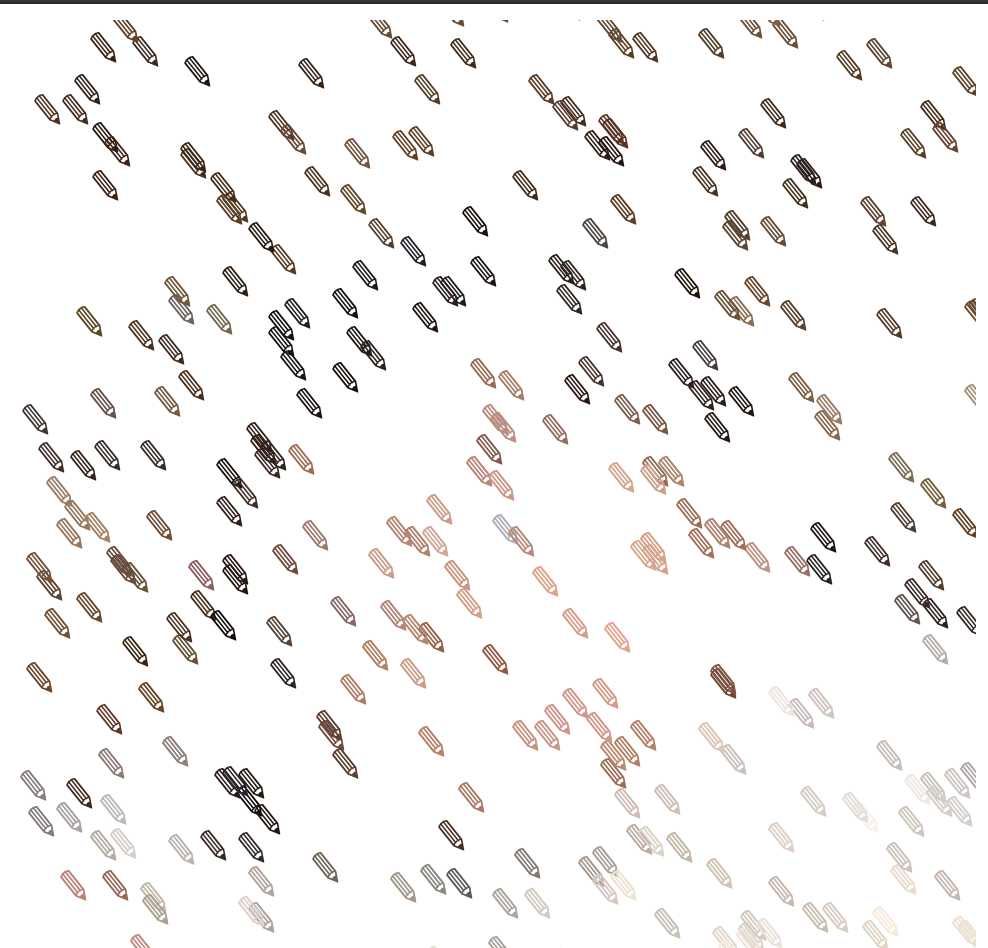
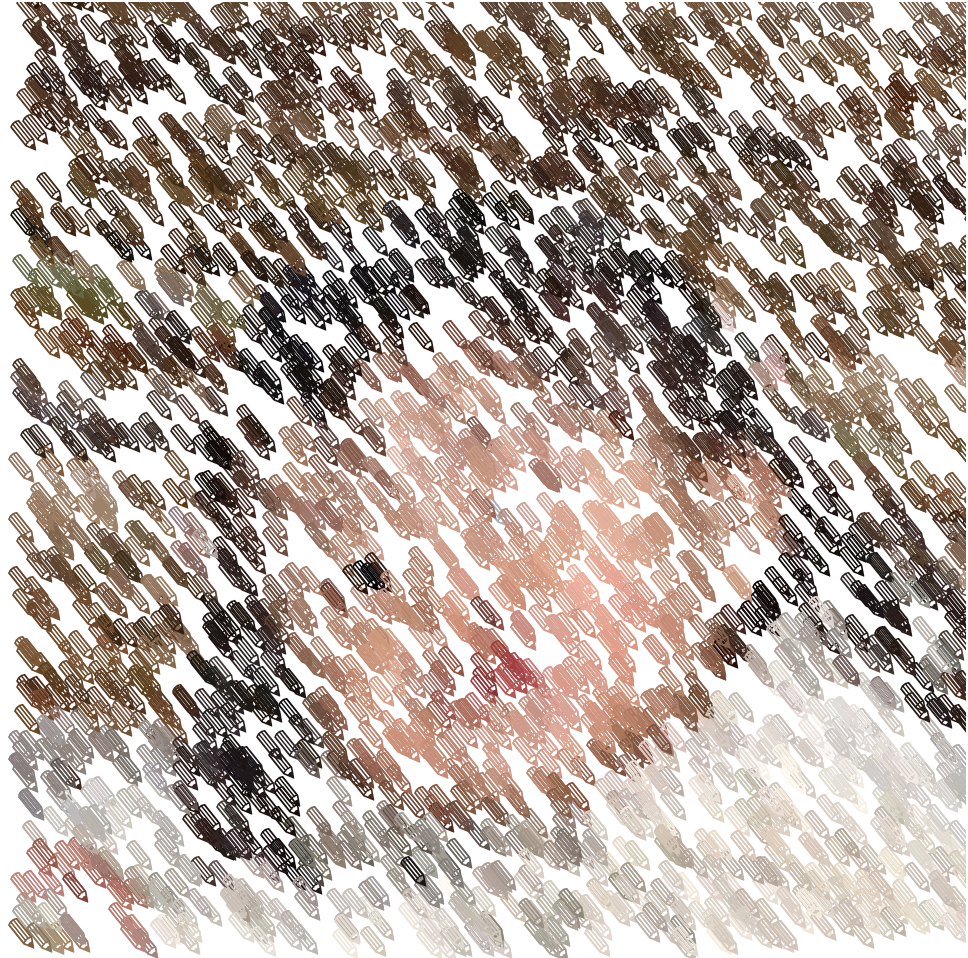
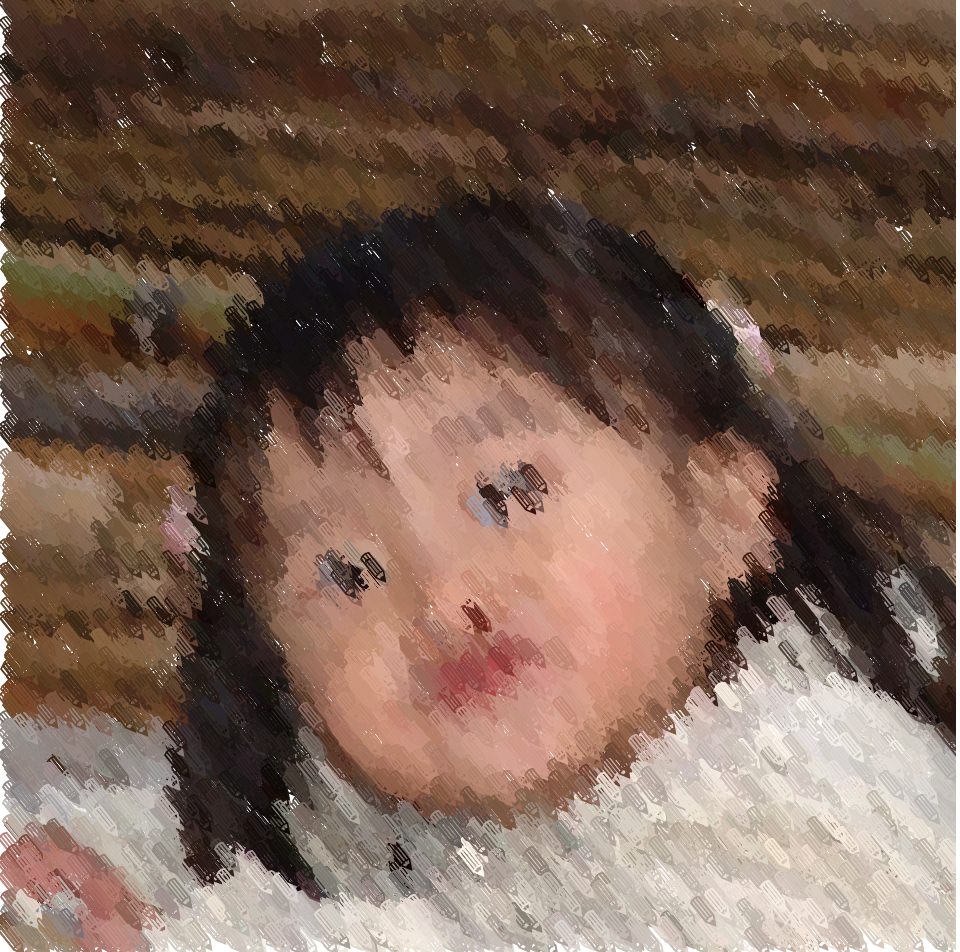
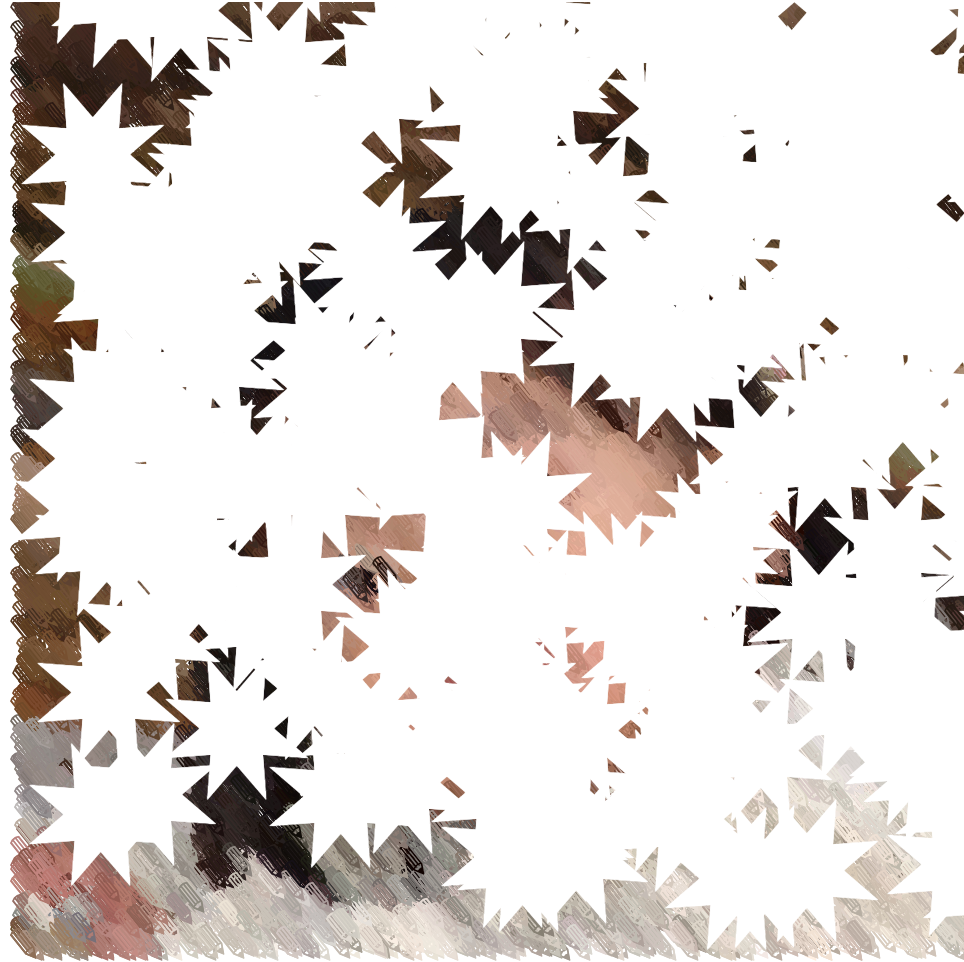
var img;
var txttype = 1;
function preload() {
img = loadImage("https://i.imgur.com/iUeoP8t.jpg");
}
function setup() {
createCanvas(480, 480);
background(255);
img.loadPixels();
frameRate(10000000);
}
function draw() {
//image(img, -20, -10, 640, 500); //fix warped image
//image (img, 0, 0);
var x = floor(random(img.width)); // random x position
var y = floor(random(img.height)); // random y position
var pixel = img.get(x, y); // associate locations of pixels to image
fill(pixel); //text color is pixel color
noStroke();
//var time = millis(); //text movement = rotation to milliseconds
//rotateX(time / 1000);
//rotateY(time / 1000);
textSize(20);
if (txttype ==1){ //drawmode
// rect(x, y, 10, 10);
textSize(20);
text ("✎", x, y);
}
if (txttype ==2){ //erase mode
push();
fill(255);
textSize(100); //erase faster than draw
text ("✸", x, y);
pop();
}
}
function mousePressed(){
//switch between draw and
if (txttype ==1){
txttype =2;
}
else
if (txttype ==2){
txttype =1;
}
}
Project 9
let img;
function preload() {
img = loadImage("https://i.imgur.com/CPxq6MI.jpg");
}
function setup() {
createCanvas(480, 480);
background(110, 16, 0);
img.resize(width, height);
img.loadPixels();
imageMode(CENTER);
//image(img, 240, 240);
frameRate(1000);
}
function draw() {
let x = floor(random(img.width)); //x position of pixels
let y = floor(random(img.height)) //y position of pixels
let shapePixel = img.get(x, y); //get pixels from photo
let textPixel = img.get(mouseX, mouseY); //get pixel from mouse location
//randomly generated pixels that fill up the page
let shapeR = random(15);
noStroke();
fill(shapePixel);
circle(x, y, shapeR);
//draw star shapes when mouse is pressed
if (mouseIsPressed) {
fill(textPixel);
textSize(random(5, 40));
text('★', mouseX, mouseY); //star shape follows mouse
}
}
//erases when double click
function doubleClicked() {
fill(110, 16, 0);
circle(mouseX, mouseY, 70);
}
This project is dedicated to celebrating my grandfather, who had just gotten his medal for 70 year anniversary since serving in the war. As you press your mouse across the screen stars will follow, and if you double click you can erase part of the drawing.
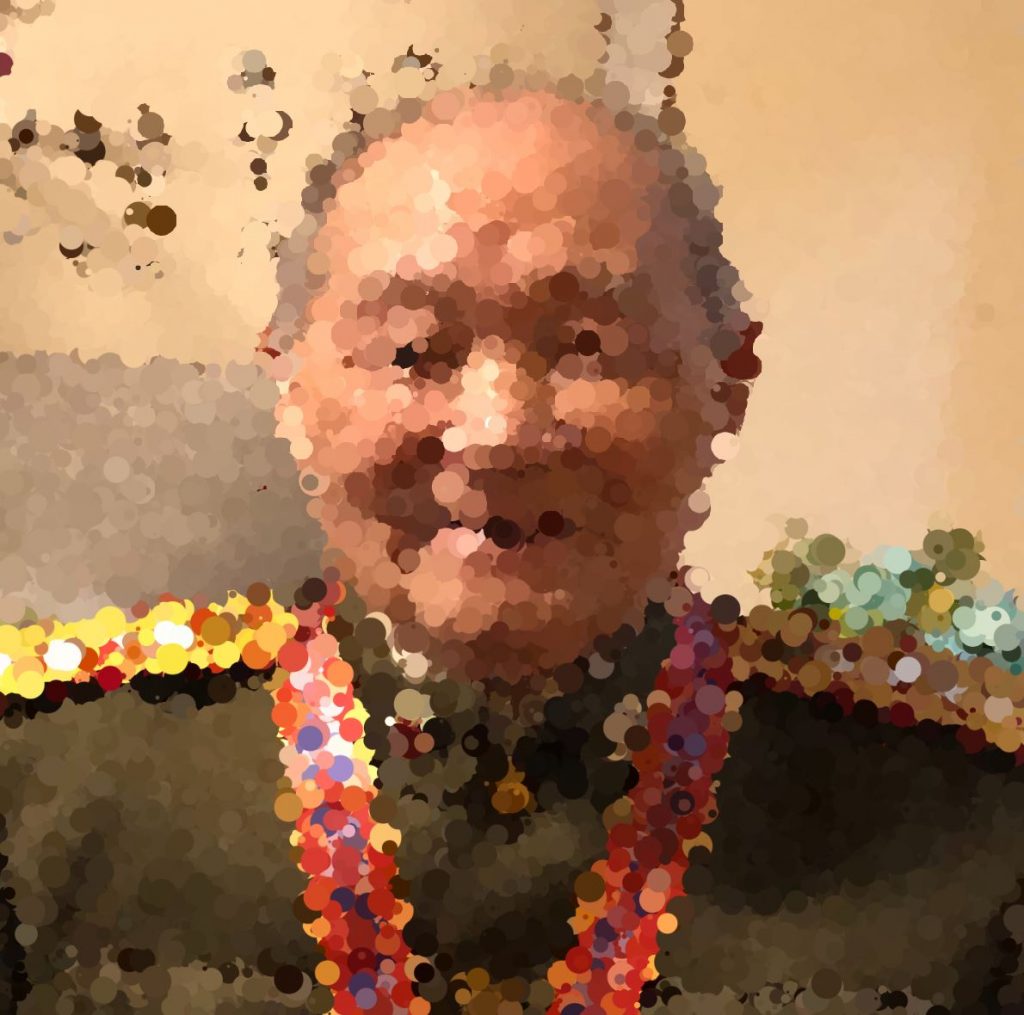
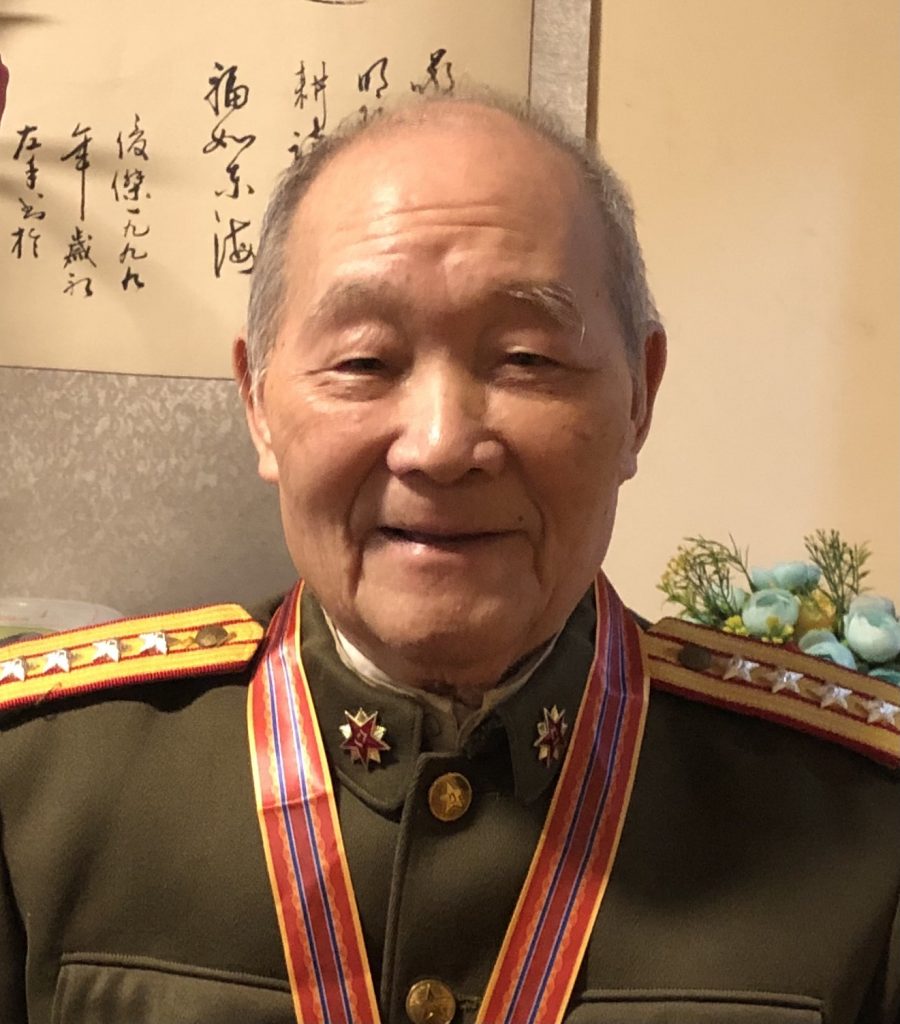
Project-09-Portrait
let foto;
function preload() {
foto = loadImage('https://i.imgur.com/3x32MS3.jpg?1'); //load image
tak = ["dickson"] //establish text
print(tak)
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
noStroke();
background(240);
foto.loadPixels();
}
function draw() {
let x = floor(random(foto.width)); //randomizing where text pops up
let y = floor(random(foto.height));
let pic = foto.get(x, y);
fill(pic, 128); //setting color to match the photo
textSize(mouseX/30) //adjustable text size
text(tak,x,y)
}
function mousePressed() {
filter(GRAY) //click to turn photo black and white
}
I thought it would be interesting to literally build myself with my name. This strongly resembles my identity through my name and my face. With each click, color is drawn away from the drawing to slowly resemble me as just a face and less of a person.
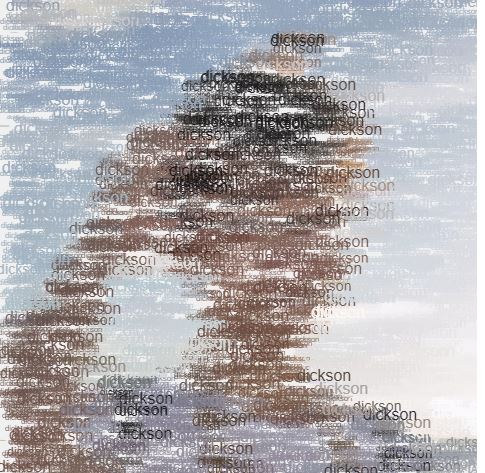
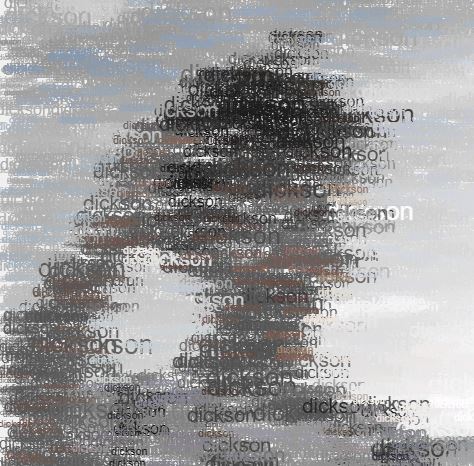
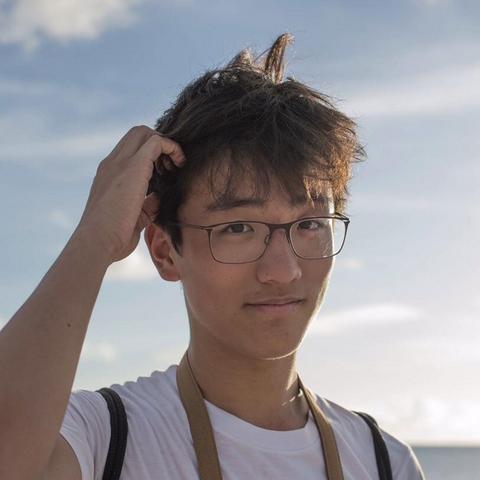
Project 09 – Computational Portrait (Custom Pixel)
I chose to use a selfie of me for this project. I resized the image so that my face would fit in the canvas, and then had words appearing to reveal the selfie. I used the following words : “annie”, “jean”, “flag”, “blue”, “brown”, “tan”, “red”, etc. I used these words because it described images in the actual picture, such as the background, me, and colors within the picture.
I had a little difficulty at first about trying to figure out another creative way to reveal the picture. However, from a previous LO post that I had made, I was inspired by an artist who used common words associated with each other on Google to graphically display data, therefore I chose the words that would be related to/associated with my selfie to appear as it seemed most reasonable.
//Annie Kim
//anniekim@andrew.cmu.edu
//Section B
//anniekim-09-Project
var img;
//words describing pic
var words = ["annie", "jean", "flag", "selfie", "snapchat", "blue", "red", "tan", "brown"]
function preload() {
var selfie = "https://i.imgur.com/AB85yVlb.jpg";
img = loadImage(selfie);
}
function setup() {
createCanvas(480, 480);
background(0);
img.loadPixels();
frameRate(1000);
}
function draw() {
//resizing selfie
img.resize(480, 480);
var x = random(width);
var y = random(height);
//getting exact pixel color to fill in words later
var pixelx = constrain(floor(x), 0, width);
var pixely = constrain(floor(y), 0, height);
var pixelcolor = img.get(pixelx, pixely);
noStroke();
fill(pixelcolor);
textSize(random(8, 18));
textFont('Helvetica');
text(words[Math.floor(random(0, 9))], x, y);
}
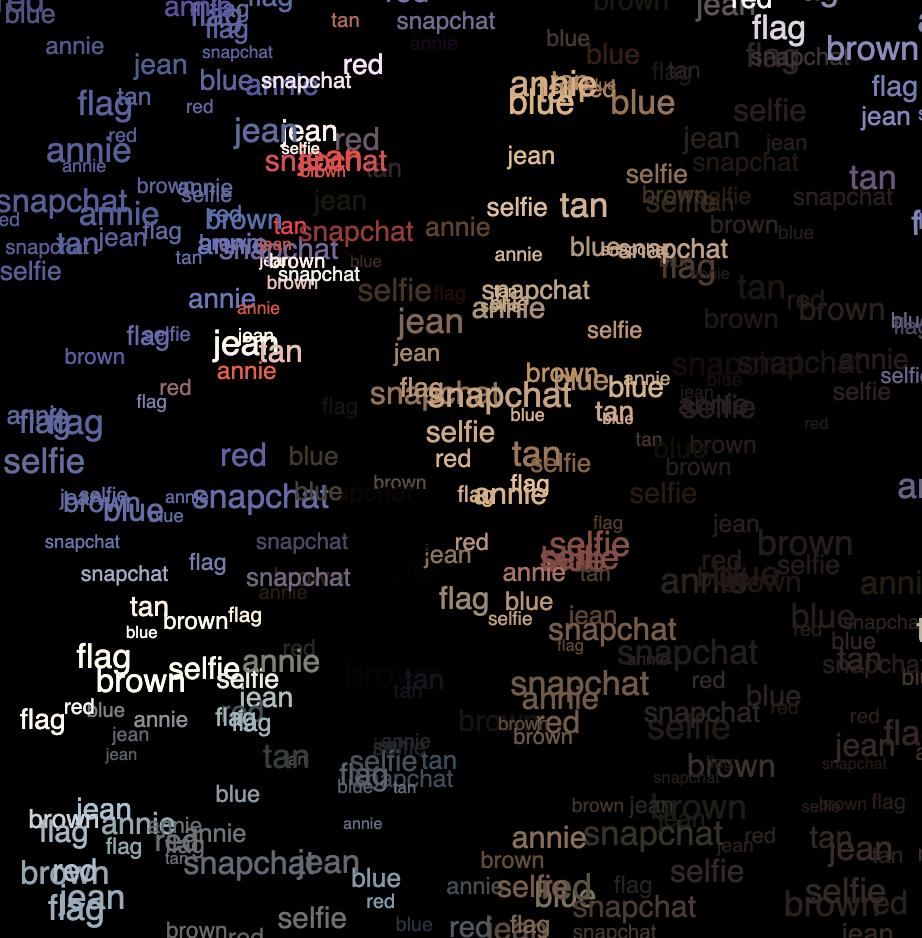
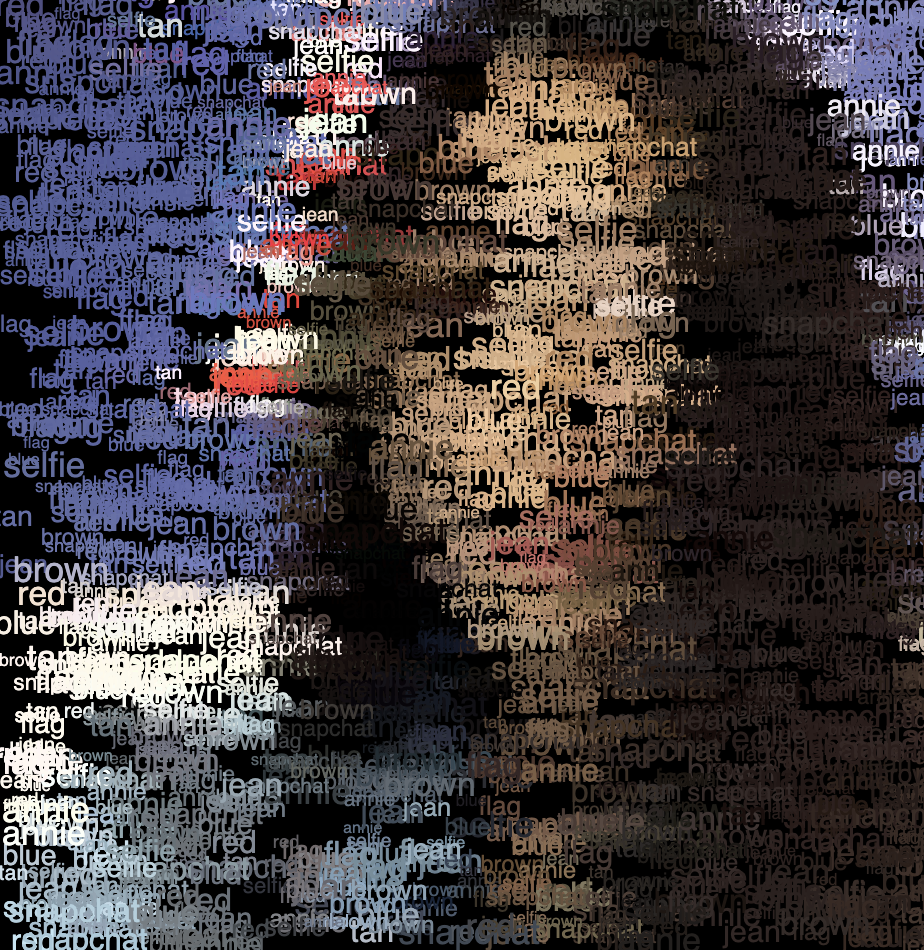
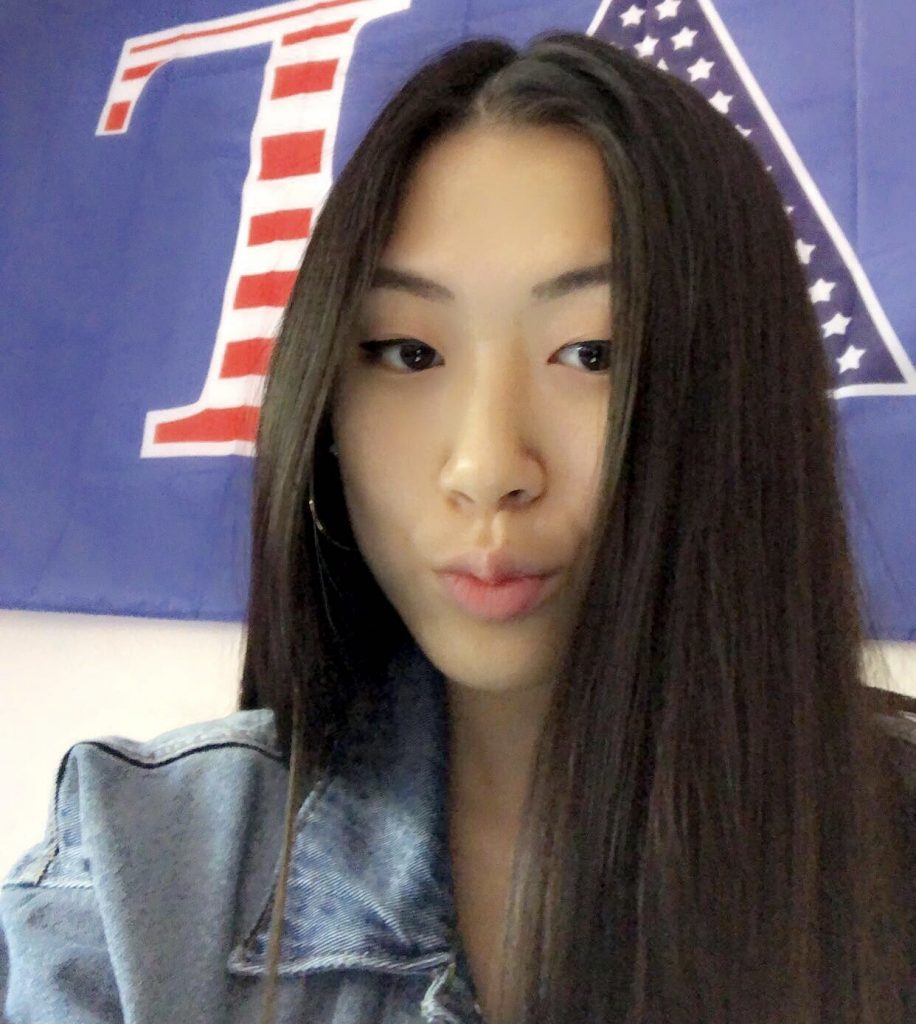
Project 9 – Computational Portrait (Custom Pixel)
Following the original image’s brick theme, I kept the project simple while trying to mimic the aesthetic of bricks/tiles.
var portrait;
function preload() {
portrait = loadImage("https://i.imgur.com/rlroaMx.jpg");
}
function setup() {
createCanvas(480, 320);
background(255);
portrait.loadPixels();
portrait.resize(width, height);
frameRate(30);
}
function draw() {
//image(portrait, 0, 0, width, height);
var x = random(width);
var y = random(height);
var porX = constrain(floor(x), 0, width);
var porY = constrain(floor(y), 0, height);
var colr = portrait.get(porX, porY);
stroke(255);
fill(colr);
rect(x, y, random(10, 25), random(10, 25));
}
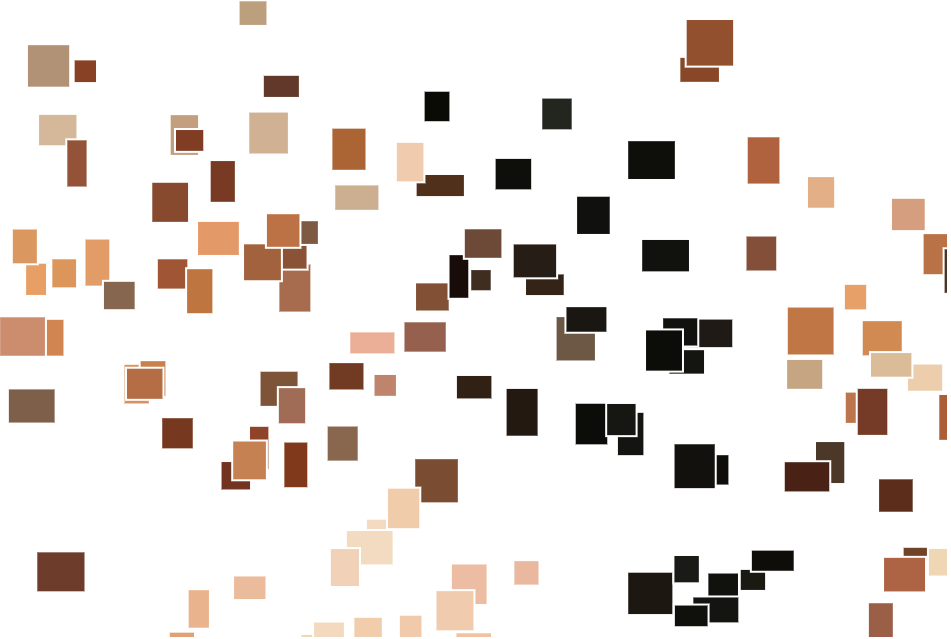
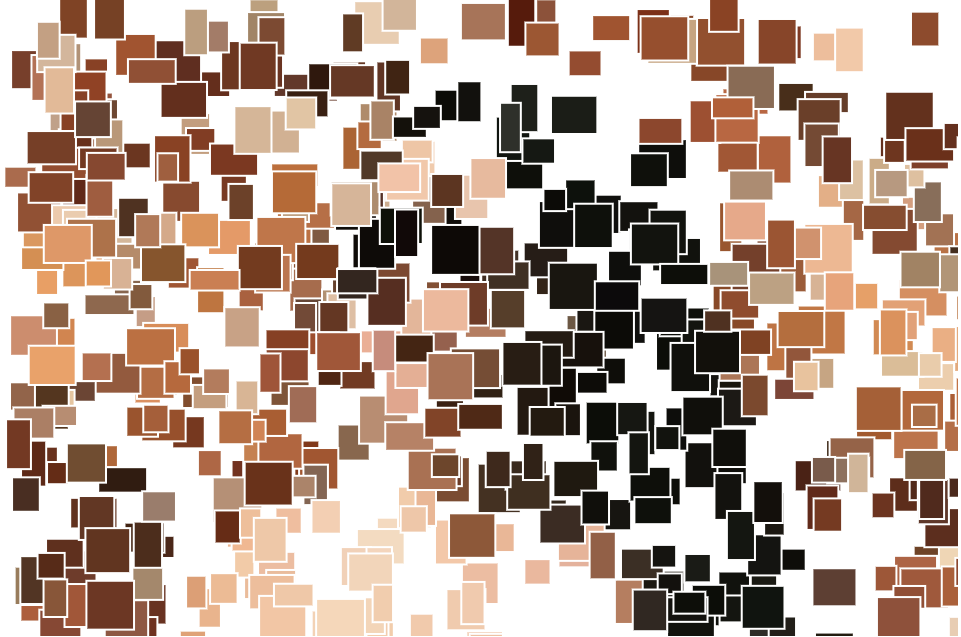
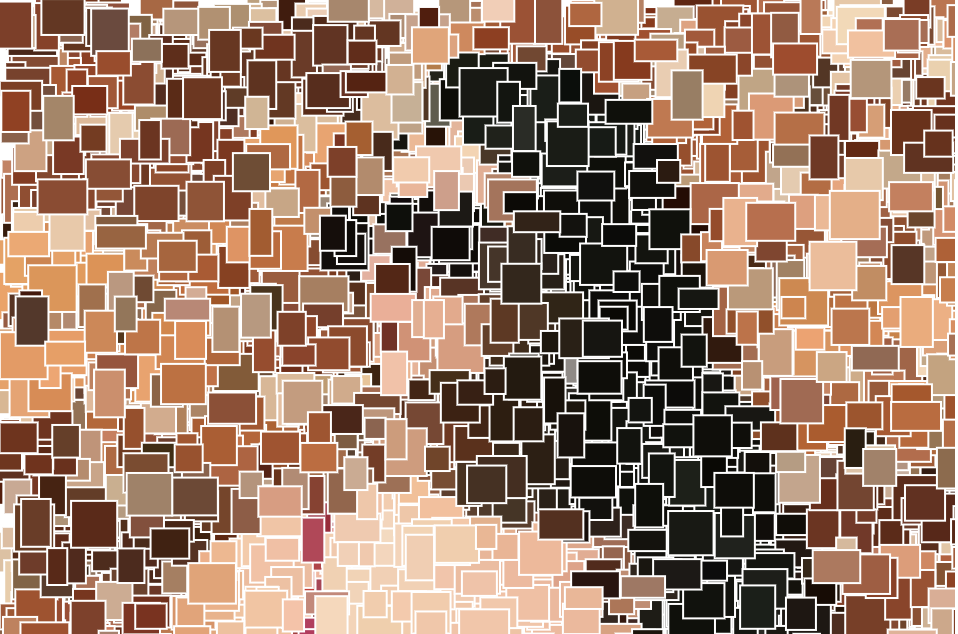
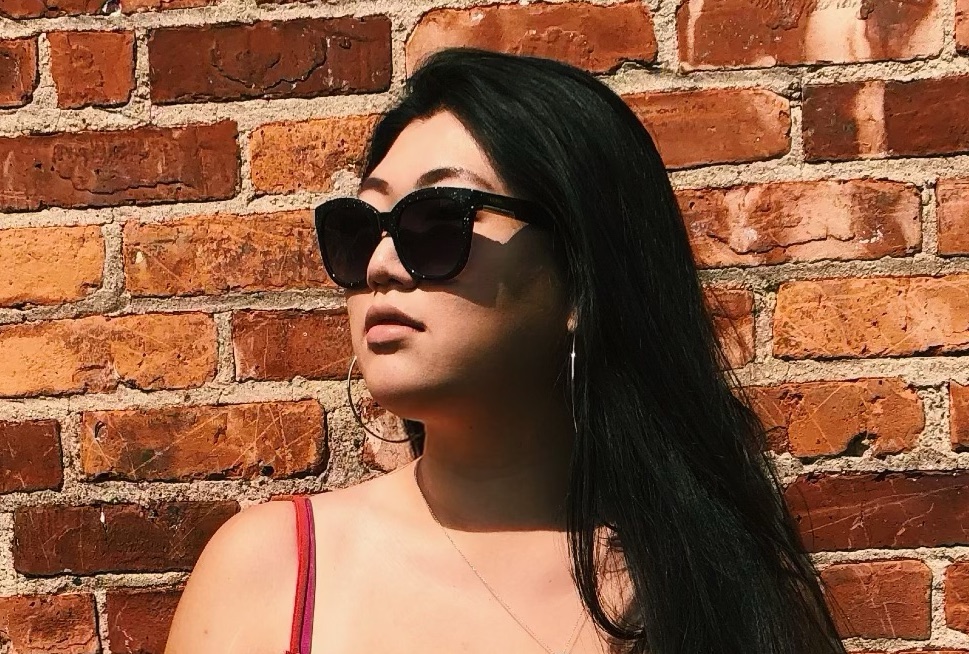
Project 09 – Portrait
let img;
let smallPoint, largePoint;
var angle = 0
function preload() {
img = loadImage("https://i.imgur.com/43WGeu8.jpg");
}
function setup() {
createCanvas(500,500);
imageMode(CENTER);
noStroke();
background(255);
img.loadPixels();
}
function draw() {
//retrive the image pixels in different sections to be able to assign specific
//pixel sizes for each section
let x = floor(random(img.width));
let y = floor(random(img.height/4));
let y1 = floor(random(img.height/2));
let y2 = floor(random(img.height/4*3));
let y3 = floor(random(img.height));
let pix = img.get(x, y);
let pix1 = img.get(x, y1);
let pix2 = img.get(x, y2);
let pix3 = img.get(x, y3);
//pixels are drawn where the mouse location is
let pixM = img.get(mouseX,mouseY);
//when the mouse is pressed, make the rectangles horizontally oriented,
//sizes change according to mouseY value
if (mouseIsPressed){
fill(pix3);
rectMode(CENTER)
rect(x,y3, 50, mouseY/50);
fill(pix2)
rect(x,y2, 25,mouseY/100)
fill(pix1)
rect(x,y1, 10, mouseY/150)
fill(pix)
rect(x,y, 5, mouseY/200)
fill(pixM)
rect(mouseX,mouseY,5,10)
}
//naturally, rectangles are vertical. They are larger at the top of the canvas
//and towards the bottom, get smaller in size to get fading away/gradient effect.
//get larger proportionally (across different sections) as mouseX increases
else{
fill(pix);
rectMode(CENTER)
rect(x,y, mouseX/50, 50);
fill(pix1)
rect(x,y1, mouseX/100,25)
fill(pix2)
rect(x,y2, mouseX/150,10)
fill(pix3)
rect(x,y3, mouseX/200,5)
fill(pixM)
rect(mouseX,mouseY,5,10)
}
}
//when the mouse is clicked, the colors invert
function mousePressed(){
filter(INVERT)
}
I had a lot of trouble with being able to manipulate the individual pixels – I wanted to make the pixels deliberately dynamic and varied, which I had trouble with because I couldn’t change them after they appeared. So, my solution was the divide up the image canvas – when I was using the “get pixels” function, I only retrieved the pixels for portions of the image. This way, I could depict those different portions with different pixels. I chose to use rectangles throughout, but of varying sizes across the different portions of the canvas in order to create the effect that the picture is fading or loading into existence. The sizes of the rectangles furthermore varies proportionally across the sections according to the mouseX location. When the mouse is pressed, the rectangles are oriented horizontally instead. I also added an invert filter that creates color interest when the mouse is clicked.
few seconds into render further into render mouse has been pressed mouse pressed and clicked image is re-coloring completed image
Project 09: Computational Portrait
This whole portrait is a picture of me sitting on a ledge outside of CFA, comprised of tinted covers of some of my favorite albums of music. It really took some experimentation to get the number of blocks correct, since with too many it would crash the program and with too few you couldn’t really notice the image. Tint() also wasn’t very happy with the original covers, so I had to edit them to make sure the colors would appear correctly. Finally, I added a random component to their generation just to add something interesting as they loaded in.
var pic;
var pixel = []
var covers = []
function preload(){ ///loads the album covers from the imgur posts
var coverFile = []
pic = loadImage("https://i.imgur.com/9if5OBK.jpg") ////some of my favorite albums to play on guitar and listen to
coverFile[0] = "https://i.imgur.com/vh9pN9D.png"
coverFile[1] = "https://i.imgur.com/ojxUjeG.png"
coverFile[2] = "https://i.imgur.com/udq9ixk.png"
coverFile[3] = "https://i.imgur.com/CgaJ3WV.png"
coverFile[4] = "https://i.imgur.com/JjaAoNi.png"
coverFile[5] = "https://i.imgur.com/BBocb2g.jpg"
coverFile[6] = "https://i.imgur.com/qx4C4pJ.jpg"
coverFile[7] = "https://i.imgur.com/hrqxmIj.jpg"
for (var e = 0; e < coverFile.length; e++) {
covers[e] = loadImage(coverFile[e]);
}
}
function drawPixel(){ ///draws and colors each album cover
tint(pic.get(this.x, this.y))
image(covers[this.selection], this.x, this.y, 20, 20)
}
function makePixel(pixSelect, pixX, pixY){ ///creates the individual pieces of the portrait
p = {x: pixX, y: pixY, selection: pixSelect,
drawFunction: drawPixel
}
return p
}
function setup(){ ///loads each pixel of the image, creates a square, and sends it to an array
createCanvas(480, 480)
frameRate(100)
pic.loadPixels()
noStroke()
for (i = 0; i <= 24; i++){
for (k = 0; k <= 24; k++){
var p = makePixel(round(random(0, 7)), i * 20, k * 20, )
pixel.push(p)
}
}
}
function draw(){ ///draws in squares randomly, up to 448, total number of the image
var p = pixel[round(random(0, 448))]
p.drawFunction()
}