var anxiety; //sound for computer anxiety
var sing; //sound for birds singing
var doorclose; //sound for door closing
var alarm; //sound for the clock
var squish; //sound for the virus
var drawer;
function preload() { //loading sounds
anxiety = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2021/11/Anxiety.wav");
sing = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2021/11/birds.wav");
doorclose = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2021/11/doorclose.m4a");
alarm = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2021/11/clock.wav");
squish = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2021/11/squish.wav")
drawer = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2021/11/drawer.wav")
}
function soundSetup() {
//setting the volume for each sound
anxiety.setVolume(1);
sing.setVolume(0.2);
doorclose.setVolume(1);
alarm.setVolume(1);
squish.setVolume(1);
drawer.setVolume(1);
}
var virus = []; //array of "germs"
var painted = []; //paint on the wall
var x = [];
var y = [];
var reliefBubbles = [];
var landscape = []; //array that creates hills
var rock = []; //array that creates rock
var trees = []; //trees on the close side of the road
var trees2 = []; //trees on the far side of the road
var cars = []; //cars on the road
var flocks = []; //flocks of birds
var bx = 180; //bird coordinates and
var by = 180;
var bdx = 0;
var bdy= 0;
var textX = 100; //the scrolling text on the TV
var germShot = -1; //which germ has been "shot" with the syringe
var r = 40; //radius of clock
var theta = 0; // angle of rotation (starting) for clock
var c = 10; //to move the chart
var count = -10; //to control where the germs "stop" on the screen
var EamonnX = 100; //The x location of me as I walk
var opacity = 255;
var size = 10;
var noiseParam = 0;
var noiseStep = 0.03; //extremity of the slopes
function setup() {
createCanvas(400,300);
background(220);
frameRate(5)
noStroke();
fill(136,238,143); //first scene background
rect(0,0,400,height);
t1 = makeTurtle(380,200); //constructing turtles for the computer anxiety
t2 = makeTurtle(380,200);
t3 = makeTurtle(380,200);
t4 = makeTurtle(380,200);
t5 = makeTurtle(380,200);
//setting the color and weight
t1.lowerPen();
t1.setColor(color(0));
t1.setWeight(random(1,3));
t2.lowerPen();
t2.setColor(color(0));
t2.setWeight(random(1,3));
t3.lowerPen();
t3.setColor(color(0));
t3.setWeight(random(1,3));
t4.lowerPen();
t4.setColor(color(0));
t4.setWeight(random(1,3));
t5.lowerPen();
t5.setColor(color(0));
t5.setWeight(random(1,3));
noStroke();
for(var i=0;i<=width/5;i++){ //creating hills
var n= noise(noiseParam); //picks value 0-1
var value = map(n,0,1,150,250); //scales it to the canvas size
landscape.push(value); //adds to array
noiseParam+=noiseStep; //moves to the right for the next point in the array
}
for(var i=0;i<=width/5;i++){ //creating rocks
var n2= noise(noiseParam); //picks value 0-1
var value2 = map(n2,0,1,250,300); //scales it to the canvas size
rock.push(value2); //adds to array
noiseParam+=noiseStep; //moves to the right for the next point in the array
}
//plotting the starting objects
for (var i = 0; i < 30; i++){
var Tx = random(width);
var Ty = random(280,height);
trees[i] = makeTree(Tx,Ty);
}
for (var i = 0; i < 30; i++){
var Tx2 = random(width);
var Ty2 = random(240,260);
trees2[i] = makeTree(Tx2,Ty2);
}
for (var i = 0; i < 2; i++){
var Fx = random(width);
var Fy = random(0,200);
flocks[i] = makeFlock(Fx,Fy);
}
for (var i = 0; i < 10; i++){
var Cx = random(width);
cars[i] = makeCar(Cx);
}
useSound();
}
function draw() {
//storyboard for the animation
if(frameCount > 0 & frameCount<=100){
openingScene();
}
if(frameCount>100 & frameCount<=200){
anxiety.stop();
sceneTwo();
}
if(frameCount==100){
squish.play();
}
if(frameCount>200 & frameCount<=380){
sceneThree();
squish.stop();
}
if(frameCount==200){
alarm.play();
}
if(frameCount>380){
alarm.stop();
sing.play();
finalScene();
}
if(frameCount==380){
sing.play();
}
if(frameCount>1000){
sing.stop();
}
}
function openingScene(){
Window(100,50); //drawing the room
bed(0,200);
chair(270,190);
laptop(350,210);
Eamonn(295,160);
if(frameCount>20){ //initializing the computer anxiety
push();
computerAnxiety();
pop();
}
if(frameCount==20){
anxiety.play();
}
desk(340,210);
bird();
fill(255); //white
rect(147.5,50,5,150); //frames
rect(100,122.5,100,5);
}
//functions for the first scene
function Window(x,y){
push();
translate(x,y);
fill(155,245,245); //light blue
rect(0,0,100,150); //glass
fill(255); //white
rect(47.5,0,5,150); //frames
rect(0,72.5,100,5);
pop();
}
function bed(x,y){
push();
translate(x,y);
fill(10,95,193); //dark blue
rect(0,0,100,height,10); //beds
rect(200,0,200,height,10);
pop();
}
function chair(x,y){
push();
translate(x,y);
fill(255); //white
rect(0,0,8,110); //chair
rect(0,55,55,8);
rect(55,55,8,60);
pop();
}
function desk(x,y){
push();
translate(x,y);
fill(236,188,75); //yellow-brown
rect(0,0,75,100);
fill(131,92,3); //dark brown
rect(-227,0,75,100)
fill(166,123,21); //light brown
rect(-220,10,60,30);
fill(131,92,3); //dark brown
ellipse(-190,25,10,10); //knob
if(mouseX>110 & mouseX<180 && mouseY>220 && mouseY<250 && mouseIsPressed){ //if mouse is pressed on drawer, open it
fill(0); //black
rect(-217,10,54,30); //inside of drawer
fill(166,123,21);
rect(-220,20,60,30);
fill(131,92,3);
ellipse(-190,35,10,10); //knob
}
pop();
}
function laptop(x,y){
push();
translate(x,y);
fill(150); //gray
rect(1,-2,30,3); //laptop keyboard
quad(30,0,30,3,43,-20,41,-23); //screen
pop();
}
function Eamonn(x,y){
push();
translate(x,y);
fill(0); //black
rect(-10,65,65,20,10); //legs
rect(35,65,20,75,10);
fill(186); //gray
rect(32,130,30,10,10); //shoe
fill(255,220,200); //beige
ellipse(2,0,29); //head
rect(-2,0,8,20); //neck
fill(242,201,69); //golden brown
ellipse(-6,0,13,27); //hair
ellipse(4,-10,27,10);
fill(176,58,58); //maroon
rect(-13,20,30,60,15); //shirt
fill(176); //gray
push();
rotate(radians(-80)); //reaching out to laptop
rect(-40,5,15,50,15); //arm
pop();
fill(255,220,200); //beige
ellipse(47,41,15); //head
pop();
}
function bird(){
push();
if(mouseX>90 &mouseX<190 &&mouseY>50 &&mouseY<190){ //is mouse is on window, show bird
bdx = mouseX-bx; //"easing" the bird
bdy= mouseY-by;
bx = bx + 0.1*bdx;
by = by + 0.1*bdy;
fill(138,102,18); //dark brown
triangle(bx,by-5,bx,by+5,bx+20,by+random(-5,5)); //wings
triangle(bx,by-5,bx,by+5,bx-20,by+random(-5,5));
fill(213,99,12); //red brown
triangle(bx+1,by-13,bx+1,by-8,bx+11,by-10); //beak
fill(182,139,54); //dark brown
ellipse(bx,by,12,15); //head
fill(138,102,18); //light brown
ellipse(bx,by-10,10,10);
}
pop();
}
function computerAnxiety(){
var EamonnHeadX = 295; //coordinates of my head
var EamonnHeadY = 160;
t1.forward(random(1,3)); //move at varying speeds
t2.forward(random(1,3));
t3.forward(random(1,3));
t4.forward(random(1,3));
t5.forward(random(1,3));
t1.turnToward(EamonnHeadX, EamonnHeadY, 80); //move toward my head
t2.turnToward(EamonnHeadX, EamonnHeadY, 20);
t3.turnToward(EamonnHeadX, EamonnHeadY, 50);
t4.turnToward(EamonnHeadX, EamonnHeadY, 60);
t5.turnToward(EamonnHeadX, EamonnHeadY, 70);
t1.left(random(-1, 1)); //to make the movement erratic
t2.left(random(-1, 1));
t3.left(random(-1, 1));
t4.left(random(-1, 1));
t5.left(random(-1, 1));
}
function sceneTwo(){
fill(255,200,104); //orange
rect(0,0,width,height); //background
Television(70,50);
fill(161,105,9); //brown
rect(45,235,300,100); //desk
chart(135,65);
TVAnxiety();
syringe();
if (mouseIsPressed & (germShot > -1)) { //if mouse is pressed on germ, remove it from array
virus.splice(germShot,1);
}
}
function Television(x,y){
push();
translate(x,y);
stroke(0);
strokeWeight(10);
fill(166,250,255); //light blue
rect(0,0,250,150); //screen
rect(115,150,10,20); //stand (stroke color)
rect(25,175,200,5); //stand
fill(252,49,49); //red
noStroke();
rect(5,115,240,15); //news banner
textSize(10);
fill(255); //white
text("CORONAVIRUS RISING IN US",textX,127);
textX-=5; //move text
if(textX<5){ //reset text if reaches end of TV
textX = 100;
}
pop();
}
function chart(x,y){
push();
translate(x,y);
fill(255); //white
strokeWeight(2);
stroke(0);
rect(0,0,120,90); //chart background
line(10,20,10,80); //axis
line(10,80,100,80);
fill(255,0,0); //red
textSize(20);
text("CASES", 30,20);
strokeWeight(5);
var ttl = makeTurtle(12,78); //create a turtle
ttl.setWeight(5);
ttl.setColor(color(255,0,0)); //red
ttl.left(45); //making graph line
ttl.forward(30);
ttl.right(90);
ttl.forward(15);
ttl.left(90);
ttl.forward(c);
c+=2 //chart is going up
pop();
}
function TVAnxiety(){
if(frameCount % 4 == 0){ //every 4 frames, make new germ
virus.push(makeGerm(200,150));
}
for(var i=0;i<virus.length;i++){ //move and show germs
virus[i].show();
virus[i].move();
}
count+=1;
}
function makeGerm(startX,startY){ //germ constructor
var germ = {x: startX,
y:startY,
dx: random(-10,10), //speeds
dy:random(-10,10),
breadth:random(20,40), //width of germ
color:color(0),
moving:true,
show: showGerm,
move: moveGerm
}
return germ;
}
function showGerm(){ //germ drawing
push();
fill(6,158,31); //dark green
ellipse(this.x,this.y,this.breadth,this.breadth); //germ body
var t=makeTurtle(this.x,this.y) //make a turtle for each germ
t.setWeight(5);
t.setColor(color(6,158,31)); //dark green
for(var i=0;i<7;i++){ //creating the "spikes"
t.forward(this.breadth-5); //one spike
t.raisePen();
t.back(this.breadth-5); //going back to center, turning
t.right(60);
t.lowerPen();
}
pop();
}
function moveGerm(){
if(this.moving==true){ //move germ diagonally
this.x += this.dx;
this.y += this.dy;
}
if(this.x>width - count || this.x<0+count || this.y>height-count || this.y<0+count){ //if it hits a boundary, defined by a count
this.moving=false;
}
}
function syringe(){
push();
rectMode(CENTER);
translate(mouseX,mouseY);
rotate(radians(45));
fill(220); //light gray
rect(0,0,20,50); //bottle
rectMode(CORNER);
rect(-5,25,10,5); //cap
rect(-10,25,20,2);
fill(247,131,236); //pink
rect(-10,0,20,25); //syringe liquid
strokeWeight(2);
stroke(220); //gray
line(0,-25,0,-50); //needle
pop();
}
function sceneThree(){
fill(250,240,191); //beige
rect(0,0,width,height); //background
paint();
clock(300,100);
fill(164,115,1); //brown
rect(0,250,width,height); //floor
fill(245,217,152); //white-beige
rect(width-20,0,20,height); //wall
table(180,160);
fill(121,61,2); //dark brown
rect(375,115,5,155); //door(closed)
push();
frameRate(20)
if(frameCount<=320){ //animating me walking
if(frameCount%4 ==0){ //alternating animations
EamonnWalk(EamonnX,130);
}else{
EamonnStand(EamonnX,130);
}
EamonnX+=2 //moving me
}
if(frameCount>=320 & frameCount<340){ //reaching out to the door
arm();
EamonnStand(335,130);
}
if(frameCount>330 & frameCount<360){
fill(121,61,2); //dark brown
rect(315,115,60,155); //door(open)
fill(232,219,108); //gold
ellipse(325,200,9,9); //knob
}
if(frameCount==310){
doorclose.play();
}
pop();
}
//functions for scene three
function EamonnStand(x,y){
push();
translate(x,y);
fill(255,220,200); //beige
ellipse(0,0,29); //head
rect(-4,0,8,20); //neck
fill(242,201,69); //golden brown
ellipse(-6,0,13,27); //hair
ellipse(4,-10,27,10);
fill(176,58,58); //maroon
rect(-13,20,25,60,15); //shirt
fill(0); //black
quad(-13,70,12,70,9,130,-10,130); //leg
fill(176); //gray
rect(-8,20,15,50,15); //arm
fill(255,220,200); //beige
ellipse(-1,70,15); //hand
fill(186); //gray
rect(-13,130,30,10,10); //shoe
pop();
}
function EamonnWalk(x,y){
push();
translate(x,y);
fill(255,220,200);
ellipse(0,0,29);
rect(-4,0,8,20);
fill(242,201,69);
ellipse(-6,0,13,27);
ellipse(4,-10,27,10);
fill(176,58,58);
rect(-13,20,25,60,15);
fill(0);
quad(-13,70,12,70,-5,130,-24,130); //legs
quad(-13,70,12,70,25,130,5,130);
fill(176)
rect(-8,20,15,50,15);
fill(255,220,200)
ellipse(-1,70,15)
fill(186,186,186);
rotate(radians(5)); //rotated shoes
rect(-15,130,30,10,10);
rotate(radians(-10));
rect(-7,130,30,10,10);
pop();
}
function arm(){
push();
fill(176);
rotate(radians(-35));
rect(180,320,15,50,15); //arm reaching out to door
pop();
fill(255,220,200); //beige
ellipse(366,196,15); //hand
}
function clock(x,y){
push();
translate(x,y);
var shake = random(-3,3); //to shake the clock
fill(0);
ellipse(0+shake,0+shake,50,50); //clock
fill(255);
ellipse(0+shake,0+shake,r,r);
frameRate(20);
var clockX = r/2 * cos(radians(theta));
var clockY = r/2 * sin(radians(theta));
stroke(0);
line(0+shake, 0+shake, clockX, clockY); //clock hand
theta +=10; //turn the hand
pop();
}
function table(x,y){
push();
translate(x,y);
fill(121,61,2); //brown
rect(0,0,60,70); //body
rect(0,70,10,30); //legs
rect(50,70,10,30);
pop();
}
function paint(){ //draw function for paint object
push();
x.push(mouseX); //draw a line to where the mouse is
y.push(mouseY);
for (var i=0; i < x.length-1; i++) {
strokeWeight(map(mouseY,0,height,0,10)); //chamge stroke weight with y position
stroke(color(random(255),random(255),random(255))); //change color randomly
line(x[i],y[i],x[i+1],y[i+1]); //draw line from one position to next (mouse positions)
}
pop();
}
//functions for the final scene
function finalScene(){
sunset(2);
fill(255,252,229); //white-yellow
ellipse(200,200,50,50); //sun
hills();
fill(189); //gray
rect(0,260,width,20); //road
removeFlock();
newFlock();
removeCar();
newCar();
//showing and moving objects
for(var i=0;i<trees2.length;i++){
trees2[i].show();
}
for(var i=0;i<cars.length;i++){
cars[i].show();
cars[i].move();
}
for(var i=0;i<trees.length;i++){
trees[i].show();
}
for(var i=0;i<flocks.length;i++){
flocks[i].show();
flocks[i].move();
}
fill(240,221,159,60); //opaque orange
rect(0,0,width,height); //create haze
rocks();
relief();
Eamonn3(260,200);
textSize(20);
fill(0,0,255);
text("THE END",300,100);
}
function sunset(size) {
var green=215;
var blue=238;
var red=141
for (var y = size/2; y < height + 50; y += size) { //nested loop for grid
for (var x = size/2; x < width + 50; x += size) {
fill(red,green,blue);
rect(x, y, size, size);
}
red += (200/(width/size)); //create gradient
green +=(100/(height/size));
blue -=(50/(height/size));
}
}
function makeTree(startX,startY){ //tree constructor
var tree = {x: startX,
y:startY,
breadth:5,
color: color(random(130,200),random(100,150),0),
show: showTree
}
return tree;
}
function showTree(){
push();
translate(this.x, this.y); //appear at width,280
fill(121,90,5); //gray
rect(0,-20,this.breadth,20); //draw railing markers
fill(this.color);
ellipse(2,-30,20,20); //leaves
pop();
}
function removeCar(){ //remove car from array once out of sight
var carsToKeep = [];
for (var i = 0; i < cars.length; i++){
if (cars[i].x > 0) {
carsToKeep.push(cars[i]);
}
}
cars = carsToKeep;
}
function newCar() { //create a new car at a given probability
var newCarChance = 0.05;
if (random(0,1) < newCarChance) {
cars.push(makeCar(width)); //create new car at the edge
}
}
function moveCar(){
this.x += this.speed; //mpve cars
}
function showCar(){
push();
noStroke();
translate(this.x, 260);
fill(this.color)
rect(0,-7,this.breadth,this.height); //body of car
if(this.speed>0){
rect(25,0,10,10); //car facing right
}else{
rect(-10,0,10,10); //car facing left
}
fill(0); //black
ellipse(5,10,10,10); //wheels
ellipse(25,10,10,10);
pop();
}
function makeCar(startX){
var car = {x: startX,
breadth:25,
height:random(15,20),
speed: random(-5,5),
color: color(random(0,255),random(0,255),random(0,255)),
move: moveCar,
show: showCar
}
return car;
}
function moveFlock(){
this.x += this.speed; //move horizontally
this.y -= this.speed/2;
}
function removeFlock(){
var flocksToKeep = [];
for (var i = 0; i < flocks.length; i++){
if (flocks[i].x > 0) {
flocksToKeep.push(flocks[i]);
}
}
flocks = flocksToKeep;
}
function newFlock() {
var newFlockChance = 0.01;
if (random(0,1) < newFlockChance) {
flocks.push(makeFlock(0,random(0,200)));
}
}
function showFlock(){
push();
stroke(0);
translate(this.x,this.y);
for(var i=0;i<this.numBirds;i++){
line(0+(2*i)+random(-1,1),0+(2*i),5+(2*i),5+(2*i)); //wings of birds
line(5+(2*i),5+(2*i),10+(2*i),0+(2*i)+random(-1,1));
}
pop();
}
function makeFlock(startX,startY){
var flock = {x: startX,
y: startY,
numBirds: random(3,7),
speed:2,
move: moveFlock,
show: showFlock
}
return flock;
}
function hills(){
beginShape();
noStroke();
fill(60,131,67); //green
for (i=0;i<width/5; i++){
curveVertex(i*5,landscape[i]) //creates slopes by connecting two points
curveVertex((i+1)*5,landscape[i+1]);
}
curveVertex(width,height); //stable values that create the green fill
curveVertex(0,height);
endShape(CLOSE); //end shape and fill it
}
function rocks(){
beginShape();
noStroke();
fill(129); //gray
for (i=0;i<width/5; i++){
curveVertex(i*5,rock[i]) //creates slopes by connecting two points
curveVertex((i+1)*5,rock[i+1]);
}
curveVertex(width,height); //stable values that create the gray fill
curveVertex(0,height);
endShape(CLOSE); //end shape and fill it
}
function relief(){
if(frameCount % 10 == 0){ //every 4 frames, make new Bubble
reliefBubbles.push(makeBubble(260,200));
}
for(var i=0;i<reliefBubbles.length;i++){ //move and show Bubbles
reliefBubbles[i].show();
reliefBubbles[i].move();
}
}
function makeBubble(startX,startY){ //bubble constructor
var bubble = {x: startX,
y:startY,
opacity:255,
size:10,
show: showBubble,
move: moveBubble
}
return bubble;
}
function showBubble(){
push();
fill(220,this.opacity); //light gray
ellipse(this.x,this.y,this.size,this.size); //germ body
pop();
}
function moveBubble(){
this.size+=1; //grow bubbles
this.opacity-=3; //fade
}
function Eamonn3(x,y){
push();
translate(x,y);
fill(255,220,200); //beige
ellipse(0,0,40,40); //head
rect(-8,12,15,15); //neck
fill(242,201,69); //golden brown
ellipse(0,-2,38,35); //hair
push();
fill(0);
rotate(radians(50)); //legs in criss cross
rect(63,0,25,70,15);
rotate(radians(-100));
rect(-87,0,25,70,15)
pop();
fill(176,58,58);
rect(-23,25,45,70,15);
pop();
}
function mousePressed(){
if(frameCount<100){ //play drawer sound if mouse is pressed on drawer
if(mouseX>110 & mouseX<180 && mouseY>220 && mouseY<250){
drawer.play();
}
}
germShot = -1;
for (var i = 0; i < virus.length; i++) {
var d = dist(mouseX, mouseY,
virus[i].x, virus[i].y)
if (d < virus[i].breadth) { //if clicked within a given germ
germShot = i; //pass this index to the splice function
}
}
}
//turtle code, courtesy of Tom Cortina
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
lowerPen: turtleLowerPen, raisePen: turtleRaisePen,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtleLowerPen() {
this.penDown = true;
}
function turtleRaisePen() {
this.penDown = false;
}
function turtleGoTo(newx, newy) {
if (this.penDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, newx, newy);
}
this.x = newx;
this.y = newy;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
this.angle %= 360;
}
Category: Uncategorized
Generative Beach Landscape
When I saw the prompt for the project this week, I immediately thought of doing something with a noise function. I felt as though mountains wouldn’t allow for enough variability, so I decided to do a beach. I laid out the very basic sand, water, and sky, and then added embellishing elements to try to create a drive by beach scene.
var boats = []; //arrays to hold respective objects
var clouds = [];
var umbrellas = [];
var planes = [];
var buildings = [];
var railings = [];
var people = [];
var towels = [];
var landscape = []; //array that creates slope of shoreline
var noiseParam = 0;
var noiseStep = 0.005; //defines extremity of shoreline slope
function setup() {
createCanvas(480,300);
for (var i = 0; i < 3; i++){ //make a certain amount of (object) to start out, at random locations within a constraint
var rx = random(width);
var ry = random(80,150)
boats[i] = makeBoat(rx,ry);
}
for (var i = 0; i < 3; i++){
var Cx = random(width);
var Cy = random(0,60)
clouds[i] = makeCloud(Cx,Cy);
}
for (var i = 0; i < people.length; i++){
var Tx = random(width);
var Ty = random(250,300)
towels[i] = makeTowel(Tx,Ty);
}
for (var i = 0; i < people.length; i++){
var Px = random(width);
var Py = random(250,300)
people[i] = makePerson(Px,Py);
}
for (var i = 0; i < 5; i++){
var Ux = random(width);
var Uy = random(250,300)
umbrellas[i] = makeUmbrella(Ux,Uy);
}
for (var i = 0; i < 15; i++){
var Bx = random(width);
buildings[i] = makeBuilding(Bx);
}
for (var i = 0; i < 0.9; i++){
var Px = random(width);
var Py = random(0,20)
planes[i] = makePlane(Px,Py);
}
for(var i = 0;i <= width / 5;i++){
var n = noise(noiseParam); //picks value 0-1
var value = map(n,0,1,50,height); //scales it to the canvas size
landscape.push(value); //adds to array
noiseParam += noiseStep; //moves to the right for the next point in the array
}
frameRate(5);
}
function draw() {
background(3,220,245);
fill(13,66,212,230); //dark blue
rect(0,height / 4,width,height); //body of water
fill(227,232,164,175); //pale beige
rect(0,height / 4-7,width,7); //distant shoreline
//call the functions to spawn and despawn respective obejcts
removeBoat();
newBoat();
removeCloud();
newCloud();
removeUmbrella();
removePlane();
newPlane();
removeBuilding();
newBuilding();
removeRailing();
newRailing();
removePerson();
removeTowel();
//call the move and show functions to continually draw and redraw objects after updated coordinates
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].show();
}
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].show();
}
for (var i = 0; i < boats.length; i++){
boats[i].move();
boats[i].show();
}
displayHorizon();
for (var i = 0; i < towels.length; i++){
towels[i].move();
towels[i].show();
}
for (var i = 0; i < people.length; i++){
people[i].move();
people[i].show();
}
for (var i = 0; i < umbrellas.length; i++){
umbrellas[i].move();
umbrellas[i].show();
}
for (var i = 0; i < planes.length; i++){
planes[i].move();
planes[i].show();
}
fill(159,159,159);
rect(0,height-50,width,40);
fill(110,110,110);
rect(0,height-45,width,30);
for (var i = 0; i < railings.length; i++){
railings[i].move();
railings[i].show();
}
}
//functions to remove an object from a displayed array once "out of sight"
function removeBoat(){
var boatsToKeep = []; //the boats that should still be displayed
for (var i = 0; i < boats.length; i++){
if (boats[i].x + boats[i].breadth > 0) { //if the boat is closer than it's width to the edge
boatsToKeep.push(boats[i]); //add this boat to the boats that will be displayed
}
}
boats = boatsToKeep; // only display the baots currently being kept
}
function removeCloud(){
var cloudsToKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].breadth > 0) {
cloudsToKeep.push(clouds[i]);
}
}
clouds = cloudsToKeep;
}
function removeUmbrella(){
var umbrellasToKeep = [];
for (var i = 0; i < umbrellas.length; i++){
if (umbrellas[i].x + umbrellas[i].breadth > 0) {
umbrellasToKeep.push(umbrellas[i]);
}
}
umbrellas = umbrellasToKeep;
}
function removePlane(){
var planesToKeep = [];
for (var i = 0; i < planes.length; i++){
if (planes[i].x + 150 > 0) {
planesToKeep.push(planes[i]);
}
}
planes = planesToKeep;
}
function removeBuilding(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep;
}
function removeRailing(){
var railingsToKeep = [];
for (var i = 0; i < railings.length; i++){
if (railings[i].x > 0) {
railingsToKeep.push(railings[i]);
}
}
railings = railingsToKeep;
}
function removePerson(){
var peopleToKeep = [];
for (var i = 0; i < people.length; i++){
if (people[i].x > 0) {
peopleToKeep.push(people[i]);
}
}
people = peopleToKeep;
}
function removeTowel(){
var towelsToKeep = [];
for (var i = 0; i < towels.length; i++){
if (towels[i].x > 0) {
towelsToKeep.push(towels[i]);
}
}
towels = towelsToKeep;
}
//functions to create new objects that come into sight
function newBoat() {
var newBoatChance = 0.009; //the chance that a new boat will appear
if (random(0,1) < newBoatChance) { //activate probability
boats.push(makeBoat(width,random(100,150))); //add a new boat if the porbability condition is met
}
}
function newCloud() {
var newCloudChance = 0.001;
if (random(0,1) < newCloudChance) {
clouds.push(makeCloud(width,random(0,60)));
}
}
function newPlane() {
var newPlaneChance = 0.003;
if (random(0,1) < newPlaneChance) {
planes.push(makePlane(width, random(0,50)));
}
}
function newBuilding() {
var newBuildingChance = 0.1;
if (random(0,1) < newBuildingChance) {
buildings.push(makeBuilding(width));
}
}
function newRailing() {
var newRailingChance = 0.8;
if (frameCount % 2 == 0 & random(0,1) < newRailingChance) { //spawn every two frames, if probability condition is met
railings.push(makeRailing(width));
}
}
//functions to move the objects across the screen
function moveBoat() {
this.x += this.speed; //add a speed value to the the x position to chance it every frame
}
function moveCloud(){
this.x += this.speed;
}
function moveUmbrella(){
this.x += this.speed;
}
function movePlane(){
this.x += this.speed;
}
function moveBuilding(){
this.x += this.speed;
}
function moveRailing(){
this.x += this.speed;
}
function movePerson(){
this.x += this.Xspeed;
this.y += this.Yspeed/3; //move very slightly in Y direction
}
function moveTowel(){
this.x += this.speed;
}
//functions to show (object) by drawing it at the requested coordinates
function showBoat() {
var floorHeight = 10;
push();
translate(this.x, this.y); //plot the drawing at the the coordinates given
noStroke();
for (var i = 0; i < this.nFloors; i++) {
fill(this.color,this.y*2); //random color, with varying opacity based on y value (distance from viewer)
rect(0, -15 - (i * floorHeight), this.breadth-10*i, 10); //the boat levels
if(this.nFloors == 3){
fill(255,this.y*2); //white, varying opacity
rect(3,-15-(i*floorHeight), this.breadth-12*i,7) //to make a "yacht"
}
if(this.nFloors == 2){
fill(161,227,238,this.y*2); //light blue, varying opacity
rect(5,-13-(floorHeight),this.windowBreadth,7); //window
}
if(this.nFloors == 1){
strokeWeight(5);
stroke(this.color,this.y*2); //rnadom color of boat, varying opacity
line(7,0-(floorHeight),-6,-15-(floorHeight)); //fishing gig
strokeWeight(2);
line(-6,-15-(floorHeight),random(-12,-16),5-(floorHeight)); //fishing line
}
}
pop();
}
function showCloud(){
var cloudHeight = 10; //height of a cloud
var cHeight = this.nClouds * cloudHeight; //height dpends on how many clouds
fill(255,200); //pale white
noStroke();
push();
translate(this.x, this.y); //appear at width, and random y in a constraint
for (var i = 0; i < this.nClouds; i++) {
ellipse(0, -15 - (i * cHeight), this.breadth-10, 30); //draw cloud
}
pop();
}
function showUmbrella(){
var umbrellaHeight = 10;
noStroke();
push();
translate(this.x, this.y+15); //appear at width, random y between shoreline and height(adjusted to ensure no umbrellas in water)
noStroke();
fill(255); //white
rect(0,0, this.breadth, this.height); //umbrella stand
fill(this.color); //random color
rotate(radians(this.angle)); //turn at different angles
arc(0,10,40,40,180,TWO_PI); //umbrella dome
pop();
}
function showPlane(){
push();
translate(this.x, this.y); //appear at width, random y within constraint
noStroke();
scale(this.scale); //scale randomly
fill(220,this.scale*400); //opacity deoends on size
ellipse(50,42.5,10,30); //plane body and wings
ellipse(50,50,50,15);
ellipse(50,60,10,30);
fill(50,this.scale*400); //dark gray, varying opacity
ellipse(33,47,4,4); //cockpit window
for(var x=39;x < 55;x+=4) //plane windows
ellipse(x,50,4,4);
strokeWeight(1);
stroke(0,this.scale*400); //black
line(55,57.5,110,57.5); //flag ropes
line(55,42.5,110,42.5);
fill(this.color); //random color
noStroke();
rect(110,37.5,45+random(-2,2),25); //flag, moving
pop();
}
function showBuilding(){
// var floorHeight = 2; //height of a floor
//var buildingHeight = this.nFloors * floorHeight; //building height depends on how many floors
fill(this.color);
push();
noStroke();
translate(this.x, 70); //appear at width,70 (far shoreline)
rect(0, -10, this.breadth, this.height*2); //draw building
pop();
}
function showRailing(){
push();
translate(this.x, 280); //appear at width,280
fill(200,200,200); //gray
stroke(0); //black
rect(0, -20,this.breadth,20); //draw railing markers
pop();
}
function showPerson(){
push();
translate(this.x, this.y+20); //appear at width, and a random heught between shoreline and bottom of canvas(adjusted to ensure nobody in the water)
fill(this.skinColor); //random brown tone
ellipse(0,-5,5,5); //head
rectMode(CENTER);
fill(this.shirtColor); //random color
rect(0,10,this.breadth,this.height); //body
fill(this.pantsColor); //random color
rect(0,25,this.breadth,this.height); //legs
pop();
}
function showTowel(){
push();
translate(this.x, this.y+30); //appear at width, random y between shoreline and height
fill(this.towelColor); //random color
rect(0,0,this.breadth,this.height); //draw towel
pop();
}
//constructors for each object
function makeBoat(startX,startY) {
var boat = {x: startX, //where it gets translated to
y:startY,
breadth: round(random(20,40)), //it's width
windowBreadth: random(5,10), //window width
speed: random(-2,-0.1), //how fast it moves across canvas
nFloors: round(random(1,3)), //how many floors there are
color: random(150,255), //color of boat
move: moveBoat, //functions to move and show the boat
show: showBoat}
return boat;
}
function makeCloud(startX,startY){
var cloud = {x: startX,
y:startY,
breadth: round(random(60,100)),
speed: random(-0.7,-0.3),
nClouds: round(random(1,3)),
move: moveCloud,
show: showCloud}
return cloud;
}
function makeUmbrella(startX,startY){
var umbrella = {x: startX,
y:startY,
breadth: round(random(2,4)),
speed: round(random(-5,-4.99)),
height: random(25,30),
color: color(random(0,255),random(0,255),random(0,255)), //color of hood
angle: random(-20,20), //angle of hood rotation
move: moveUmbrella,
show: showUmbrella
}
return umbrella;
}
function makePlane(startX,startY){
var plane = {x: startX,
y:startY,
scale: random(1 / 4,1), //size of plane
speed: random(-7,-5),
color: color(random(0,255),random(0,255),random(0,255)),
move: movePlane,
show: showPlane
}
return plane;
}
function makeBuilding(startX){
var building = {x: startX,
breadth: 10,
height:10,
speed: -1.0,
color: random(50,200),
//distance: random(100,200),
//nFloors: round(random(2,8)),
move: moveBuilding,
show: showBuilding
}
return building;
}
function makeRailing(startX){
var railing = {x: startX,
breadth:10,
speed: -20,
move: moveRailing,
show: showRailing
}
return railing;
}
function makePerson(startX,startY){
var person = {x: startX,
y:startY,
breadth: random(5,8),
height: random(14,17),
skinColor: color(random(200,250),random(100,150),random(20,100)),
shirtColor: color(random(0,255), random(0,255),random(0,255)),
pantsColor: color(random(0,255), random(0,255),random(0,255)),
Xspeed: random(-7,-4),
Yspeed: random(-0.5,0.5),
move: movePerson,
show: showPerson}
return person;
}
function makeTowel(startX,startY){
var towel = {x: startX,
y:startY,
breadth: random(10,20),
height: random(5,15),
towelColor: color(random(0,255), random(0,255),random(0,255)),
speed: -5,
move: moveTowel,
show: showTowel}
return towel;
}
function displayHorizon(){
landscape.shift(); //removes the first array element
n = noise(noiseParam); //selecting a noise value
value = map(n,0,1,50,height); //plotting it onto the canvas by mapping
landscape.push(value); //adding it to the lanscape array
noiseParam+=noiseStep; //adding step to parameter
push();
beginShape();
noStroke();
fill(227,232,164); //green
for (i=0;i<width/5; i++){
var newUmbrellaChance = 0.001; //frequencies for objects
var newPersonChance = 0.003;
var newTowelChance = 0.002;
var r = random(0,1); //predictor
if (r < newUmbrellaChance){
umbrellas.push(makeUmbrella(width,random(landscape[i],height))); //construct an object between slope line and the bottom of the canvas
}
if(r<newPersonChance){
people.push(makePerson(width,random(landscape[i],height)));
}
if(r<newTowelChance){
towels.push(makeTowel(width,random(landscape[i],height)));
}
curveVertex(i*5,landscape[i]) //creates slopes by connecting two points
curveVertex((i+1)*5,landscape[i+1])
}
curveVertex(width,height+50); //stable values that create the green fill
curveVertex(0,height+50);
endShape(CLOSE); //end shape and fill it
pop();
}
Dynamic Factory
This code simulates a dynamic factory environment, with variable elements including functionality of the factory, speed of the factory, the background factories, and the time of day. The idea for gears came from another class of mine in which we used squares to create interesting compositions. One of mine was created by overlaying two squares to create a “gear” so I knew I wanted to visualize it in motion for this project. I coded the gears to that they appear to work together, and appear to create an output when doing so. The rest stemmed from this “factory” theme.
var angle = 0; //gear turn
var t = 34; //gear translate
var eSize = 10; //ball sizes, center gear
var ballY = 409; //front ball Y position
var recX = 7*t; //top piston X translation
var RecX = 7*t; //bottom piston X tranlation
var recY=10.5*t; //pistons Y translation
var dir = 1; //top piston direction
var direction = 1; //bottom piston direction
var speed = 2; //piston speed
var recSize = 18; //piston length
var BallY = 125; //middle ball Y position
var windowSize = 25; //window size
var Bally = 300; //furthest ball start
var bright = 0;
function setup() {
createCanvas(500, 409);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background (210,146, 6); //orange brown
push();
if(mouseIsPressed){
if(mouseButton == RIGHT){ //if the right button is pressed
background(210 - mouseY/2, 146 - mouseY/2, 6 - mouseY/2); //gets darker with higher Y
if(mouseY > height/2){ //if the mouse Y is greater half the height
fill(255); //stars
ellipse (100,200,1,1);
ellipse (200,100,5,5);
ellipse (300,100,3,3);
ellipse (300,200,5,5);
ellipse (300,300,3,3);
ellipse (150,50,5,5);
ellipse (420,120,2,2);
ellipse (200,100,5,5);
ellipse (150,150,5,5);
ellipse (180,220,2,2);
ellipse (300,200,1.5,1.5);
ellipse (300,250,1,1);
ellipse (450,100,2,2);
ellipse (100,289,5,5);
ellipse(50,70,2.5,2.5);
ellipse (190,120,2.4,2.4);
ellipse (100,289,5,5);
ellipse(50,70,2.5,2.5);
ellipse (200,60,1.2,1.2);
ellipse(90,90,2,2);
ellipse (230,200,2.4,2.4);
ellipse (460,60,5,5);
ellipse(440,100,2.7,2.7);
ellipse (250,250,2.4,2.4);
ellipse (260,200,3,3);
ellipse(240,220,2.1,2.1);
ellipse(300,110,1.7,1.7);
ellipse (280,190,2.3,2.3);
ellipse (290,140,3.1,3.1);
ellipse(215,210,2,2);
ellipse (400,90,2.3,2.3);
ellipse (410,120,1.5,1.5);
ellipse(420,50,2,2);
}
if(mouseY < height/2){ //if mouse Y is lower than the height
fill(255,255,117);
ellipse(0,mouseY,60,60); //sun
}
}
}
pop();
push();
fill(0,0,0,200);
rectMode(CORNER);
var m = max(min(mouseX, 250), 0);
var size = m * 350.0 / 400.0;
fill(0,0,0,height - mouseY);
rect(10,height/2-recSize*1.5,75,500); //left chimney
rect(0,height/2,size,500); //left building
size = 350-size;
fill(0,0,0,mouseY+50);
rect(110+m*190/400,height/2-recSize*2,size,500); //back building
var ballWidth = max(min(mouseY, 100), 0);
rect(110+m*190/400,height/2-recSize*4,ballWidth,37); //back chimney
if(BallY < 0){ //if ball goes past 0
BallY = height/2-recSize*4; //reappear at height of back chimney
}
BallY = BallY-1; //move up by 1 point
ellipse(110+m*190/400+ballWidth/2,BallY,ballWidth,-ballWidth); //middle ball
if(Bally < 0){ //if ball goes past 0
Bally = 250; //reset ball height to 250
}
Bally = Bally-2 ; //move up by 2 points
fill(0,0,0,mouseX); //opacity changes with mouseX
if(mouseY > 200){ //if mouse Y is greater than half the height
fill(210,146, 6); //fill changes to background (disappears)
}
ellipse(380,Bally,height / 4 - ballWidth,height / 4 - ballWidth); //right ball
fill(0,0,0);
rectMode(CENTER); //rectangles oriented by center point
fill(150);
rect(recX,recY + 5,3.2*recSize,1 / 2*recSize); //top piston still
rect(RecX,recY + 25,3.5*recSize,1 / 2*recSize); //bottom piston still
if(mouseX < 1.5*t & mouseY < 1.5*t){ //if mouse is in the top left corner
if(recX > width-220 || recX < width-266){ //piston range
dir =- dir; //top piston bounces
}
if(RecX > width-220 || RecX < width-266){ //piston range
direction = -direction; //bottom piston bounces
}
fill(150); //light gray
push();
if(mouseIsPressed){
if(mouseButton == LEFT){ //if left mouse button pressed
ballY = ballY-20; //ball speed up to 20 points
speed = 10; //pistons speed up by factor of 10
angle += 4; //angle doubles
}
}
else{
speed = 2; //speed reset to 2
}
pop();
push();
recX += dir*speed //piston move
rect(recX,recY+5,3.2*recSize,1 / 2*recSize); //top piston
RecX += -direction*speed; //piston move
rect(RecX,recY+25,3.5*recSize,1 / 2*recSize); //bottom piston
}
pop();
push();
translate (6.7*t, 11*t); //last piston
if(mouseX < 1.5*t & mouseY < 1.5*t){ //first gear in place
rotate(radians(angle)); //spin rate
}
fill(150);
rect(0,0,50,50); //gear part
quad (t,0,0,t,-t,0,0,-t) //gear part
fill(50); //dark gray
ellipse(0,0, eSize, eSize); //gear center
pop();
push();
translate(t,6.7*t); //first piston
if(mouseX < 1.5*t & mouseY < 1.5*t){
rotate(radians(-angle));
}
fill(150);
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
fill(50);
ellipse(0,0, eSize, eSize);
pop();
rectMode(CORNER);
fill(150)
rect(width-220,height-200,220,200); //front building
rect(width-100,height-250,50,100); //front chimney
fill(175,129,3); //gray-orange
rect(width-205,height-180,windowSize, windowSize*2); //top row windows
rect(width-165,height-180,windowSize,windowSize*2);
rect(width-125,height-180,windowSize,windowSize*2);
rect(width-40,height-180,windowSize,windowSize*2);
rect(width-205,height-120,windowSize, windowSize*2); //middle row windows
rect(width-165,height-120,windowSize,windowSize*2);
rect(width-125,height-120,windowSize,windowSize*2);
rect(width-40,height-120,windowSize,windowSize*2);
rect(width-205,height-60,windowSize, windowSize*2); //bottom row windows
rect(width-165,height-60,windowSize,windowSize*2);
rect(width-125,height-60,windowSize,windowSize*2);
rect(width-40,height-60,windowSize,windowSize*2);
push();
angle += 2
fill(150);
translate(mouseX,mouseY); //cursor gear
rotate (radians(angle));
rectMode(CENTER);
noStroke();
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
pop();
fill(50);
ellipse(mouseX,mouseY,eSize,eSize); //cursor gear center
fill(150);
rectMode(CENTER);
noStroke();
push();
translate(t,2.9*t); //first gear
if(mouseX < 1.5*t & mouseY < 1.5*t){
rotate(radians(-angle))
}
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
fill(50);
ellipse(0,0, eSize, eSize)
pop();
push();
translate(1.5*t,4.8*t); //second gear
if(mouseX < 1.5*t & mouseY < 1.5*t){
rotate(radians(angle))
}
fill(150);
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
fill(50);
ellipse(0,0, eSize, eSize)
pop();
push();
translate(1.5*t,8.6*t); //third gear
if(mouseX < 1.5*t & mouseY < 1.5*t){
rotate(radians(angle))
}
fill(150);
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
fill(50);
ellipse(0,0, eSize, eSize)
pop();
push();
translate(t,10.5*t); //fourth gear
if(mouseX < 1.5*t & mouseY < 1.5*t){
rotate(radians(-angle))
}
fill(150);
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
fill(50);
ellipse(0,0, eSize, eSize)
pop();
push();
translate (2.9*t, 11*t) //fifth gear
if(mouseX < 1.5*t & mouseY < 1.5*t){
rotate(radians(angle))
}
fill(150);
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
fill(50);
ellipse(0,0, eSize, eSize)
pop();
push();
translate (4.8*t, 10.5*t) //sixth gear
if(mouseX < 1.5*t & mouseY < 1.5*t){
rotate(radians(-angle))
}
fill(150);
rect(0,0,50,50);
quad (t,0,0,t,-t,0,0,-t)
fill(50);
ellipse(0,0, eSize, eSize)
pop();
push();
fill(125);
if(mouseX<1.5*t & mouseY<1.5*t){
if(ballY<0){
ballY = 409;
}
ballY = ballY-2; //moves up by 2 points
fill(150);
ellipse(width-75,ballY,eSize*3,eSize*3) //front ball
}
}
Beach
var boats = []; //arrays to hold respective objects
var clouds = [];
var umbrellas = [];
var planes = [];
var buildings = [];
var railings = [];
var people = [];
var towels = [];
var landscape = []; //array that creates slope of shoreline
var noiseParam = 0;
var noiseStep = 0.005; //defines extremity of shoreline slope
function setup() {
createCanvas(480,300);
for (var i = 0; i < 3; i++){ //make a certain amount of (object) to start out, at random locations within a constraint
var rx = random(width);
var ry = random(80,150)
boats[i] = makeBoat(rx,ry);
}
for (var i = 0; i < 3; i++){
var Cx = random(width);
var Cy = random(0,60)
clouds[i] = makeCloud(Cx,Cy);
}
for (var i = 0; i < people.length; i++){
var Tx = random(width);
var Ty = random(250,300)
towels[i] = makeTowel(Tx,Ty);
}
for (var i = 0; i < people.length; i++){
var Px = random(width);
var Py = random(250,300)
people[i] = makePerson(Px,Py);
}
for (var i = 0; i < 5; i++){
var Ux = random(width);
var Uy = random(250,300)
umbrellas[i] = makeUmbrella(Ux,Uy);
}
for (var i = 0; i < 15; i++){
var Bx = random(width);
buildings[i] = makeBuilding(Bx);
}
for (var i = 0; i < 0.9; i++){
var Px = random(width);
var Py = random(0,20)
planes[i] = makePlane(Px,Py);
}
for(var i=0;i<=width/5;i++){
var n= noise(noiseParam); //picks value 0-1
var value = map(n,0,1,50,height); //scales it to the canvas size
landscape.push(value); //adds to array
noiseParam+=noiseStep; //moves to the right for the next point in the array
}
frameRate(5);
}
function draw() {
background(3,220,245);
fill(13,66,212,230); //dark blue
rect(0,height/4,width,height); //body of water
fill(227,232,164,175); //pale beige
rect(0,height/4-7,width,7); //distant shoreline
//call the functions to spawn and despawn respective obejcts
removeBoat();
newBoat();
removeCloud();
newCloud();
removeUmbrella();
removePlane();
newPlane();
removeBuilding();
newBuilding();
removeRailing();
newRailing();
removePerson();
removeTowel();
//call the move and show functions to continually draw and redraw objects after updated coordinates
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].show();
}
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].show();
}
for (var i = 0; i < boats.length; i++){
boats[i].move();
boats[i].show();
}
displayHorizon();
for (var i = 0; i < towels.length; i++){
towels[i].move();
towels[i].show();
}
for (var i = 0; i < people.length; i++){
people[i].move();
people[i].show();
}
for (var i = 0; i < umbrellas.length; i++){
umbrellas[i].move();
umbrellas[i].show();
}
for (var i = 0; i < planes.length; i++){
planes[i].move();
planes[i].show();
}
fill(159,159,159);
rect(0,height-50,width,40);
fill(110,110,110);
rect(0,height-45,width,30);
for (var i = 0; i < railings.length; i++){
railings[i].move();
railings[i].show();
}
}
//functions to remove an object from a displayed array once "out of sight"
function removeBoat(){
var boatsToKeep = []; //the boats that should still be displayed
for (var i = 0; i < boats.length; i++){
if (boats[i].x + boats[i].breadth > 0) { //if the boat is closer than it's width to the edge
boatsToKeep.push(boats[i]); //add this boat to the boats that will be displayed
}
}
boats = boatsToKeep; // only display the baots currently being kept
}
function removeCloud(){
var cloudsToKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].breadth > 0) {
cloudsToKeep.push(clouds[i]);
}
}
clouds = cloudsToKeep;
}
function removeUmbrella(){
var umbrellasToKeep = [];
for (var i = 0; i < umbrellas.length; i++){
if (umbrellas[i].x + umbrellas[i].breadth > 0) {
umbrellasToKeep.push(umbrellas[i]);
}
}
umbrellas = umbrellasToKeep;
}
function removePlane(){
var planesToKeep = [];
for (var i = 0; i < planes.length; i++){
if (planes[i].x + 150 > 0) {
planesToKeep.push(planes[i]);
}
}
planes = planesToKeep;
}
function removeBuilding(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep;
}
function removeRailing(){
var railingsToKeep = [];
for (var i = 0; i < railings.length; i++){
if (railings[i].x > 0) {
railingsToKeep.push(railings[i]);
}
}
railings = railingsToKeep;
}
function removePerson(){
var peopleToKeep = [];
for (var i = 0; i < people.length; i++){
if (people[i].x > 0) {
peopleToKeep.push(people[i]);
}
}
people = peopleToKeep;
}
function removeTowel(){
var towelsToKeep = [];
for (var i = 0; i < towels.length; i++){
if (towels[i].x > 0) {
towelsToKeep.push(towels[i]);
}
}
towels = towelsToKeep;
}
//functions to create new objects that come into sight
function newBoat() {
var newBoatChance = 0.009; //the chance that a new boat will appear
if (random(0,1) < newBoatChance) { //activate probability
boats.push(makeBoat(width,random(100,150))); //add a new boat if the porbability condition is met
}
}
function newCloud() {
var newCloudChance = 0.001;
if (random(0,1) < newCloudChance) {
clouds.push(makeCloud(width,random(0,60)));
}
}
function newPlane() {
var newPlaneChance = 0.003;
if (random(0,1) < newPlaneChance) {
planes.push(makePlane(width, random(0,50)));
}
}
function newBuilding() {
var newBuildingChance = 0.1;
if (random(0,1) < newBuildingChance) {
buildings.push(makeBuilding(width));
}
}
function newRailing() {
var newRailingChance = 0.8;
if (frameCount % 2 == 0 & random(0,1)
Final Proj: Quarantine Baking Simulator
//iris yip
//15-104
//deliverable week 15
//final project
var page = 1; //set up a bunch of different "scenes"
let spatula;
function setup() {
createCanvas(500, 500);
}
function preload(){
img1 = loadImage("https://i.imgur.com/cAQMiab.png");
img2 = loadImage("https://i.imgur.com/Uab6uYu.png");
img3 = loadImage("https://i.imgur.com/usB1pJE.png");
img4 = loadImage("https://i.imgur.com/Imd0Dek.png");
img5 = loadImage("https://i.imgur.com/XLEACUR.png");
img6 = loadImage("https://i.imgur.com/iljGqZW.png");
img7 = loadImage("https://i.imgur.com/Y2B65ub.png");
img8 = loadImage("https://i.imgur.com/ZI2Soyy.png");
day1 = loadImage("https://i.imgur.com/TPgUWUu.png");
day3 = loadImage("https://i.imgur.com/XwD89x3.png");
day4 = loadImage("https://i.imgur.com/i58a6SY.png");
day6 = loadImage("https://i.imgur.com/WIZ958Z.png");
day7 = loadImage("https://i.imgur.com/9f5exQ8.png");
day8 = loadImage("https://i.imgur.com/fWXxfYN.png");
fin = loadImage("https://i.imgur.com/IDq3zRs.png");
die = loadImage("https://i.imgur.com/smkS7Ca.png");
//spatula
spatula1= loadImage("https://i.imgur.com/FMitxk1.png");
//knife
knife1 = loadImage("https://i.imgur.com/a9yMiM4.png");
}
function draw() { //scenes
//page 1
if (page == 1) {
image(img1, 0, 0);
}
//page 2
else if (page == 2) {
image(img2,0,0);
}
//page 3
else if (page == 3) {
image(img3,0,0);
}
//page 4
else if (page == 4){
image(img4,0,0);
}
//page 5
else if(page ==5){
image(img5,0,0);
image(spatula1,mouseX,mouseY);
}
//page 6
else if(page ==6){
image(img6,0,0);
}
else if(page ==7){
image(day1,0,0);
}
else if(page ==8){
image(day3,0,0);
}
else if(page ==9){
image(day4,0,0);
}
else if(page ==10){
image(day6,0,0);
}
else if(page ==11){
image(day7,0,0);
}
else if(page ==12){
image(day8,0,0);
}
else if(page ==13){
image(img7,0,0);
}
else if(page ==14){
image(img8,0,0);
image(knife1,mouseX,mouseY);
}
//bad end
else if (page == 20) {
image(die,0,0);
}
}
function mousePressed() {
// PAGE 1 COMMANDS
if (page == 1) {
if (mouseX > 90 & mouseX < 300 && mouseY > 220 && mouseY < 400) {
page = 2; //proceed forwards into the game
}
else if (mouseX > 300 & mouseX < 400 && mouseY > 350 && mouseY < 400) {
page = 1; //stays the same
}
}
//PAGE 3 COMMANDS
if (page ==3) {
if(mouseX > 350 & mouseY > 200){
page = 4; //proceed forward
}
}
//page 4
if (page ==4){
if(mouseX>400 & mouseY> 220){
page = 5;
}
}
//page 5
if (page ==5){
if(mouseX>150 & mouseX<250 && mouseY>50 && mouseY<250){
page = 6
}
}
//page 6
if(page ==6){
if(mouseX > 400 && mouseX<480 && mouseY> 60 && mouseY<200){
page = 7;
}
}
//page 7
if(page ==7){
if(mouseX>400 & mouseX<480 && mouseY> 60 && mouseY<200){
page = 8;
}
}
if(page ==8){
if(mouseX>400 & mouseY > 60){
page =9;
}
}
if(page ==9){
if(mouseX>50 & mouseX<480 && mouseY>60 && mouseY<300){
page= 13;
}
}
if(page ==13){
if(mouseX>10 & mouseY>10){
page= 14;
}
}
if(page ==14){
if(mouseX>130 & mouseX < 250 && mouseY> 150 && mouseY<200){
page=15;
}
}
}
function keyPressed(){
//PAGE 2 COMMNADS
if(page==2){
if(key=='a'){
page =3; //proceed forwards
}
else if(key != 'a'){
page =20; //dies
}
}
//day1
if(page== 7 & 8 && 9 && 11 && 12){
if(key=='k'){
page = 20
}
else if(key != 'k'){
page =20;
}
}
//last step
if(page ==15){
if(key=='360'){
page = fin
}
else if(key !='360'){
page =20;
}
}
}
For my final project, I wanted to make a ‘point-and-click’ adventure style game about baking during quarantine, which would act like a little tutorial adventure of sorts. Growing up, I played a lot of Cooking Mama on the Nintendo, which is where the primary inspiration for this game came from. The instructions for each step are given on the screen, which ranges from clicking on certain parts of the screen to pressing a key and clicking and dragging.
Overall, there were a few parts that I could not get to work (certain scenes being skipped, etc.) but it was really cool to try to replicate the type of mini-game that I would play a lot when I was younger! If I had more time, I’d go back and clean up the code so that it would run more efficiently, and also add in more complex scenes and a grading scale/system rather than the instant death mechanic function.
Week 15 – Final Project
My project is a simple interactive and informative game based on the theme of Covid-19. The setting takes place in a store with racks that display different supplies people use to prevent the spread of the disease. The main problem I realize these days is that people don’t seem to know which supplies and masks are necessary/appropriate during the pandemic. When you run my program, you should drag the objects in order from numbers 1-12 to either the shopping bag or the trash can. You should place the supply on the shopping bag if you think the object is necessary/effective during the pandemic, and place the supply on the trash can if you think the object is not necessary/effective during the pandemic. You will be able to see texts that explain whether the supplies are helpful or not in preventing Covid-19 when you place them onto the shopping bag or trash can. Also, Christmas season is coming. In celebration of the holiday, I’ve added some Christmas lights to the shop, like how real stores do during the holiday season. If I had more time, I would like to find out other ways to drag objects without having them collect multiple objects when they are overlapped in similar locations on canvas. Although it isn’t a problem in running my program and playing the game, adding codes that help objects be selected one by one despite overlapped locations would be great if I had more time to work on the project.
//Stefanie Suk
//ssuk@andrew.cmu.edu
//15-104 Section D
//Covid-19 Object Variables
var dentalMask = makedentalMask();
var n95Mask = maken95Mask();
var spongeMask = makespongeMask();
var clothMask = makeclothMask();
var homemadeMask = makehomemadeMask();
var bandanaMask = makebandanaMask();
var thermometer = makeThermometer();
var waterBottle = makewaterBottle();
var toiletPaper = maketoiletPaper();
var soap = makeSoap();
var lowSanitizer = makelowSanitizer();
var highSanitizer = makehighSanitizer();
//Shopping bag and Trash Can Variables
var shoppingBag = makeshoppingBag();
var trashCan = maketrashCan();
//Array of Covid-19 supplies
//Array of Shopping bag and Trashcan
var covidObjects = [dentalMask, n95Mask, spongeMask, clothMask, homemadeMask, bandanaMask,
thermometer, waterBottle, toiletPaper, soap, lowSanitizer, highSanitizer];
var bagandTrash = [shoppingBag, trashCan];
//offset variable for mouse press and drag supplies
var offsetX;
var offsetY;
//Objects for Covid-19 supplies, Shopping bag, and Trashcan with specific datas
function makeshoppingBag() {
var shoppingBag = {
imageUrl: "https://i.imgur.com/WdeIUp0.png", //imageURL: url of object images
positionX: 75, //positionX: X position of objects on canvas
positionY: 375, //positionY: Y position of objects on canvas
}
return shoppingBag;
}
function maketrashCan() {
var trashCan = {
imageUrl: "https://i.imgur.com/5jjADwl.png",
positionX: 330,
positionY: 375,
}
return trashCan;
}
function makedentalMask() {
var dentalMask = {
imageUrl: "https://i.imgur.com/Lsg9xeK.png",
imagex: 45,
imagey: 25,
positionX: 41,
positionY: 167,
informationTwo: "Dental Masks, "
+ "Dental masks are effective in preventing Covid-19.", //informationTwo: texts when placed on trashcan
information: "Dental Masks, Effective. "
+ "Dental masks are designed to prevent the spread of droplets and splatters. "
+ "Wearing surgical masks can reduce the spread of respiratory infection."
+ "*Important* Surgical masks are designed to be used only once." //information: texts when placed on shopping bag
}
return dentalMask;
}
function maken95Mask() {
var n95Mask = {
imageUrl: "https://i.imgur.com/b5hh3hx.png",
imagex: 45,
imagey: 25,
positionX: 106,
positionY: 167,
informationTwo: "N95 Masks, N95 masks are effective in preventing Covid-19.",
information: "N95 Masks, Effective. N95 is the most protective mask against coronavirus and other respiratory "
+ "diseases. It filters out 95% of particles from the air."
}
return n95Mask;
}
function makespongeMask() {
var spongeMask = {
imageUrl: "https://i.imgur.com/KhnI7An.png",
imagex: 45,
imagey: 25,
positionX: 171,
positionY: 169,
informationTwo: "Sponge Mask, Not Effective. Sponge mask, or neoprene mask, may be designed to be comfortable, but "
+ "is not effective in preventing the spread of Covid-19 disease. "
+ "*Tips* Adding replaceable filer can help with the mask's effectiveness.",
information: "Sponge Mask, Sponge masks are not effective in preventing Covid-19."
}
return spongeMask;
}
function makeclothMask() {
var clothMask = {
imageUrl: "https://i.imgur.com/SierEPM.png",
imagex: 45,
imagey: 25,
positionX: 41,
positionY: 242,
informationTwo: "Effective, Cloth Masks bought in stores are effective in preventing Covid-19.",
information: "Cloth Mask, Effective. A typical store-bought cloth mask is approximately 50% protective, but "
+ "depending on its construction and quality, it can protect the virus to about 80-95%."
}
return clothMask;
}
function makehomemadeMask() {
var homemadeMask = {
imageUrl: "https://i.imgur.com/scSV8qR.png",
imagex: 45,
imagey: 25,
positionX: 106,
positionY: 242,
informationTwo: "Handmade Mask, Not Effective. A single-layered handmade mask only filters 1% of particles "
+ "from air. A two-layered cotton mask can filter only about 35% of particles. *Tips* More "
+ "layers with more dense woven fabrics can increase the protection.",
information: "Not Effective, Handmade Masks are not effective in preventing Covid-19."
}
return homemadeMask;
}
function makebandanaMask() {
var bandanaMask = {
imageUrl: "https://i.imgur.com/QX6uDBJ.png",
imagex: 45,
imagey: 25,
positionX: 171,
positionY: 242,
informationTwo: "Bandanas, Not Effective. Covering your mouth and nose with a bandana provides little "
+ "protection against droplets and does not filter particles from air.",
information: "Not Effective, Bandanas are not effective in preventing Covid-19."
}
return bandanaMask;
}
function makeThermometer() {
var thermometer = {
imageUrl: "https://i.imgur.com/qbeSsFP.png",
imagex: 30,
imagey: 40,
positionX: 285,
positionY: 160,
informationTwo: "Effective, Thermometers are effective in preventing Covid-19.",
information: "Thermometer, Effective. Using a thermometer to check your body temperature daily is always "
+ "important in preventing the spread of Covid-19. *Important* Make sure to check your body temperature does not go over 37.8C."
}
return thermometer;
}
function makewaterBottle() {
var waterBottle = {
imageUrl: "https://i.imgur.com/0h5YpiF.png",
imagex: 20,
imagey: 45,
positionX: 359,
positionY: 156,
informationTwo: "Not Effective, Water. Suggestions are that drinking every 15 minutes would wash any virus down the throat, but there are at "
+ "least thousands of viruses that we come into contact with and it’s highly unlikely you would wash all that virus down. "
+ "Thus, the primary way the virus is transmitted is through respiratory droplets in the air.",
information: "Not Effective, Drinking water is not effective in preventing Covid-19."
}
return waterBottle;
}
function maketoiletPaper() {
var toiletPaper = {
imageUrl: "https://i.imgur.com/pxMwAgb.png",
imagex: 35,
imagey: 35,
positionX: 415,
positionY: 161,
informationTwo: "Toilet Papers, Not effective. There is absolutely no reason to buy toilet papers for preventing Covid-19. Stop stockpiling "
+ "toilet papers, because they don’t help you prevent the spread of Covid-19.",
information: "Not Effective, Toilet Papers are not effective in preventing Covid-19."
}
return toiletPaper;
}
function makeSoap() {
var soap = {
imageUrl: "https://i.imgur.com/JQBa2uV.png",
imagex: 40,
imagey: 30,
positionX: 285,
positionY: 239,
informationTwo: "Effective, Soaps are effective in preventing Covid-19.",
information: "Soaps, Effective. Washing your hands regularly with soap and water break germs up and remove them, which is one of the most "
+ "effective ways to prevent Covid-19 from spreading."
}
return soap;
}
function makelowSanitizer() {
var lowSanitizer = {
imageUrl: "https://i.imgur.com/YdnSXSO.png",
imagex: 20,
imagey: 45,
positionX: 359,
positionY: 231,
informationTwo: "40% Alcohol Sanitizers, Not Effective. Sanitizers with 40% alcohol doesn’t really help with disinfection. *Important* "
+ "Not only sanitizers, but any alcohol solutions that contain less than 70% alcohol is ineffective.",
information: "Not Effective, Sanitizers with 40% alcohol are not effective in preventing Covid-19."
}
return lowSanitizer;
}
function makehighSanitizer() {
var highSanitizer = {
imageUrl: "https://i.imgur.com/YZihMaD.png",
imagex: 20,
imagey: 45,
positionX: 424,
positionY: 231,
informationTwo: "Effective, Sanitizers with 70% alcohol are effective in preventing Covid-19.",
information: "70% Alcohol Sanitizers, Effective. Sanitizers (or any alcohol solutions) with 70% alcohol or more are "
+ "perfect for disinfecting your hands and objects."
}
return highSanitizer;
}
function preload() {
//Preload background image
backgroundImage = loadImage("https://i.imgur.com/uRLr2HC.png");
//Preload object images, push objects to new array
bagandtrashImages = [];
for (var i = 0; i < bagandTrash.length; i++) {
bagandtrashImages.push(loadImage(bagandTrash[i].imageUrl));
}
covidobjectImages = [];
for (var i = 0; i < covidObjects.length; i++) {
covidobjectImages.push(loadImage(covidObjects[i].imageUrl));
}
}
function setup() {
createCanvas(500, 600);
frameRate(10); //Frame rate for christmas lights
}
function draw() {
//load images of background, covid objects, bag and trash
background(220);
image(backgroundImage, 0, 0, 500, 600);
for (var i = 0; i < bagandTrash.length; i++) {
image(bagandtrashImages[i], bagandTrash[i].positionX, bagandTrash[i].positionY, 90, 100);
}
for (var i = 0; i < covidObjects.length; i++) {
image(covidobjectImages[i], covidObjects[i].positionX, covidObjects[i].positionY, covidObjects[i].imagex, covidObjects[i].imagey);
//drag object with mouse
if (covidObjects[i].drag) {
covidObjects[i].positionX = offsetX + mouseX;
covidObjects[i].positionY = offsetY + mouseY;
}
//When objects placed in specific locations (bag and trashcan), text appears
if ((covidObjects[i].positionX > 65) & (covidObjects[i].positionX < 145)) {
if ((covidObjects[i].positionY > 400) && (covidObjects[i].positionY < 460)) {
noStroke();
fill(163, 160, 72);
rect(3, 502, 486, 100);
fill(0);
text(covidObjects[i].information, 20, 520, 460, 70); //texts appear when placed on shopping bag
}
}
if ((covidObjects[i].positionX > 310) & (covidObjects[i].positionX < 395)) {
if ((covidObjects[i].positionY > 365) && (covidObjects[i].positionY < 465)) {
noStroke();
fill(163, 160, 72);
rect(3, 502, 486, 100);
fill(0);
text(covidObjects[i].informationTwo, 20, 520, 460, 70); //texts appear when placed on trashcan
}
}
}
//lightbulbs on ceiling for Christmas holiday season
for (var i = 5; i < 600; i = i+13) {
push();
translate(i, 5);
makelightBulb();
pop();
}
}
function mousePressed() {
//drag covid objects with mouse when mousepressed
//drag when mouse pressed in specific range of object
for (var i = 0; i < covidObjects.length; i++) {
if (mouseX > covidObjects[i].positionX - 25 & mouseX < covidObjects[i].positionX + 25 && mouseY > covidObjects[i].positionY - 25 && mouseY < covidObjects[i].positionY + 25) {
offsetX = covidObjects[i].positionX - mouseX;
offsetY = covidObjects[i].positionY - mouseY;
covidObjects[i].drag = true;
}
}
}
function mouseReleased() {
for (var i = 0; i < covidObjects.length; i++) {
covidObjects[i].drag = false;
}
}
function makelightBulb() {
//variables for lightbulb colors
var lightColor;
var lightVariations;
//red, green, blue, yellow randomly used
lightColor = floor(random(1, 5));
if (lightColor === 1) {
lightVariations = [255, 0, 0];
}
if (lightColor === 2) {
lightVariations = [0, 255, 0];
}
if (lightColor === 3) {
lightVariations = [0, 0, 255];
}
if (lightColor === 4) {
lightVariations = [255,255,0];
}
//left side of canvas, square lightbulbs
//right side of canvas, circle lightbulbs
fill(lightVariations);
if (mouseX > width/2) {
ellipse(5, 5, 7, 7);
} else {
rect(2, 2, 7, 7);
}
}
Final Project: COVID cases and temperatures
https://editor.p5js.org/ssahasra/present/mf6vOSPgw
The idea was to correlate temparatures and COVID cases of two months far apart from each other in the year 2020.
I used arrays to fill in the values of the number of cases (height of bar) with loops and used the buttons [mouse interactions] and array, objects to create effects of different weather conditions. For some of the weather effects I sourced code from current projects on the internet.
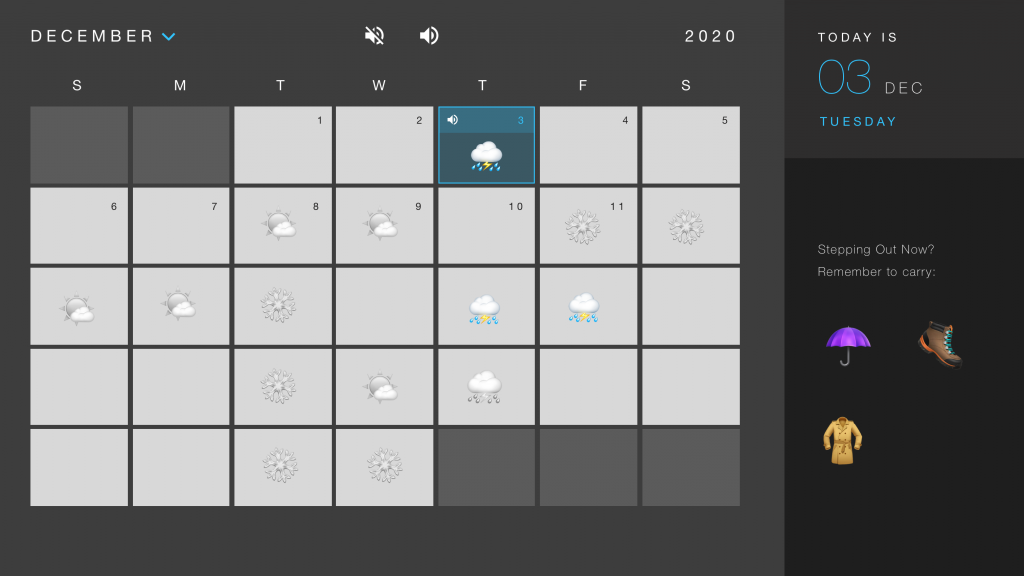
After receiving feedback on my proposal, I made two key changes:
1. Instead of using files to load data I added the values to indices of an array, to create a data visualization + monthly calendar that shows the number of COVID cases
2. I made it more relevant to the theme of 2020. Earlier I was working only with temperature data, but now I decided to represent the relationship between temperature and COVID cases.
The central idea of my projects is to create an interactive visualization + calendar that allows the viewer to compare different months of the year and see how temperature affects the rise in COVID cases (although there are of course other factors for the rise) Furthermore, I want to use mouse interactions to filter certain days when temperatures are in particular range using the buttons on the right (see diagram and code). I am still in the process of adding interactivity and controls using the <function mousePressed()> Optionally, a hover state over one or two of the bars (if time permits, to show the temperature and number of COVID cases to make it a more realistic visualisation)
When the button is pressed, some bar graphs that are not in that temperature range, will disappear and I want to use random motion, I learnt in class to create effects of snow, rain, sun etc in the background of each month. If I am successful in this, as a next step, I would also like to add sound files that create sound effects along with the visual effects of the weather. For the visual effects, my plan is to use the code I used for the snow and change parameters, color, speed etc, to create the other weather effects. For examples: raindrops will be blue and would sway less, and fall down in straighter lines than snow.
Below is the code snippet for the entire final program:
let lastFramePress = false;
let toggle = false;
let value = 0;
let button;
let snowflakes = []; // array to hold snowflake objects
let rain = [];
let snow ;
mayCases = [1540, 1307, 1008, 913, 975, 1022, 1160, 1352, 1160,
1376, 520, 850, 787, 964, 1020, 1050, 517, 931, 728,
747, 1101, 1053, 797, 554, 700, 517, 773, 666, 761, 716, 429]
mayMidTempCases = [0, 1307, 1008, 0, 0, 0, 1160, 0, 0,
1376, 0, 0, 787, 964, 0, 1050, 0, 931, 728,
747, 1101, 1053, 797, 0, 0, 0, 0, 0, 761, 716, 429] //on high temparature days
mayHighTempCases = [0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 1020, 0, 517, 0, 0,
0, 0, 0, 0, 554, 700, 517, 773, 666, 761, 716, 429] //on mid temparature days
mayLowTempCases = [1540, 0, 0, 913, 975, 1022, 0, 1352, 1160,
0, 520, 850, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] //on low temparature days
novCases = [2815, 3648, 3236, 2580, 4562, 4322, 4098, 6023,
5834, 4395, 4647, 6241, 5721, 6486, 6881, 7028,
5874, 6541, 6462, 6365, 6956, 7427, 8362, 6883,
5144, 5584, 6956, 8654, 11268, 5000]
novHighTempCases = [0, 0, 3236, 2580, 4562, 4322, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0]
novMidTempCases = [2815, 3648, 0, 0, 0, 0, 4098, 6023,
0, 0, 4647, 0, 0, 0, 6881, 7028,
5874, 6541, 0, 0, 0, 7427, 8362, 6883,
0, 5584, 0, 0, 0, 0]
novLowTempCases = [0, 0, 0, 0, 0, 0, 0, 0,
0, 4395, 0, 6241, 5721, 6486, 0, 0,
0, 0, 6462, 0, 6956, 0, 0, 0,
5144, 0, 6956, 8654, 11268, 5000]
function preload() {
cloud = loadImage('https://i.imgur.com/MsvLwqH.png');
snowFlake = loadImage('https://i.imgur.com/wvzYocn.png');
sun = loadImage('https://i.imgur.com/AGRTqDm.png');
}
function setup() {
//star-size
let SWH1 = random(2, 8);
let SWH2 = random(6, 10);
let SWH3 = random(10, 13);
background(51,59,68);
createCanvas(1300, 900);
button = createButton("☀️");
button.mousePressed();
button.position(1180, 90);
button.size(50,50);
button.style('font-size', '25px');
button = createButton("☁️");
button.mousePressed();
button.position(1180, 160);
button.size(50,50);
button.style('font-size', '25px');
button = createButton("❄️");
button.mousePressed();
button.position(1180, 230);
button.size(50,50);
button.style('font-size', '25px');
}
function draw() {
background(51,59,68);
fill(255);
textSize(12);
text ('M A Y : C O V I D C A S E S', 50, 80);
text ('N O V E M B E R : C O V I D C A S E S', 50, 780);
textSize(21);
text ('D o C O V I D C a s e s I n c r e a s e W h e n T e m p ar a t u r e s L o w e r ?', 50, 840, 900, 60);
strokeWeight(3.2);
fill(255,10);
noStroke();
rect(50,80,width-200,320);
rect(50,450,width-200,320);
translate(30,50);
fill(255, 80);
may();
fill(255,0,0,80);
november();
calendar();
//sunrays();
rainfall();
noStroke();
fill(255);
//mayHigh();
mousePressed();
fill(255,10);
rect(1137, 27, 60, 60);
rect(1137, 97, 60, 60);
rect(1137, 167, 60, 60);
}
function calendar() {
fill(51,59,68);
for (let i=0;i<31;i++) {
let index = i + 1;
//stroke(255, 50);
push();
fill(255);
noStroke();
textSize(12);
text(index, i*(245/7)+38, 380);
pop();
}
}
//____////____////____////____////____////____////____////____////____////____////____////____//
function createSnowflake() {
// create a random number of snowflakes each frame
let t = frameCount / 60; // update time
for (let i = 0; i < random(5); i++) {
fill(255);
snowflakes.push(new snowflake()); // append snowflake object
}
// loop through snowflakes with a for..of loop
for (let flake of snowflakes) {
flake.update(t); // update snowflake position
flake.display(); // draw snowflake
}
}
function snowflake() {
//referenced from the p5.js open source examples
// initialize coordinates
this.posX = 0;
this.posY = random(-50, 0);
this.initialangle = random(0, 2 * PI);
this.size = random(2, 5);
// radius of snowflake spiral
// chosen so the snowflakes are uniformly spread out in area
this.radius = sqrt(random(pow(width / 2, 2)));
this.update = function(time) {
// x position follows a circle
let w = 0.6; // angular speed
let angle = w * time + this.initialangle;
this.posX = width / 2 + this.radius * sin(angle);
// different size snowflakes fall at slightly different y speeds
this.posY += pow(this.size, 0.5);
// delete snowflake if past end of screen
if (this.posY > height) {
let index = snowflakes.indexOf(this);
snowflakes.splice(index, 1);
}
};
this.display = function() {
ellipse(this.posX, this.posY, this.size);
}
}
//____////____////____////____////____////____////____////____////____//
function createRain() {
// create a random number of snowflakes each frame
let t = frameCount / 60; // update time
for (let i = 0; i < random(5); i++) {
fill(255);
rain.push(new rainfall()); // append snowflake object
}
// loop through snowflakes with a for..of loop
for (let drop of rain) {
drop.update(t); // update snowflake position
drop.display(); // draw snowflake
}
}
function rainfall() {
this.posX = 10;
this.posY = random(0, width);
this.initialangle = random(0, PI);
this.size = random(4, 20);
// radius of snowflake spiral
// chosen so the snowflakes are uniformly spread out in area
this.radius = sqrt(random(0,1000), 1);
this.update = function(time) {
// x position follows a circle
let w = 1; // angular speed
let angle = w * time + this.initialangle;
this.posX = width / 35 + 1000 * sin(angle); // change this angle for rain
// different size snowflakes fall at slightly different y speeds
this.posY += pow(this.size, 5);
// delete snowflake if past end of screen
if (this.posY > 260) {
let index = rain.indexOf(this);
rain.shift(index, 3);
}
}
this.display = function() {
ellipse(this.posX, this.posY, this.size);
}
}
//____////____////____////____////____////____////____////____////____////____//
function sunrays() {
rays = random(280, 650);
//stars in the universe
fill(250, 200, 0);
ellipse(mouseX*1.1, mouseY*1.8, random(2, 8), random(2, 8));
ellipse(mouseX*1.3, mouseY*2.5, random(6, 10), random(6, 10));
ellipse(mouseX*2.3, mouseY*1.5, random(10, 13), random(10, 13));
ellipse(mouseX/1.1, mouseY/1.8, random(2, 8), random(2, 8));
ellipse(mouseX/1.3, mouseY/2.5, random(6, 10), random(6, 10));
ellipse(mouseX/2.3, mouseY/1.5, random(10, 13), random(10, 13));
//glow
fill(200, 130, 10, 20);
ellipse(0, 0, (frameCount % 500)*2, (frameCount % 500)*2);
ellipse(0, 0, (frameCount % 500)*4, (frameCount % 500)*4);
ellipse(0, 0, (frameCount % 500)*8, (frameCount % 500)*8);
ellipse(0, 0, (frameCount % 500)*16, (frameCount % 500)*16);
ellipse(0, 0, (frameCount % 500)*24, (frameCount % 500)*24);
//sun
fill(250, 200, 0);
ellipse(5, -11, rays - 20, rays - 30);
}
function may() {
for (var i=0; i <= mayCases.length; i++) {
fill(255, 99);
rect((i*35+3)+25,350,33,-mayCases[i]*0.035);
//ellipse((i*35+3)+25,190,-mayCases[i]*0.035);
}
if (mayCases[i]*0.25 >= 20) {
fill(255, 99);
}
}
function mayHigh() {
for (var i=0; i <= mayHighTempCases.length; i++) {
fill(255,99);
rect((i*35+3)+25,350,33,-mayHighTempCases[i]*0.035);
}
if (mayHighTempCases[i]*0.25 >= 20) {
fill(255,0,0,99);
}
}
function mayMid() {
for (var i=0; i <= mayMidTempCases.length; i++) {
fill(255,99);
rect((i*35+3)+25,350,33,-mayMidTempCases[i]*0.035);
}
if (mayMidTempCases[i]*0.25 >= 20) {
fill(255,99);
}
}
function mayLow() {
for (var i=0; i <= mayLowTempCases.length; i++) {
fill(255,99);
rect((i*35+3)+25,350,33,-mayLowTempCases[i]*0.035);
}
if (mayLowTempCases[i]*0.25 >= 20) {
fill(255,0,0,99);
}
}
function november() {
for (var i=0; i <= novCases.length; i++) {
rect((i*35+3)+25,400,33,novCases[i]*0.035);
//ellipse((i*35+3)+25,560,-novCases[i]*0.035);
}
}
function novLow() {
for (var i=0; i <= novLowTempCases.length; i++) {
fill(255,0,0,99);
rect((i*35+3)+25,400,33,novLowTempCases[i]*0.035);
//ellipse((i*35+3)+25,560,-novCases[i]*0.035);
}
if (novLowTempCases[i]*0.25 >= 20) {
fill(255,0,0,99);
}
}
function novMid() {
for (var i=0; i <= novMidTempCases.length; i++) {
fill(255,0,0,99)
rect((i*35+3)+25,400,33,novMidTempCases[i]*0.035);
//ellipse((i*35+3)+25,560,-novCases[i]*0.035);
}
if (novMidTempCases[i]*0.25 >= 20) {
fill(255,0,0,99);
}
}
function novHigh() {
for (var i=0; i <= novHighTempCases.length; i++) {
fill(255,0,0,99);
rect((i*35+3)+25,400,33,novHighTempCases[i]*0.035);
//ellipse((i*35+3)+25,560,-novCases[i]*0.035);
}
if (novHighTempCases[i]*0.25 >= 20) {
fill(255,0,0,99);
}
}
function mousePressed() {
//rect(1137, 27, 60, 60);
//rect(1137, 97, 60, 60);
//rect(1137, 167, 60, 60);
if (mouseX >= 1137 && mouseX <= 1197 && mouseY >= 40 && mouseY <= 110) {
mayHigh();
novHigh();
sunrays();
} else if (mouseX >= 1137 && mouseX <= 1197 && mouseY >= 110 && mouseY <= 180) {
mayMid();
novMid();
createRain();
} else if (mouseX >= 1137 && mouseX <= 1197 && mouseY >= 200 && mouseY <= 250) {
mayLow();
novLow();
createSnowflake();
}
}
Final Project
This program is a Covid-19 version of whack-a-mole called put-on-a-mask. The idea was inspired by an online game that my friends and I played during the pandemic called Covidopoly, a Covid-19 version of the board game Monopoly. Like this game, I wanted to make some light out of this dark situation we are in a make a fun, easy, time-passing game that will (hopefully) keep making you play until the pandemic is over.
To play is simple: the player must put masks on the viruses as fast as they can. There will be a total of 4 viruses on the screen at all times and once a virus is successfully clicked (accompanied by a “pop” sound), it will regenerate in a random color to a random position. If the player misses or takes too long to click a virus, they will get a strike– an accumulation of 3 strikes will end the game. The virus that was on the screen for too long will disappear on its own and regenerate in a new random color and position. Every 20 points, the player will level up which makes the time it takes a virus to disappear on its own shorter.
If I had more time, I would like to add more complex elements to the game like making the viruses move around, add more viruses to the screen after a certain amount of levels, or add more clickable elements for bonus points or strike reductions.
var covidviruses = [];
var covidImageLinks = ["https://i.imgur.com/iUnzt1x.png",
"https://i.imgur.com/V9cdbDy.png",
"https://i.imgur.com/OjUptCF.png",
"https://i.imgur.com/heDuTxd.png",
"https://i.imgur.com/PoNLrQ1.png",
"https://i.imgur.com/oIF3cp7.png",
"https://i.imgur.com/jc8muyt.png",
"https://i.imgur.com/X6MPvtK.png"]
var mask;
var coronavirusSound;
var popSound;
var clickSound;
var covidX = [150, 200, 300, 350];
var covidY = [300, 150, 200, 250];
var strike = 0;
// array of virus objects
var virus = [];
var page = 1;
var score = 0;
var ageIncrease = 1;
var maxScore = 0;
var gameLevel = 1;
var angle = 20;
function preload() {
// load pictures (different color viruses)
for(var i = 0; i < covidImageLinks.length; i ++) {
covidviruses[i] = loadImage(covidImageLinks[i]);
}
mask = loadImage("https://i.imgur.com/DdBChjv.png");
coronavirusSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/12/coronavirus.wav");
popSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/12/pop.wav");
clickSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/12/click.wav")
}
function setup() {
createCanvas(480, 480);
useSound();
// create array of objects of 4 starter viruses (location, size, color, age)
for(var i = 0; i < 4; i ++) {
virus[i] = new Object();
virus[i].x = covidX[i];
virus[i].y = covidY[i];
virus[i].color = floor(random(0, 8));
virus[i].size = 90;
virus[i].age = 0;
}
frameRate(40);
noStroke();
}
function soundSetup() {
coronavirusSound.setVolume(.5);
popSound.setVolume(.5);
clickSound.setVolume(.5);
}
function draw() {
// title page
if(page == 1) {
cursor();
createTitlePage();
}
// game
if(page == 2) {
noCursor();
background("#BFEDFF");
// draw 4 viruses to begin
for(var i = 0; i < 4; i ++) {
image(covidviruses[virus[i].color], virus[i].x, virus[i].y, virus[i].size, virus[i].size);
// add virus age
virus[i].age += ageIncrease;
// if virus has been unclicked for 200 frames --> strike + new virus replacement
if(virus[i].age == 200) {
makeNewVirus(i);
strike += 1;
}
}
// 3 misses --> game over
if(strike == 3) {
// go to end page
page = 3;
}
// mask on cursor
imageMode(CENTER);
image(mask, mouseX, mouseY, 90, 90);
updateScore();
// level up every 20 points and increase aging rate (so virus disappears faster)
if(score%20 == 0 & score > maxScore) {
ageIncrease += 1;
maxScore = score;
gameLevel += 1;
}
}
// end page
if(page == 3) {
cursor();
createEndPage();
}
// help page
if(page == 4) {
cursor();
createHelpPage();
}
}
function mousePressed() {
// thresholds of a success click for viruses
var d = [];
for(var i = 0; i < 4; i ++) {
d[i] = dist(mouseX, mouseY, virus[i].x, virus[i].y);
}
// title page
if(page == 1) {
// play button --> reset everything
if(mouseX > 190 & mouseX < 290 && mouseY > 305 && mouseY < 355) {
// go to game page
clickSound.play();
strike = 0;
score = 0;
ageIncrease = 1;
maxScore = 20;
gameLevel = 1;
page = 2;
}
if(mouseX > 165 & mouseX < 315 && mouseY > 135 && mouseY < 285){
// sound effect
coronavirusSound.play();
}
if(mouseX > 140 & mouseX < 340 && mouseY > 365 && mouseY < 415) {
clickSound.play();
page = 4;
}
}
// game page
else if(page == 2) {
// if clicks are successes for any 4 of the viruses --> make pop sound, score increase by 1, new virus replacement
if (d[0] < 45) {
popSound.play();
makeNewVirus(0);
score += 1;
}
else if (d[1] < 45) {
popSound.play();
makeNewVirus(1);
score += 1;
}
else if(d[2] < 45) {
popSound.play();
makeNewVirus(2);
score += 1;
}
else if(d[3] < 45) {
popSound.play();
makeNewVirus(3);
score += 1;
}
else { // a miss
strike += 1;
}
}
// end page
else if(page == 3) {
// play again button --> reset everything
if(mouseX > 125 & mouseX < 355 && mouseY > 290 && mouseY < 370) {
clickSound.play();
strike = 0;
score = 0;
ageIncrease = 1;
maxScore = 20;
gameLevel = 1;
page = 2;
}
// go back to main page
if(mouseX > 140 & mouseX < 340 && mouseY > 365 && mouseY < 415) {
clickSound.play();
page = 1;
}
}
// help page
else if(page == 4) {
// play button --> reset everything
if(mouseX > 190 & mouseX < 290 && mouseY > 305 && mouseY < 355) {
// game page
clickSound.play();
strike = 0;
score = 0;
ageIncrease = 1;
maxScore = 20;
gameLevel = 1;
page = 2;
}
// return to main page
if(mouseX > 140 & mouseX < 340 && mouseY > 365 && mouseY < 415) {
clickSound.play();
page = 1;
}
}
}
// replace clicked viruses or missed viruses
function makeNewVirus(index) {
covidX[index] = random(90, 390);
covidY[index] = random(135, 390);
virus[index].x = covidX[index];
virus[index].y = covidY[index];
virus[index].color = floor(random(0, 8));
virus[index].size = 90;
virus[index].age = 0;
}
// title page (page 0)
// button to play
// button to go to help page
// interactive graphic (makes sound)
function createTitlePage() {
background("#D7C9FF");
// play button
if(mouseX > 190 & mouseX < 290 && mouseY > 305 && mouseY < 355) {
fill("pink");
}
else{
fill("white");
}
rectMode(CENTER);
rect(width/2, 330, 100, 50);
// help button
if(mouseX > 140 & mouseX < 340 && mouseY > 365 && mouseY < 415) {
fill("pink");
}
else{
fill("white");
}
rect(width/2, 390, 200, 50);
textSize(30);
textAlign(CENTER);
// text, box labels
fill("black");
text("put on a mask!", width/2, height/4);
text("play!", width/2, 340);
text("how to play", width/2, 400);
textSize(10);
text("click on me!", width/2, 280);
// animation using transformation
// viruses moving around the borders
imageMode(CORNER);
virusBorder();
// sound effect virus
// mouse hover --> rotate
push();
translate(240, 210);
if(mouseX > 165 & mouseX < 315 && mouseY > 135 && mouseY < 285){
rotate(radians(angle));
}
imageMode(CENTER);
image(covidviruses[6], 0, 0, 150, 150);
pop();
angle += 5;
}
// page 3
function createEndPage() {
background("#D7C9FF");
// play button
if(mouseX > 125 & mouseX < 355 && mouseY > 290 && mouseY < 370) {
fill("pink");
}
else {
fill("white");
}
rectMode(CENTER);
rect(width/2, 330, 200, 50);
// return to main page
if(mouseX > 140 & mouseX < 340 && mouseY > 365 && mouseY < 415) {
fill("pink");
}
else {
fill("white");
}
rect(width/2, 390, 200, 50);
// text, button labels
fill("black");
textSize(30);
textAlign(CENTER);
text("game over!", width/2, 150);
textSize(20);
text("Score: " + str(score), width/2, 200);
text("Highest Level: " + str(gameLevel), width/2, 230);
textSize(30);
text("play again!", width/2, 340);
text("main page", width/2, 400);
imageMode(CORNER);
virusBorder();
}
// keep track of score, strikes, level on game page
function updateScore() {
// print current score, strikes, and level at top
textSize(20);
textAlign(CENTER);
text("Score: " + str(score), width/2, 50);
text("Strikes: " + str(strike), width/2, 70);
text("Level " + str(gameLevel), width/2, 90);
}
// border pattern
function virusBorder() {
for(var i = 0; i < width; i += 40) {
image(covidviruses[floor(random(0, 8))], i, 0, 40, 40);
image(covidviruses[floor(random(0, 8))], i, 440, 40, 40);
image(covidviruses[floor(random(0, 8))], 0, i, 40, 40);
image(covidviruses[floor(random(0, 8))], 440, i, 40, 40);
}
}
// help page, page 4
function createHelpPage() {
background("#D7C9FF");
rectMode(CENTER);
// play button
if(mouseX > 190 & mouseX < 290 && mouseY > 305 && mouseY < 355) {
fill("pink");
}
else{
fill("white");
}
rect(width/2, 330, 100, 50);
// return to home button
if(mouseX > 140 & mouseX < 340 && mouseY > 365 && mouseY < 415) {
fill("pink");
}
else{
fill("white");
}
rect(width/2, 390, 200, 50);
// text, labels
fill("black");
textSize(30);
textAlign(CENTER);
// page button
text("play!", width/2, 340);
// help button
text("main page", width/2, 400);
text("how to play:", width/2, 120);
textSize(15);
text("like whack-a-mole but covid edition! click on the viruses as they appear and cover them with masks! make sure to be fast because the higher the score, the faster they disappear! the game ends after you get 3 misses!",
width/2, 300, 300, 300);
imageMode(CORNER);
virusBorder();
}
Project 11: Landscape
/*
Nicholas Wong
Section A
nwong1@andrew.cmu.edu
*/
var rockForeground = []; //Rocks in foreground
var rockMidground = []; //Rocks in midground
var mountains = []; //Mountains in background
//Rock variables
var foregroundRockChance = 0.03; //Probability of rock spawn per frame
var midgroundRockChance = 0.03; //Probability of rock spawn per frame
//Mountain variables
var noiseParam = 1; //Noise param for mountain generation
var noiseStep = 0.01; //Noise step for mountain generation
//Sky variables
var offset = 23; //Wave offset
//Floor grid variables
var lineStart = []; //Line at horizon
var lineEnd = []; //Line at bottom of canvas
function setup()
{
frameRate(30);
createCanvas(480, 300);
//Initialize mountains
for(let i = 0; i < width/4; i++)
{
var n = noise(noiseParam);
mountains[i];
var value = map(n, 0, 1, 0, height);
mountains.push(value);
noiseParam += noiseStep;
}
//Initialize Floor lines
for(let i = 0; i<49; i++)
{
lineStart[i] = 10*i;
lineEnd[i] = -1200 + (60*i);
}
}
function draw()
{
background(255, 166, 200);
//Draw sky wave
drawSkyPattern();
//Draw mountains
drawMountains();
//Draw floor
drawFloor();
//Draw floor lines
drawFloorLines();
//Color for rocks
var foregroundColor = color(115, 31, 77);
var midgroundColor = color(130, 57, 84);
//Create rocks based on probability
if(random(0,1) < foregroundRockChance)
{
rockForeground.push(createRock(width + 20,220 + random(0,20),20,random(20,30),foregroundColor)); //Rocks in foreground
}
else if (random(0,1) < midgroundRockChance)
{
rockMidground.push(createRock(width + 20, 190 + random(-20,20),10, 20, midgroundColor)); //Rocks in background
}
//Update positions
updateMidgroundRocks();
updateForegroundRocks();
}
//Draw wave
function drawSkyPattern()
{
push();
noFill();
for(let w = 0; w <= height; w += 10) //Number of waves
{
strokeWeight(1)
stroke(255)
//Create curvy lines
beginShape();
for(let x = 0; x < width; x++)
{
let angle = offset + x *0.01;
let y = map(sin(angle), -1, 1, 0, 50);
vertex(x,y + w);
}
vertex(width,height);
vertex(0,height);
endShape();
}
pop();
}
//Draw mountains
function drawMountains()
{
//Add and remove noise values to mountain list
mountains.shift();
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
noiseParam += noiseStep;
mountains.push(value);
//Draw mountain shapes based on noise values
beginShape();
for(var i = 0; i <= width/4; i++)
{
fill(224, 105, 149);
noStroke();
vertex((i * 6), mountains[i] - 50);
vertex((i + 1) * 6, mountains[i + 1] - 50);
vertex(300, 100000);
}
endShape(CLOSE);
//Draw mountain shapes based on noise values
beginShape();
for(var i = 0; i <= width/3; i++)
{
fill(191, 86, 125);
noStroke();
vertex((i * 10), mountains[i]- 10);
vertex((i + 1) * 10, mountains[i + 1] - 10);
vertex(300, 100000);
}
endShape(CLOSE);
}
//Update and cull rocks
function updateForegroundRocks()
{
for(let i = 0; i < rockForeground.length; i++)
{
rockForeground[i].move();
rockForeground[i].display();
//Check to see if rocks are off canvas
if(rockForeground[i].x + rockForeground[i].size < 0)
{
rockForeground.shift(); //Remove rock
}
}
}
//Update and cull rocks
function updateMidgroundRocks()
{
//Check to
for(let i = 0; i < rockMidground.length; i++)
{
rockMidground[i].move();
rockMidground[i].display();
//Check to see if rocks are off canvas
if(rockMidground[i].x + rockMidground[i].size < 0)
{
rockMidground.shift(); //Remove rock
}
}
}
//Draw floor lines
function drawFloorLines()
{
push();
for(let i = 0; i < 49; i++)
{
stroke(255, 148, 194);
strokeWeight(1);
//Draw line
line(lineStart[i],170,lineEnd[i],height);
//Move lines
lineStart[i] -= 5;
lineEnd[i] -= 30;
//Reset lines if off canvas
if(lineStart[i] == 0)
{
lineStart[i] = width;
}
if(lineEnd[i] == -1200)
{
lineEnd[i] = 1680;
}
}
pop();
}
//Draw floor rectangle
function drawFloor()
{
push();
noStroke();
fill(158, 70, 102);
rect(0,170,width,height);
pop();
}
//Create rock
function createRock(xPos, yPos,rockSpeed, rockSize, rockColor, )
{
var fRock = {x: xPos,
y: yPos,
speed: rockSpeed * -1,
sides: random(3,5),
size: rockSize,
color: rockColor,
variation: random(0.5,1),
move: rockMove,
display: rockDisplay}
return fRock;
}
function rockMove()
{
this.x += this.speed; //Move the rock
}
function rockDisplay()
{
//Change rock color
fill(this.color);
noStroke();
//Create rock based on size, variation, and number of sides
let angle = TWO_PI / this.sides;
beginShape();
for (let a = 0; a < TWO_PI; a += angle)
{
let sx = this.x + cos(a * this.variation) * this.size;
let sy = this.y + sin(a * this.variation) * this.size;
vertex(sx, sy);
}
endShape(CLOSE);
}
A stylized desert. Rocks are objects that are randomly generated, and are automatically culled when off the canvas. Rocks further away from the bottom of the canvas move slower than rocks closer to the bottom of the canvas. Mountains vary in speed to give the illusion of depth.
Inspiration:
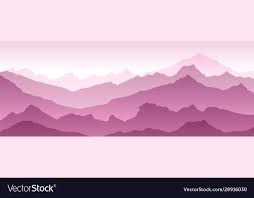
Project 11
var hills = [];
var noiseParam = 0;
var noiseStep = 0.03;
var xTree = [];
var yTree = [];
var car;
function preload() {
car = loadImage("https://i.imgur.com/dk31naF.png");
}
function setup() {
createCanvas(400, 250);
for (var i = 0; i <= width/5; i ++) {
var n = noise(noiseParam);
var value = map(n, 0, 1, 0 ,height);
print(n);
hills.push(value);
noiseParam += noiseStep;
}
frameRate(10);
imageMode(CENTER);
}
function draw() {
background(0, 59, 107); //dark sky
hills.shift();
var n = noise(noiseParam);
var value = map(n, 0, 1, 0 ,height);
hills.push(value);
noiseParam += noiseStep;
for (var i = 0; i < width/5; i ++) {
var x = i*5;
noStroke();
fill(22, 105, 120);
beginShape(); //drawing the hills
vertex(x, height);
vertex(x, hills[i]);
vertex(x+5, hills[i+1]);
vertex(x+5, height);
endShape();
//draw tree if hillValue[i] is smaller than the points before & after
if (hills[i] < hills[i+1] && hills[i] < hills[i-1]) {
tree(x, hills[i]);
}
}
//insert car image
image(car, width/2, height/2, 450, 300);
}
function tree(x, hills) {
fill(1, 120, 33)
triangle(random(x-2, x-5), hills*1.2, random(x, x+3),
hills*0.8, x+5, hills*1.2);
stroke(46, 19, 19);
strokeWeight(2);
line(x, hills*1.2, x, hills*1.5);
}
This animation shows a baby sitting inside a car in the middle of a long drive at night, staring out into the mountains.