Linyi Dai was an architecture student at RISD in 2015. Their piece, which was featured in Fast Company’s article “The Value of Randomness in Art and Design” uses architectural rendering and I admire how they manipulated the random function to create a 3-dimensional shape. I think the way artists can use computers to make shapes is really cool, especially 3-D ones. Dai restricted the random values to a certain parameter and used the value of each register over 50 steps to generate the rungs of a sphere. Dai is an architect and I think this piece demonstrates the ability to render 3-D shapes in a way that is necessary for their field.
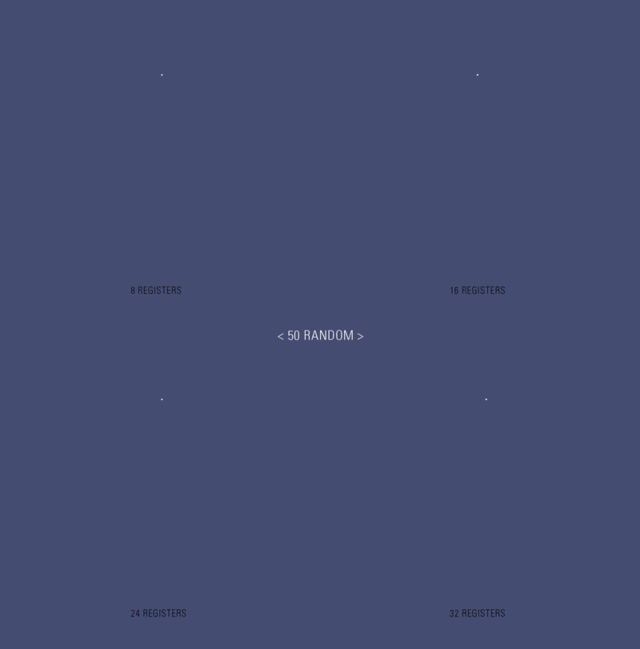