/*
* Andrew J Wang
* ajw2@andrew.cmu.edu
* Section A
*
* This program draws line arts
*/
var maxNum = 30;
function setup() {
createCanvas(400, 300);
background(0);
}
function draw() {
background(0);
//using the 3 functions for 3 different shapes
backgroundStrings (50);
stroke(255);
circleStrings(200,150,200,500);
stroke(255,0,0,255);
circleStrings(200,150,100,58);
push();
fill(255);
ellipse(200,150,100);
pop();
stroke(255,0,0,255);
hexagonStrings(200,150,100,5,10);
}
function circleStrings(Cx,Cy,Cd,Cl) {
for (var k = 0; k < maxNum; k++)
{
//grabing points on the circle
y=sin((k*(360/maxNum))*Math.PI/180)*(Cd/2)+Cy;
x=cos((k*(360/maxNum))*Math.PI/180)*(Cd/2)+Cx;
//making lines that is perpandicular to the radius with adjustable lengths
p1X = x + cos(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p1Y = y + sin(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p2X = x - cos(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p2Y = y - sin(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
line (p2X,p2Y,p1X,p1Y);
}
}
function hexagonStrings(Hx,Hy,Hd,num,seg) {
//make arrays
const array = [];
var angle = 360/num;
for (var k = 0; k < num; k++)
{
array[k] = new Array();
y=sin(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)+Hy;
x=cos(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)+Hx;
line (Hx,Hy,x,y);
for (var s = 0; s<seg; s++)
{
//3D array grabing every points on the line of the star
array[k][s] = new Array();
var y1=sin(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)*s/seg+Hy;
var x1=cos(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)*s/seg+Hx;
array[k][s][0] = x1;
array[k][s][1] = y1;
}
}
//cross connecting those lines using for loop
for (var k = 0; k < num-1; k++)
{
for (var s = 0; s<seg; s++)
{
line(array[k][s][0],array[k][s][1],array[k+1][seg-1-s][0],array[k+1][seg-1-s][1]);
}
}
for (var s = 0; s<seg; s++)
{
line(array[num-1][s][0],array[num-1][s][1],array[0][seg-1-s][0],array[0][seg-1-s][1]);
}
}
function backgroundStrings (num) {
for (var k=0; k<num; k++)
{
//cross connecting with for loop
stroke(255/num*k,0,0,255);
line (k*width/num,0,width,k*height/num);
line (width-k*width/num,height,0,height-k*height/num);
line (k*width/num,height,width,height-k*height/num);
line (width-k*width/num,0,0,k*height/num);
}
}
Month: September 2022
Looking Outwards 04 – Sound Art
Material Sequence – Physical materiality of sound
Mo H Zareei
19/11/2021

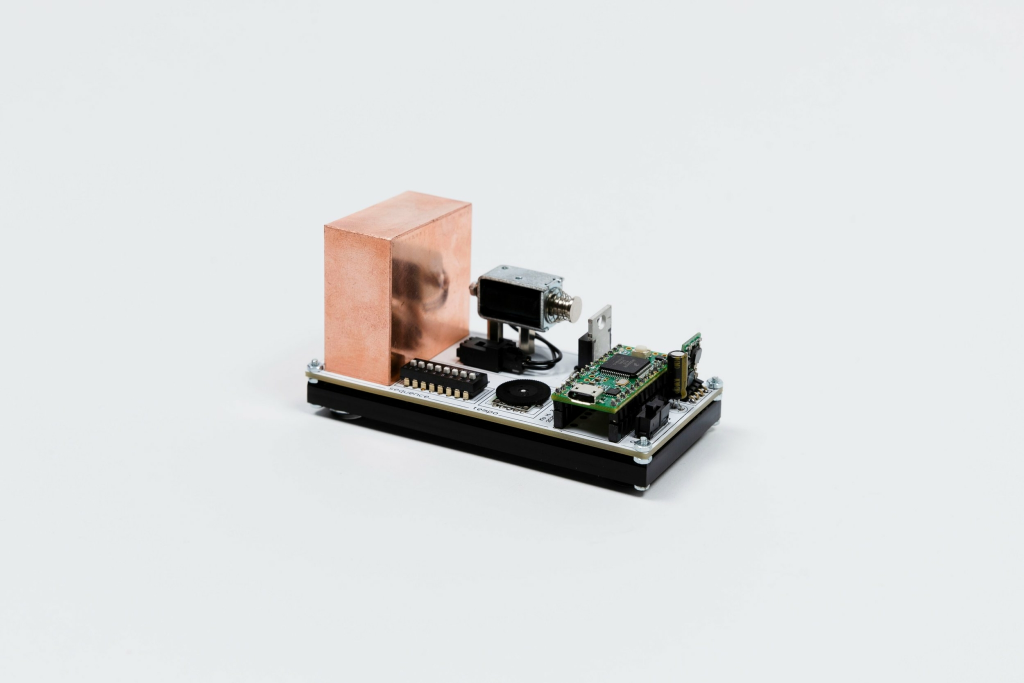
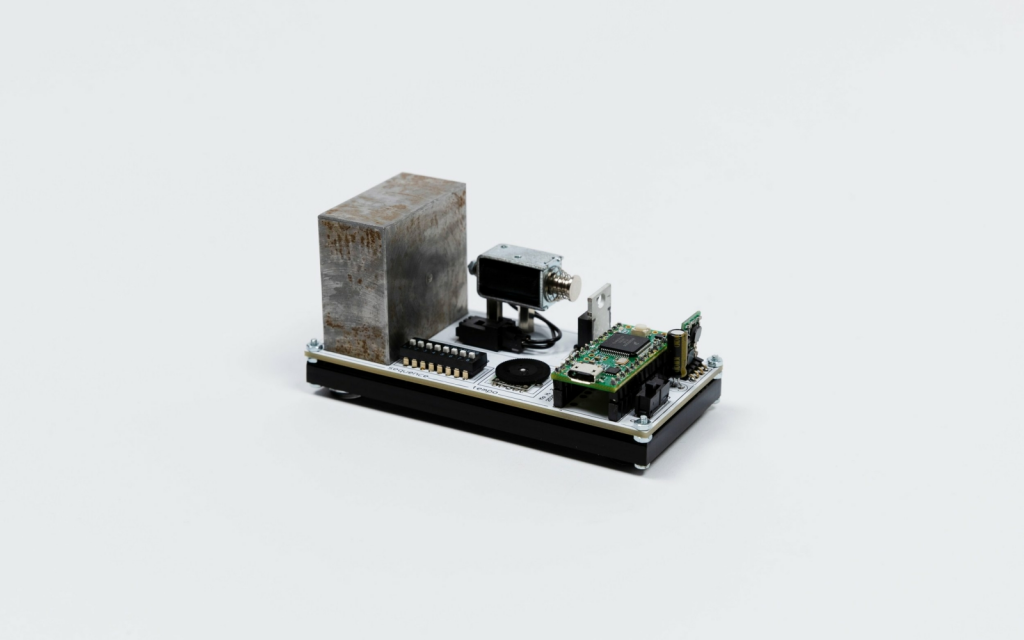
The project I’ve chosen is the “Material Sequencer: Physical materiality of sound” by Mo H Zareei. The sequencer itself is quite simple, composed mainly of 4 parts: 1, control switches; 2, onboard dial; 3, actuator/solenoid; 4, material block. The control switches inform the beat pattern within a 8 step rhythmic sequence in which the solenoid strikes the material block; the onboard dial controls the tempo of the sequence; while the material block determines the timbre of the sounds generated. By just watching it operate, the functions of each component are easily discernible, laying out the process of conversion from input to electrical to kinetic to output via sound in a clear and easily digestible manner, demystifying an otherwise ‘black box’ process. I admire the simplicity of the material artifact and the elegance with which it incorporates exploration of the sound profile of various materials. Zareei positions this work as being “a reductionist celebration of unadorned raw material through rigorous functionalism” that takes “the sequencing process outside the black box and into the acoustic realm, flaunting its materiality and physicality.”
From my understanding, each switch has a individual hard coded behavior(ie. only firing once every 5, 7, 10, etc. seconds), so toggling different switches at the same time allows for various combinations of beat patterns as a result of mixing different behaviors. The creator’s artistic sensibilities are inflected in the final sonic output via the choices of materials, switch combination, choice of the solenoid, and interaction with the tempo dial such that the output is able to manipulated according the user’s preferences.
Links:
https://www.creativeapplications.net/sound/material-sequencer-physical-materiality-of-sound/
LO 4 – Sound Art
I really like the albums that the Fat Rat, a music producer, made because I really like his style and his unique use of those special sound effects. (I’ll put a link for one of the songs he made (Unity) here) Most digital music producers always use a DAW (digital audio workstation) to make their music, and I would briefly explain how those producers use a DAW to make their music. First, they put down chords on some specific track lists (that look like a timeline) using pre-recorded chords of different instruments (which the Fat Rat mostly uses pre-recorded game sound effects, usually electronic music using programmable sound generator sound chips) in order like building blocks and then, they arrange those musical sentences (chords) so it all fits together to form a rhythm. After arranging those notes, producers have to adjust the audio levels of each track list (usually different instruments) to make them sound right, and this process is called the mix stage. In between the stages, the producer can easily add effects or change chords (like laying on top of the base note), making the music original and flexible to changes.
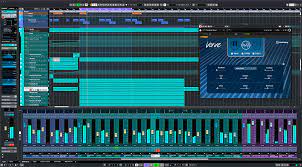
Looking Outwards-04-Section B
Experiments in Musical Intelligence, EMI or EMMY, is a program that analyzes musical compositions and generates entirely new compositions that emulate the sound, style, mood, and emotion of the original. Written by composer, author, and scientist, David Cope, this project allows entirely new compositions to be algorithmically generated in the style of any composer. Compositions have been generated in the styles of Bach, Beethoven, Chopin, and many more, including Cope himself. In fact, Cope’s original inspiration for the software project was writer’s block. He was stuck and wanted to identify his own compositional style.
Although the software is data driven and bases its compositions on works by the original composer, it never repeats or copies the original work. The compositions generated are unique. Cope’s software deconstructs the original works; then records their time signatures. The final step runs the data through a recombinant algorithm for which Cope holds a patent.
This project was truly revolutionary for its time. It inspires questions about creativity and the mind. Originally written in the LISP programming language in the 1980s, it has been modified to use AI techniques as they have advanced. Interestingly, the generative compositions have been used in a type of Turing Test. One particular test set out to see if audiences could identify which of three compositions was actually composed by Bach, which was an emulated composition written by a human, and which was generated by a computer. Audiences chose the EMMY generated composition as the actual Bach. Perhaps EMMY is the first piece of software to pass the Turing Test.
To learn more about David Cope and EMMY click here and here.
LookingOutwards-04 Sound Arts
Light & Sound Synthesis: In Conversation with Amay Kataria by Amay Kataria
The project Supersynthesis use creates an interaction between light and sounds. I admire how the project changes the patterns of the lights depending on the patterns of the sounds. I admired the changing light patterns, because it satisfied my imagination of how programming influences reality by creating lights that simultaneously change in pattern with sound waves. In this sense, these lights just visualized sound waves.
It’s complicated for me to understand how the programs behind this work, but I can summarize it in an abstract manner. The artwork was divided into 24 light sources that have 24 pitches, which will be triggered when different pitches are detected. The program Amay created can allow machines to detect each pitch and control the light sources.
The Amay Kataria’s artistic sensibilities manifest in the final form through visualization of the invisible sounds waves with lights. It was visually pleasant to watch these light changes in wave patterns in the dark according to the sound of the drums.
Link: Light & Sound Synthesis: In Conversation with Amay Kataria – CreativeApplications.Net
Video:
LO 04: VOLUME by SOFTlab, NY 2017
Volume, as described by the author is an interactive cube of responsive mirrors that redirect light and sound responsive to the surrounding movement. The installation commissioned by HP consists of a square grid of 100 mirrored panels with individually addressable LED lights sandwiched along the vertical sides. These panels are mounted on posts with rotating motors. The installation comes to life when the panels rotate and align to human movement, where the human becomes the attractor point for the mirrors to align and face. The sound transmission is also controlled tracing the attractor point and the light in turn react to the sound produced by the panels. This interconnected network of reactive movement and sound fills the atmosphere around the installation. To trace the human motion, array of depth camera is used and identify them as points. Much like the mouseX and mouseY used to locate the mouse pointer. The position tracking uses 6 Kinetic V2 cameras to identify blobs and depth image is retrieved using KineticPV2 library.
Looking Outwards – 04
Sole is an artificial sun by Quiet Ensemble. The purpose of this project is to create a 360 degree video – mapping to simulate the movement of the sun around the inside of a space. Their project used the space of the Salone degli Incanti in Trieste.
Using artificial shadows and lights, the user is able to experience an unreal simulation of a space at any real or unreal context showing imaginary as well as possible paths, “broadening our horizons”. I like the combination between light and technology in order to make a replication of possibilities or non-possibilities.
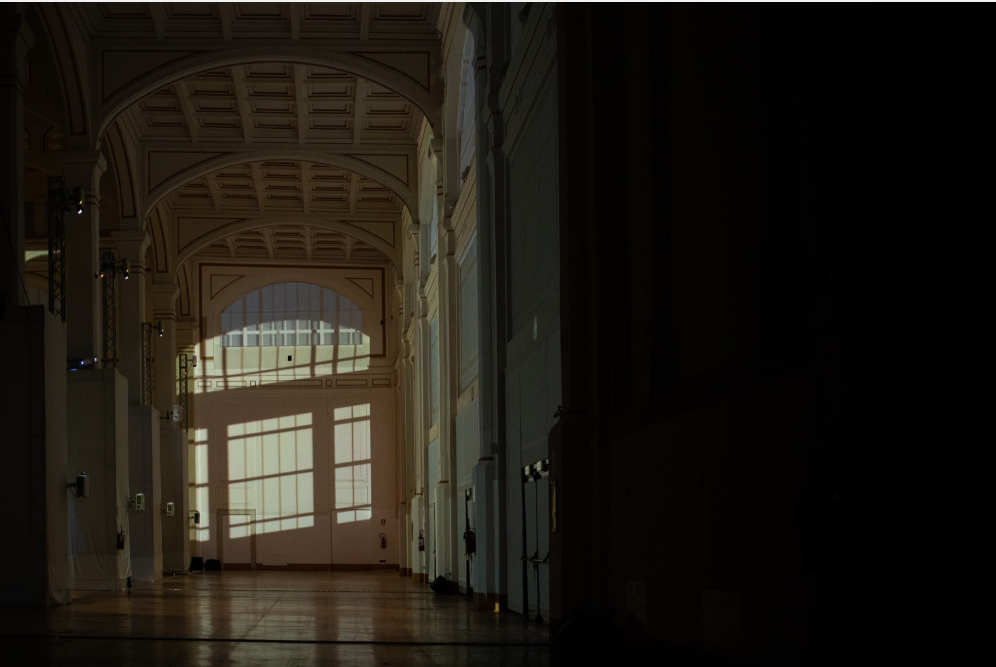
Project 4 – String Art
When you reload it changes the color of the bottom left quadrant.
var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 30;
function setup() {
createCanvas(400, 300);
background(50);
strokeWeight(0.01);
stroke(255);
line(50, 150, 200, 250);
line(50, 150, 200, 50);
line(200, 50, 350, 150);
line(350, 150, 200, 250);
line(50, 150, 350, 150);
line(200, 50, 200, 250);
//line(50, 50, 150, 300);
//line(300, 300, 350, 100);
dx1 = (200-50)/(1.05*numLines); // top left line X
dy1 = (150-50)/(1.05*numLines); // "" Y
dx2 = (350-200)/(1.05*numLines); // top right line X
dy2 = (150-50)/(1.05*numLines); // "" Y
dx3 = (350-200)/(1.05*numLines); //bottom right line X
dy3 = (250-150)/(1.05*numLines); // "" Y
dx4 = (200-50)/(1.05*numLines); //bottom left line X
dy4 = (250-150)/(1.05*numLines); // "" Y
}
function draw() {
strokeWeight(0.3);
stroke(0, 255, 0); //Quadrant 1 Y Changing (Green)
var x1 = 50;
var y1 = 150;
var x2 = 200;
var y2 = 50;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
y2 += dy2;
}
stroke(255, 0, 0);//Quadrant 2 Y Changing (Red)
var x3 = 350;
var y3 = 150;
var x2 = 200;
var y2 = 50;
for (var i = 0; i <= numLines; i += 1) {
line(x3, y3, x2, y2);
y2 += dy2;
}
stroke(255, 0, 0); //Quadrant 3 Y Changing (Red)
var x3 = 350;
var y3 = 150;
var x4 = 200;
var y4 = 250;
for (var i = 0; i <= numLines; i += 1) {
line(x3, y3, x4, y4);
y4 -= dy4;
}
stroke(random(255), random(255), random(255)); //Quadrant 4 Y Changing (Random);
var x1 = 50;
var y1 = 150;
var x4 = 200;
var y4 = 250;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x4, y4);
y4 -= dy4;
}
stroke(77, 77, 255); //Quadrant 1 X Changing (Blue)
var x2 = 200;
var y2 = 50;
var x1 = 50;
var y1 = 150;
for (var i = 0; i <= numLines; i += 1) {
line(x2, y2, x1, y1);
x1 += dx1;
}
stroke(80, 200, 120);
var x2 = 200; //Quadrant 2 X Changing (Green)
var y2 = 50;
var x3 = 350;
var y3 = 150;
for (var i = 0; i <= numLines; i += 1) {
line(x2, y2, x3, y3);
x3 -= dx3;
}
stroke(0, 0, 255); //Quadrant 3 X Changing (Blue)
var x4 = 200;
var y4 = 250;
var x3 = 350;
var y3 = 150;
for (var i = 0; i <= numLines; i += 1) {
line(x4, y4, x3, y3);
x3 -= dx3;
}
stroke(random(255), random(255), random(255)); //Quadrant 4 X Changing (Random Color)
var x4 = 200;
var y4 = 250;
var x1 = 50;
var y1 = 150;
for (var i = 0; i <= numLines; i += 1) {
line(x4, y4, x1, y1);
x1 += dx1;
}
noLoop();
}
Project 04 String Art
Throughout this project, I felt it was a little difficult to figure out what I wanted to create, but it was interesting to consider how forms could appear with the use of repeated lines. Originally, I was thinking about a butterfly with the ground and sky as a background, but I decided to use translate and rotate to twist the ground to instead become colorful lines emphasizing the butterfly’s movement (instead of the ground) which I think makes it a little more fun and dynamic.
// Rachel Legg / rlegg / Section C
var dx1;
var dy1;
var dx2;
var dy2;
var dx3;
var dy3;
var dx4;
var dy4;
var numLines = 15;
function setup() {
createCanvas(300, 400);
background(0);
}
function draw() {
background(0);
//move over orgin and rotate so aligns w/ butterfly
push();
translate(-132, -20);
rotate(radians(15));
//light blue lines toward center @ slant
stroke(0);
strokeWeight(1);
line(0, 300, 10, 390);
line(250, 250, 200, 350);
dx1 = (10-0)/numLines;
dy1 = (390 - 300)/numLines;
dx2 = (350-250)/numLines;
dy2 = (200 - 250)/numLines;
stroke(135, 206, 235); //lightblue
var x1 = 0;
var y1 = 400;
var x2 = 180;
var y2 = 300;
for (var i = 0; i <= numLines; i += 1){
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
//layer and tranlate green group to the right
push();
translate(0, 25);
rotate(radians(-15)); //more tilt and move orgin
noStroke();
strokeWeight(1);
line(0, 300, 10, 390);
line(250, 250, 200, 350);
dx1 = (10-0)/numLines;
dy1 = (390 - 300)/numLines;
dx2 = (350-250)/numLines;
dy2 = (200 - 250)/numLines;
stroke(144, 238, 144); //light green
var x1 = 0;
var y1 = 400;
var x2 = 180;
var y2 = 300;
for (var i = 0; i <= numLines; i += 1){
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
pop();
//layer and tranlate pink group to the right
push();
translate(0, 55);
rotate(radians(-30));
noStroke();
strokeWeight(1);
line(0, 300, 10, 390);
line(250, 250, 200, 350);
dx1 = (10-0)/numLines;
dy1 = (390 - 300)/numLines;
dx2 = (350-250)/numLines;
dy2 = (200 - 250)/numLines;
stroke(255, 0, 127); //pink
var x1 = 0;
var y1 = 400;
var x2 = 180;
var y2 = 300;
for (var i = 0; i <= numLines; i += 1){
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
pop();
//layer and tranlate purple group to the right
push();
translate(0, 120);
rotate(radians(-45));
noStroke();
strokeWeight(1);
line(0, 300, 10, 390);
line(250, 250, 200, 350);
dx1 = (10-0)/numLines;
dy1 = (390 - 300)/numLines;
dx2 = (350-250)/numLines;
dy2 = (200 - 250)/numLines;
stroke(147, 112, 219); //purple
var x1 = 0;
var y1 = 400;
var x2 = 180;
var y2 = 300;
for (var i = 0; i <= numLines; i += 1){
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
pop();
//layer and tranlate yellow group to the right
push();
translate(0, 170);
rotate(radians(-50));
noStroke();
strokeWeight(1);
line(0, 300, 10, 390);
line(250, 250, 200, 350);
dx1 = (10-0)/numLines;
dy1 = (390 - 300)/numLines;
dx2 = (350-250)/numLines;
dy2 = (200 - 250)/numLines;
stroke("yellow"); //yellow
var x1 = 0;
var y1 = 400;
var x2 = 180;
var y2 = 300;
for (var i = 0; i <= numLines; i += 1){
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
pop();
pop();
//butterfly!!!
//blue part of wings : top left to bottom right
push();
translate(175, 15); //make smaller and move to align w/ lines
rotate(radians(12));
scale(2/5);
stroke("blue");
strokeWeight(2);
line(0, 0, 50, 180);
line(250, 220, 300, 400);
dx1 = (50 - 0)/numLines;
dy1 = (180 - 0)/numLines;
dx2 = (300 - 250)/numLines;
dy2 = (400 - 220)/numLines;
var x1 = 0;
var y1 = 0;
var x2 = 250;
var y2 = 220;
for (var i = 0; i <= numLines; i += 1){
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
//magenta part of wings : top right to bottom left
stroke("magenta");
strokeWeight(2);
line(50, 220, 0, 400);
line(300, 0, 250, 180);
dx3 = (0-50)/numLines;
dy3 = (400 - 220)/numLines;
dx4 = (250 - 300)/numLines;
dy4 = (180 - 0)/numLines;
var x3 = 50;
var y3 = 220;
var x4 = 300;
var y4 = 0;
for (var i = 0; i <= numLines; i += 1){
line(x3, y3, x4, y4);
x3 += dx3;
y3 += dy3;
x4 += dx4;
y4 += dy4;
}
//butterfly body in center of criss cross
stroke("yellow");
strokeWeight(1);
noFill();
for(var l = 10; l <= 200; l += 10){ //repeated rings make up body
ellipse(150, l + 90, 10, 10);
}
line((width/2) + 3, height/4, (width/2) + 30, (height/4) - 35);
line((width/2) - 3, height/4, (width/2) - 30, (height/4) - 35);
pop();
noLoop();
}
Project 04 – String Art
This project consists of abstract string art made with multiple stacking of straight lines, creating an illusion of circles that look like octagons. The most challenging part of this project is organizing the direction the lines are going.
//Jenny Wang
//Section B
var dx1;
var dy1;
var dx2;
var dy2;
var dx3;
var dy3;
var dx4;
var dy4;
var dx5;
var dy5;
var dx6;
var dy6;
var dx7;
var dy7;
var dx8;
var dy8;
var numLines = 15
function setup() {
createCanvas(400, 300);
background("black");
var cx = width/2 //center x
var cy = height/2 //center y
//draw ellipse
noStroke();
fill(110);
ellipse(cx,cy,110,110);
fill(180);
ellipse(cx,cy,80,80);
fill(230);
ellipse(cx,cy,50,50);
stroke("blue")
line(cx,cy,width/2,0);//line 1
line(cx,cy, width, height/2);//line2
line(cx,cy,width/2,height);//line 3
line(cx,cy, 0,height/2);//line4
//Q1
dx1 = (cx-cx)/numLines;
dy1 = (cy-0)/numLines;
dx2 = (width-cx)/numLines;
dy2 = (cy-cy)/numLines;
//Q2
dx3 = (cx-cx)/numLines;
dy3 = (cy-height)/numLines;
dx4 = (width-cx)/numLines;
dy4 = (cy-cy)/numLines;
//Q3
dx5 = (cx-cx)/numLines;
dy5 = (cy-height)/numLines;
dx6 = (0-cx)/numLines;
dy6 = (cy-cy)/numLines;
//Q4
dx7 = (cx-cx)/numLines;
dy7 = (cy-0)/numLines;
dx8 = (0-cy)/numLines;
dy8 = (cy-cy)/numLines;
}
function draw() {
//Q1.1
var x1 = width/2;
var y1 = 0;
var x2 = width/2;
var y2 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke("lightblue")
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
//Q2.1
var x3 = width/2;
var y3 = height;
var x4 = width/2;
var y4 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke("pink")
line(x3, y3, x4, y4);
x3 += dx3;
y3 += dy3;
x4 += dx4;
y4 += dy4;
}
//Q2.2
var x3 = width;
var y3 = height/2;
var x4 = width/2;
var y4 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke("white")
line(x3, y3, x4, y4);
x3 += dx3;
y3 += dy3;
x4 += dx4;
y4 += dy4;
}
//Q2.3
var x3 = 0;
var y3 = height/2;
var x4 = width/2;
var y4 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke("grey")
line(x3, y3, x4, y4);
x3 += dx3;
y3 += dy3;
x4 += dx4;
y4 += dy4;
}
//Q3.1
var x5 = width/2;
var y5 = height;
var x6 = width/2;
var y6 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke("lightgreen")
line(x5, y5, x6, y6);
x5 += dx5;
y5 += dy5;
x6 += dx6;
y6 += dy6;
}
//Q3.2
var x5 = 0;
var y5 = height/2;
var x6 = width/2;
var y6 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke("white")
line(x5, y5, x6, y6);
x5 += dx5;
y5 += dy5;
x6 += dx6;
y6 += dy6;
}
//Q3.3
var x5 = width;
var y5 = height/2;
var x6 = width/2;
var y6 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke("grey")
line(x5, y5, x6, y6);
x5 += dx5;
y5 += dy5;
x6 += dx6;
y6 += dy6;
}
//Q4.1
var x7 = width/2;
var y7 = 0;
var x8 = width/2;
var y8 = height/2;
for (var i = 0; i <= numLines; i += 1) {
stroke(224,199,166)
line(x7, y7, x8, y8);
x7 += dx7;
y7 += dy7;
x8 += dx8;
y8 += dy8;
}
noLoop();
}