For my image, I noticed that the background lights were blurry, and I wanted to implement that in the code with mousPressesed. So each time you use you press the mouse, a filter is applied to the whole canvas and the whole canvas gets a bit blurry. Additionally, I wanted there to be a difference in shapes for the background and the person.
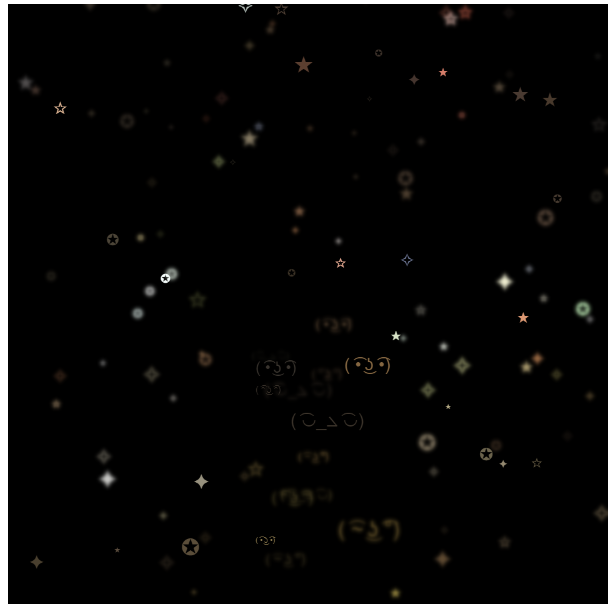
Small Blur
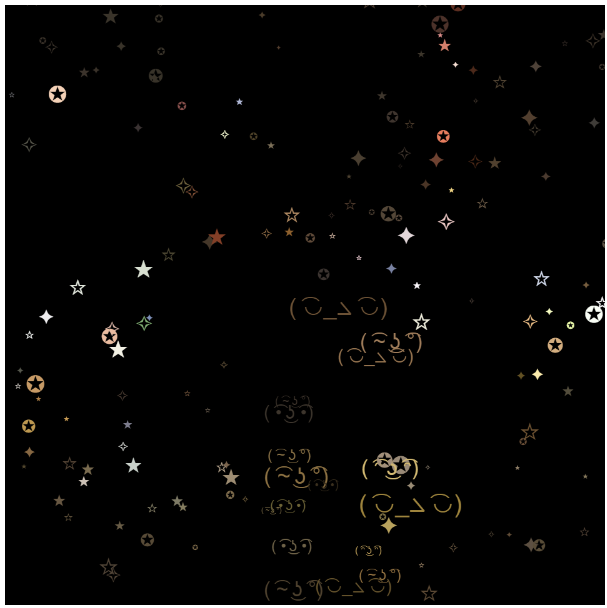
No Blur
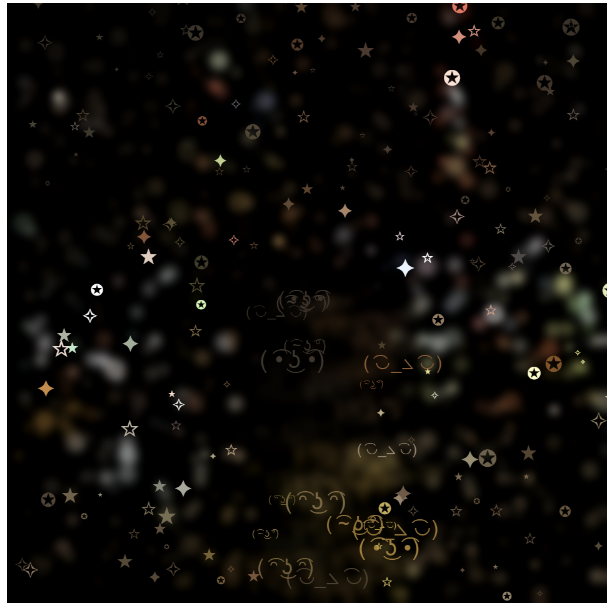
Medium Blur
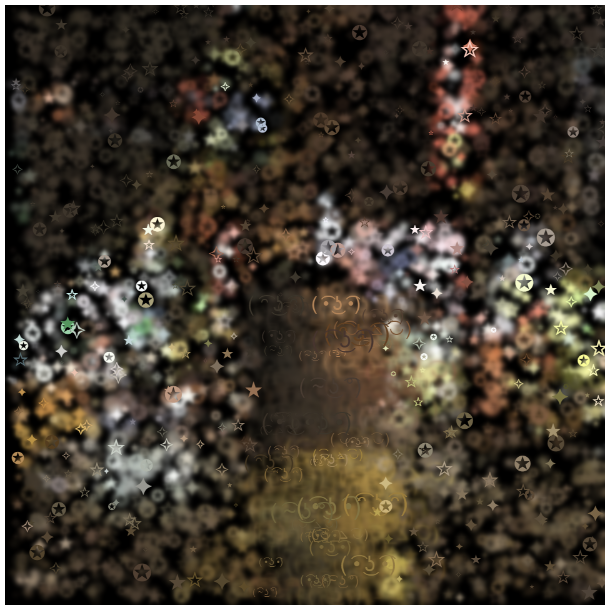
Lots of Blur
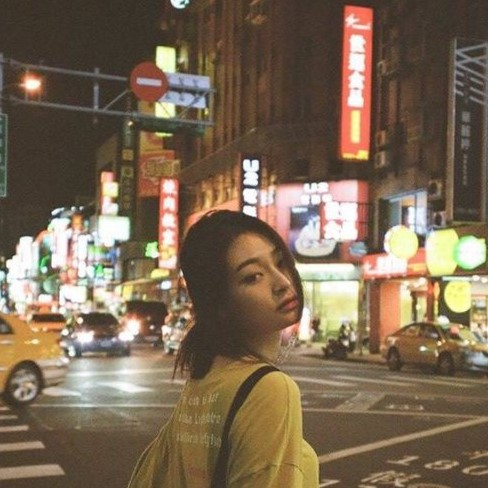
Image
// Your name: Ashley Su
// Your andrew ID: ashleysu
// Your section (C):
var img;
var stars = [" ★ ", " ☆ "," ✪ "," ✦ ", " ✧ "]
var faces = ['( ͡• ͜ʖ ͡•)', '( ͡ᵔ ͜ʖ ͡ᵔ)', '( ͡~ ͜ʖ ͡°)', '( ͡◡_⦣ ͡◡)']
function preload() {
img = loadImage("https://i.imgur.com/spqIDsb.jpeg")
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
img.loadPixels();
img.resize(480, 480); //resize image to canvas
frameRate(50000);
background(0);
}
function draw() {
//pick random x and y
var x = floor(random(img.width));
var y = floor(random(img.height));
//pull pixel color from that x and y
var pixelColor = img.get(x, y);
//fill using the pixel color
fill(pixelColor);
if (x>((width/2)-50) & x<((width/2)+50) && y>height/2 && y < height) { //setting the faces smilies to be next where the actaul face is
strokeWeight(5);
textSize(random(5, 20)); // smaller near face
text(random(faces), x, y);
} else {
textSize(random(5, 20));
text(random(stars), x, y); //draws stars everywhere else
}
}
function mousePressed() {
filter(BLUR,3); // when mouse is pressed, it blurs the layer
}