For this portrait project, I chose to feature a picture I took of my youngest sister eating a donut.
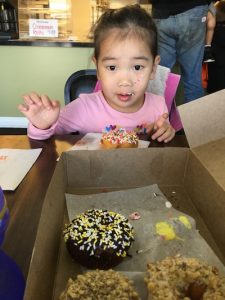
I wanted to play with varying shape and size of pixels, so I created a program that starts out with drawing large rings of the image, and then with each click of the mouse, the rings incrementally decrease in size (until a certain point). Additionally, in order to play with shape, rectangles instead of rings are drawn when the user moves the mouse across the canvas (and the rectangles also decrease in size as the mouse is clicked).
Below are two renditions of what the image could ultimately look like. Of course, there are many more possible images that could be generated, since the presence of rings or rectangles and their respective sizes are controlled by the user’s command of the mouse.
/*Emma Shi
Section B
eyshi@andrew.cmu.edu
Project 9
*/
var img;
var outlineWH = 30;
function preload(){
var imgURL = "http://i.imgur.com/8xc0iz2.jpg"
img = loadImage(imgURL);
}
function setup() {
createCanvas(400, 533);
background(0);
img.loadPixels();
}
function draw() {
var px = random(width);
var py = random(height);
var ix = constrain(floor(px), 0, width);
var iy = constrain(floor(py), 0, height);
var ColorXY = img.get(ix, iy);
stroke(ColorXY);
strokeWeight(2);
noFill();
ellipse(ix, iy, outlineWH, outlineWH);//draws circles that appear to make the image complete
rect(mouseX, mouseY, outlineWH, outlineWH);//draws squares according to mouse movement
}
function mousePressed() {
if (outlineWH == 30) {
outlineWH = 25;
} else if (outlineWH == 25) {
outlineWH = 20;
} else if (outlineWH == 20) {
outlineWH = 15;
} else if (outlineWH == 15) {
outlineWH = 10;
} else if (outlineWH == 10) {
outlineWH = 5;
}
//the size of the circles and squares decreases by 5 with each click
}