// Alec Albright
// aalbrigh@andrew.cmu.edu
// Section B
// Project 09
var original; // original image
var words; // bank of words to be displayed
function preload(){
// original image URL
var imageURL = "https://i.imgur.com/pfJvLUW.jpg";
// load original image
original = loadImage(imageURL);
// populating the words array
words = ["Years", "from", "now", "our", "past", "will", "be", "a", "story"];
}
function setup(){
createCanvas(384, 480);
// resize image to fit in constraints
original.resize(384, 480);
// load pixels
original.loadPixels();
background("black");
frameRate(20);
}
function draw(){
// initializing random places to place words
var px = random(width);
var py = random(height);
// to be used for finding the color from the original image
var ix = constrain(floor(px), 0, width-1);
var iy = constrain(floor(py), 0, height-1);
// getting color from original image
var theColorAtLocationXY = original.get(ix, iy);
// text size dependent on mouseX
textSize(map(mouseX, 0, 384, 8, 14));
// displaying words dependent on where they're placed
// top row
if(py < 160) {
// "years"
if(px < 128) {
fill(theColorAtLocationXY);
text(words[0], px, py);
// "from"
} else if(px >= 128 & px < 256) {
fill(theColorAtLocationXY);
text(words[1], px, py);
// "now"
} else {
fill(theColorAtLocationXY);
text(words[2], px, py);
}
// middle row
} else if(py >= 160 & py < 320) {
// "our"
if(px < 128) {
fill(theColorAtLocationXY);
text(words[3], px, py);
// "past"
} else if(px >= 128 & px < 256) {
fill(theColorAtLocationXY);
text(words[4], px, py);
// "will"
} else {
fill(theColorAtLocationXY);
text(words[5], px, py);
}
// bottom row
} else {
// "be"
if(px < 128) {
fill(theColorAtLocationXY);
text(words[6], px, py);
// "a"
} else if(px >= 128 & px < 256) {
fill(theColorAtLocationXY);
text(words[7], px, py);
// "story"
} else {
fill(theColorAtLocationXY);
text(words[8], px, py);
}
}
// fill in dots depending on where the mouse is
var mouseColor = original.get(mouseX, mouseY);
noStroke();
fill(mouseColor);
ellipse(mouseX, mouseY, 5);
}
In approaching this project, I immediately knew I wanted to create a portrait of my long-time girlfriend, displaying our favorite quote: “Years from now, our past will be a story”. I wasn’t quite sure how to go about displaying the quote, so I decided to section off areas of the canvas for each specific word so it read like a book from left to right and top to bottom. Once this was ironed out, the difficult part was implementing text size so that the quote was readable but also allowed for a decently quick creation of the image. Thus, I used this as an opportunity to implement a cool feature, so I mapped text size to the x coordinate of the mouse.
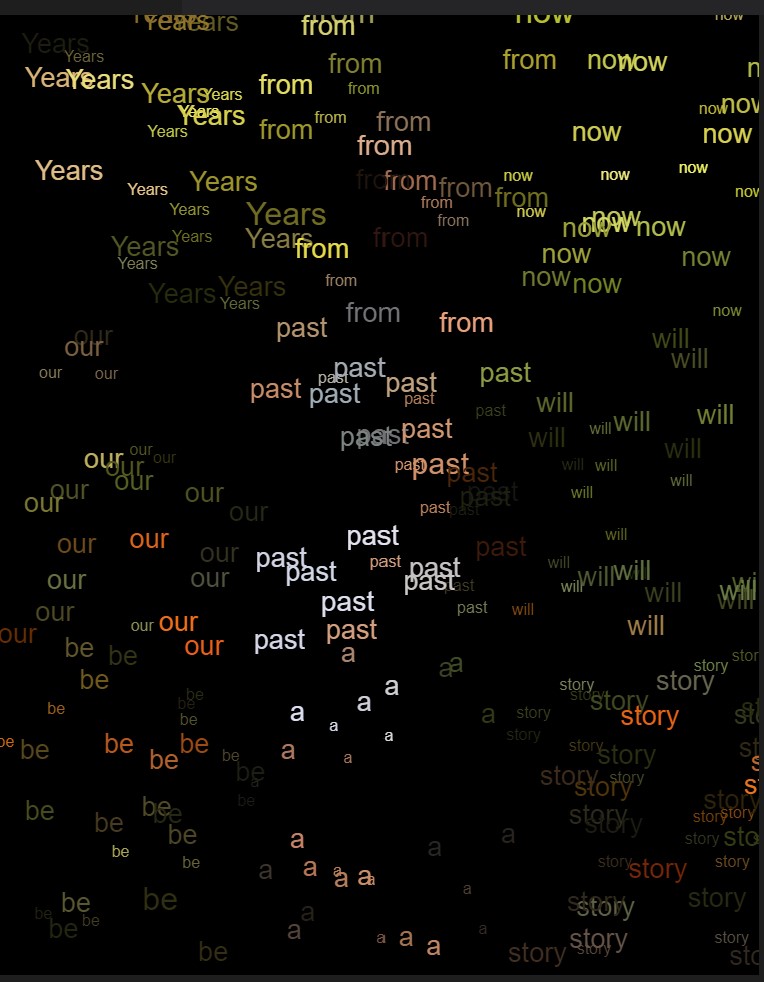
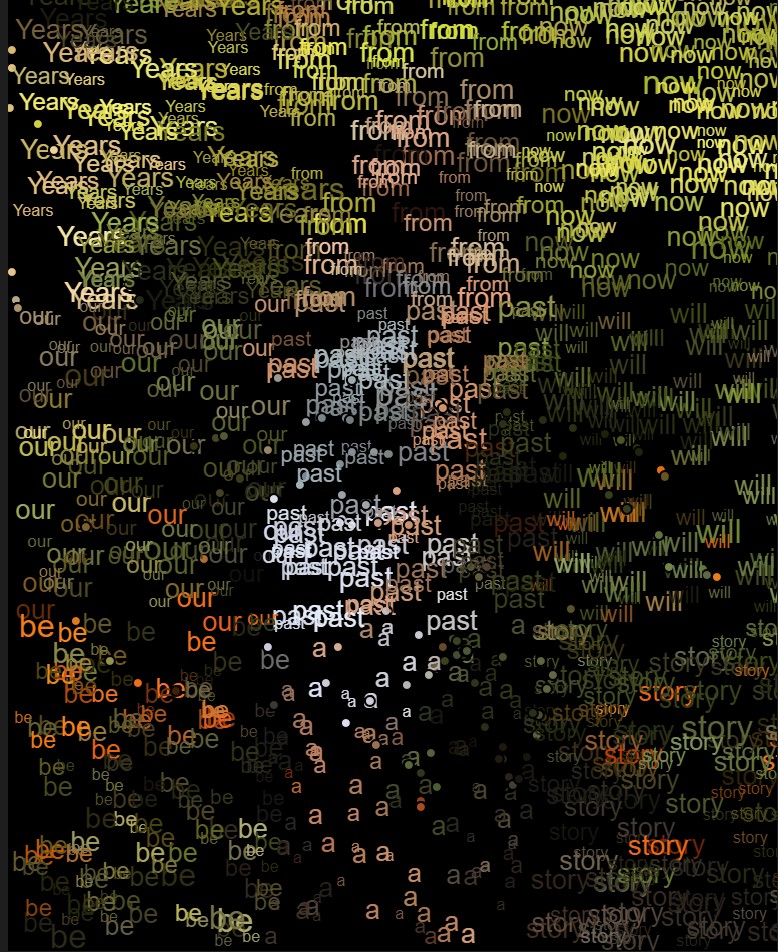
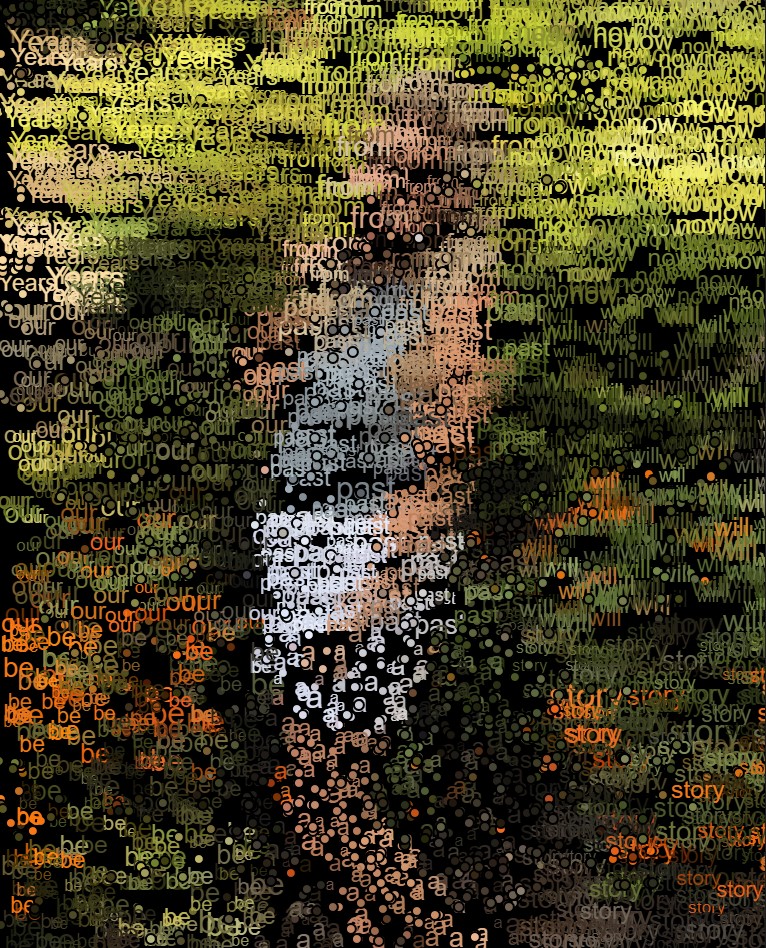
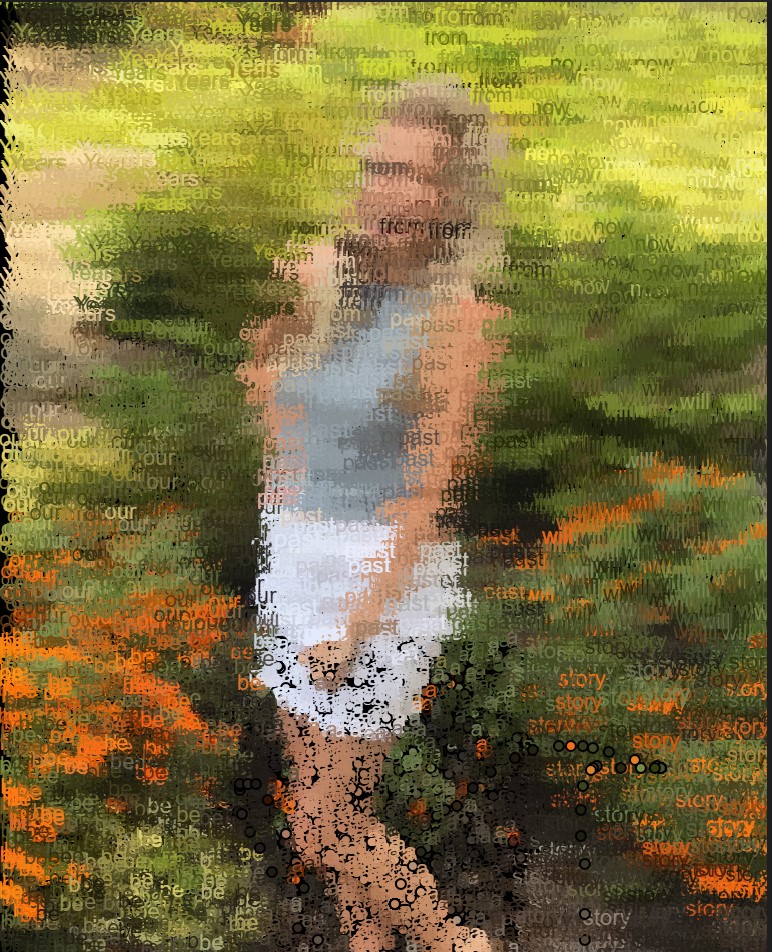
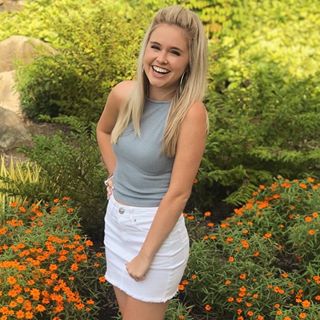