// Alec Albright
// aalbrigh@andrew.cmu.edu
// Section B
// Project 11
var time = 0; // time of day (by frame rate)
var timeRate = 1; // rate of time passing
var angle = 0; // rotation angle of sun/moon system
var angleRate = 180 / 255; // rate of change of the angle
var birdsX = []; // xcoords of birds on the screen
var birdsY = []; // ycoords of birds on the screen
var birdsSpeed = []; // speeds of all birds on the screen
var birdsColor = []; // colors of all birds on the screen
function setup() {
createCanvas(480, 400);
ellipseMode(CENTER);
frameRate(30);
angleMode(DEGREES);
// birds
for (var i = 0; i <= 30; i ++){
birdsSpeed.push(random(1, 10));
birdsX.push(600);
birdsY.push(random(70, 320));
birdsColor.push(color(random(0, 255), random(0, 255), random(0, 255)));
}
}
function draw() {
// managing time
time += timeRate;
if (time == 255) {
timeRate = -1;
} else if (time == 0) {
timeRate = 1;
}
// coloring sky
colorSky(time);
// drawing sun/moon
push();
translate(240, 250);
rotate(angle);
drawSun(0, 200);
drawMoon(0, -200);
pop();
angle -= angleRate
// ground
ground();
for (var i = 0; i < birdsY.length; i ++){
drawBird(birdsX[i], birdsY[i], birdsColor[i]);
birdsX[i] -= birdsSpeed[i];
if (birdsX[i] < -10) {
birdsX[i] = 600;
}
}
}
function drawSun(x, y) {
// draws sun
noStroke();
fill("yellow");
ellipse(x, y, 100);
}
function drawMoon(x, y) {
// draws moon
noStroke();
fill("grey");
ellipse(x, y, 70);
}
function colorSky(time) {
// draws the sky according to the time of day
var blue = time;
var green = map(time, 0, 255, 0, 204);
noStroke();
fill(0, green, blue);
rect(0, 0, width, height);
}
function drawBird(x, y, color) {
fill(color);
noStroke();
triangle(x, y, x - 3, y + 5, x, y + 5);
triangle(x + 7, y, x + 13, y - 7, x + 11, y)
rect(x, y, 15, 5);
triangle(x + 5, y, x + 8, y - 7, x + 8, y);
}
function ground() {
fill("darkgreen");
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * .005) + (millis() * .0005);
var y = map(noise(t), 0, 1, 150, 350);
vertex(x, y);
}
vertex(width + 100, height);
vertex(0, height);
endShape();
}
For this project, I wanted to depict something creative based in something very realistic. Thus, I came up with the idea of doing a rolling landscape that depicted the passing of days in a logical manner but featuring an assortment of randomly placed and colored birds. In this way, I am also able to emphasize the pop on the screen that the birds have in an interesting way.
The process was very difficult for me in automating everything correctly while still maintaining readability of code while debugging. Thankfully, however, I was able to get past that! Below are some initial sketches from my laptop as to what my first idea was.
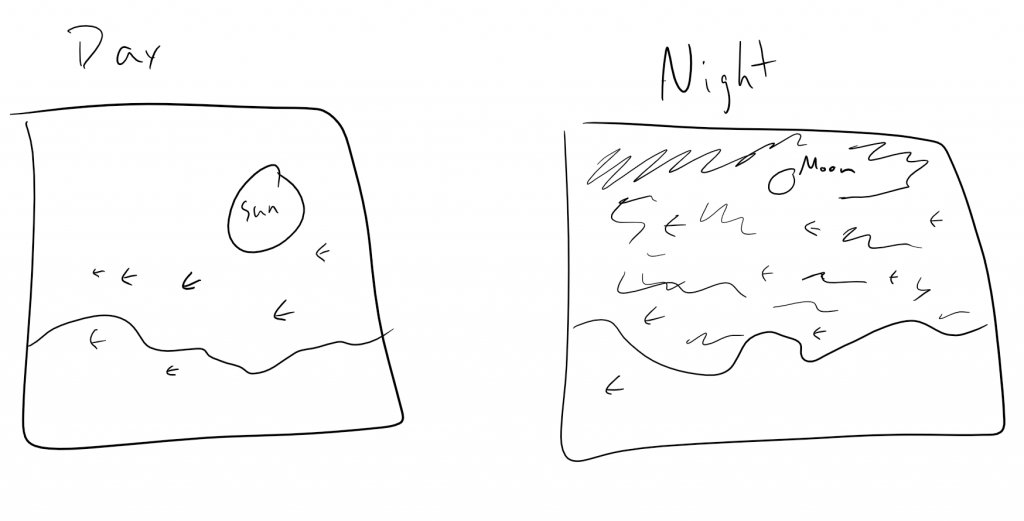