//Caroline Song
//chsong@andrew.cmu.edu
//Section E
//Project 05 - Wallpaper
var color1;
var color2;
function setup() {
createCanvas(600, 600);
//Defining colors
color1 = color(159, 233, 255);
color2 = color(1, 95, 124);
setGradient(color1, color2);
}
function draw() {
noFill();
stroke(255);
strokeWeight(1);
for (var y = 0; y <= 12; y++) {
if (y % 2 == 0) {
for(var x = 0; x <= width; x += 50) {
triangle(0 + x, 50+(y*50), 25 + x, 0+(y*50), 50 + x, 50+(y*50));
}
}
else {
for(var x = 0; x <= width; x += 50) {
triangle(-25 + x, 100+((y-1)*50), 0 + x, 50+((y-1)*50), 25 + x, 100+((y-1)*50));
}
}
}
}
function setGradient(c1, c2) {
//Creating blue gradient
for(var i = 0; i <= height; i++) {
var x1 = map(i, 0, height, 0, 1);
var c3 = lerpColor(color1, color2, x1);
stroke(c3);
line(0, i, width, i);
}
}
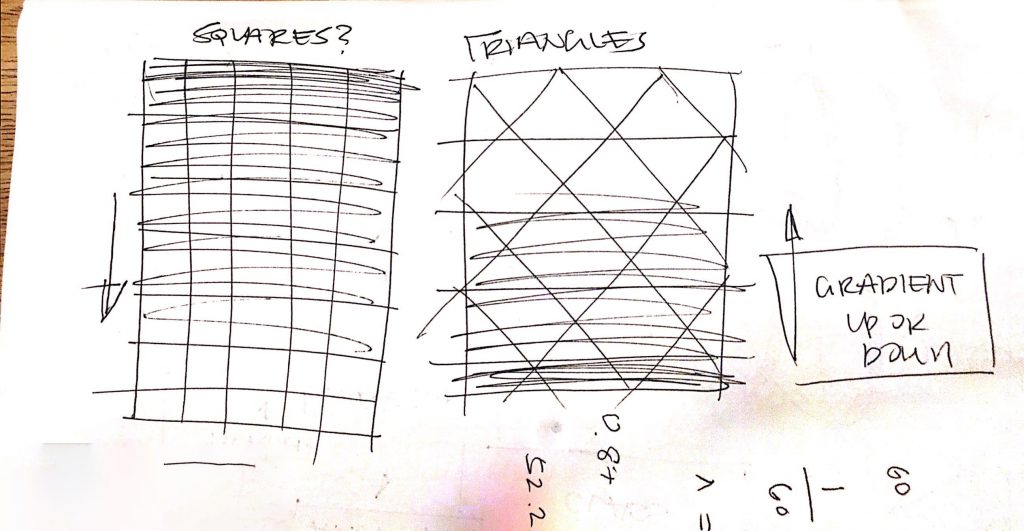
For this wallpaper, I wanted to play around with colors so that my finished product would resemble something from the ocean or the beach. And so, I created a soft blue gradient. Overlaying that, I made a repeating pattern of triangles in a white color in order to keep the soft color palette I was aiming for.