My week could be described with this emoji: 🤡, so I decided to lock myself in my apartment to code this as a coping mechanism.
I thought it was interesting that clowns are interpreted very differently depending on their context and appearance. I decided to take advantage of this project to demonstrate the unsettling feeling they give me. I was surprised to see that the incremental changes in color and size of the clown’s features actually increased the cursed factor significantly.
clown!
//global variables
var eyewhite = 42;
var eyeshadow = 60;
var pupil = 20;
var faceWidth = 315;
var faceHeight = 315;
var nose = 63;
var cheek = 58;
var eyebrow = 50;
var shoulderWidth = 480;
var mouthType = 1
//shirtcolor
var shirtR = 0;
var shirtG = 192;
var shirtB = 184;
//red default color
var r = 255;
var g = 5;
var b = 37;
//eyeshadowcolor
var shadowR = 97;
var shadowG = 116;
var shadowB = 255;
function setup() {
createCanvas(480, 640);
}
function draw() {
background(255, 160, 59);
var centerX = width / 2;
var centerY = height / 2;
//body
//shoulders
noStroke();
fill (shirtR, shirtG, shirtB);
rect(0, 480, shoulderWidth, 165, 90, 90, 0, 0);
//bottomneck
noStroke();
fill (255, 237, 219);
ellipse (centerX, 486, 144, 82);
//neck
noStroke();
fill (255, 237, 219);
rect (168, 419, 144, 66);
//buttons
noStroke();
fill (255, 250, 116);
ellipse (centerX, 557, 27, 27);
noStroke();
fill (255, 250, 116);
ellipse (centerX, 592, 27, 27);
noStroke();
fill (255, 250, 116);
ellipse (centerX, 626, 27, 27);
//face
noStroke();
fill(255, 245, 227);
ellipse ( centerX, centerY, faceWidth, faceHeight);
//cheeks
noStroke();
fill(255, 51, 86, 100);
ellipse (140, centerY, cheek, cheek);
noStroke();
fill(255, 51, 86, 100);
ellipse (333, centerY, cheek, cheek);
//nose
noStroke();
fill (r - 18, g, b); //darker red
ellipse (centerX, 320, nose, nose);
//eye
var eyeLX = 185;
var eyeRX = 291;
var eyeY = 271;
//eyeshadow
noStroke();
fill (shadowR, shadowG, shadowB);
ellipse (eyeLX, eyeY, eyeshadow);
noStroke();
fill (shadowR, shadowG, shadowB);
ellipse (eyeRX, eyeY, eyeshadow);
//eyewhite
noStroke ();
fill (255);
ellipse (eyeLX, eyeY, eyewhite);
noStroke ();
fill (255);
ellipse (eyeRX, eyeY, eyewhite);
//pupil
noStroke ();
fill (0);
ellipse (eyeLX, eyeY, pupil, pupil);
noStroke ();
fill (0);
ellipse (eyeRX, eyeY, pupil, pupil);
//mouth
var mouthLX = 170;
var mouthRX = 310;
var smileY = 352;
var neutralY = 374;
var frownY = 400;
//draw three different mouth expressions
if (mouthType ==1){
//smile
noFill();//lips
stroke(r, g, b);
strokeWeight(36);
beginShape ();
curveVertex( mouthLX, smileY)
curveVertex( mouthLX, smileY)
curveVertex( 195, 377)
curveVertex (centerX, 393)
curveVertex( width - 195, 377)
curveVertex( mouthRX, smileY)
curveVertex( mouthRX, smileY)
endShape();
noFill();//teeth
stroke(255);
strokeWeight(11);
beginShape ();
curveVertex( mouthLX, smileY)
curveVertex( mouthLX, smileY)
curveVertex( 195, 377)
curveVertex (centerX, 393)
curveVertex( width - 195, 377)
curveVertex( mouthRX, smileY)
curveVertex( mouthRX, smileY)
endShape();
} else if (mouthType ==2){
//neutral
noFill();//lips
stroke(r,g,b);
strokeWeight (36);
line (mouthLX, neutralY, mouthRX, neutralY);
noFill();//teeth
stroke(255);
strokeWeight (11);
line (mouthLX, neutralY, mouthRX, neutralY);
} else if (mouthType ==3){
//frown
noFill();//lips
stroke(r, g, b);
strokeWeight(36);
beginShape ();
curveVertex( mouthLX, frownY)
curveVertex( mouthLX, frownY)
curveVertex( 195, 370)
curveVertex( centerX, 359)
curveVertex( width - 195, 370)
curveVertex( mouthRX, frownY)
curveVertex( mouthRX, frownY)
endShape();
noFill();//teeth
stroke(255);
strokeWeight(11);
beginShape ();
curveVertex( mouthLX, frownY)
curveVertex( mouthLX, frownY)
curveVertex( 195, 370)
curveVertex( centerX, 359)
curveVertex( width - 195, 370)
curveVertex( mouthRX, frownY)
curveVertex( mouthRX, frownY)
endShape();
}
//hair
//left
noStroke();
fill(r,g,b);
ellipse (83, 260, 42);
ellipse (91, 230, 42);
ellipse (57, 209, 42);
ellipse (77, 203, 42);
ellipse (68, 167, 42);
ellipse (52, 147, 42);
ellipse (56, 209, 42);
ellipse (47, 182, 42);
ellipse (67, 235, 60);
ellipse (98, 198, 50);
ellipse (57, 125, 29);
//right
noStroke();
fill(r,g,b);
ellipse (width- 83, 260, 42);
ellipse (width- 91, 230, 42);
ellipse (width- 57, 209, 42);
ellipse (width- 77, 203, 42);
ellipse (width- 68, 167, 42);
ellipse (width- 52, 147, 42);
ellipse (width- 56, 209, 42);
ellipse (width- 47, 182, 42);
ellipse (width- 67, 235, 60);
ellipse (width- 98, 198, 50);
ellipse (width- 57, 125, 29);
}
function mousePressed() {
eyewhite = random (30, 60);
eyeshadow = random( 55, 70);
pupil = random (15, 30);
faceWidth = random(300, 330);
faceHeight = random(315, 380);
nose = random(45, 90);
cheek = random(55, 90);
mouthType = int(random(1,4));
//shirtcolor
shirtR = random(0, 255);
shirtG = random(0, 255);
shirtB = random(0, 255);
//eyeshadowcolor
shadowR = random(0, 255);
shadowG = random(0, 255);
}
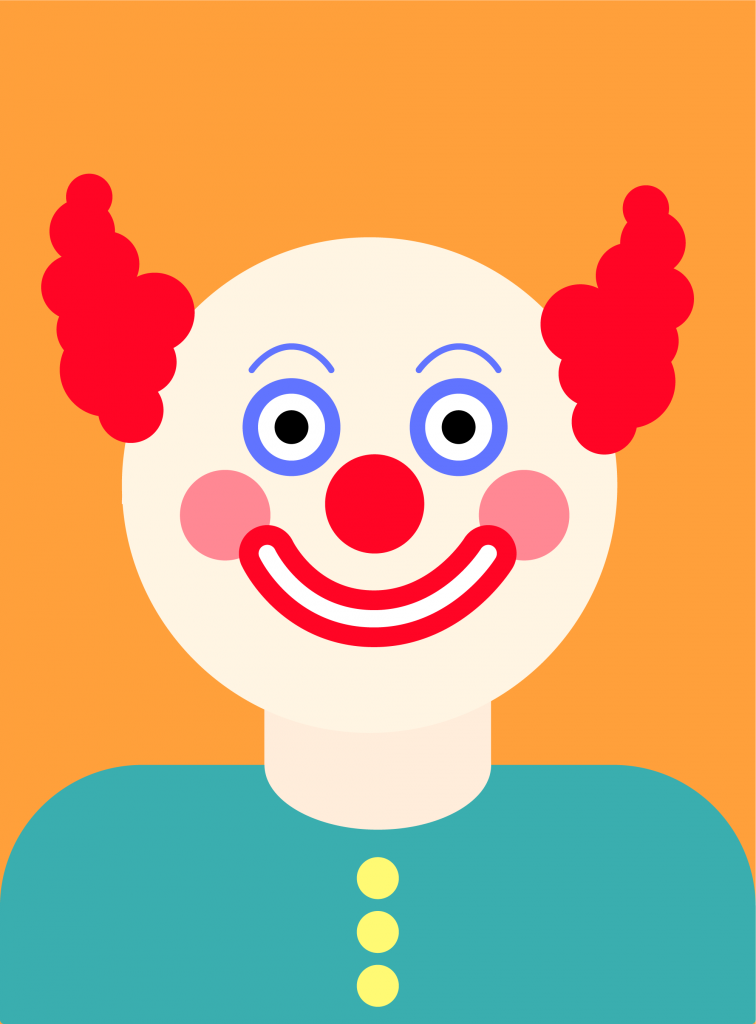