This wallpaper was inspired by a phone case I recently purchased online. Watermelons are one of my favorite fruits and green is my favorite color so I thought it’d be fun to code this design. To make it more dynamic, I decided to make the positions of the watermelons somewhat random and the placement of the seeds random inside so that each slice is unique.
sketch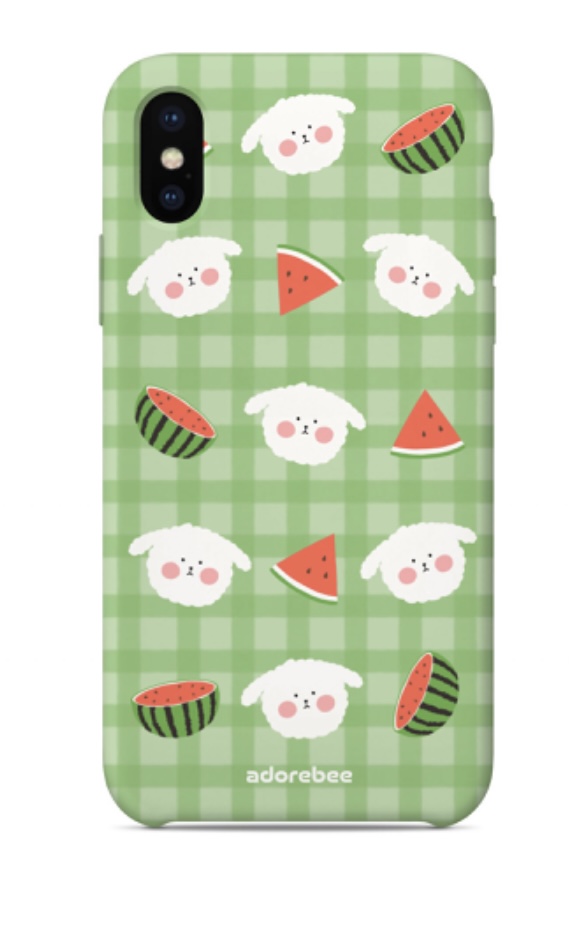
function setup() {
createCanvas(600, 400);
background(101, 173, 119); // green background
for(var x = 0; x <= width; x += 20){ // vertical stripes
stroke(136, 213, 156);
strokeWeight(5);
line(x, 0, x, height);
}
for(var y = 0; y <= height; y += 20){ // horizontal stripes
stroke(136, 213, 156);
strokeWeight(5);
line(0, y, width, y);
}
}
function watermelonHalf() { // draw watermelon halves
//semicircle
rotate(radians(random(-30, 30))); // random position
noStroke();
fill(38, 144, 65);
arc(0, 0, 60, 60, 0, PI); // green part
fill(255);
arc(0, 0, 55, 55, 0, PI); // white part
fill(236, 80, 102);
arc(0, 0, 50, 50, 0, PI); // red part
for(var seeds = 0; seeds <= 5; seeds += 1){ // random seed positions
fill(0);
ellipse(random(-20, 20), random(5, 15), 2, 3);
}
noLoop();
}
function watermelonSlice() { // draw watermelon slices
//triangular slice
rotate(radians(random(60, 90))); // random position
noStroke();
fill(38, 144, 65);
arc(0, 0, 70, 70, 0, PI/3); // green
fill(255);
arc(0, 0, 65, 65, 0, PI/3); // white
fill(236, 80, 102);
arc(0, 0, 60, 60, 0, PI/3); // red
for(var seeds = 0; seeds <= 2; seeds += 1){ // seeds
fill(0);
ellipse(random(15, 20), random(5, 20), 2, 3);
}
noLoop();
}
var column = 0; // column counter to alternate
function draw() {
for(var x = 30; x <= width; x += 80){
if(column%2 == 0){ // if odd number columns
for(var y = 0; y <= height; y += 120){ // first slice
push();
translate(x, y);
watermelonSlice();
pop();
}
for(var y = 60; y <= height; y += 120){ // second half
push();
translate(x, y);
watermelonHalf();
pop();
}
column += 1; // add to column counter
}
else{ // if even number columns
for(var y = 0; y <= height; y += 120){ // first half
push();
translate(x, y);
watermelonHalf();
pop();
}
for(var y = 60; y <= height; y += 120){ // second slice
push();
translate(x, y);
watermelonSlice();
pop();
}
column += 1; // add to counter
}
}
}