When creating this wallpaper, I kept thinking of the kitschy nautical patterns you’d find plastered on the walls of seaside restaurants. I drew a lot of inspiration from cute illustrations on Pinterest that emulate this niche aesthetic.
nautical
function setup() {
createCanvas(550, 550);
background(220);
bgColor = color(180, 218, 221);
floatyColor = color(215, 53, 76);
wheelColor = color(115, 175, 193);
}
function draw() {
background(bgColor);
//seashells
for(var c = -5; c < width; c += 93) {
for(var d = -5; d < height; d += 200) {
push();
translate(c, d);
seashell();
pop();
}
}
//seashells again
for(var e = -10; e < width; e += 95) {
for(var f = 95; f < height; f += 190) {
push();
translate(e, f);
seashell();
pop();
}
}
//wheel
for(var x = 45; x < width; x += 190) {
for(var y = 40; y < height; y += 200) {
push();
translate(x, y);
woodenWheel();
pop();
}
}
//flotation devices
for(var a = 5; a < width; a += 180) {
for(var b = 0; b < height; b += 180) {
push();
translate(a, b);
floaty();
pop();
}
}
}
//the blue & white steering wheels
function woodenWheel() {
fill(bgColor);
noStroke();
ellipse(38, 37, 45, 45);
handles = 8; //number of handles on the steering wheels
handleAngle = 360/handles; //angle between each handle
radius = 75/2; //length of each handle
strokeWeight(4);
stroke(wheelColor);
for (angle = 270; angle < 600; angle = angle + handleAngle) {
x = cos(radians(angle)) * radius;
y = sin(radians(angle)) * radius;
line(radius, radius, x + radius, y + radius);
}
noFill();
strokeWeight(5);
stroke(255);
ellipse(38, 37, 50, 50); //outer wheel
ellipse(38, 37, 10, 10); //inner spoke
}
function floaty() {
noFill();
strokeWeight(20);
stroke(255);
ellipse(0, 0, 75, 75);
noStroke();
fill(floatyColor);
arc(9, 9, 75, 75, 0, PI/2); //bottom right red
arc(-9, 9, 75, 75, HALF_PI, PI); //bottom left red
arc(-9, -9, 75, 75, PI, HALF_PI + PI); //top left red
arc(9, -9, 75, 75, HALF_PI + PI, 0); //top right red
fill(bgColor);
ellipse(0, 0, 55, 55);
}
function seashell() {
push();
noStroke();
fill(255);
arc(0, 0, 50, 50, PI + QUARTER_PI, QUARTER_PI - HALF_PI);
arc(0, -5, 25, 20, QUARTER_PI, PI - QUARTER_PI);
pop();
grooves = 4; //number of lines on seashell
grooveAngles = 60/grooves; //angle of lines on seashell
radius = 50/2; //length of each line
strokeWeight(1);
stroke(bgColor);
translate(-25, -25);
for (angle = 225; angle < 325; angle = angle + grooveAngles) {
x = cos(radians(angle)) * radius;
y = sin(radians(angle)) * radius;
line(radius, radius, x + radius, y + radius);
}
}

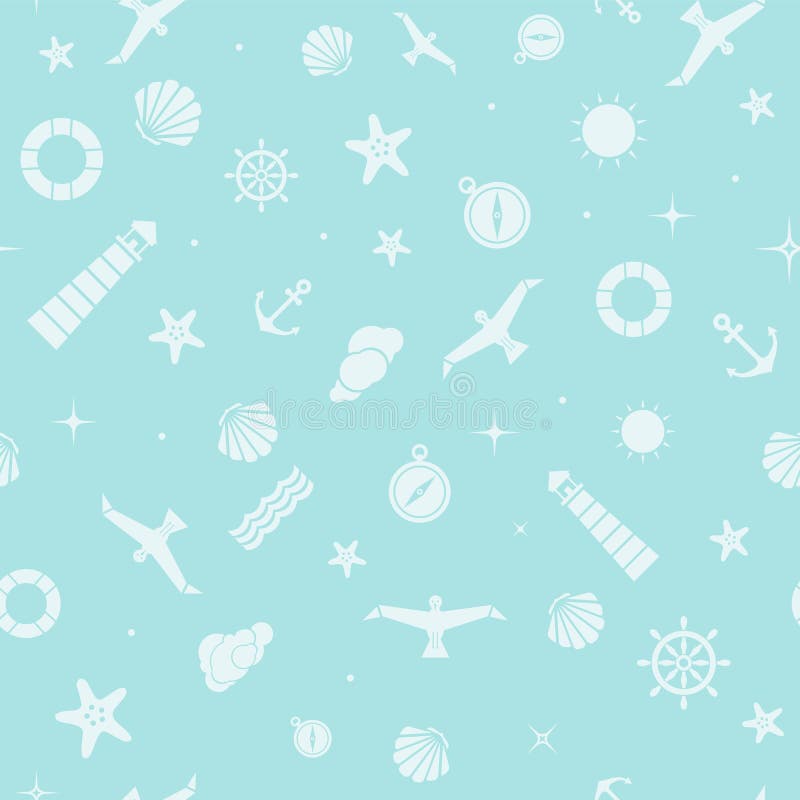