For my abstract clock I built it off the idea that we (or at least I), run on coffee. The day starts with a cup of coffee and is essentially continuously fueled by yet another cup. The clock begins with 12 empty cups on the screen that represent each hour in the AM or PM. Then with each minute – the coffee level increases in the cup until its filled, capped, and sleeved. Ticking along is a coffee bean counter that is representative of each second that goes by (the brewing/drip process) that helps fill the cup.
During the AM hours, the background represents a daytime sky with a sun. Then during PM hours, there is a night sky with a moon and randomly generated stars.
//Helen Cheng
//helenc1@andrew.cmu.edu
//Section C
//Project-06
var mugX = [];
var currH;
var starX = [];
var starY = [];
function setup() {
createCanvas(480, 300);
rectMode(CENTER);
//initializes array with num of cups
for (h=0; h<12; h++) {
mugX[h] = h*40 + 20
}
for (i=0; i< 20; i++) {
starX[i] = random(width);
starY[i] = random(height);
}
}
function draw() {
//colors sky based on AM or PM
if(hour()<12){
dayTime();
}
else{
nightTime();
}
//draws table
fill(235, 227, 218);
rect(240, 250, 480, 200);
//draws a coffee bean per second
for(s =0; s<second(); s++){
coffRow = Math.floor(s/20);
coffeeBean(45 + 20*(s-20*coffRow), 200 + 30*coffRow);
}
//draws coffee cups depicting hours
currH = hour()%12;
for (h=0; h<12; h++) {
if (h<currH){
fill(92, 48, 7);
strokeWeight(1);
rect(mugX[h], height/2, 30, 50);
strokeWeight(0);
ellipse(mugX[h], height/2 + 25, 30, 10);
//cap
fill(191, 155, 187);
rect(mugX[h], height/2-25, 35, 10);
ellipse(mugX[h], height/2-30, 25, 10);
//cup sleeve
rect(mugX[h], height/2, 35, 20);
}
else{
fill(240, 234, 223);
strokeWeight(1);
rect(mugX[h], height/2, 30, 50);
arc(mugX[h], height/2 + 25, 30, 10, 0, PI);
}
}
//fills coffee cup per minute
for (m=0; m<minute(); m++) {
strokeWeight(0);
fill(92, 48, 7);
ellipse(hour()%12*40 + 20, (height/2+25)-(m*50/60), 30, 10);
}
}
function coffeeBean(x,y){
strokeWeight(2);
fill(92, 48, 7);
ellipse(x, y, 10, 20);
line(x, y-10, x, y+10);
}
function nightTime() {
background(35, 41, 94);
fill(242, 242, 233);
circle(width/3, height/5, 30);
for (i=0; i< 20; i++) {
circle(starX[i], starY[i], 5);
}
}
function dayTime() {
background(152, 210, 237);
fill(245, 205, 103);
circle(width/3, height/5, 40);
}
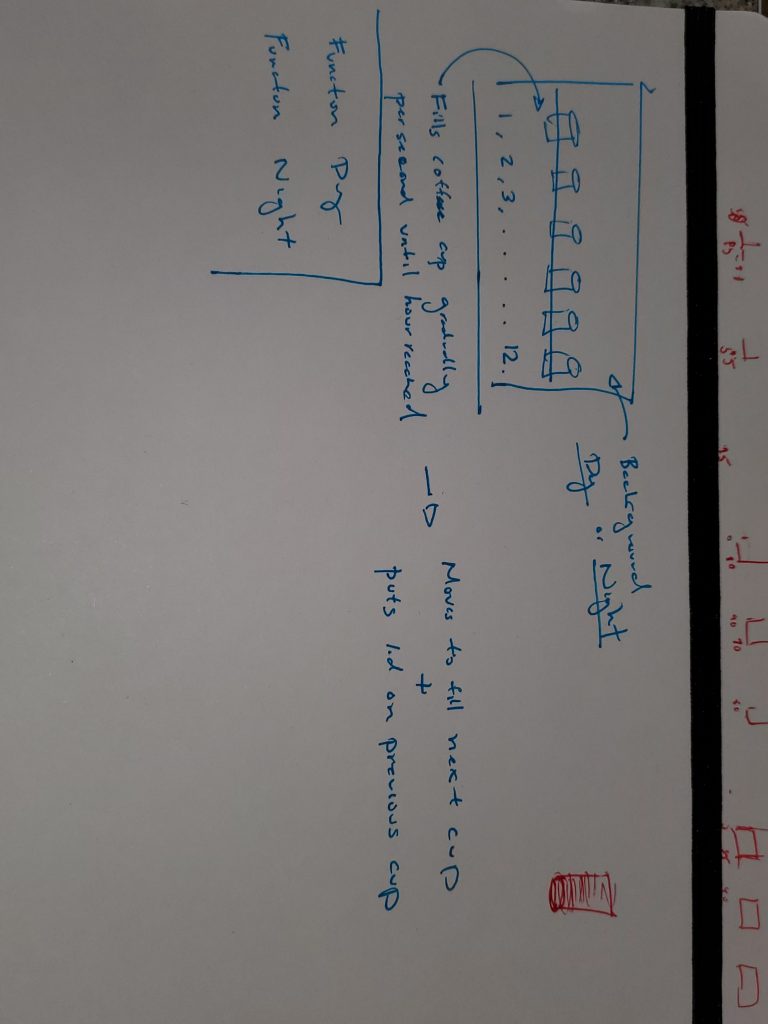