/*
* Eric Zhao
* ezhao2@andrew.cmu.edu
*
* Abstract clock that represents time indirectly
* through proximity to base units (for ex. if a minute
* is close to elapsing). The background color indicates
* the approximate hour and time of day.
*
*/
var x;
var y ;
var thetaSec;
var thetaMin;
var secondRad = 75;
var minuteRad = 175;
var speedMult; //rate of acceleration
var baseSpeed = 2; //speed of circles at [time unit] = 0;
function setup() {
thetaSec = -PI;
thetaMin = -PI;
thetaHour = -PI;
createCanvas(600, 600);
background(220);
frameRate(60);
}
function draw() {
//draws the revolving circles and background
push();
translate(300, 300);
BG();
secondHand();
minuteHand();
pop();
}
function secondHand(){
/*the "hand" is the inner circle that exponentially
revolves faster as the seconds count grows
closer to a minute exactly, then resets to the
base speed when the seconds count goes back to zero. */
speedMult = pow(second(), 1.75) / 150;
x = secondRad * cos(radians(thetaSec));
y = secondRad * sin(radians(thetaSec));
circle(x, y, 50);
thetaSec += baseSpeed + speedMult;
print(speedMult + baseSpeed);
}
function minuteHand(){
//see comment in secondHand(), works the same but by min/hour.
speedMult = pow(minute(), 1.75) / 200;
x = minuteRad * cos(radians(thetaMin));
y = minuteRad * sin(radians(thetaMin));
circle(x, y, 50);
thetaMin += baseSpeed + speedMult;
print(speedMult + baseSpeed);
}
function BG(){
//draws a background with a fill color proportional to time.
let fillR = map(hour(), 0, 11, 30, 255);
let fillG = map(hour(), 0, 11, 45, 235);
let fillB = map(hour(), 0, 11, 70, 150);
if(hour() >= 12){
fillR = map(24-hour(), 1, 12, 30, 255);
fillG = map(24-hour(), 1, 12, 45, 235);
fillB = map(24-hour(), 1, 12, 70, 150);
}
background(fillR, fillG, fillB);
/*Fill conditions:
*Goes from dark blue to pale yellow if 0 < hour < 12
*Goes from pale yellow to dark blue if 12 >= hour > 24
*/
if(fillR > 190) {
stroke(39, 58, 115);
fill(39, 58, 115, 65);
} else {
stroke(255);
fill(255, 65);
}
//changes stroke to dark/light based on brightness of background
circle(0, 0, secondRad*2);
circle(0, 0, minuteRad*2);
}
I was inspired by mob spawners in Minecraft when making this clock. The mob models in spawners spin faster and faster until a mob spawns from it, then it slows down and starts speeding up again until the next mob spawns.
This clock shows how close the next minute is to passing and how soon the next hour will pass, rather than displaying the exact time. The circles rotating the center speed up exponentially as the time nears the next unit. The outer circle represents minutes/hour and the inner one represents seconds/minute. The background also changes color across a gradient every hour (see code comments).
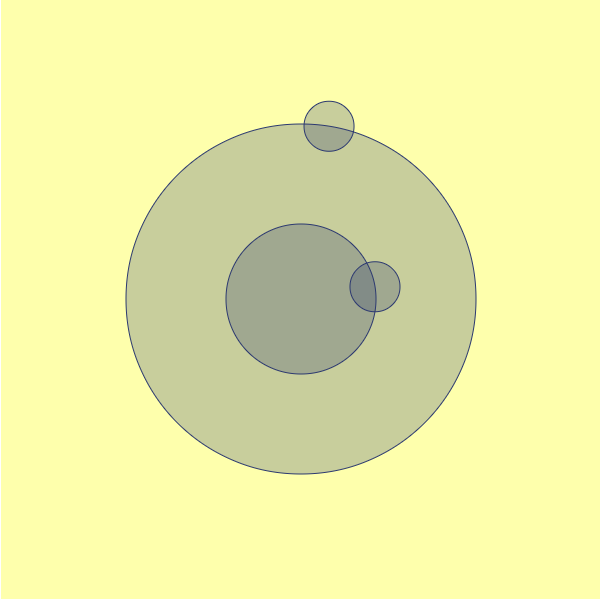
Daytime hours
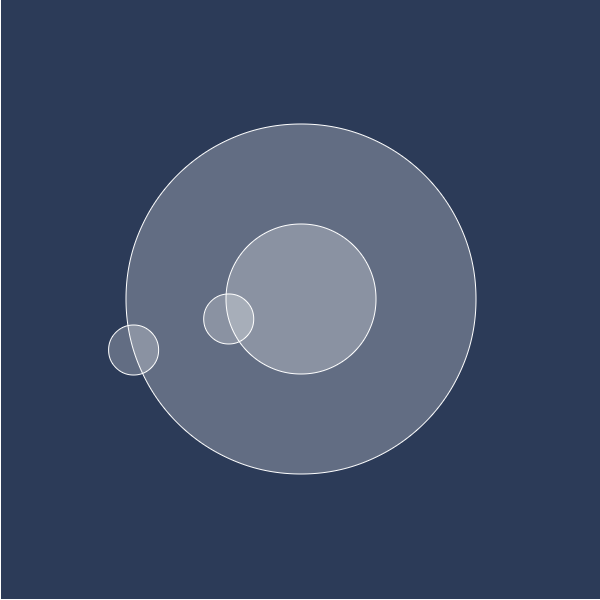