sketchDownload
/*
Nicholas Wong
Section A
*/
var d; //Days
var h; //Hours
var m; //Minutes
var s; //Seconds
var angle; //Angle for sin-wave frequency for pulse
function setup()
{
createCanvas(480,480);
angleMode(DEGREES); //Degrees instead of radians (makes mapping easier)
}
function draw()
{
translate (width/2,height/2) //Make origin at center
rotate(-90) //Rotate -90 so starting points for most circle counters face up
background(0); //Black background
//Day circle (Outer circle)
dayCircle();
//Detail circles
push();
fill(0)
strokeWeight(1)
stroke(250,0,0);
circle(0,0,200);
pop();
//Dark red circles
push();
noFill();
stroke(1)
stroke(50,0,0)
circle(0,0)
circle(0,0,65)
circle(0,0,80);
circle(0,0,100);
circle(0,0,150);
pop();
//Hour circle (Second outer ring)
hourCircle();
//Minute circle (Tertiary rings)
minuteCircle();
//Second circle (Dotted inner ring)
secondCircle();
//Center-most pusling circle
secondPulse();
angle += (360/60) / 2; //1 period is 360/framerate, half makes 1 pulse/s
}
//Circle of 31 arcs
function dayCircle()
{
var d = day();
//Loop runs 31 times, the maximum number of days for any month
for (let i=1; i<=31; i++)
{
push();
noStroke();
fill(50,0,0)
arc(0,0,250,250,(i-1)*360/31,(i-1)*360/31 + 10.5); //Make 31 arcs
//If the day is today, make the arc red
if(i == d)
{
fill(250,0,0)
}
else //If not today, make arc dark red
{
fill(70,0,0)
}
//First day of month is slightly brighter red arc
if(i==1)
{
fill(90,0,0)
}
arc(0,0,235,235,(i-1)*360/31,(i-1)*360/31 + 10.5); //Outer arc for aesthetics
pop();
//Additional circles for aesthetics
noFill();
stroke(255,0,0);
circle(0,0,250);
circle(0,0,200);
}
}
//Circle with 24 segments
function hourCircle()
{
var h = hour();
//Loop is run for the amount of hours passed today
for (let i=0; i<=h; i++)
{
push();
noFill();
stroke(200,0,0);
strokeWeight(3)
arc(0,0,150,150,i*15,i*15 + 6); //Add arcs for every hour that has passed today
strokeWeight(0.35)
push(0);
rotate(-90)
line(75*sin((-i*15)),75*cos((-i*15)),100*sin((-i*15)),100*cos((-i*15))) //Line drawn from arcs for aesthetics
pop();
stroke(255)
strokeWeight(1)
arc(0,0,140,140,i*15,i*15 + 6); //Secondary arc for aesthetics
pop();
}
}
//Circle of variable arc lengths that show minutes in an hour
function minuteCircle()
{
var m = minute();
let m_circle = map(m,0,60,0,360); //Map minutes in an hour to angles on a circle
push();
noFill();
strokeWeight(2);
stroke(200,0,0);
//Creates an arc with arc length representing how many minutes has passed in this hour
arc(0,0,100,100,0,m_circle);
strokeWeight(2);
stroke(250,100,100);
arc(0,0,80,80,0,m_circle); //Aeshtetic secondary arc
pop();
}
//Circle of 60 segments that show seconds in a minute
function secondCircle()
{
var s = second();
//Loop runs for the amount of seconds passed in this minute
for (i=0; i<=s; i++)
{
push();
noFill();
stroke(255,150,150);
strokeWeight(1)
arc(0,0,65,65,i*6,i*6 + 1); //Creates an arc for every second that has passed in this minute
pop();
}
}
//Circles in center that pulse every second
function secondPulse()
{
if (frameCount/60 == 1) //If the frame count is divisible by 60 (is a second)
{
angle = 180; //Angle is set to 180
}
let s_diam = sin(angle) * 40; //Pulse maximum diameter
push();
noFill();
strokeWeight(1)
stroke(250,150,150)
circle(0,0,s_diam); //Draw circle with variable diameter
stroke(250,250,250)
circle(0,0,s_diam * 0.75); //Second circle for aesthetics
pop();
}
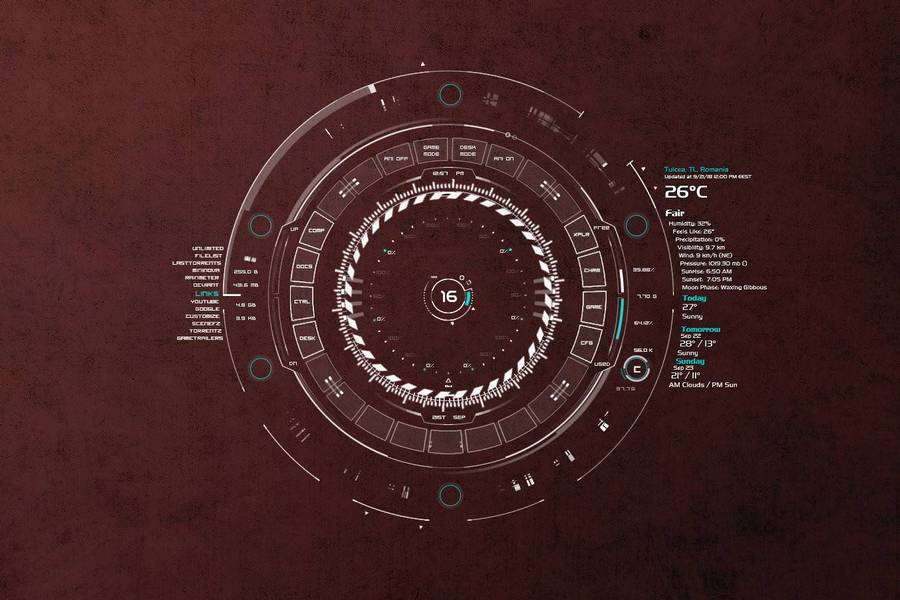
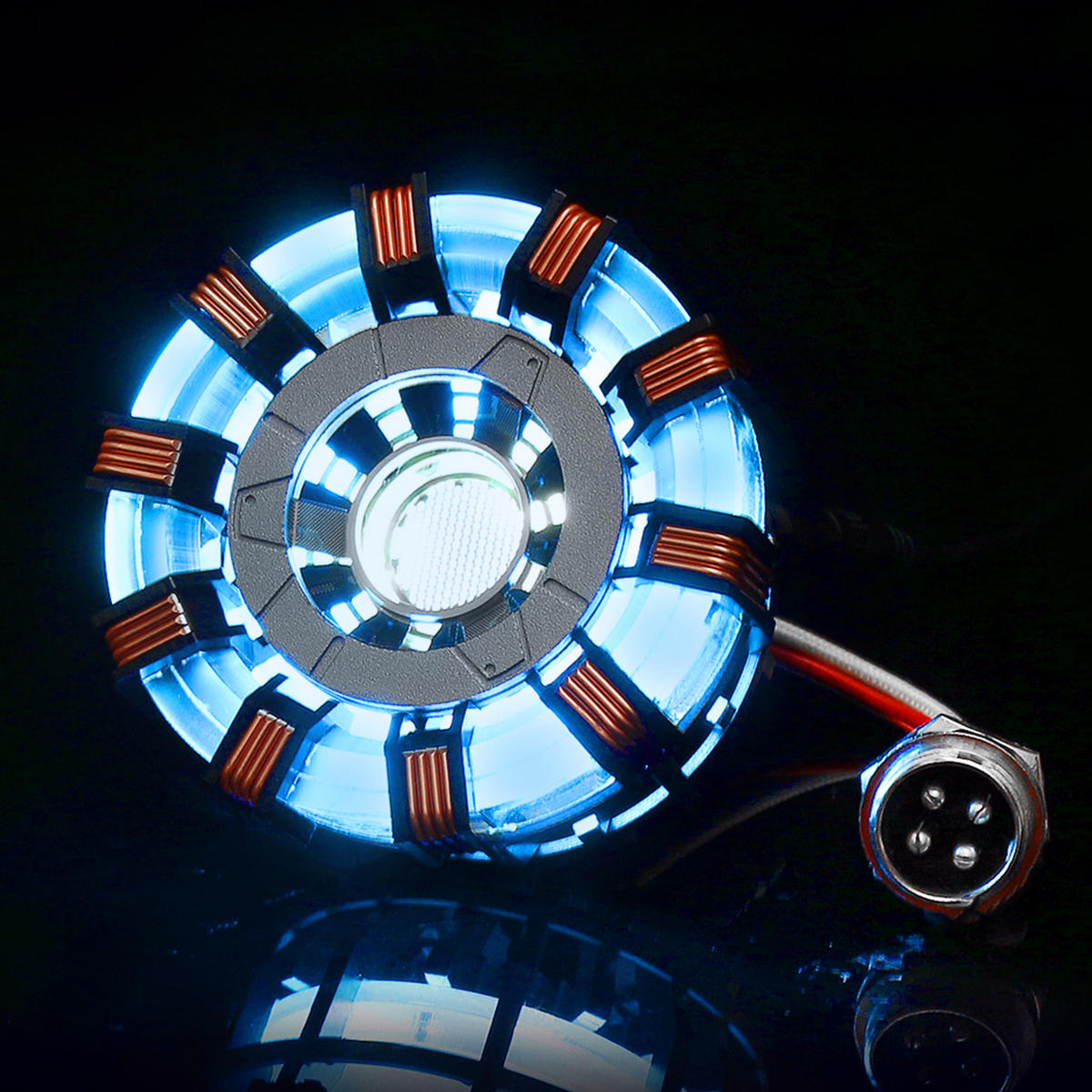
Inspired by tech stuff. Mostly Iron Man, I just re-watched Endgame.