I began this project in a very different place than where I ended up – after exploring many different ways of how I could potentially represent the CMU fence as a clock, I ditched that idea and went with creating a binary clock.
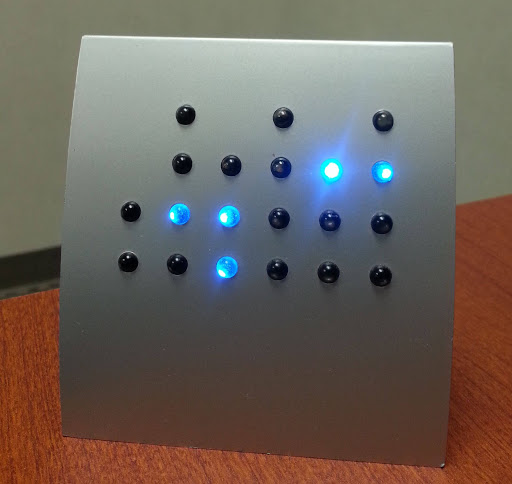
When I was a kid, my dad had a clock like this. Although I could never read it, it looked fancy and important to me and made me feel like maybe one day I would deserve to know what it all meant. It wasn’t until this project that I found out how to read it!
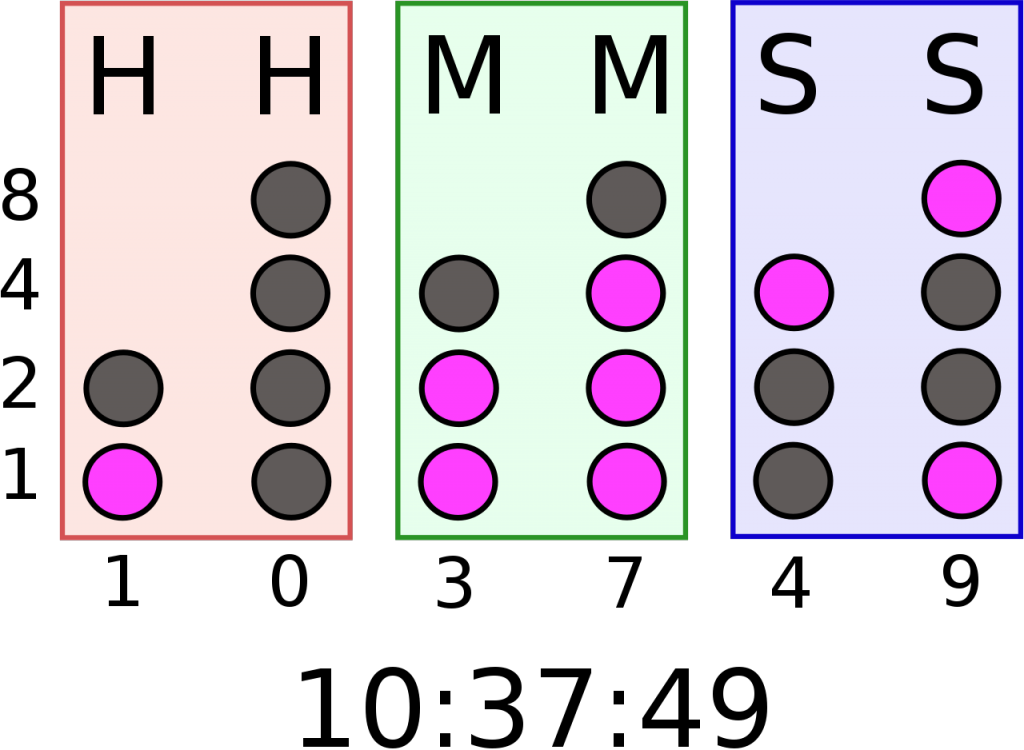
My dad told me that the clock always looked like a cityscape to him. I agreed, so naturally that is what I chose to code for this project.
// Assignment 06 - PROJECT – ABSTRACT CLOCK
// Name: Jubbies Steinweh-Adler
// Email: jsteinwe@andrew.cmu.edu
// Class: Section D
var lightOn;
var lightOff;
var column = [2, 4, 3, 4, 3, 4];
var canvasMargin = 100;
var innerMargins = 10;
function setup() {
createCanvas(480, 480);
//WINDOW COLORS
lightOn = color(180, 180, 0);
lightOff = color(70, 70, 40);
}
function draw() {
background(27, 27, 128); //Blue
var timeString = whatTime(); // run whatTime function once and rename output
var cLength = column.length;
rWidth = (width - 2 * canvasMargin) / cLength;
//- BACKGROUND-
//moon
fill(248, 243, 200);
circle(width * (2 / 3), height * (1 / 3), 250);
//buildings
//back
fill(5);
rect(0, height / 2, width, height);
rect(60, height / 2 - 40, 100, 200);
rect(width - 100, height / 2 - 40, 80, 200);
//front
fill(20);
rect(105, 130, 145, 235, 0, 80, 0);
rect(262, 173, 127, 190);
fill(50);
rect(202, 220, 132, 146);
rect(248, 173, 44, 61);
rect(264, 148, 12, 28);
//reflected buildings
push();
translate(0, 200);
fill(20);
rect(105, 165, 145, 235);
rect(262, 165, 127, 190);
fill(50);
rect(202, 165, 132, 146);
pop();
//- TIME FORMATTING -
//CONVERT TIME STRING TO BINARY STRING
for (var i = 0; i < cLength; i++) { //run 6 times
var rx = canvasMargin + rWidth * (i + 0.5);
var binary = floor(timeString[i]).toString(2); //converts time to binary string
//test proper binary conversion
print("a = " + binary.toString());
//REVERSING BINARY DATA
//positional data for drawing depends on reversal
binary = binary.split("");
binary = reverse(binary);
binary = binary.join("");
//test proper binary reversal
print("b = " + binary.toString());
//-DRAWING CLOCK ELEMENTS -
for (var p = 0; p < column[i]; p++) {
var ry = (height - 50) - ((rWidth * p) + canvasMargin);
var reflectY = height - canvasMargin + (p * rWidth);
var rectWidth = rWidth - (1.8 * innerMargins);
//FILL WINDOW WITH CORRECT COLOR
//if character in pth position is 1, turn on
if (binary.charAt(p) === '1') {
fill(lightOn);
//if not, turn off
} else {
fill(lightOff);
}
//WINDOW SHAPE
rect(rx, ry, rectWidth / 2, rectWidth * (3 / 4));
// - FOREGROUND -
//Water reflection
rect(rx, reflectY, rectWidth / 2, rectWidth * (3 / 4));
fill(40, 40, 120, 30);
noStroke();
rect(0, height - 115, width, height);
}
}
}
function whatTime() {
//creates live time string with no spaces
var h = hour();
if (h > 9) { // if hour value greater than 9, return as is
hrs = h.toString();
} else { //if less than 9, add zero as spacer digit
hrs = "0" + h.toString();
}
var m = minute();
if (m > 9) {
mins = m.toString();
} else {
mins = "0" + m.toString();
}
var s = second();
if (s > 9) {
secs = s.toString();
} else {
secs = "0" + s.toString();
}
//FUNCTION OUTPUT - (hhmmss)
return hrs + mins + secs;
}
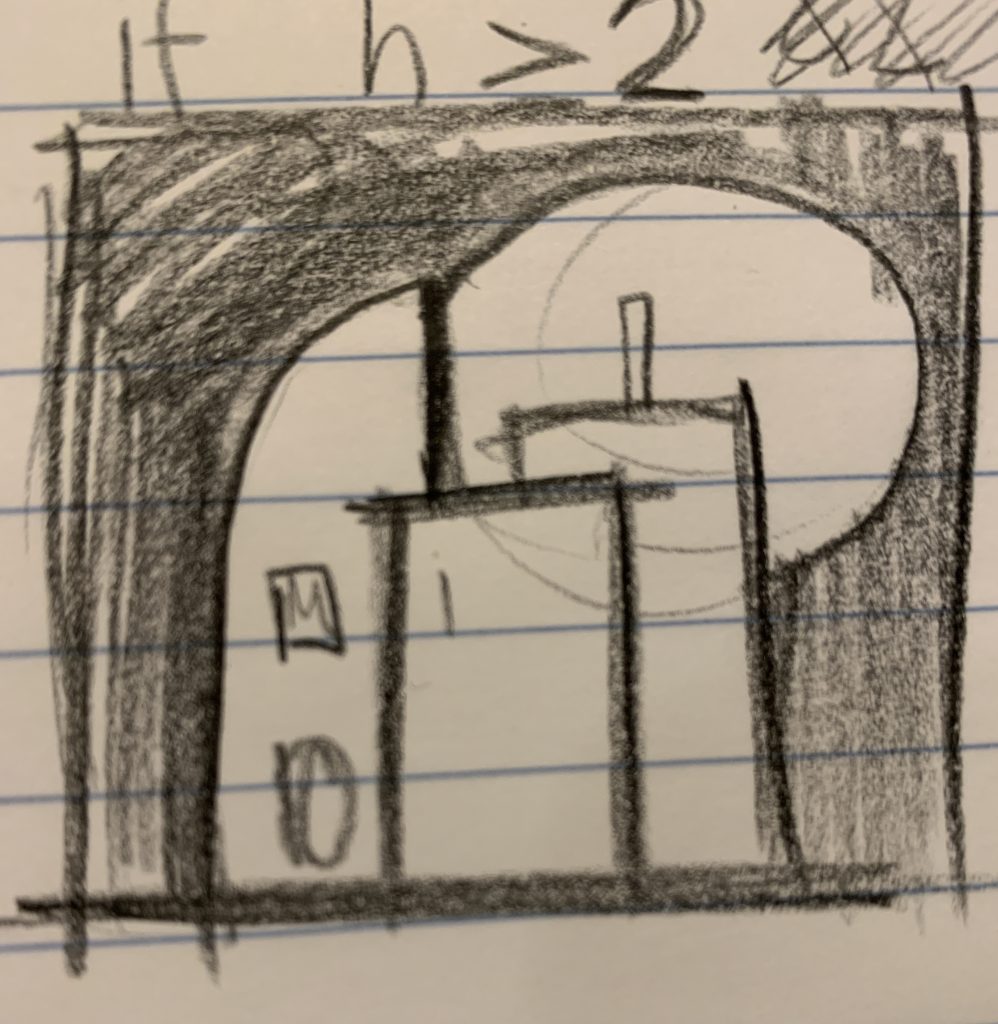