I really wanted to create a psychedelic piece with curves. To do this, I made a rainbow effect of colors. The main aspect of the art was a hypotrochoid. This is made with a fixed circle and lines created by another circle rolling along the edge. The piece changes different mathematical aspects of the hypotrochoids based upon the position of the mouse. The curve is created with parametric equations based upon the radius of the fixed circle and the radius of the moving circle. These are the elements that are adjusted with the mouse. The loops created many hypotrochoids and in certain positions on the canvas, this can make very interesting designs.
function setup() {
createCanvas(480, 480);
background(220);
frameRate(20);
}
function draw() {
background(0);
//moves the shapes to the center of the canvas
translate(width/2, height/2);
for(var i = 0; i<20; i++){
stroke(random(255), random(255), random(255)); //randomizes colors
noFill();
//creates loop of hypotrochoids based upon mouse position
hypotrochoid(i*mouseX*.5, i*mouseY);
}
}
//defines the shape hypotrochoid
//https://mathworld.wolfram.com/Hypotrochoid.html
function hypotrochoid(a, mouseY){
var x; //used for parametric form
var y; //used for parametric form
var a; //radius of the fixed circle
var b = a/10 //radius of the rolling ball
var h = constrain(mouseY/10, 0, a) //var h depends on mouseY and restricted
//defines the actual shape
beginShape();
for (var i = 0; i < 200; i++){
var t = map(i, 0, 600, 0, 6*PI);
x = (a-b)*cos(t)+h*cos(((a-b)/b)*t); //parametric equation for x
y = (a-b)*sin(t)-h*sin(((a-b)/b)*t); //parametric equation for y
vertex(x,y);
}
endShape(CLOSE);
}
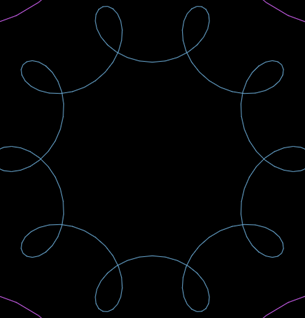
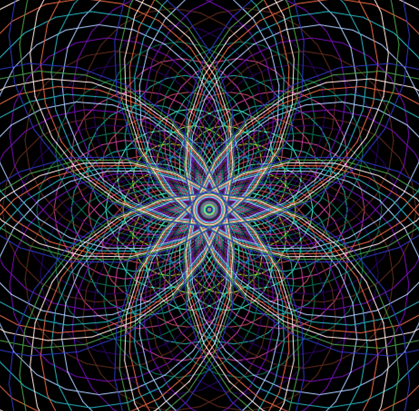