I decided to go with a butterfly curve because I thought it looked cool. Because of the name, I decided to make my project a butterfly flying in the sky. For the background, I just reused my clouds that I made for last week’s deliverables. Instead of having them move depending on what minute it was, I just set a constant to add to the x values of the ellipses the clouds were made of.
butterflyDownload
var translateX;
var translateY;
var nPoints=1000;
var butterfly=true;
var cloudScale=[0.5, 0.75, 1, 1.25, 1.5];
var x=0;
var dx=0.1;
function setup() {
createCanvas(480, 480);
translateX=width/2;
translateY=height/2;
}
function draw() {
background(222, 245, 253);
drawClouds();
push();
translate(translateX, translateY);
drawButterfly();
pop();
}
function drawButterfly(){
//Butterfly curve https://mathworld.wolfram.com/ButterflyCurve.html
//this draws a butterfly curve to make the butterfly
beginShape();
scale(50);
strokeWeight(0.02);
stroke(255, 200);
var x;
var y;
var angle=radians(map(mouseY, 0, height, 0, 360)); //rotation depends on mouseY
rotate(angle);
var m=map(mouseX, 0, width, 0, 25); //the size/extent of the curve of the butterfly depends on mouseX
for (var i=0; i<nPoints; i++){
var t=map(i, 0, nPoints, 0, m*TWO_PI); //var m is multiplied times the upper limit of the map function
x=sin(t)*(exp(cos(t))-2*cos(4*t)+pow(sin((1/12)*t), 5));
y=cos(t)*(exp(cos(t))-2*cos(4*t)+pow(sin((1/12)*t), 5));
vertex(x, y);
fill(228, 198, 245, 150);
}
endShape();
}
function mousePressed(){
//changes position depending on where you click
translateX=mouseX;
translateY=mouseY;
}
function drawClouds(){
//smallest cloud--cloud 1
push();
fill(250, 250);
noStroke();
scale(cloudScale[0]);
translate(500,100);
cloud();
pop();
//cloud 2
push();
fill(250, 250);
noStroke();
scale(cloudScale[1]);
translate(100, 250);
cloud();
pop();
//cloud 3
push();
fill(250, 250);
noStroke();
scale(cloudScale[2]);
translate(400, 350);
cloud();
pop();
//cloud 4
push();
fill(250, 250);
noStroke();
scale(cloudScale[3]);
translate(0, 50);
cloud();
pop();
//cloud 5
push();
fill(250, 250);
noStroke();
scale(cloudScale[4]);
translate(-50, 250);
cloud();
pop();
}
function cloud(){
ellipse(x+30, 10, 60, 40);
ellipse(x+40, 40, 90, 50);
ellipse(x, 40, 100, 50);
ellipse(x, 15, 55, 35);
x=x+dx;
//once the clouds go off the screen, they reappear on the other side
if(x>width){
x=0;
}
}
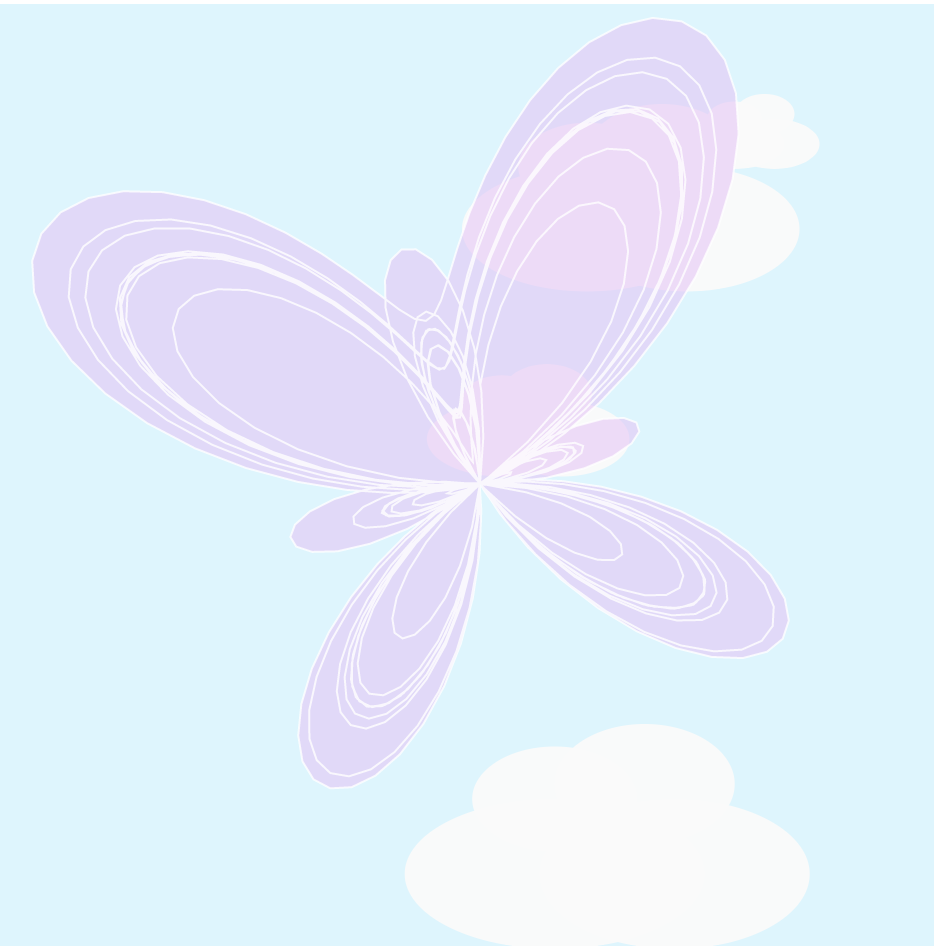
It rotates depending on where mouseY is and also draws the curve as mouseX goes from left to right.
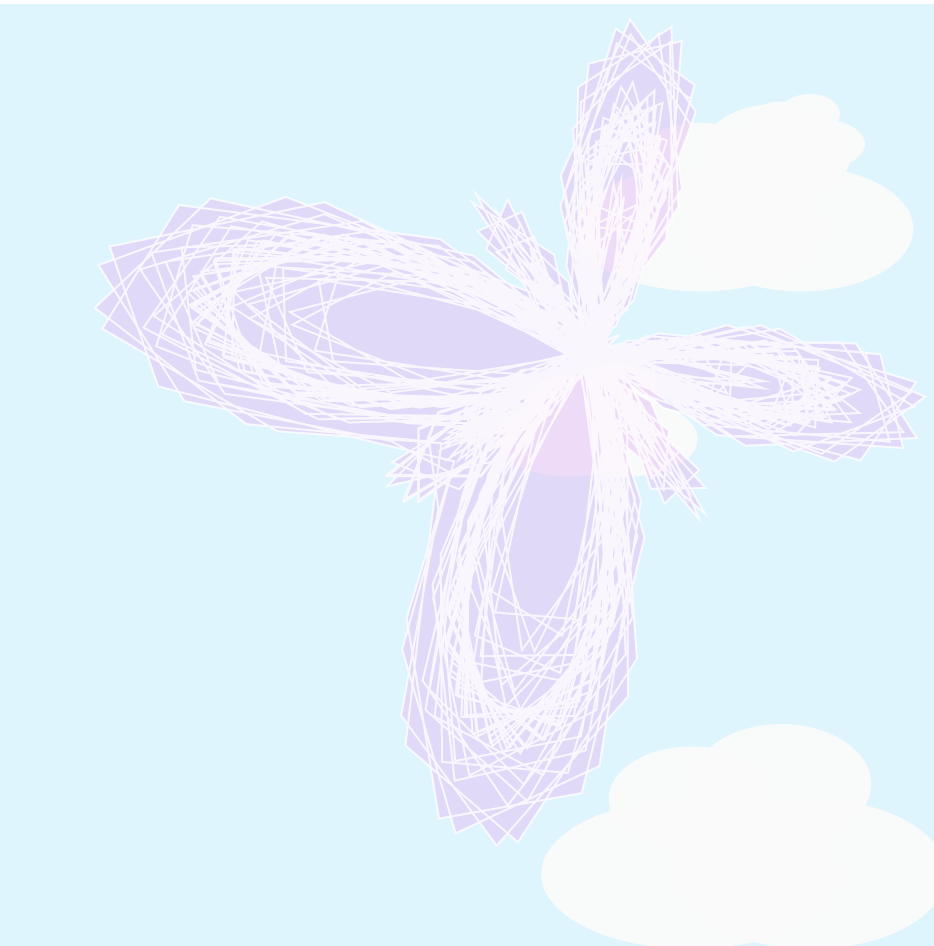
You can also click to change the origin of the image and move the butterfly around.