For this week’s project, I decided to use 2 different curves that reminded me of flowers, a 10 cusp Epicycloid and a 10 cusp Hypotrochoid. I decided to make them rotate and change in size based on the mouse movements but in opposite directions to give off a pulsating effect. I also utilized mousePressed so that the curves can alternate. The mouse positions also control the colors of the curves and the background so that the final output is unique in all positions. The colors of the flowers and background are quite muted to give off a slight vintage wallpaper vibe with a modern twist.
(below are screenshots of the code at different mouse positions)
sketch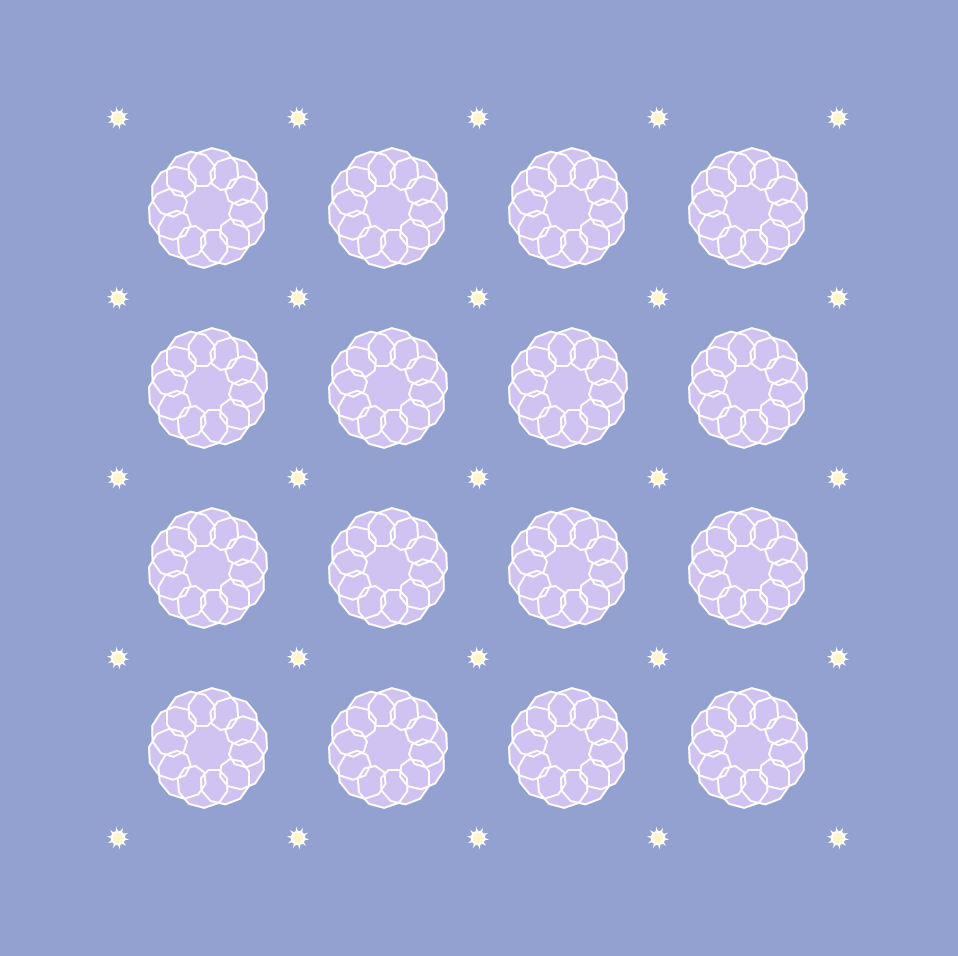
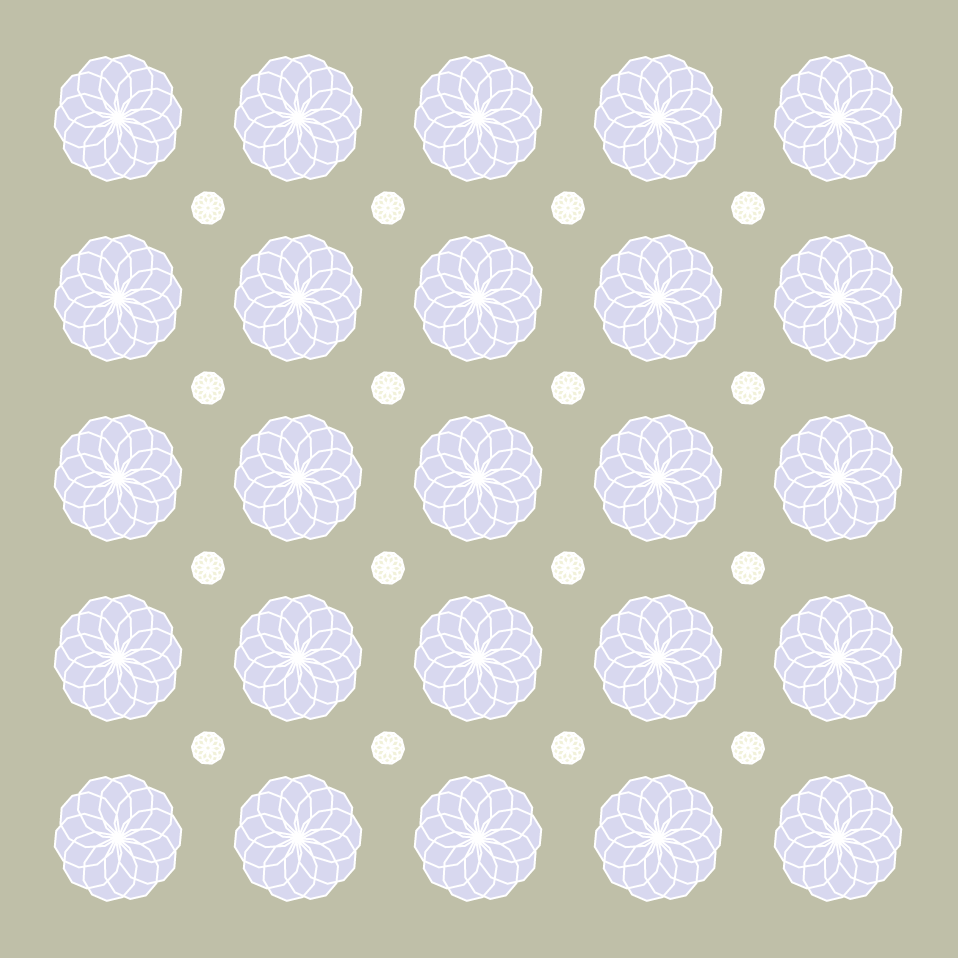
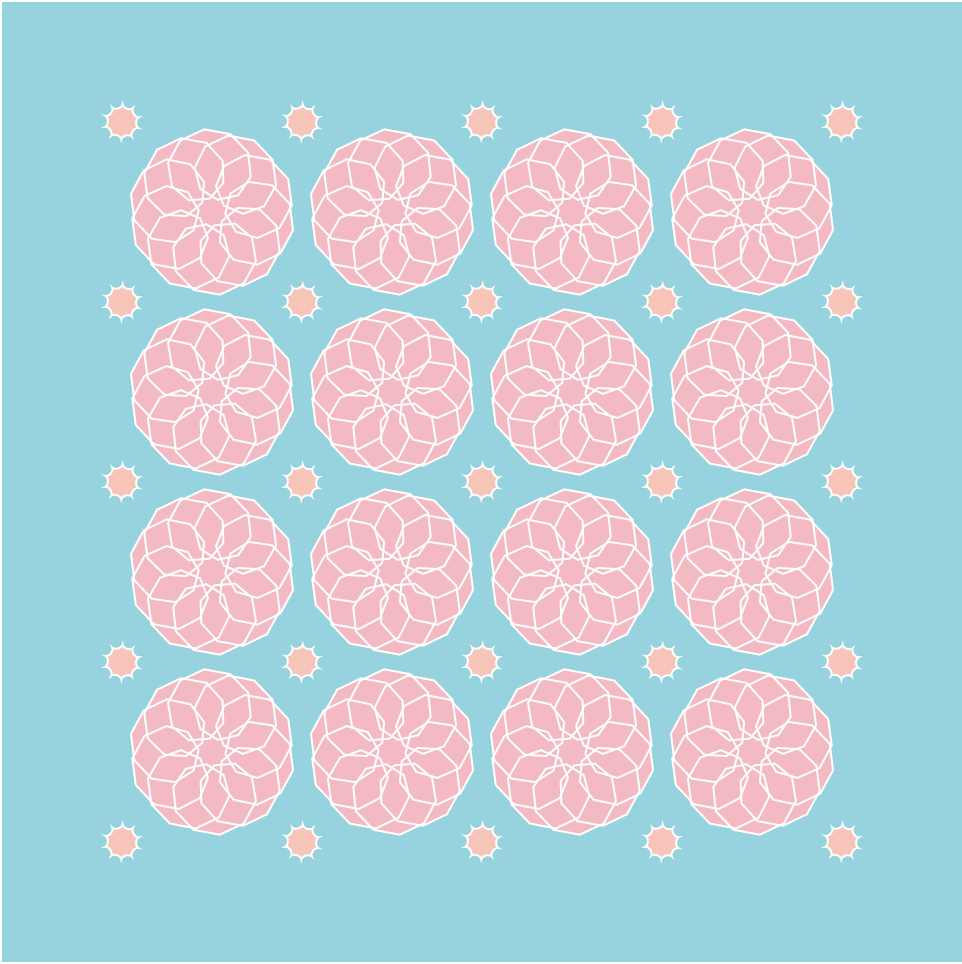
function setup() {
createCanvas(480, 480);
}
var nPoints = 100;
// background color codes
var r = 205;
var g = 152;
var b = 152;
// to switch curves based on mouse click
var whichCurve = 0;
// curve color codes
var curver = 202;
var curveg = 255;
var curveb = 255;
function draw() {
// to add/subtract color codes based on mouse position
var colordiffX = map(mouseX, 0, 480, 0, 53);
var colordiffY = map(mouseY, 0, 480, 0, 53);
background(r - colordiffX, g + colordiffY, b + colordiffX);
stroke(255); // white outline
// 5 x 5 curves (outer)
for(var x = 60; x < width; x += 90){
for(var y = 60; y < height; y += 90){
push();
translate(x, y);
fill(curver + colordiffX, curveg - colordiffY, curveb - colordiffX);
if(whichCurve == 0) {
drawHypotrochoid();
}
else {
drawEpicycloid();
}
pop();
}
}
// 4 x 4 curves (inner)
for(var x = 105; x < width - 90; x += 90) {
for(var y = 105; y < height - 90; y += 90) {
push();
translate(x, y);
fill(curver + colordiffY, curveg - colordiffX, curveb - colordiffY);
if(whichCurve == 1) {
drawHypotrochoid();
}
else {
drawEpicycloid();
}
pop();
}
}
}
// draw rotating 10 cusp epicycloid
function drawEpicycloid() {
var x;
var y;
var a = mouseX / 30;
var b = a / 10;
var h = constrain(mouseY / 8, a / 10, 8*b);
var ph = mouseX / 50;
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (a + b) * cos(t) - h * cos(ph + t * (a + b) / b);
y = (a + b) * sin(t) - h * sin(ph + t * (a + b) / b);
vertex(x, y);
}
endShape(CLOSE);
}
// draw rotating 10 cusp hypotrochoid
function drawHypotrochoid() {
var x;
var y;
var a = (width - mouseX)/20; // opposite movement from epicycloid
var b = a / 10;
var h = constrain((height - mouseY)/8, a/10, 10*b);
var ph = (width - mouseX) / 50;
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (a - b) * cos(t) + h * cos(ph + t * (a - b) / b);
y = (a - b) * sin(t) - h * sin(ph + t * (a - b) / b);
vertex(x, y);
}
endShape(CLOSE);
}
// mouse pressed alternates order of curves
function mousePressed() {
if(whichCurve == 0){
whichCurve = 1;
}
else {
whichCurve = 0;
}
}