curves cb
//global variables
var nPoints = 1000;
var angle = 0;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(mouseY, 100, mouseX); //change background color
//draw 2 sets of 36 hypotrochoids
for (var x = 80; x <= 400; x += 64) {
for (var y = 80; y <= 400; y += 64) {
push();
translate(x, y);
drawHypotrochoid1();
drawHypotrochoid2();
pop();
}
}
}
function drawHypotrochoid1() {
var a = map(mouseX, 0, 480, 0, 80); //radius of outside circle
var b = map(mouseX, 0, 480, 0, 20); //radius of inside circle
var h = map(mouseY, 0, 480, 0, 40); //point distance from center of inner circle
strokeWeight(.5);
stroke(mouseX, mouseY, 100); //change stroke color
noFill();
beginShape(); //create hypotrochoid
for (var i=0; i<nPoints; i++) {
var angle = map(i, 0, 100, 0, TWO_PI);
x = (a-b) * cos(angle) + h * cos(((a-b)/b) * angle);
y = (a-b) * sin(angle) - h * sin(((a-b)/b) * angle);
vertex(x, y);
}
endShape();
}
function drawHypotrochoid2() {
var a = map(mouseX, 0, 480, 2, 80); //radius of outside circle
var b = map(mouseX, 0, 480, 2, 20); //radius of inside circle
var h = map(mouseY, 0, 480, 2, 40); //point distance from center of inner circle
strokeWeight(.35);
stroke(mouseX, mouseX, mouseY); //change stroke color
noFill();
beginShape(); //create hypotrochoid
for (var i=0; i<nPoints; i++) {
var angle = map(i, 0, 100, 0, TWO_PI);
x = (a-b) * cos(angle) + h * cos(((a-b)/b) * angle);
y = (a-b) * sin(angle) - h * sin(((a-b)/b) * angle);
vertex(x, y);
}
endShape();
}
After exploring the WolframMathWorld site, I found hypotrochoids and thought that they were very dynamic and interesting. I decided to create my project with two sets of overlapping hypotrochoids to create a field of growing flower-like shapes. To make the composition interactive, I coded the mouse position to control the variables and colors of the hypotrochoids as well as the background color.
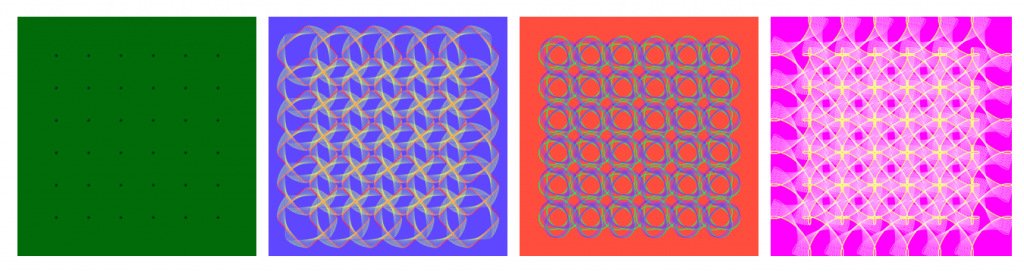
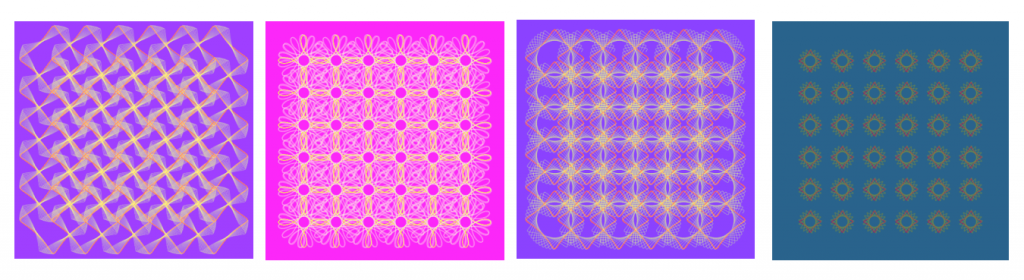