I wanted to create a portrait that used random points from the canvas to generate the image, while being modified by the cursor position.
sketch
let img;
let thin, thick;
function preload() {
img = loadImage('https://i.imgur.com/l7MihtV.jpg');
}
function setup() {
createCanvas(536, 600);
thin = 5;
thick = 45;
imageMode(CENTER);
noStroke();
background(255);
img.loadPixels();
}
function draw() {
//Maps mouseX and mouseY to 5 and 45 to scale both dimensions of the rectangle
let sizeX = map(mouseX, 0, width, thin, thick);
let sizeY = map(mouseY, 0, height, thin, thick)
//Picks random points from the image and stores them in x and y
let x = floor(random(img.width));
let y = floor(random(img.height));
//Gets the exact pixel
let pixel = img.get(x, y);
//Draws rectangle according to randomly chosen position
//and cursor position
fill(pixel, 128);
rect(x, y, sizeX, sizeY);
}
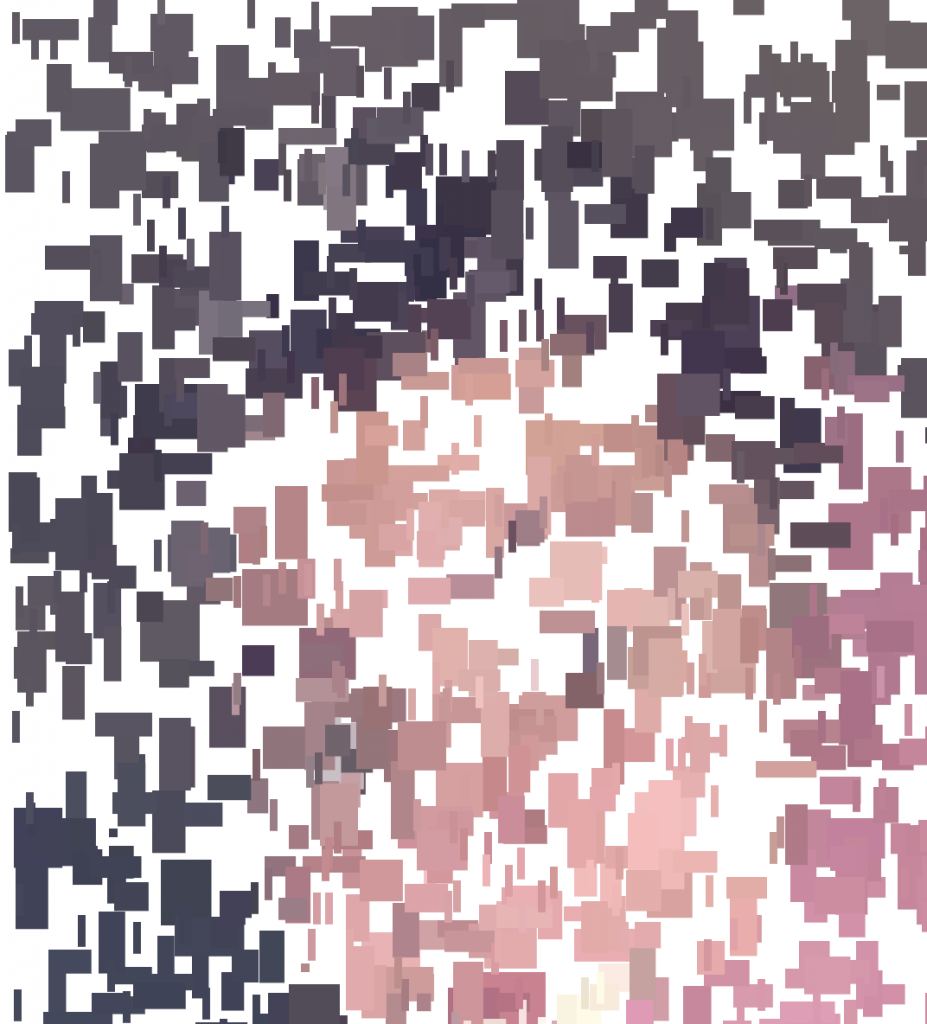
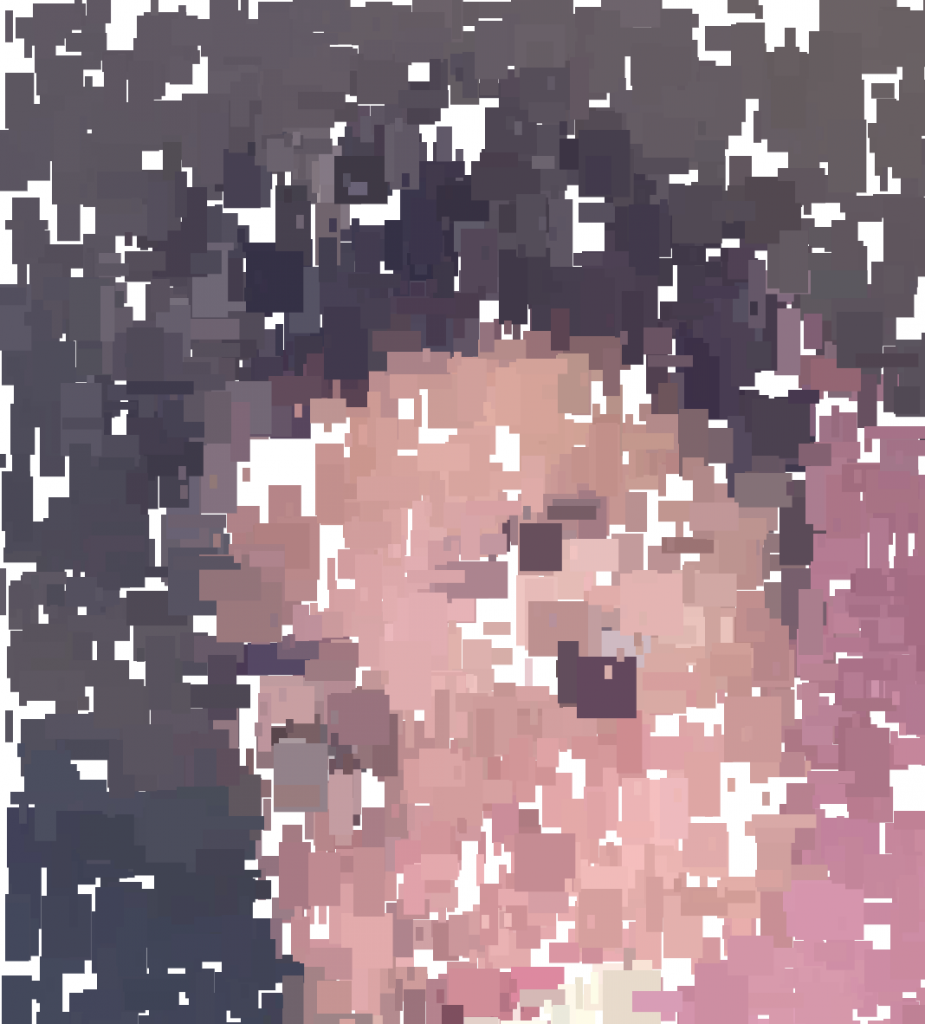
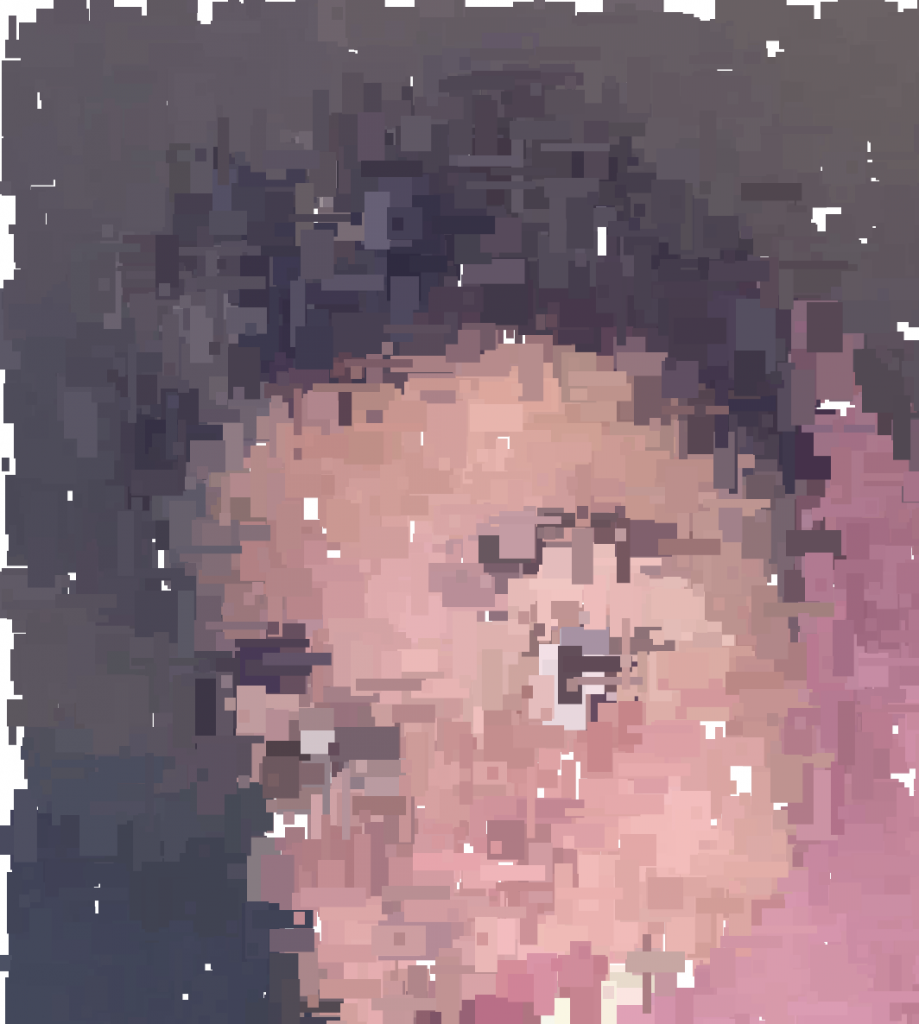
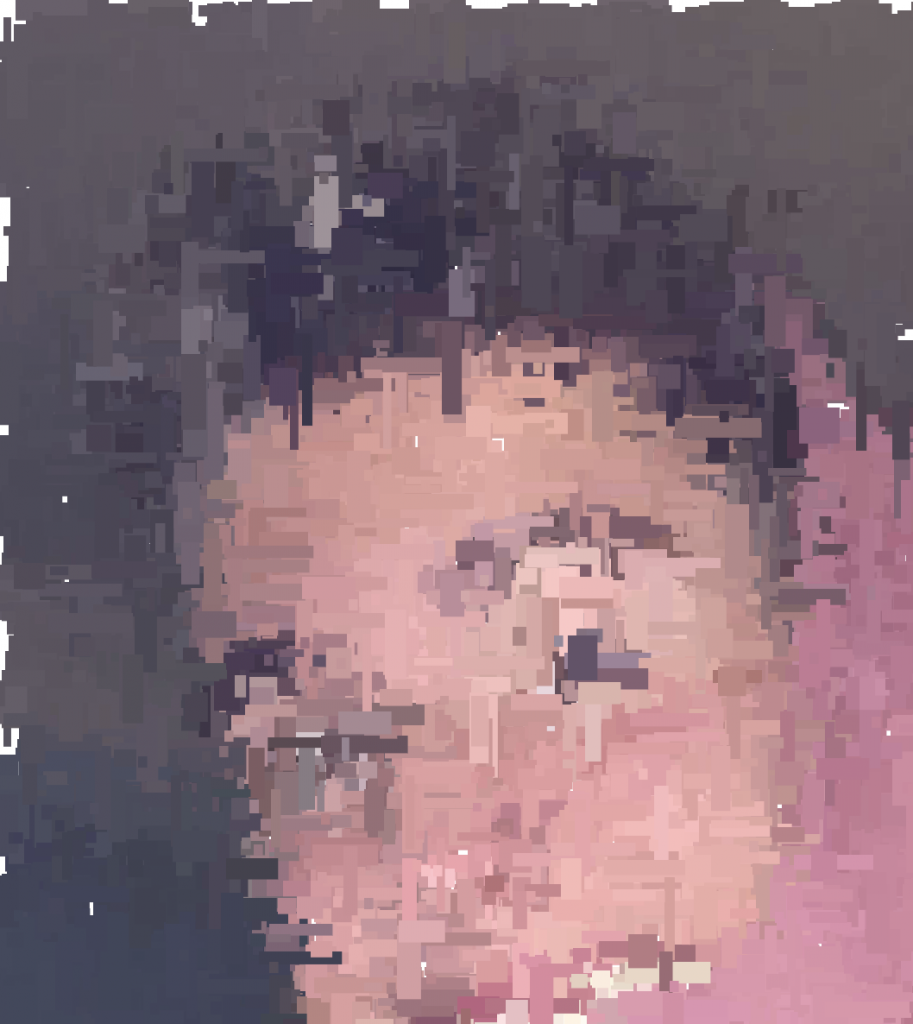
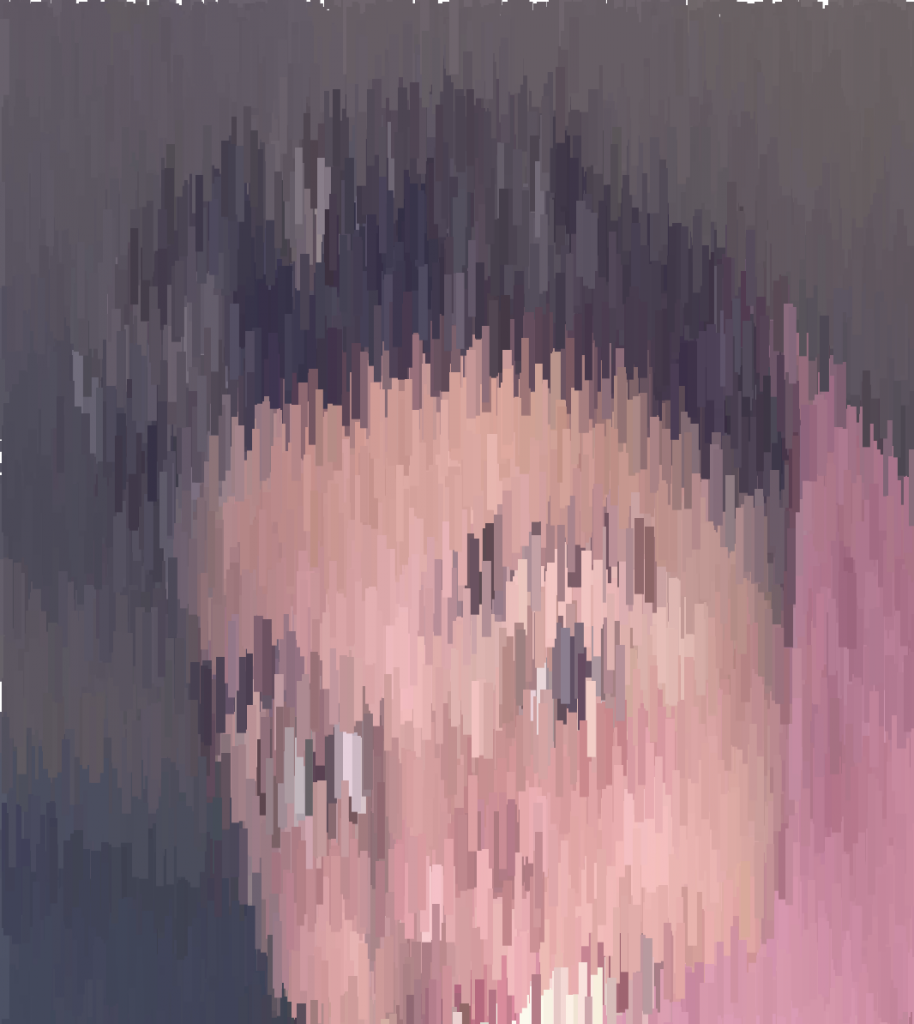
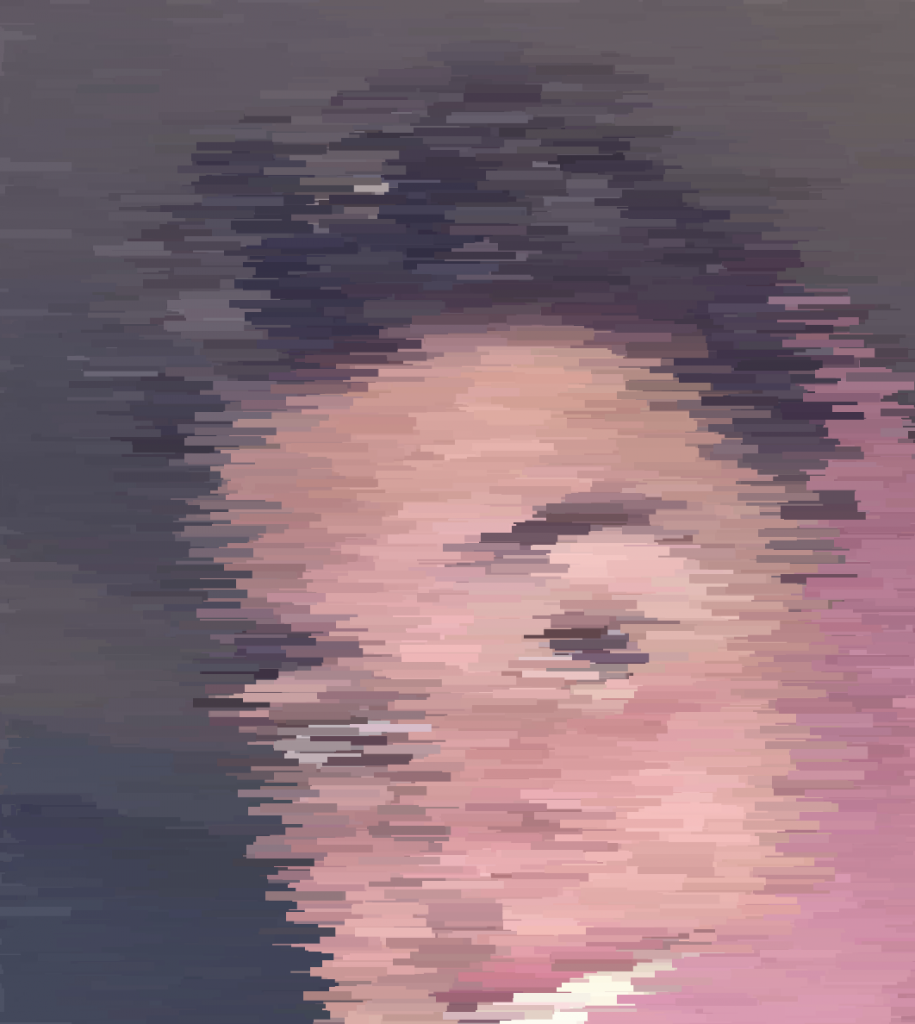
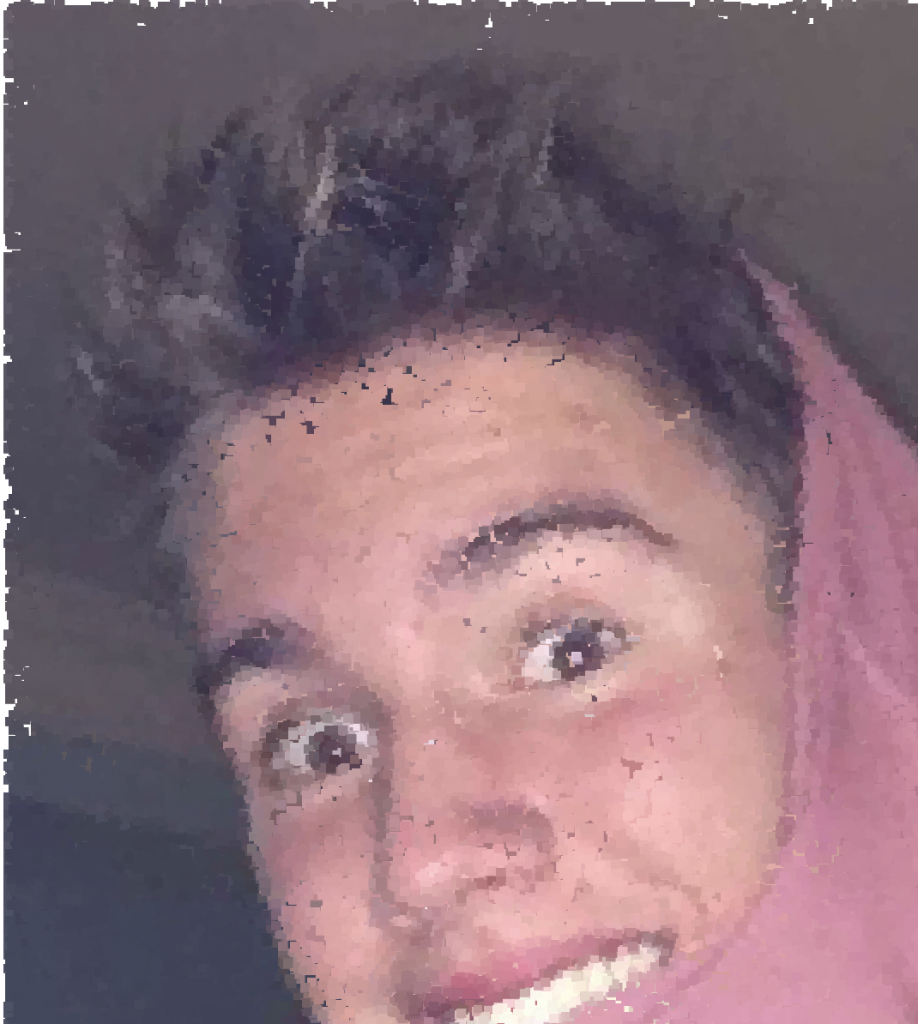